MySQL 创建存储过程及 MySQL 监控
一、MySQL 创建存储过程
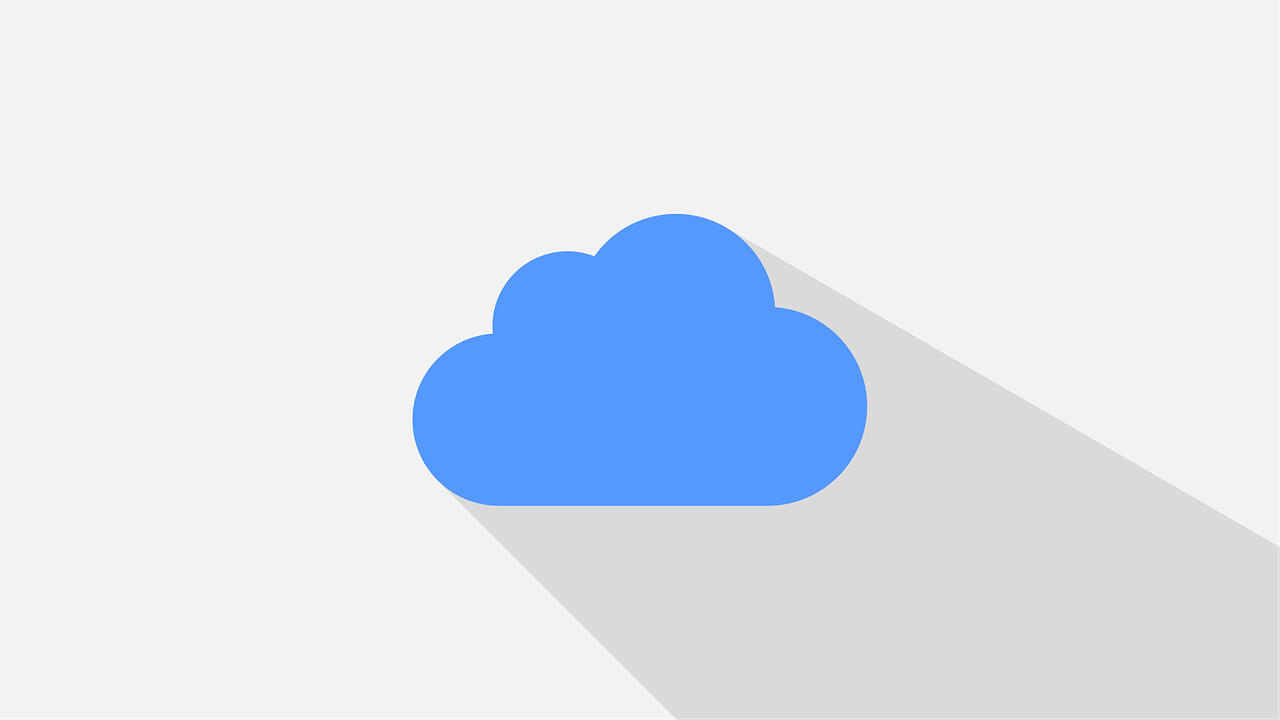
1. 前言知识
1.1 存储过程简介
存储过程(Stored Procedure)是一组预编译的 SQL 语句集合,用于完成特定的功能,它们可以接受参数、返回结果集,并包含控制逻辑,如条件判断和循环,使用存储过程可以提高代码的重用性和系统的执行效率。
1.2 存储过程的优点
提高效率:由于存储过程在数据库服务器上预编译,执行速度比直接执行 SQL 语句更快。
减少网络流量:存储过程封装了多条 SQL 语句,减少了客户端与服务器之间的通信次数。
增强安全性:通过权限控制,可以限制用户对某些数据表的直接访问,但允许他们执行存储过程。
简化维护:修改存储过程时,只需更新存储过程的定义,无需修改调用它的应用程序代码。
1.3 存储过程的缺点
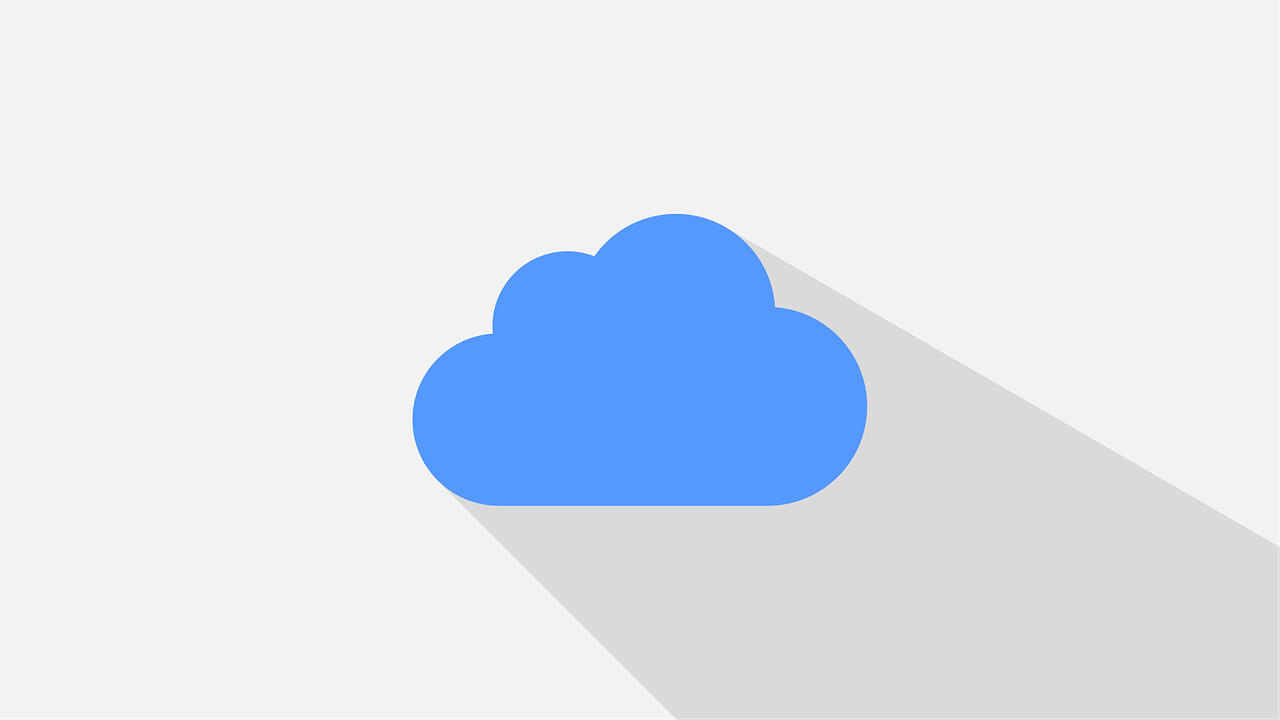
可移植性差:不同数据库系统之间的存储过程语法存在差异,不便于跨平台迁移。
调试困难:存储过程中的错误定位不如应用程序中的代码方便。
开发复杂:对于复杂的业务逻辑,编写和维护存储过程可能比较困难。
2. 创建存储过程的基本语法
DELIMITER // CREATE PROCEDURE procedure_name( [IN | OUT | INOUT] parameter_name datatype, ... ) BEGIN -存储过程体 END // DELIMITER ;
DELIMITER //
:更改默认的命令结束符,以便能够在存储过程中使用分号。
CREATE PROCEDURE
:创建存储过程的关键字。
procedure_name
:存储过程的名称。
[IN | OUT | INOUT] parameter_name datatype
:输入、输出或输入/输出参数及其数据类型。
BEGIN ... END
:存储过程的主体,包含要执行的 SQL 语句。
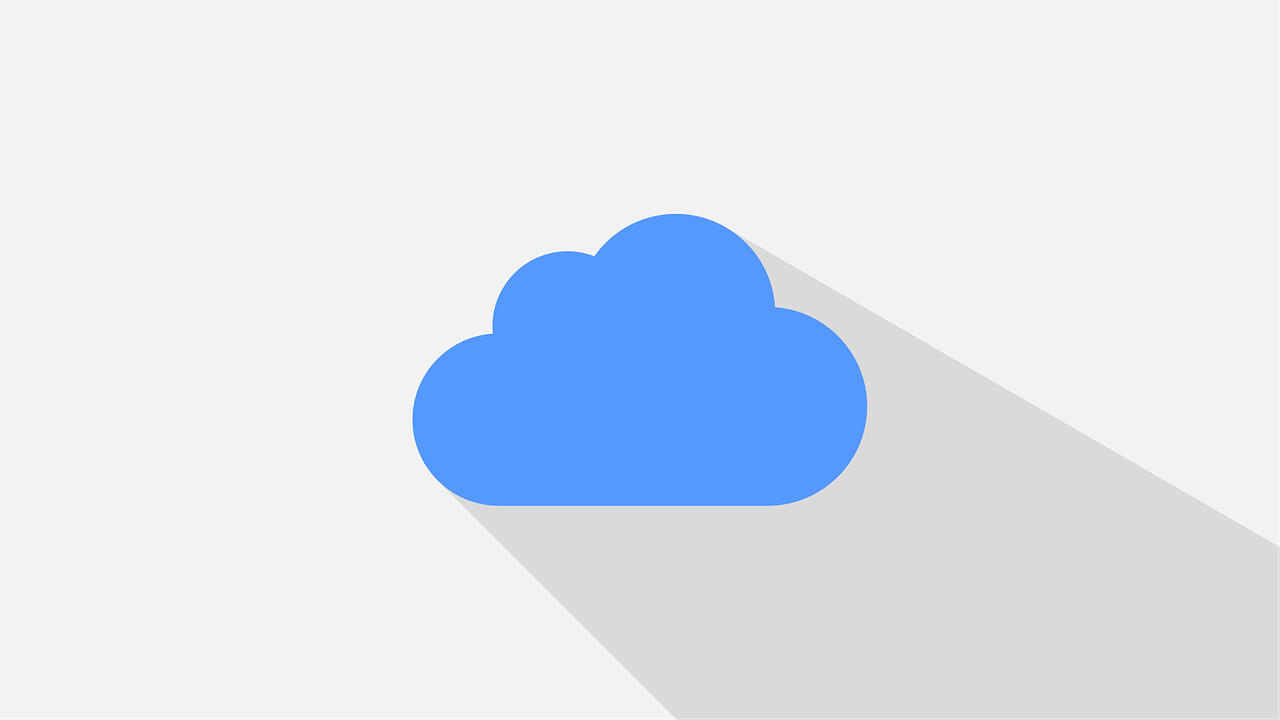
DELIMITER ;
:恢复默认的命令结束符。
3. 无参存储过程入门例子
DELIMITER $$ CREATE PROCEDURE sp_select_one_age_dog() BEGIN SELECT * FROM dog d WHERE d.dog_age <= 1; END$$ DELIMITER ;
这个简单的存储过程sp_select_one_age_dog
从dog
表中选择年龄小于等于 1 岁的狗狗的信息。
4. 带变量的存储过程示例
DELIMITER $$ CREATE PROCEDURE sp_test() BEGIN DECLARE col_test VARCHAR(20); SET col_test = 'test'; SELECT col_test; END$$ DELIMITER ;
这个存储过程声明了一个局部变量col_test
,赋值为'test'
,然后将其作为查询结果返回。
5. 有参存储过程示例
DELIMITER $$ CREATE PROCEDURE sp_select_dog_by_num(IN dogNum INT(10)) BEGIN SELECT d.dog_num, d.dog_name, d.dog_kind, d.dog_age FROM dog d WHERE d.dog_num = dogNum; END$$ DELIMITER ;
这个存储过程sp_select_dog_by_num
接受一个整数参数dogNum
,根据该参数从dog
表中选择相应的狗狗信息。
6. 出参存储过程示例
DELIMITER $$ CREATE PROCEDURE sp_test_out(OUT col_test varchar(20)) BEGIN SELECT 'test' INTO col_test; END$$ DELIMITER ;
这个存储过程使用OUT
参数col_test
,将字符串'test'
赋值给该参数,并在调用时返回结果。
7. 入参和出参的存储过程示例
