创建一个图书管理数据库的JSP(JavaServer Pages)应用程序是一个多步骤的过程,涉及数据库设计、后端逻辑编写以及前端页面展示,下面将详细介绍如何实现这一过程。
一、数据库设计
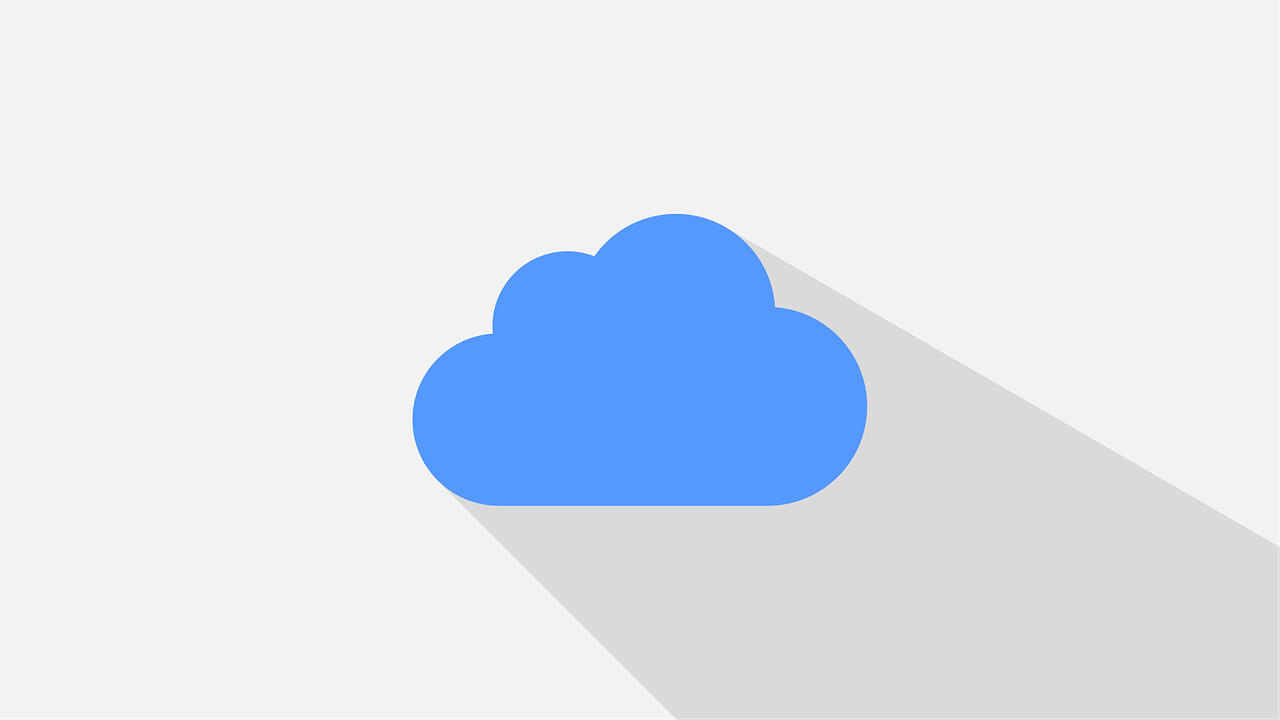
我们需要在MySQL等关系型数据库中创建图书管理所需的表结构,假设我们使用MySQL数据库,可以按照以下步骤进行:
1、安装并配置MySQL:确保你的开发环境中已经安装了MySQL,并且能够通过命令行或图形化工具访问。
2、创建数据库:打开MySQL命令行或使用图形化工具,执行以下SQL语句来创建一个名为library
的数据库:
CREATE DATABASE library; USE library;
3、创建图书信息表:在library
数据库中创建一个名为books
的表,用于存储图书信息,可以使用以下SQL语句:
CREATE TABLE books ( book_id INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(255) NOT NULL, author VARCHAR(255) NOT NULL, publish_date DATE, isbn VARCHAR(20), genre VARCHAR(100), price DECIMAL(10, 2), quantity INT );
二、搭建JSP开发环境
为了开发JSP应用程序,你需要配置一个支持JSP的Web服务器,如Apache Tomcat,以下是基本的配置步骤:
1、下载并安装JDK:确保你的系统中安装了Java Development Kit (JDK)。
2、下载并安装Tomcat:从Apache Tomcat官方网站下载适合你操作系统的版本,并按照说明进行安装。
3、配置Tomcat环境变量:将Tomcat的bin
目录添加到系统的PATH
环境变量中,以便于在命令行中直接运行Tomcat。
4、部署JSP项目:将你的JSP文件和相关资源放置在Tomcat的webapps
目录下,或者将它们打包为WAR文件后放置在该目录下。
三、编写JSP代码
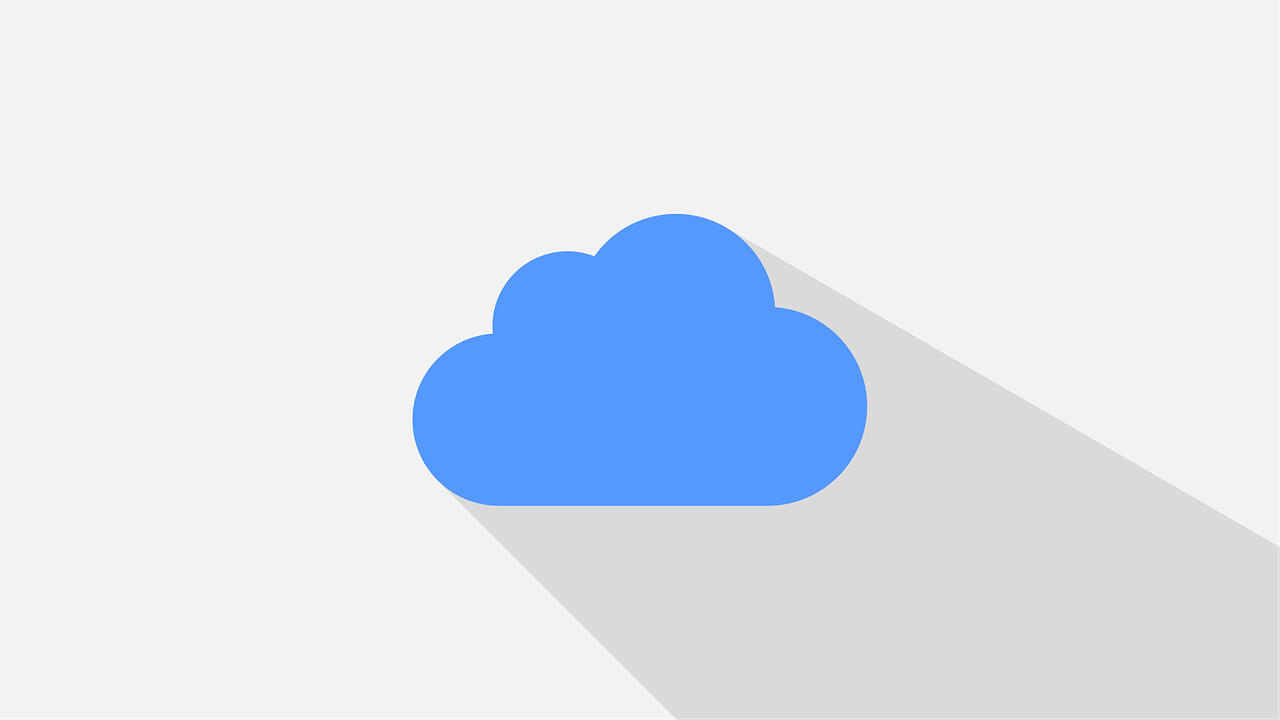
我们将编写几个简单的JSP页面来实现图书管理的基本功能,包括添加图书、查看图书列表和删除图书。
1. 添加图书页面(add_book.jsp)
<!DOCTYPE html> <html> <head> <title>添加图书</title> </head> <body> <h2>添加新图书</h2> <form action="add_book_action.jsp" method="post"> 书名: <input type="text" name="title"><br> 作者: <input type="text" name="author"><br> 出版日期: <input type="text" name="publish_date" placeholder="YYYY-MM-DD"><br> ISBN: <input type="text" name="isbn"><br> 类型: <input type="text" name="genre"><br> 价格: <input type="text" name="price"><br> 库存量: <input type="text" name="quantity"><br> <input type="submit" value="添加"> </form> </body> </html>
2. 添加图书动作页面(add_book_action.jsp)
