在Vue项目中,通过CDN引入字体是一种快速且高效的方法,CDN(内容分发网络)能够显著加快网页加载速度,因为它可以从离用户最近的服务器上提供资源,以下将详细介绍如何在Vue项目中使用CDN引入字体。
一、选择并获取CDN链接
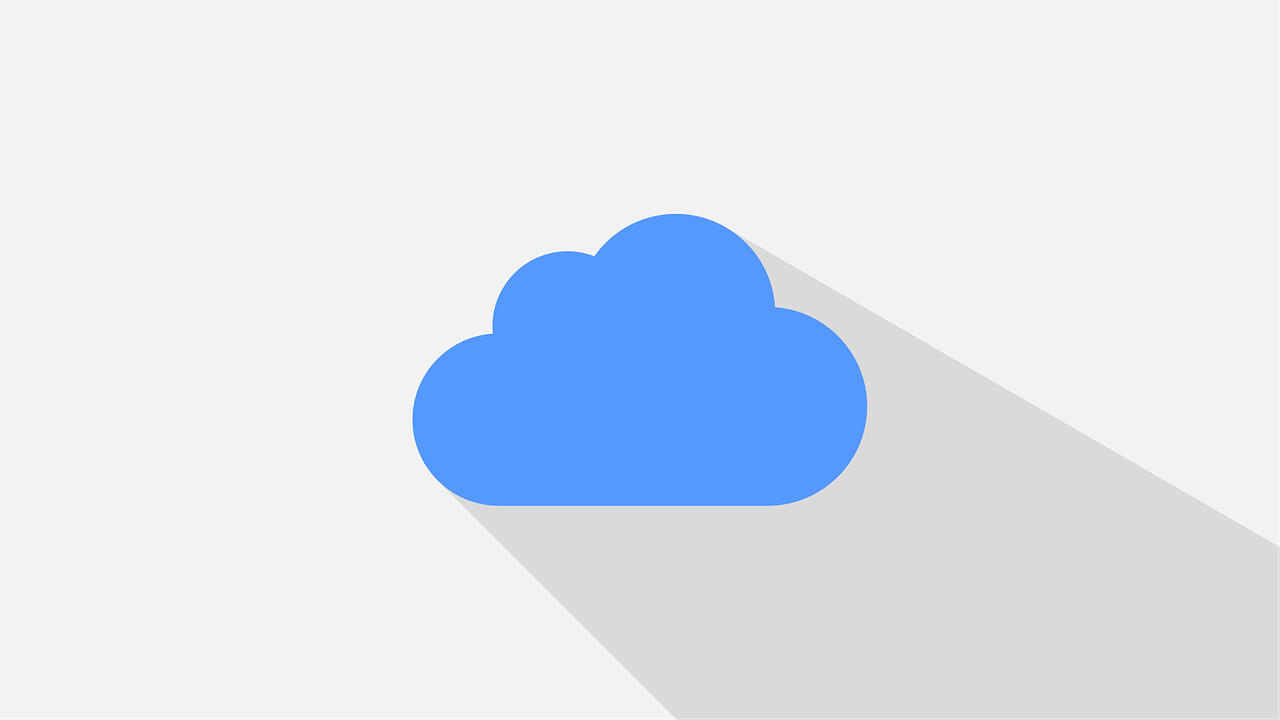
需要选择所需的字体并获取其CDN链接,常见的字体提供商有Google Fonts和Font Awesome等,以下是具体步骤:
1、访问Google Fonts网站:打开[Google Fonts](https://fonts.google.com/),选择所需字体,点击“Select this style”按钮,然后复制生成的CDN链接,对于Roboto字体,链接如下:
<link href="https://fonts.googleapis.com/css2?family=Roboto:wght@400;700&display=swap" rel="stylesheet">
2、访问Font Awesome网站:打开[Font Awesome](https://fontawesome.com/),选择所需图标和字体,注册并获取CDN链接,对于Font Awesome 5.15.3,链接如下:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css">
二、在Vue项目中引入CDN链接
获取CDN链接后,将其添加到Vue项目的public/index.html
文件中,以下是具体步骤:
1、<head>
2、添加CDN链接:将复制的CDN链接粘贴到<head>
标签中。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Vue App</title> <!-Google Fonts CDN --> <link href="https://fonts.googleapis.com/css2?family=Roboto:wght@400;700&display=swap" rel="stylesheet"> <!-Font Awesome CDN --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"> </head> <body> <div id="app"></div> </body> </html>
三、在CSS中使用字体
引入CDN链接后,可以在CSS中使用这些字体,以下是一些示例代码:
/* 使用Google Fonts中的Roboto字体 */ body { font-family: 'Roboto', sans-serif; } /* 使用Font Awesome图标 */ .fa-star { color: #fff; font-size: 2.5rem; } .fa-heart { color: #fff; font-size: 2rem; } .fa-user { color: #fff; font-size: 1.5rem; } .fa-film { color: #fff; font-size: 1.25rem; } .fa-sun { color: #fff; font-size: 1rem; } .fa-moon { color: #fff; font-size: 1rem; } .fa-cloud { color: #fff; font-size: 1rem; } .fa-envelope { color: #fff; font-size: 1rem; } .fa-pencil { color: #fff; font-size: 1rem; } .fa-link { color: #fff; font-size: 1rem; } .fa-camera { color: #fff; font-size: 1rem; } .fa-video { color: #fff; font-size: 1rem; } .fa-chart { color: #fff; font-size: 1rem; } .fa-tree { color: #fff; font-size: 1rem; } .fa-download-alt { color: #fff; font-size: 1rem; text-decoration: none; } .fa-chart-add { color: #fff; font-size: 1rem; text-decoration: none; }
四、在组件中使用字体图标
在Vue组件的模板中,可以直接使用Font Awesome图标,以下是一些示例代码:
<template> <div class="icon"> <i class="fas fa-home"></i> Home </div> </template> <style scoped> .icon { font-family: 'Font Awesome 5 Free'; } </style>
五、相关问答FAQs
Q1:如何在Vue项目中引入本地字体?
