MySQL存储图片:数据转发至MySQL存储
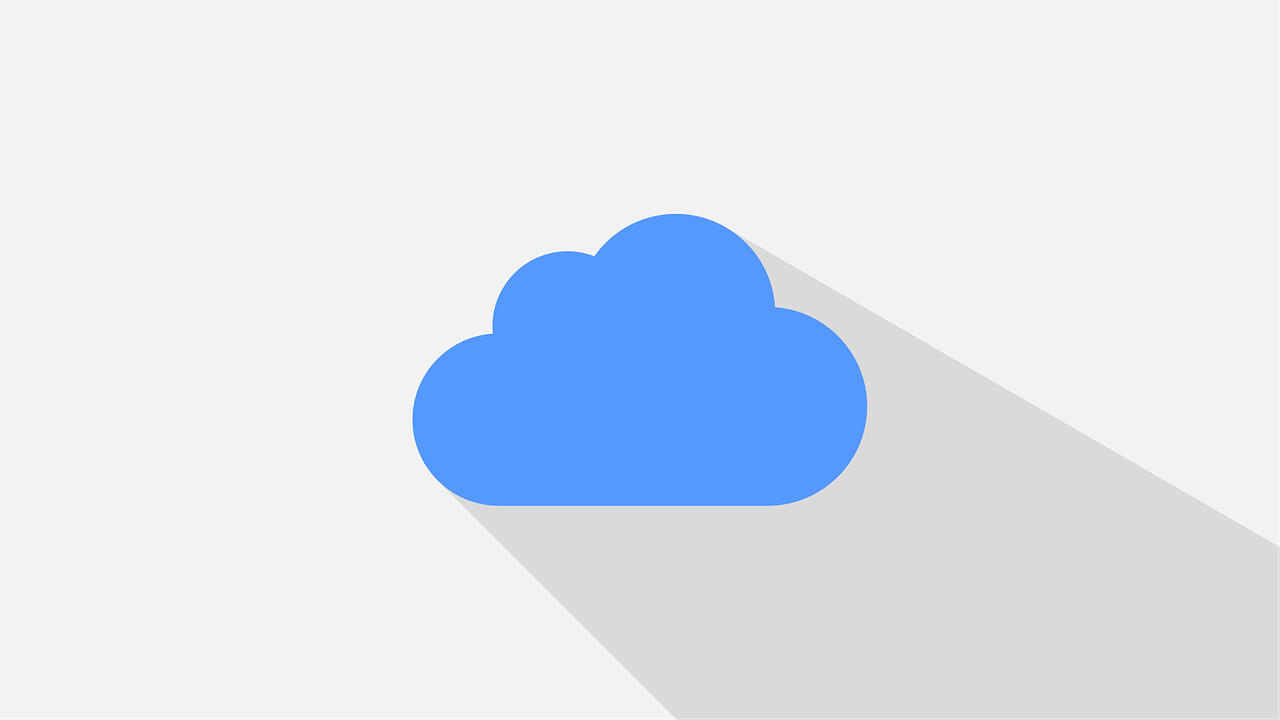
在现代应用程序开发中,数据的存储和管理是一个关键问题,特别是对于图像数据这种大型二进制文件,选择合适的存储方式至关重要,本文将详细介绍如何在MySQL数据库中存储图片,包括使用BLOB数据类型、Base64编码以及文件路径存储的方法。
一、使用BLOB数据类型
1、创建数据库表
我们需要创建一个包含BLOB字段的表来存储图片数据,以下是SQL示例:
CREATE TABLE Images ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, data LONGBLOB NOT NULL );
2、插入图片
使用Python和MySQL连接库(如mysql-connector-python),我们可以将图片数据插入到表中,以下是Python代码示例:
import mysql.connector def insert_image(file_path): try: connection = mysql.connector.connect( host='localhost', database='test_db', user='your_username', password='your_password' ) cursor = connection.cursor() with open(file_path, 'rb') as file: binary_data = file.read() query = "INSERT INTO Images (name, data) VALUES (%s, %s)" cursor.execute(query, (os.path.basename(file_path), binary_data)) connection.commit() print("Image inserted successfully") except mysql.connector.Error as error: print(f"Error: {error}") finally: if connection.is_connected(): cursor.close() connection.close() insert_image('path_to_your_image.jpg')
3、读取图片
从数据库中读取图片数据并将其保存为文件:
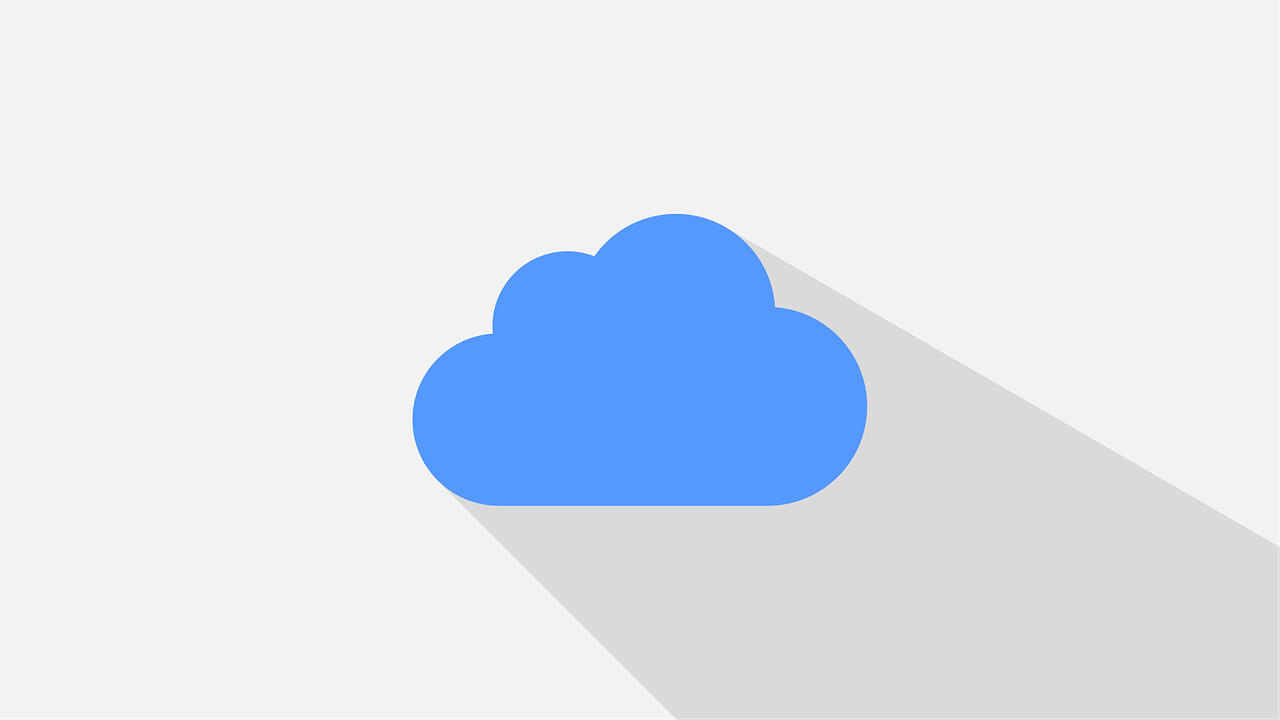
def retrieve_image(image_id, output_path): try: connection = mysql.connector.connect( host='localhost', database='test_db', user='your_username', password='your_password' ) cursor = connection.cursor() query = "SELECT data FROM Images WHERE id = %s" cursor.execute(query, (image_id,)) record = cursor.fetchone() with open(output_path, 'wb') as file: file.write(record[0]) print("Image retrieved successfully") except mysql.connector.Error as error: print(f"Error: {error}") finally: if connection.is_connected(): cursor.close() connection.close() retrieve_image(1, 'output_image.jpg')
二、通过Base64编码存储
1、创建数据库表
创建一个包含TEXT字段的表来存储Base64编码的图片数据:
CREATE TABLE Images ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, image_base64 TEXT NOT NULL );
2、插入图片
将图片文件编码为Base64并插入到数据库中:
import base64 import mysql.connector def insert_image_base64(file_path): try: connection = mysql.connector.connect( host='localhost', database='test_db', user='your_username', password='your_password' ) cursor = connection.cursor() with open(file_path, 'rb') as file: binary_data = file.read() base64_data = base64.b64encode(binary_data).decode('utf-8') query = "INSERT INTO Images (name, image_base64) VALUES (%s, %s)" cursor.execute(query, (os.path.basename(file_path), base64_data)) connection.commit() print("Image inserted successfully") except mysql.connector.Error as error: print(f"Error: {error}") finally: if connection.is_connected(): cursor.close() connection.close() insert_image_base64('path_to_your_image.jpg')
3、读取图片
从数据库中读取Base64编码的数据并解码为图片文件:
def retrieve_image_base64(image_id, output_path): try: connection = mysql.connector.connect( host='localhost', database='test_db', user='your_username', password='your_password' ) cursor = connection.cursor() query = "SELECT image_base64 FROM Images WHERE id = %s" cursor.execute(query, (image_id,)) record = cursor.fetchone() base64_data = record[0] binary_data = base64.b64decode(base64_data) with open(output_path, 'wb') as file: file.write(binary_data) print("Image retrieved successfully") except mysql.connector.Error as error: print(f"Error: {error}") finally: if connection.is_connected(): cursor.close() connection.close() retrieve_image_base64(1, 'output_image.jpg')
三、使用文件路径存储
1、创建数据库表
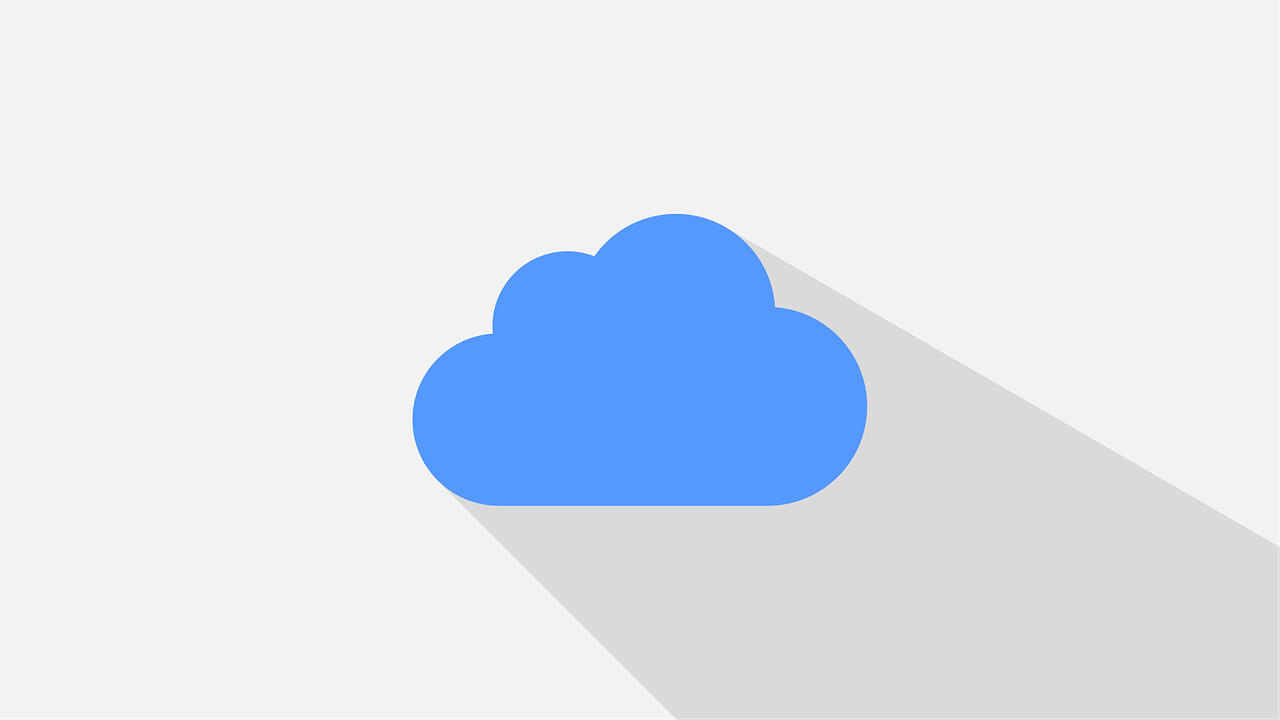
创建一个包含VARCHAR字段的表来存储图片的文件路径:
CREATE TABLE Images ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, path VARCHAR(255) NOT NULL );
2、插入图片路径
将图片文件复制到指定目录并将路径插入到数据库中:
import os import shutil import mysql.connector def insert_image_path(file_path, storage_directory): destination_path = os.path.join(storage_directory, os.path.basename(file_path)) shutil.copy(file_path, destination_path) try: connection = mysql.connector.connect( host='localhost', database='test_db', user='your_username', password='your_password' ) cursor = connection.cursor() query = "INSERT INTO Images (name, path) VALUES (%s, %s)" cursor.execute(query, (os.path.basename(file_path), destination_path)) connection.commit() print("Image path saved successfully") except mysql.connector.Error as error: print(f"Error: {error}") finally: if connection.is_connected(): cursor.close() connection.close() insert_image_path('path_to_your_image.jpg', '/path/to/storage')
3、读取图片路径
从数据库中读取图片路径并显示图片:
from PIL import Image import io import mysql.connector def display_image(image_id): try: connection = mysql.connector.connect( host='localhost', database='test_db', user='your_username', password='your_password' ) cursor = connection.cursor() query = "SELECT path FROM Images WHERE id = %s" cursor.execute(query, (image_id,)) record = cursor.fetchone() image_path = record[0] img = Image.open(image_path) img.show() except mysql.connector.Error as error: print(f"Error: {error}") finally: if connection.is_connected(): cursor.close() connection.close() display_image(1)
四、优缺点分析
方法 | 优点 | 缺点 |
BLOB | 数据完整性高 易于备份和恢复 安全性好 | 性能问题 数据库体积膨胀 |
Base64 | 兼容性好 易于调试 | 数据膨胀 性能问题 |
文件路径 | 简化数据库管理 减少数据库大小 | 需要额外的文件管理系统 依赖文件系统的稳定性 |
五、常见问题解答(FAQs)
1、Q: 为什么选择BLOB存储而不是文件路径?
A: BLOB存储将所有数据集中在一个地方,便于备份和恢复,且安全性更高,但要注意性能问题,特别是在处理大文件或大量文件时,文件路径存储则依赖于文件系统,可能会受到文件系统的限制和影响。
2、Q: Base64编码存储的优点是什么?
A: Base64编码的主要优点是兼容性好,文本数据在各种系统和传输协议中更具兼容性,且便于查看和调试,Base64编码会使数据体积增大约33%,这可能会影响性能。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1395069.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复