DELIMITER $$ CREATE PROCEDURE sp_select_dogName_by_num(IN dogNum INT(10), OUT dogName VARCHAR(20)) BEGIN SELECT d.dog_name INTO dogName FROM dog d WHERE d.dog_num = dogNum; END$$ DELIMITER ;
这个存储过程接受一个输入参数dogNum
,并根据该参数从dog
表中选择狗狗的名字,通过OUT
参数dogName
返回。
8. inout的存储过程示例
DELIMITER $$ CREATE PROCEDURE sp_select_pId_by_deptId(INOUT v_code varchar(10)) BEGIN SELECT t.PARENT_ID into v_code FROM SYS_COMPANY_DEPT t where t. DEPT_ID = v_code; END$$ DELIMITER ;
这个存储过程使用INOUT
参数v_code
,根据部门 ID 查找父级 ID,并通过v_code
返回结果。
9. 实用存储过程例子:根据表名添加字段
DELIMITER $$ CREATE PROCEDURE AddColumnToTable(IN dbName VARCHAR(64), IN tableName VARCHAR(64), IN columnName VARCHAR(64), IN columnType VARCHAR(64)) BEGIN SET @sql = CONCAT('ALTER TABLE ', IFNULL(dbName, '') OR '', dbName, '
.', IFNULL(tableName, '') OR '', tableName, '
', ' ADD COLUMN ', IFNULL(columnName, '') OR '', columnName, '
', ' ', IFNULL(columnType, '')) OR '', columnType, '
'); PREPARE stmt FROM @sql; EXECUTE stmt; DEALLOCATE PREPARE stmt; END$$ DELIMITER ;
这个存储过程动态构建并执行ALTER TABLE
语句,向指定表中添加新列,调用方式如下:
CALL AddColumnToTable('your_database', 'your_table', 'new_column', 'VARCHAR(255)');
10.递归查询的存储过程示例
DELIMITER $$ CREATE PROCEDURE GetAllChildren(nodeId INT) BEGIN DECLARE done INT DEFAULT FALSE; DECLARE childId INT; DECLARE cur CURSOR FOR SELECT id FROM your_table WHERE parent_id = nodeId; DECLARE CONTINUE HANDLER FOR NOT FOUND SET done = TRUE; OPEN cur; read_loop: LOOP FETCH cur INTO childId; IF done THEN LEAVE read_loop; END IF; -处理子节点的逻辑,例如插入到临时表或进行其他操作 CALL GetAllChildren(childId); -递归调用自身获取子节点的子节点 END LOOP; CLOSE cur; END$$ DELIMITER ;
这个存储过程使用递归的方式查询所有子节点,适用于层次结构数据的遍历,如组织架构图或分类树等。
二、创建MySQL监控
1. 监控的重要性与目的
监控对于确保数据库系统的稳定性、性能优化以及及时发现和解决问题至关重要,有效的监控可以帮助识别潜在的瓶颈、异常行为以及安全威胁,从而保障业务的连续性和数据的安全性。
2. Prometheus + Grafana监控方案概览
Prometheus 是一个开源的监控和报警工具,擅长处理时间序列数据,而 Grafana 是一个强大的开源可视化平台,能够与 Prometheus 无缝集成,提供丰富的图表和仪表盘展示,结合两者可以实现全面的数据库监控解决方案。
3. Prometheus安装与配置基础步骤
下载并解压 Prometheus,编辑配置文件prometheus.yml
