在现代网页开发中,JavaScript 扮演着至关重要的角色,而在浏览器环境中,Chrome 是广泛使用的浏览器之一,本文将深入探讨如何在 Chrome 中使用 JavaScript 实现复制功能,包括文本复制、文件复制等不同场景。
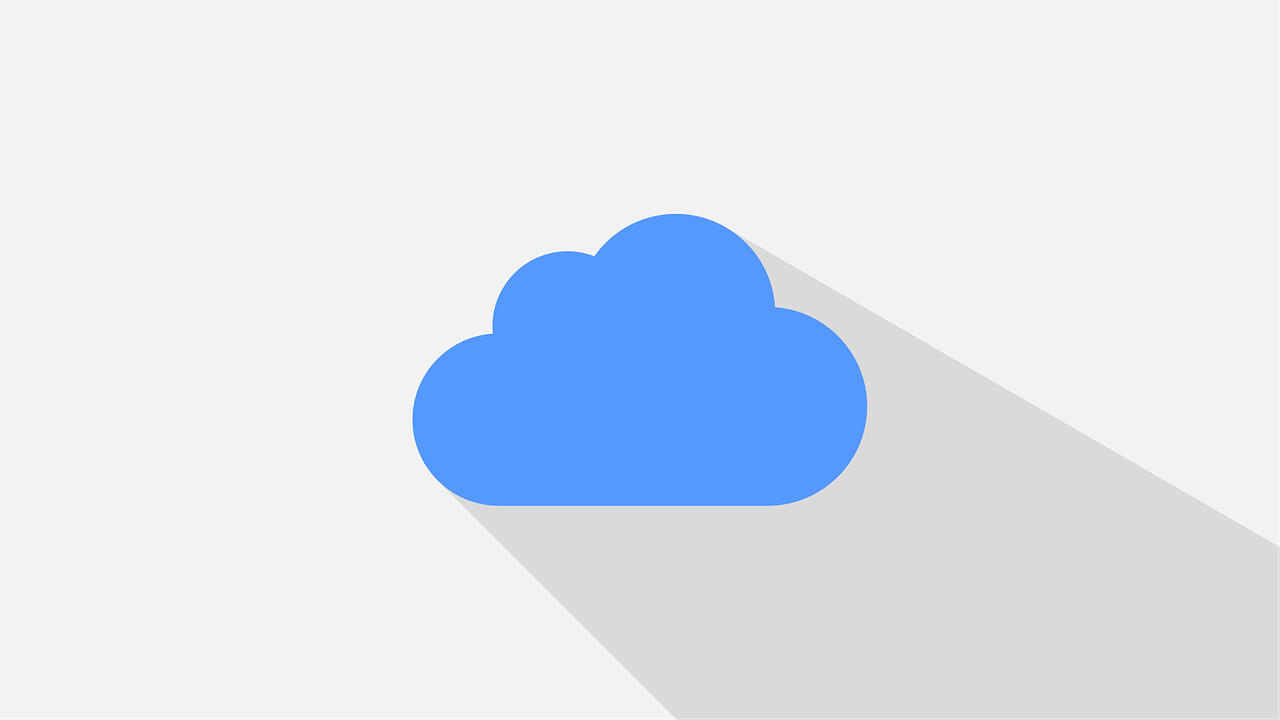
一、文本复制
1、简单文本复制
使用document.execCommand('copy')
方法可以简单地复制选定的文本,首先需要通过document.querySelector
或getElementById
等方法获取要复制的文本元素,然后调用select()
方法选中文本,最后执行document.execCommand('copy')
即可完成复制操作。
示例代码:
const textToCopy = document.getElementById('text'); textToCopy.select(); document.execCommand('copy'); alert('文本已复制');
2、动态文本复制
如果需要复制动态生成的文本,可以在合适的时机(如按钮点击事件)触发复制操作。
示例代码:
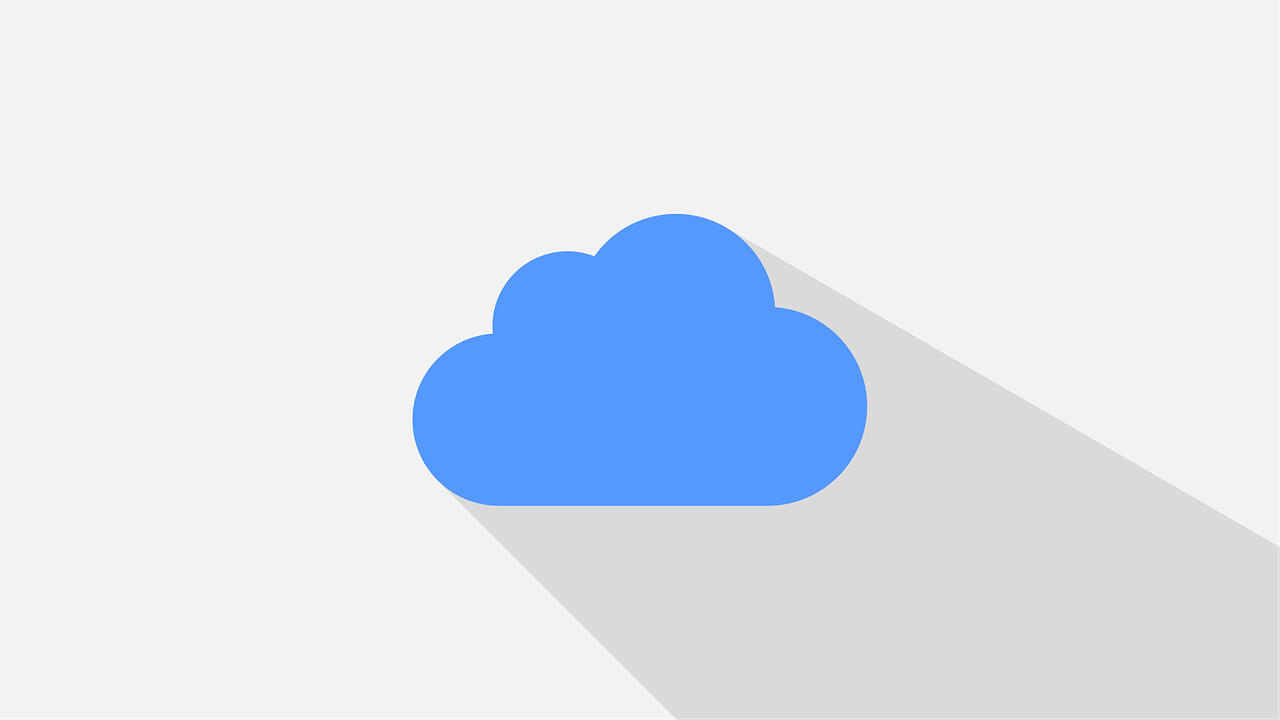
document.getElementById('copyButton').addEventListener('click', function() { const dynamicText = '这是动态生成的文本'; const tempInput = document.createElement('textarea'); document.body.appendChild(tempInput); tempInput.value = dynamicText; tempInput.select(); document.execCommand('copy'); document.body.removeChild(tempInput); alert('动态文本已复制'); });
二、文件复制
1、本地文件复制
可以使用 File API 读取本地文件内容,然后将其复制到剪贴板。
示例代码:
document.getElementById('fileInput').addEventListener('change', function(event) { const file = event.target.files[0]; const reader = new FileReader(); reader.onload = function(e) { const content = e.target.result; const tempInput = document.createElement('textarea'); document.body.appendChild(tempInput); tempInput.value = content; tempInput.select(); document.execCommand('copy'); document.body.removeChild(tempInput); alert('文件内容已复制'); }; reader.readAsText(file); });
2、远程文件复制
通过 fetch 获取远程文件内容,再进行复制。
示例代码:
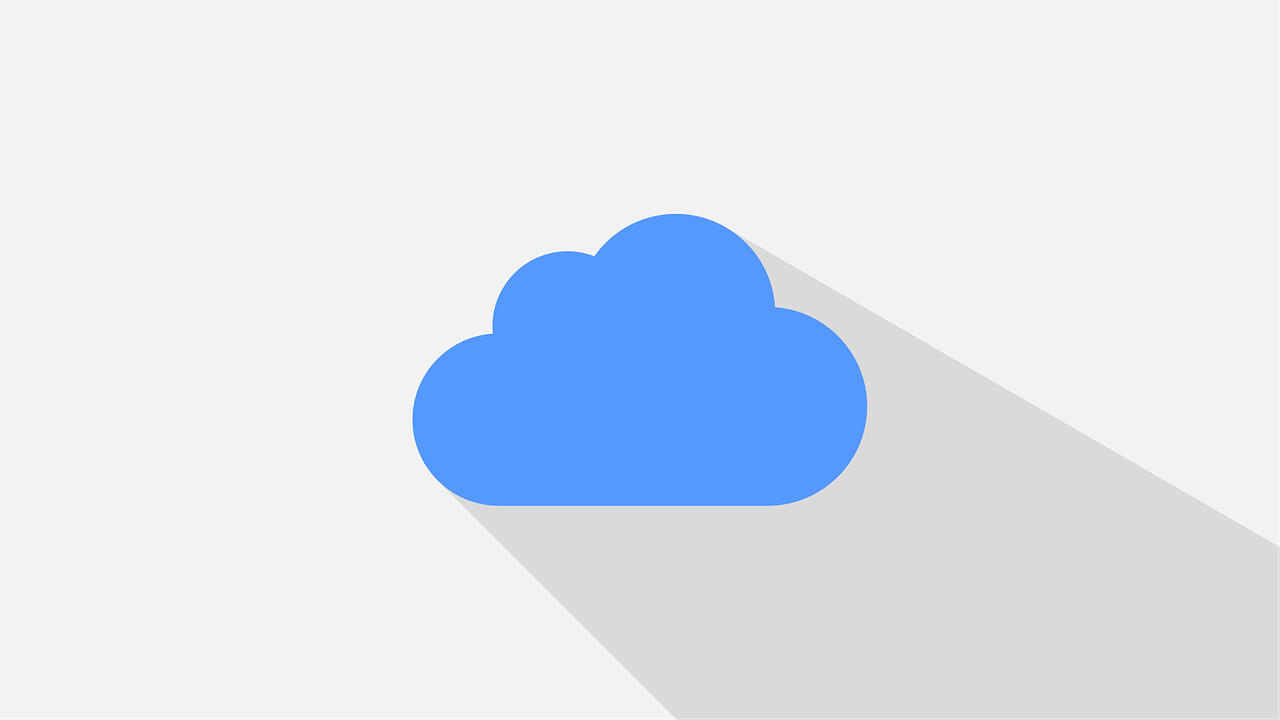
fetch('https://example.com/file.txt') .then(response => response.text()) .then(content => { const tempInput = document.createElement('textarea'); document.body.appendChild(tempInput); tempInput.value = content; tempInput.select(); document.execCommand('copy'); document.body.removeChild(tempInput); alert('远程文件内容已复制'); }) .catch(error => console.error('Error:', error));
三、复制到剪贴板的注意事项
1、兼容性问题
document.execCommand('copy')
方法在某些浏览器或特定情况下可能不被支持,需要进行兼容性处理,例如提供替代方案或提示用户。
