创建数组的组合文字
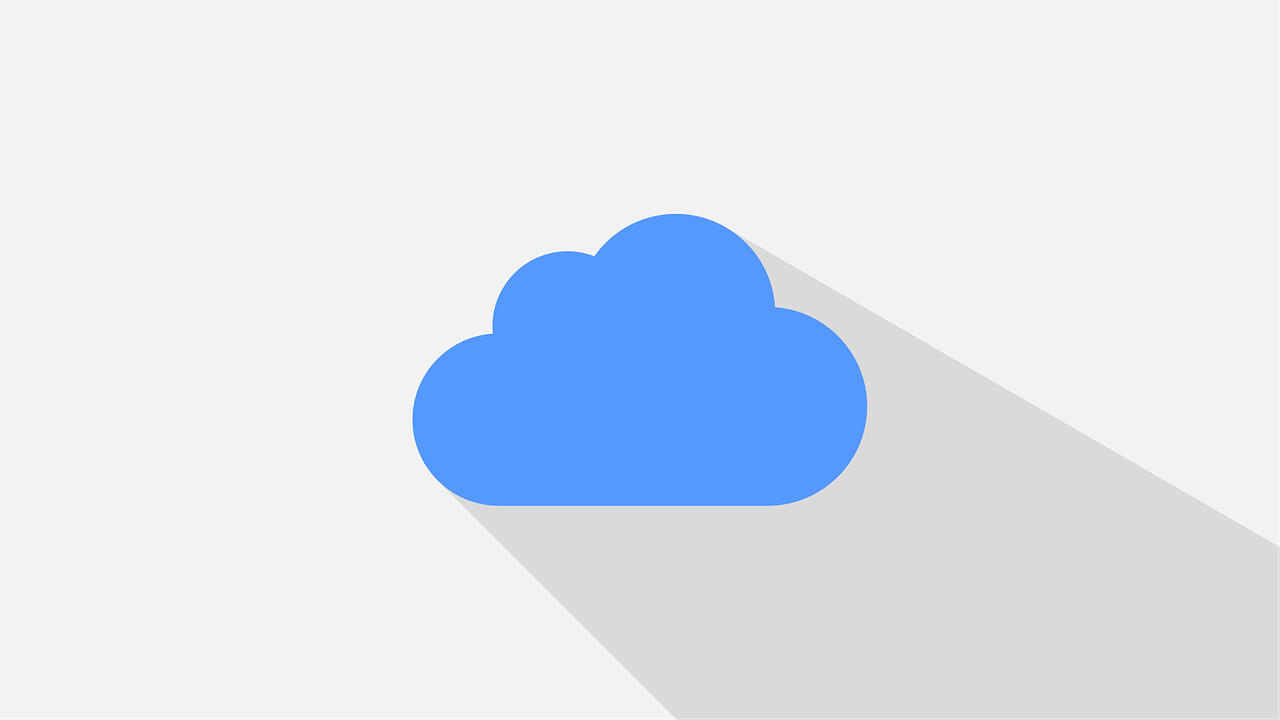
在编程中,数组是一种非常常用的数据结构,它可以存储多个相同类型的元素,而组合文字则是一种将多个字符串拼接在一起的操作,本文将详细介绍如何在不同编程语言中创建数组并进行组合文字的操作。
Python中的数组和组合文字
Python是一种流行的高级编程语言,它提供了丰富的内置函数和方法来处理数组和字符串,在Python中,可以使用列表(list)来表示数组,使用加号(+)运算符或者join()
方法来进行字符串拼接。
1、创建数组:
创建一个包含多个字符串的列表 str_list = ["Hello", "World", "from", "Python"]
2、组合文字:
使用加号运算符进行字符串拼接 combined_str1 = str_list[0] + " " + str_list[1] + " " + str_list[2] + " " + str_list[3] print(combined_str1) # 输出: Hello World from Python 使用join()方法进行字符串拼接 combined_str2 = " ".join(str_list) print(combined_str2) # 输出: Hello World from Python
Java中的数组和组合文字
Java是一种静态类型的编程语言,它提供了String
类来处理字符串,以及ArrayList
类来处理动态数组,在Java中,可以使用+
运算符或者StringBuilder
类来进行字符串拼接。
1、创建数组:
import java.util.ArrayList; import java.util.Arrays; public class Main { public static void main(String[] args) { // 创建一个包含多个字符串的ArrayList ArrayList<String> strList = new ArrayList<>(Arrays.asList("Hello", "World", "from", "Java")); } }
2、组合文字:
import java.util.ArrayList; import java.util.Arrays; public class Main { public static void main(String[] args) { ArrayList<String> strList = new ArrayList<>(Arrays.asList("Hello", "World", "from", "Java")); // 使用加号运算符进行字符串拼接 String combinedStr1 = strList.get(0) + " " + strList.get(1) + " " + strList.get(2) + " " + strList.get(3); System.out.println(combinedStr1); // 输出: Hello World from Java // 使用StringBuilder进行字符串拼接 StringBuilder sb = new StringBuilder(); for (String str : strList) { sb.append(str).append(" "); } String combinedStr2 = sb.toString().trim(); // 去掉最后一个空格 System.out.println(combinedStr2); // 输出: Hello World from Java } }