<%@ page import="java.sql.*" %> <%@ page import="javax.servlet.*" %> <%@ page import="javax.servlet.http.*" %> <% String title = request.getParameter("title"); String author = request.getParameter("author"); String publish_date = request.getParameter("publish_date"); String isbn = request.getParameter("isbn"); String genre = request.getParameter("genre"); String priceStr = request.getParameter("price"); String quantityStr = request.getParameter("quantity"); double price = Double.parseDouble(priceStr); int quantity = Integer.parseInt(quantityStr); try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/library", "root", "password"); PreparedStatement pstmt = con.prepareStatement("INSERT INTO books (title, author, publish_date, isbn, genre, price, quantity) VALUES (?, ?, ?, ?, ?, ?, ?)"); pstmt.setString(1, title); pstmt.setString(2, author); pstmc.setDate(3, Date.valueOf(publish_date)); // Assumes the date is in YYYY-MM-DD format pstmt.setString(4, isbn); pstmt.setString(5, genre); pstmt.setDouble(6, price); pstmt.setInt(7, quantity); int result = pstmt.executeUpdate(); if (result > 0) { out.println("<h2>图书添加成功!</h2>"); } else { out.println("<h2>图书添加失败,请重试。</h2>"); } } catch (Exception e) { e.printStackTrace(); out.println("<h2>发生错误:" + e.getMessage() + "</h2>"); } %>
3. 查看图书列表页面(view_books.jsp)
<!DOCTYPE html> <html> <head> <title>查看图书列表</title> </head> <body> <h2>图书列表</h2> <table border="1"> <tr> <th>书名</th> <th>作者</th> <th>出版日期</th> <th>ISBN</th> <th>类型</th> <th>价格</th> <th>库存量</th> <th>操作</th> </tr> <%@ page import="java.sql.*" %> <%@ page import="javax.servlet.*" %> <%@ page import="javax.servlet.http.