A1:要在Vue项目中引入本地字体,可以按照以下步骤进行操作:
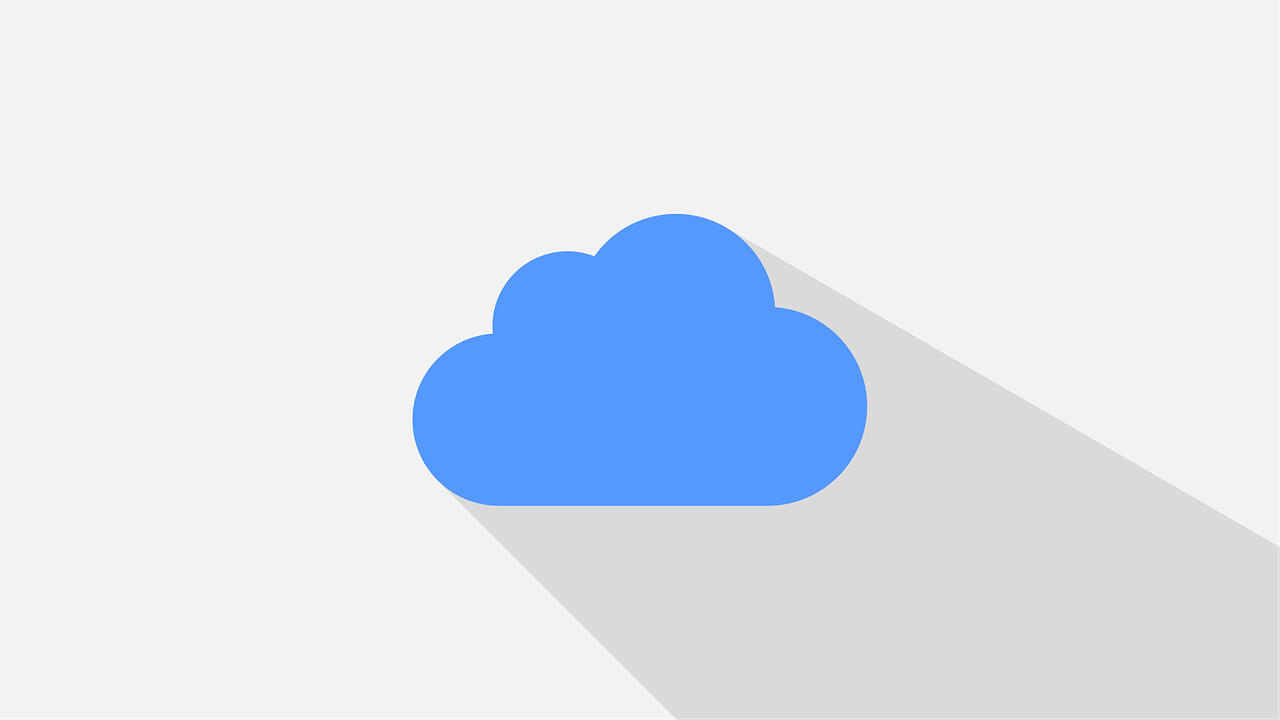
1、下载字体文件:从字体提供商网站下载所需字体文件,例如.ttf
或.woff
格式的文件。
2、放置字体文件:将下载的字体文件放在Vue项目的src/assets/fonts
目录下。
3、创建CSS文件:在项目中创建一个新的CSS文件,例如src/assets/styles/fonts.css
,并在其中定义字体的@font-face
规则:
@font-face { font-family: 'MyCustomFont'; src: url('@/assets/fonts/MyCustomFont.woff2') format('woff2'), url('@/assets/fonts/MyCustomFont.woff') format('woff'); font-weight: normal; font-style: normal; }
4、在Vue项目中引入CSS文件:在项目的入口文件(如main.js
)中引入该CSS文件:
import './assets/styles/fonts.css';
5、使用字体:在Vue组件的<style>
标签或全局CSS文件中使用新定义的字体:
body { font-family: 'MyCustomFont', sans-serif; }
6、在组件中使用字体:在需要使用字体的组件中,通过样式绑定或者直接在样式中引用字体:
<template> <div> <h1 :style="{ fontFamily: 'MyCustomFont' }">这是自定义字体</h1> <p class="my-custom-font">这是使用自定义字体的段落</p> </div> </template> <style> .my-custom-font { font-family: 'MyCustomFont', sans-serif; } </style>
Q2:如何在Vue项目中引入第三方在线字体?