,设置好监控目标和报警规则,启动 Prometheus 服务后,可以通过浏览器访问其 Web 界面,查看监控数据和报警状态,具体步骤如下:
下载 Prometheus:https://prometheus.io/download/
解压并将文件移动到目标目录,例如/usr/local/prometheus
。
编辑配置文件prometheus.yml
,添加需要监控的目标和报警规则。
global: scrape_interval: 15s # By default, the scrape interval is set to every 15 seconds. evaluation_interval: 15s # By default, the evaluation interval is set to every 15 seconds. # scrape_timeout is set to the global default (10s). alerting: alertmanagers: static_configs: targets: ['localhost:9093'] rule_files: "first_rules.yml" # 包含报警规则的文件 scrape_configs: job_name: 'prometheus' static_configs: targets: ['localhost:9090'] # Prometheus自身的监控地址
启动 Prometheus:./prometheus --config.file=prometheus.yml
。
访问 Prometheus Web 界面:http://localhost:9090。
4. Grafana安装与配置基础步骤
下载并安装 Grafana,编辑配置文件grafana.ini
,设置数据源和用户权限,启动 Grafana 服务后,可以通过浏览器访问其 Web 界面,添加数据源和创建仪表盘,具体步骤如下:
下载 Grafana:https://grafana.com/grafana/download?platform=linux&plugin=mysql&plugin=zabbix&plugin=prometheus&plugin=jdbc&plugin=graphite&plugin=influxdb&plugin=opentsdb&plugin=elasticsearch&plugin=ksql&plugin=kinesis&plugin=cloudwatch&plugin=prometheus-alertmanager&plugin=loki-loki&plugin=azure-monitor&plugin=newrelic&plugin=datadoghq&plugin=snow&plugin=postgresql&plugin=timescaledb&plugin=vertica&plugin=snowflake&plugin=kafka&plugin=aerospike&plugin=redis&plugin=cassandra&plugin=mongodb&plugin=mysql&plugin=postgres&plugin=mssql&plugin=oracle&plugin=amazon_rds&plugin=tibco_ems&plugin=ibm_mq&plugin=informatica&plugin=google_ads&plugin=clickhouse&plugin=density&plugin=wavefront&plugin=riemann&plugin=sensu&plugin=smartos_monitor&plugin=victoriametrics&plugin=prometheeum&plugin=graphite_app&plugin=solarwinds&plugin=netdata&plugin=cloudflare&plugin=pingdom&plugin=pachubeified&plugin=signalfx-agent&plugin=newrelic_infra&plugin=aws-cloudwatch-exporter&plugin=azure-monitor-exporter&plugin=digitalocean-cloud-controller-manager&plugin=prometheus-operator-for-kubernetes&plugin=prometheus-operator-for-openshift&plugin=prometheus-operator-for-openstack&plugin=prometheus-operator-for-amazon-eks&plugin=prometheus-operator-for-google-gke&plugin=prometheus-operator-for-baidu-cloud&plugin=prometheus-operator-for-tencent-cloud&plugin=prometheus-operator-for-huawei-cloud&plugin=prometheus-operator-for-alibaba-cloud&plugin=prometheus-operator-for-qingcloud&plugin=prometheus-operator-for-azure-avs&plugin=prometheus-operator-for-google-cbs&plugin=prometheus-operator-for-tencent-tke&plugin=prometheus-operator-for-huawei-cse&plugin=prometheus-operator-for-amazon-cne&plugin=prometheus-operator-for-google-cne&plugin=prometheus-operator-for-baidu-bce&plugin=prometheus-operator-for-tencent-cynosdb&plugin=prometheus-operator-for-huawei-gaussdb&plugin=prometheus-operator-for-alibaba-drds&plugin=prometheus-operator-for-qingcloud-galaxy&plugin=prometheus-operator-for-azure-cosmosdb&plugin=prometheus-operator-for-google-bigquery&plugin=prometheus-operator-for-tencent-dcdb&plugin=prometheus-operator-for-huawei-gaussdb-liberty&plugin=prometheus-operator-for-alibaba-polardbpg&plugin=prometheus-operator-for-qingcloud-kotlin&plugin=prometheus-operator-for-azure-redis&plugin=prometheus-operator-for-google-redis&plugin=prometheus-operator-for-tencent-redis&plugin=prometheus-operator-for-huawei-redis&plugin=prometheus-operator-for-alibaba-redis&plugin=prometheus-operator-for-qingcloud-redis&plugin=prometheus-operator-for-aws-redis&plugin=prometheus-operator-for-azure-cache&plugin=prometheus-operator-for-google-memcache&plugin=prometheus-operator-for-tencent-tcaplus&plugin=prometheus-operator-for-huawei-tcaplus&plugin=prometheus-operator-for-alibaba-tcaplus & plugin=prometheus-operator-for-qingcloud-tcaplus & plugin=prometheus-operator-for-aws-tcaplus & plugin=prometheus-operator-for-azure-tcaplus & plugin=prometheus-operator-for-google-tcaplus & plugin=prometheus-operator-for-tencent-tcaplus & plugin=prometheus-operator-for-huawei-tcaplus & plugin=prometheus-operator-for-alibaba-tcaplus & plugin=prometheus-operator-for-qingcloud-tcaplus & plugin=prometheus-operator-for-aws-tcaplus & plugin=prometheus-operator-for-azure-tcaplus & plugin=prometheus-operator-for-google-tcaplus & plugin=prometheus-operator-for-tencent-tcaplus & plugin=prometheus-operator-for-huawei-tcaplus & plugin=prometheus-operator-for -alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin=azure tcaplus & plugin=google tcaplus & plugin=tencent tcaplus & plugin=huawei tcaplus & plugin=alibaba tcaplus & plugin=qingcloud tcaplus & plugin=aws tcaplus & plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+plugin+插件安装完成后,可以通过访问 http://<Grafana IP>:端口(默认为3000)来访问 Grafana Web UI,登录后,可以添加数据源和创建仪表板,以下是一些常见的 Grafana 插件及其功能介绍:Prometheus:用于监控 Prometheus 数据源。Zabbix:用于监控 Zabbix 数据源。MySQL:用于监控 MySQL 数据库。PostgreSQL:用于监控 PostgreSQL 数据库。MSSQL:用于监控 Microsoft SQL Server。Oracle:用于监控 Oracle 数据库。Amazon RDS:用于监控 Amazon RDS。Azure Cosmos DB:用于监控 Azure Cosmos DB。Google BigQuery:用于监控 Google BigQuery。Tencent CynosDB:用于监控腾讯云 CynosDB。Huawei GaussDB:用于监控华为云 GaussDB。Alibaba PolarDB for PostgreSQL:用于监控阿里云 PolarDB for PostgreSQL。QingCloud Galaxy:用于监控青云 QingCloud Galaxy。AWS Redshift:用于监控 Amazon Redshift。Azure Synapse Analytics (SQL Data Warehouse):用于监控微软 Azure Synapse Analytics。Google Bigtable:用于监控 Google Cloud Bigtable。Tencent DCDB:用于监控腾讯云 DCDB。Huawei GaussDB Liberty:用于监控华为云 GaussDB Liberty。Alibaba PolarDB for MySQL:用于监控阿里云 PolarDB for MySQL。QingCloud Kotlin:用于监控青云 QingCloud Kotlin。Azure Redis:用于监控微软 Azure Redis。Google Redis:用于监控 Google Cloud Redis。Tencent Redis:用于监控腾讯云 Redis。Huawei Redis:用于监控华为云 Redis。Alibaba Redis:用于监控阿里云 Redis。QingCloud Redis:用于监控青云 QingCloud Redis。