*" %> <% try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/library", "root", "password"); Statement stmt = con.createStatement(); ResultSet rs = stmt.executeQuery("SELECT * FROM books"); while (rs.next()) { out.println("<tr>"); out.println("<td>" + rs.getString("title") + "</td>"); out.println("<td>" + rs.getString("author") + "</td>"); out.println("<td>" + rs.getDate("publish_date") + "</td>"); out.println("<td>" + rs.getString("isbn") + "</td>"); out.println("<td>" + rs.getString("genre") + "</td>"); out.println("<td>" + rs.getDouble("price") + "</td>"); out.println("<td>" + rs.getInt("quantity") + "</td>"); out.println("<td><a href='delete_book_action.jsp?book_id=" + rs.getInt("book_id") + "'>删除</a></td>"); out.println("</tr>"); } rs.close(); stmt.close(); con.close(); } catch (Exception e) { e.printStackTrace(); out.println("<h2>发生错误:" + e.getMessage() + "</h2>"); } %> </table> </body> </html>
4. 删除图书动作页面(delete_book_action.jsp)
<%@ page import="java.sql.*" %> <%@ page import="javax.servlet.*" %> <%@ page import="javax.servlet.http.*" %> <% int bookId = Integer.parseInt(request.getParameter("book_id")); try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/library", "root", "password"); PreparedStatement pstmt = con.prepareStatement("DELETE FROM books WHERE book_id=?"); pstmt.setInt(1, bookId); int result = pstmt.executeUpdate(); if (result > 0) { response.sendRedirect("view_books.jsp"); } else { out.println("<h2>图书删除失败,请重试。</h2>"); } } catch (Exception e) { e.printStackTrace(); out.println("<h2>发生错误:" + e.getMessage() + "</h2>"); } %>
1. Q: 如何在JSP页面中显示数据库查询结果? A: 你可以在JSP页面中使用JSP标签和Java代码片段来执行SQL查询,并将结果输出到HTML表格中,在view_books.jsp
页面中使用了JSP脚本标签<% %>
来执行查询并遍历结果集。
2. Q: JSP页面中的异常如何处理? A: 在JSP页面中,你可以使用try-catch块来捕获和处理异常,当发生异常时,可以在catch块中打印错误消息或将用户重定向到一个错误页面。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1404291.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复