什么是Chrome Push API?
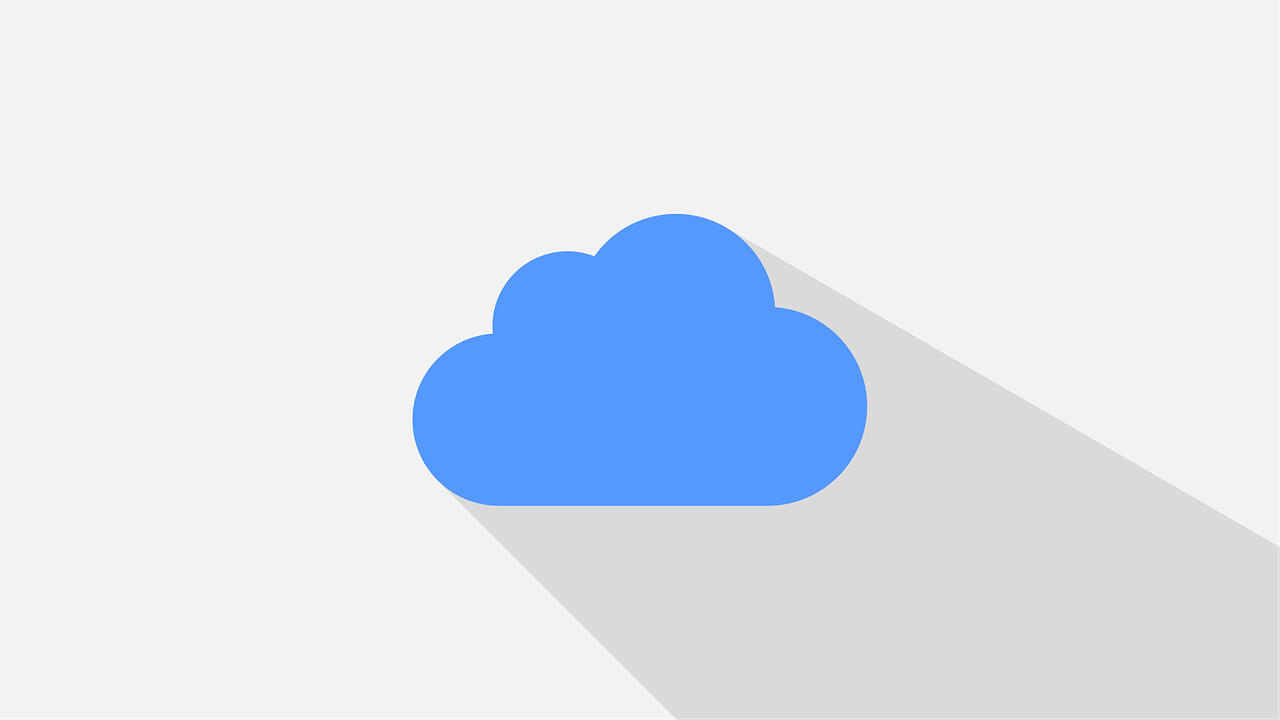
Chrome Push API是一种允许网页应用在用户关闭浏览器甚至设备后,通过推送服务(如Firebase Cloud Messaging)向用户发送通知的技术,它主要依赖于Service Worker、Push API和Notifications API这三个Web API。
技术分析
1、Service Worker:这是一个运行在后台的脚本,不依赖于页面加载状态,负责接收推送消息并在适当的时候显示。
2、Push API:允许Web应用订阅推送服务,接收服务器推送的数据。
3、Notifications API:提供了一种创建和管理桌面通知的方法,确保用户能够注意到重要的信息。
应用场景
新闻聚合器:及时推送最新资讯。
社交媒体:更新好友动态。
在线购物平台:促销活动或订单状态更新提醒。
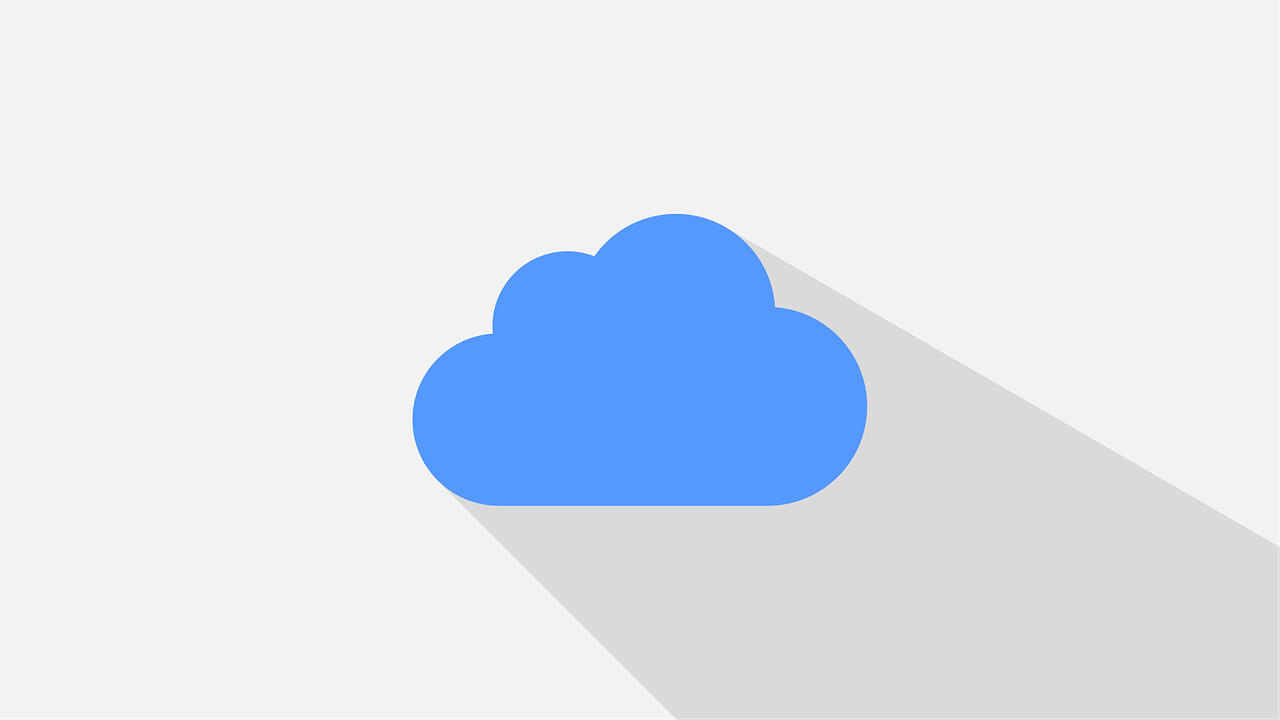
游戏:任务提醒、等级提升等游戏内通知。
项目特点
易学易用:教程结构清晰,逐步指导,适合初学者和有一定基础的开发者。
实战演练:不是纯理论讲解,而是通过实践让你理解每个步骤的含义。
跨平台兼容:支持多种现代浏览器,包括Chrome、Firefox等。
开源:项目源代码完全开放,可以自由查看和贡献。
如何实现Chrome Push API?
以下是一个简单的示例,展示了如何使用Chrome Push API实现Web应用中的推送通知功能。
配置Service Worker
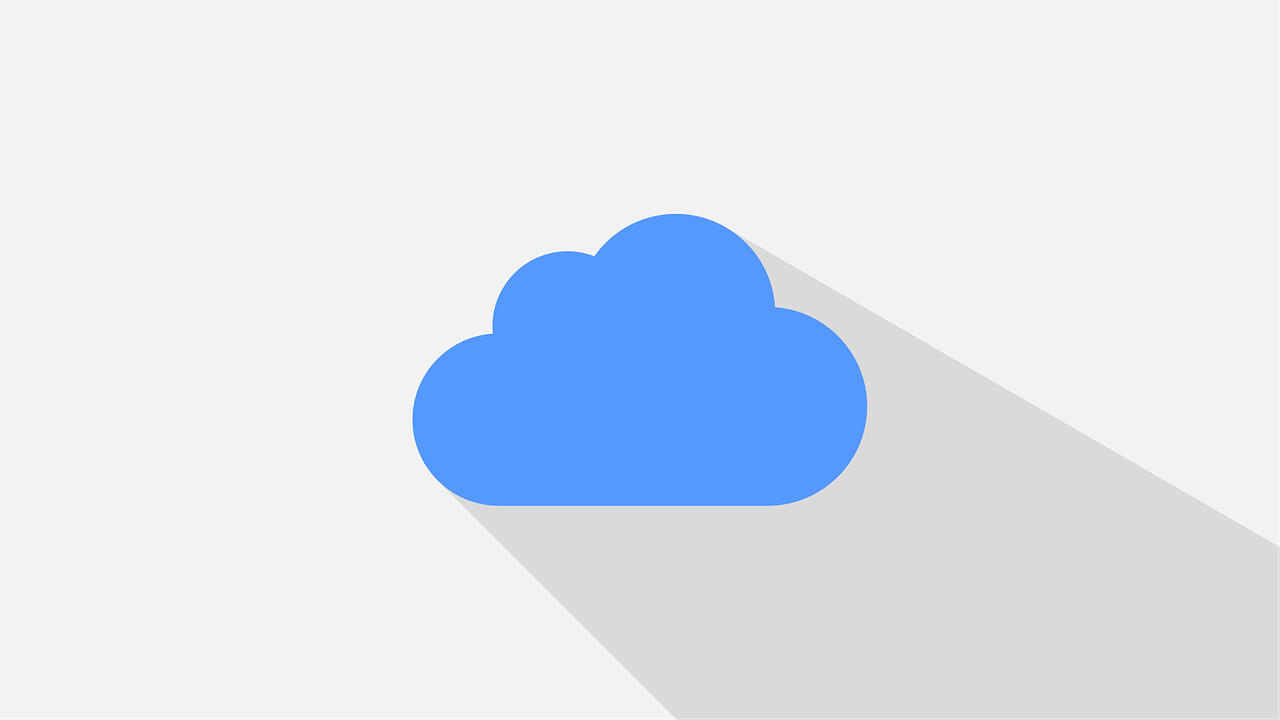
需要配置一个Service Worker,它将处理推送消息的接收和显示。
// sw.js self.addEventListener('push', function(event) { const title = 'New Message'; const options = { body: 'You have a new message.', }; event.waitUntil( self.registration.showNotification(title, options) ); });
实现推送订阅逻辑
在主线程中,添加以下代码以请求用户订阅推送通知,并在成功订阅后将订阅信息发送到服务器。
<!DOCTYPE html> <html> <head> <title>Chrome Push API</title> </head> <body> <script> if ('serviceWorker' in navigator && 'PushManager' in window) { navigator.serviceWorker.register('/sw.js').then(function(registration) { console.log('Service Worker registered with scope:', registration.scope); Subscription.request({ userVisibleOnly: true, applicationServerKey: urlBase64ToUint8Array('YOUR_PUBLIC_KEY') }).then(function(subscription) { console.log('User is subscribed:', subscription); fetch('/subscribe', { method: 'POST', headers: { 'Content-Type': 'application/json', 'Accept': 'application/json' }, body: JSON.stringify(subscription) }).then(function(response) { if (!response.ok) { throw new Error('Bad status code from server.'); } return response.json(); }).then(function(responseData) { if (!responseData.success) { throw new Error('Bad response from server.'); } }).catch(function(err) { console.error('Failed to send subscription to server:', err); }); }).catch(function(e) { if (Notification.permission === 'denied') { console.warn('Permission for notifications was denied'); } else { console.error('Unable to subscribe to push:', e); } }); }).catch(function(error) { console.error('Service Worker Error', error); }); } </script> </body> </html>
处理推送数据
在服务器端,你需要处理来自客户端的订阅请求,并将订阅信息存储起来,当有新消息需要推送时,使用存储的订阅信息将消息发送给客户端。
示例:使用Flask框架处理订阅请求 from flask import Flask, request, jsonify import json app = Flask(__name__) @app.route('/subscribe', methods=['POST']) def subscribe(): subscription = request.get_json() print('Received subscription:', subscription) # TODO: Store the subscription information in your database return jsonify({'success': True}), 201
创建和展示通知
当服务器端收到需要推送的消息时,使用存储的订阅信息将消息发送给客户端,客户端的Service Worker将接收到消息并展示通知。