2、安全性考虑
当复制敏感信息(如密码、个人信息等)时,需要注意数据的安全性和隐私保护,避免在不必要的情况下将敏感信息暴露在剪贴板上。
3、用户体验
在进行复制操作时,可以通过提示信息(如弹窗、通知等)告知用户复制成功或失败,提升用户体验。
四、常见问题解答
问题 1:为什么使用document.execCommand('copy')
没有反应?
答:可能有以下原因:
浏览器不支持该方法,可以尝试使用其他兼容的方法或库来实现复制功能。
没有正确选中要复制的内容,确保在调用document.execCommand('copy')
之前,已经通过select()
方法选中了文本。
存在安全限制,某些浏览器可能会对剪贴板操作进行限制,以确保用户数据的安全,在这种情况下,可以尝试使用其他方式来实现复制功能。
问题 2:如何复制对象到剪贴板?
答:可以将对象转换为字符串或 JSON 格式,然后再进行复制。
const obj = {name: 'John', age: 30}; const jsonString = JSON.stringify(obj); const tempInput = document.createElement('textarea'); document.body.appendChild(tempInput); tempInput.value = jsonString; tempInput.select(); document.execCommand('copy'); document.body.removeChild(tempInput); alert('对象已复制');
这样,对象就被复制到了剪贴板上,可以在需要的时候进行粘贴和解析。
在 Chrome 中使用 JavaScript 实现复制功能可以为网页开发带来更多的便利和交互性,根据具体的需求和场景,可以选择不同的方法和技巧来实现复制操作,要注意兼容性、安全性和用户体验等方面的问题,以确保功能的稳定和可靠。
以上就是关于“chrome js copy”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1406250.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复