A2:如果要在Vue项目中引入第三方在线字体,可以按照以下步骤进行操作:
1、访问第三方字体库网站:例如Google Fonts或其他字体提供商网站,选择所需字体。
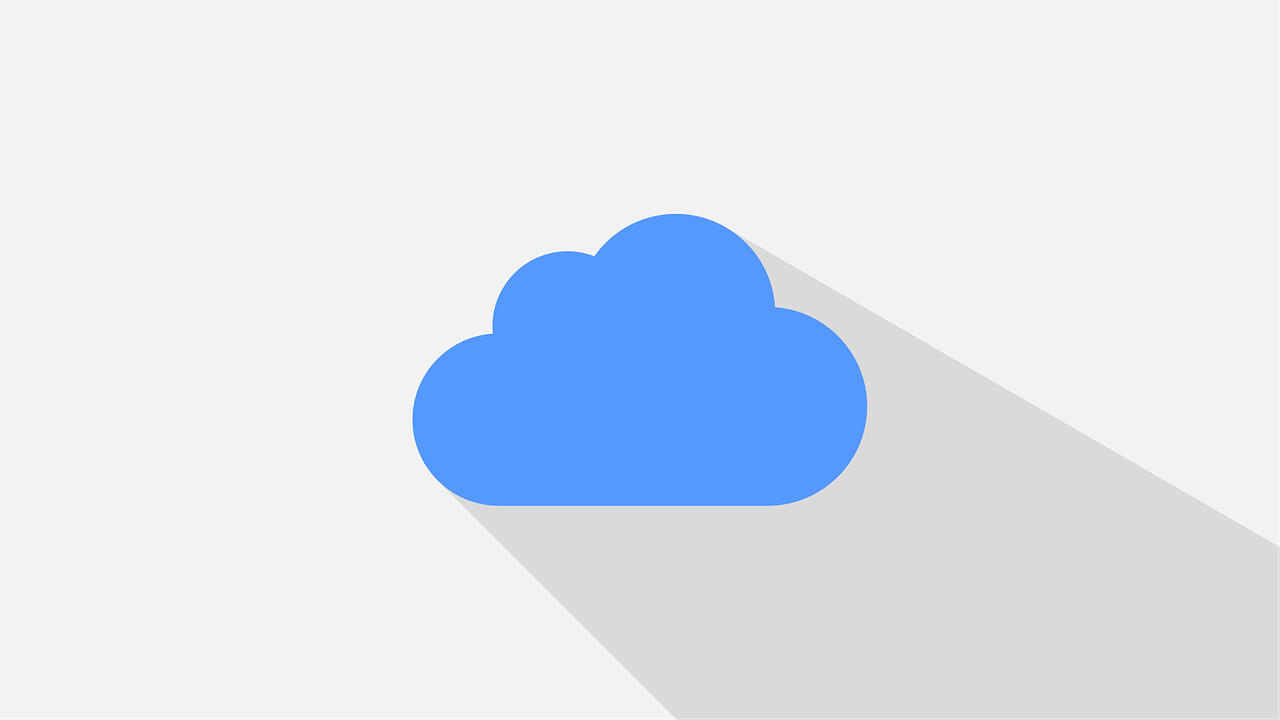
2、获取CDN链接:在字体提供商网站上生成并复制CDN链接,对于Google Fonts上的Roboto字体,链接如下:
<link href="https://fonts.googleapis.com/css2?family=Roboto:wght@400;700&display=swap" rel="stylesheet">
3、在Vue项目中引入CDN链接:将复制的CDN链接添加到Vue项目的public/index.html
文件的<head>
标签中:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Vue App</title> <!-引入第三方在线字体 --> <link href="https://fonts.googleapis.com/css2?family=Roboto:wght@400;700&display=swap" rel="stylesheet"> </head> <body> <div id="app"></div> </body> </html>
4、在CSS中使用字体:在Vue组件的<style>
标签或全局CSS文件中定义字体:
body { font-family: 'Roboto', sans-serif; }
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1383550.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复