示例:使用Flask框架发送推送消息 from flask import Flask, request, jsonify import requests import json app = Flask(__name__) VAPID_PUBLIC_KEY = 'YOUR_PUBLIC_KEY' VAPID_PRIVATE_KEY = 'YOUR_PRIVATE_KEY' FCM_URL = 'https://fcm.googleapis.com/fcm/send' headers = { 'Authorization': f'key={VAPID_PRIVATE_KEY}', 'Content-Type': 'application/json' } def send_push_notification(subscription_info, message): payload = { "to": subscription_info['endpoint'], "auth": VAPID_PUBLIC_KEY, "data": { "message": message, } } response = requests.post(FCM_URL, headers=headers, data=json.dumps(payload)) return response.status_code, response.text @app.route('/notify', methods=['POST']) def notify(): data = request.get_json() subscription_info = data['subscription'] message = data['message'] status_code, response_text = send_push_notification(subscription_info, message) return jsonify({'status': status_code, 'response': response_text}), status_code
FAQs
Q1: Chrome Push API与Web Push协议有什么关系?
A1: Chrome Push API是Web Push协议的一个实现,Web Push协议是一个标准,定义了客户端如何订阅推送服务以及服务器如何通过推送服务向客户端发送消息,Chrome Push API是Chrome浏览器对这一标准的实现。
Q2: Chrome Push API是否支持所有浏览器?
A2: Chrome Push API是专为Chrome浏览器设计的,但Web Push协议本身是跨浏览器的标准,其他浏览器也有自己的实现方式,例如Firefox使用的是Service Workers和Web Push API的组合,虽然Chrome Push API特定于Chrome,但Web Push的概念和部分实现在其他浏览器中也能找到类似的功能。
到此,以上就是小编对于“chrome push api”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1410237.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复