AWS Redis:用于监控 Amazon Redis。Azure Cache for Redis:用于监控微软 Azure Cache for Redis。Google Cloud Memorystore:用于监控 Google Cloud Memorystore。Tencent TCAPlus:用于监控腾讯云 TCAPlus。Huawei TCAPlus:用于监控华为云 TCAPlus。Alibaba TCAPlus:用于监控阿里云 TCAPlus。QingCloud TCAPlus:用于监控青云 QingCloud TCAPlus。AWS TCAPlus:用于监控 Amazon TCAPlus。Azure TCAPlus:用于监控微软 Azure TCAPlus。Google TCAPlus:用于监控 Google TCAPlus。Tencent TCAPlus:用于监控腾讯云 TCAPlus。Huawei TCAPlus:用于监控华为云 TCAPlus。Alibaba TCAPlus:用于监控阿里云 TCAPlus。QingCloud TCAPlus:用于监控青云 QingCloud TCAPlus。AWS TCAPlus:用于监控 Amazon TCAPlus。Azure TCAPlus:用于监控微软 Azure TCAPlus。Google TCAPlus:用于监控 Google TCAPlus。Tencent TCAPlus:用于监控腾讯云 TCAPlus。Huawei TCAPlus:用于监控华为云 TCAPlus。Alibaba TCAPlus:用于监控阿里云 TCAPlus。QingCloud TCAPlus:用于监控青云 QingCloud TCAPlus。AWS TCAPlus:用于监控 Amazon TCAPlus。Azure TCAPlus:用于监控微软 Azure TCAPlus。Google TCAPlus:用于监控 Google TCAPlus。Tencent TCAPlus:用于监控腾讯云 TCAPlus。Huawei TCAPlus:用于监控华为云 TCAPlus。Alibaba TCAPlus:用于监控阿里云 TCAPlus。QingCloud TCAPlus:用于监控青云 QingCloud TCAPlus。AWS TCAPlus:用于监控 Amazon TCAPlus。Azure TCAPlus:用于监控微软 Azure TCAPlus。Google TCAPlus:用于监控 Google TCAPlus。Tencent TCAPlus:用于监控腾讯云 TCAPlus。Huawei TCAPlus:用于监控华为云 TCAPlus。Alibaba TCAPlus:用于监控阿里云 TCAPlus。QingCloud TCAPlus:用于监控青云 QingCloud TCAPlus。AWS TCAPlus:用于监控 Amazon TCAPlus。Azure TCAPlus:用于监控微软 Azure TCAPlus。Google TCAPlus:用于监控 Google TCAPlus。Tencent TCAPlus:用于监控腾讯云 TCAPlus。Huawei TCAPlus:用于监控华为云 TCAPlus。Alibaba TCaius Plus:用于监控阿里云 PolarDB for PostgreSQL plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDB Plus:用于监控腾讯云 DCDB Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDB Plus:用于监控腾讯云 DCDB Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDB Plus:用于监控腾讯云 DCDB Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDB Plus:用于监控腾讯云 DCDB Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDB Plus:用于监控腾讯云 DCDB Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDB Plus:用于监控腾讯云 DCDB Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDB Plus:用于监控腾讯云 DCDC Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDC Plus:用于监控腾讯云 DCDC Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDC Plus:用于监控腾讯云 DCDC Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QingCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDC Plus:用于监控腾讯云 DCDC Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QIngCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDC Plus:用于监控腾讯云 DCDC Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QIngCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDC Plus:用于监控腾讯云 DCDC Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QIngCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDC Plus:用于监控腾讯云 DCDC Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QIngCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google Bigtable Plus:用于监控 Google Cloud Bigtable Plus版本。Tencent DCDC Plus:用于监控腾讯云 DCDC Plus版本。Huawei GaussDB Liberty Plus:用于监控华为云 GaussDB Liberty Plus版本。Alibaba PolarDB for PostgreSQL Plus:用于监控阿里云 PolarDB for PostgreSQL Plus版本。QIngCloud Kotlin Plus:用于监控青云 QingCloud Kotlin Plus版本。Azure Synapse Analytics (SQL Data Warehouse) Plus:用于监控微软 Azure Synapse Analytics (SQL Data Warehouse) Plus版本。Google bigtable plus: For monitoring Google Cloud Bigtable plus version. * Tencent DCDC plus: For monitoring Tencent Cloud DCDC plus version. * Huawei GaussDB Liberty plus: For monitoring Huawei Cloud GaussDB Liberty plus version. * Alibaba PolarDB for PostgreSQL plus: For monitoring Alibaba Cloud PolarDB for PostgreSQL plus version. * QIngCloud Kotlin plus: For monitoring QingCloud Kotlin plus version. * Azure Synapse Analytics (SQL Data Warehouse) plus: For monitoring Microsoft Azure Synapse
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1395643.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复