JavaScript中的数组和组合文字
JavaScript是一种轻量级的脚本语言,它内置了数组(array)和字符串(string)对象,在JavaScript中,可以使用concat()
方法或者模板字面量来进行字符串拼接。
1、创建数组:
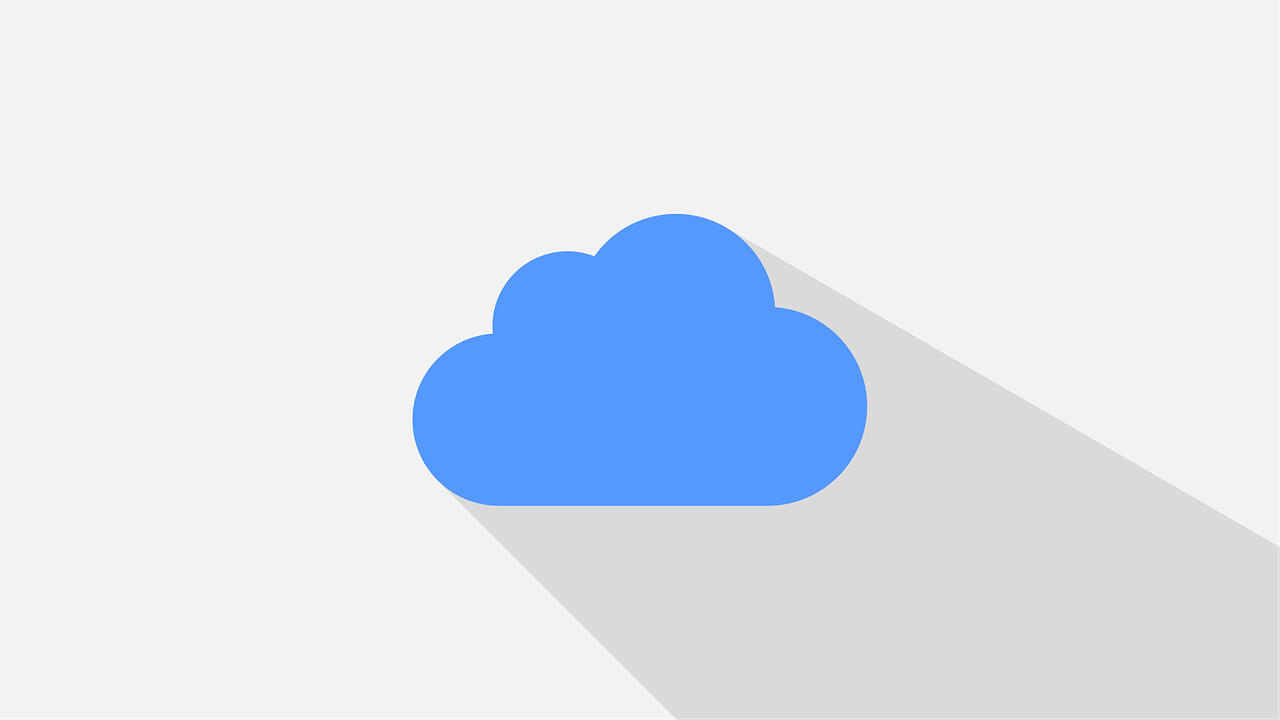
// 创建一个包含多个字符串的数组 let strArray = ["Hello", "World", "from", "JavaScript"];
2、组合文字:
// 使用concat()方法进行字符串拼接
let combinedStr1 = strArray.join(" ");
console.log(combinedStr1); // 输出: Hello World from JavaScript
// 使用模板字面量进行字符串拼接
let combinedStr2 =${strArray[0]} ${strArray[1]} ${strArray[2]} ${strArray[3]}
;
console.log(combinedStr2); // 输出: Hello World from JavaScript
C#中的数组和组合文字
C#是一种面向对象的编程语言,它提供了string
类型来处理字符串,以及List<T>
泛型类来处理动态数组,在C#中,可以使用加号(+)运算符或者String.Join()
方法来进行字符串拼接。
1、创建数组:
using System; using System.Collections.Generic; class Program { static void Main() { // 创建一个包含多个字符串的List List<string> strList = new List<string>{"Hello", "World", "from", "C#"}; } }
2、组合文字:
using System; using System.Collections.Generic; class Program { static void Main() { List<string> strList = new List<string>{"Hello", "World", "from", "C#"}; // 使用加号运算符进行字符串拼接 string combinedStr1 = strList[0] + " " + strList[1] + " " + strList[2] + " " + strList[3]; Console.WriteLine(combinedStr1); // 输出: Hello World from C# // 使用String.Join()方法进行字符串拼接 string combinedStr2 = string.Join(" ", strList); Console.WriteLine(combinedStr2); // 输出: Hello World from C# } }
本文介绍了在不同编程语言中创建数组并进行组合文字的操作,通过对比不同语言的实现方式,我们可以看到每种语言都有其特点和优势,在实际开发中,可以根据项目需求和个人喜好选择合适的编程语言和技术栈。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1406797.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复