java.net.URL
类和java.io
包中的类来读取远程文件并写入本地文件系统。在Java中下载文件是一项常见的任务,无论是从网络上下载资源还是从本地系统中读取数据,为了实现这一功能,Java提供了多种方式和工具,如使用java.net
包中的类、NIO(New Input/Output)库以及第三方库等,本文将介绍几种常用的方法来实现文件下载,并提供相关的代码示例。
方法一:使用java.net.HttpURLConnection
这是最常用的方法之一,适用于从网络下载文件,以下是一个示例代码:
import java.io.*; import java.net.HttpURLConnection; import java.net.URL; public class FileDownloader { public static void downloadFile(String fileURL, String saveDir) throws IOException { URL url = new URL(fileURL); HttpURLConnection httpConn = (HttpURLConnection) url.openConnection(); int responseCode = httpConn.getResponseCode(); if (responseCode == HttpURLConnection.HTTP_OK) { InputStream inputStream = httpConn.getInputStream(); String fileName = ""; String disposition = httpConn.getHeaderField("Content-Disposition"); if (disposition != null) { int index = disposition.indexOf("filename="); if (index > 0) { fileName = disposition.substring(index + 10, disposition.length() 1); } } else { fileName = fileURL.substring(fileURL.lastIndexOf("/") + 1); } FileOutputStream outputStream = new FileOutputStream(saveDir + File.separator + fileName); int bytesRead; byte[] buffer = new byte[4096]; while ((bytesRead = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, bytesRead); } outputStream.close(); inputStream.close(); System.out.println("File downloaded: " + saveDir + File.separator + fileName); } else { System.out.println("No file to download. Server replied HTTP code: " + responseCode); } httpConn.disconnect(); } public static void main(String[] args) { try { downloadFile("https://example.com/file.txt", "/path/to/download"); } catch (IOException ex) { ex.printStackTrace(); } } }
方法二:使用java.nio.file.Files
和java.nio.channels.Channels
这种方法使用了Java NIO库,可以更高效地处理大文件,以下是一个示例代码:
import java.io.*; import java.net.URL; import java.nio.channels.Channels; import java.nio.channels.ReadableByteChannel; import java.nio.file.Paths; import java.nio.file.StandardOpenOption; public class FileDownloaderNIO { public static void downloadFile(String fileURL, String saveDir) throws IOException { URL url = new URL(fileURL); try (ReadableByteChannel rbc = Channels.newChannel(url.openStream()); FileOutputStream fos = new FileOutputStream(saveDir).getChannel()) { fos.transferFrom(rbc, 0, Long.MAX_VALUE); System.out.println("File downloaded using NIO: " + saveDir); } } public static void main(String[] args) { try { downloadFile("https://example.com/file.txt", "/path/to/download"); } catch (IOException ex) { ex.printStackTrace(); } } }
方法三:使用Apache Commons IO库
Apache Commons IO库提供了一些简化的文件操作方法,包括下载文件,首先需要添加依赖:
<dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.8.0</version> </dependency>
然后可以使用以下代码下载文件:
import org.apache.commons.io.FileUtils; import java.io.File; import java.io.IOException; import java.net.URL; public class FileDownloaderCommonsIO { public static void downloadFile(String fileURL, String saveDir) throws IOException { File output = new File(saveDir); FileUtils.copyURLToFile(new URL(fileURL), output); System.out.println("File downloaded using Apache Commons IO: " + output.getAbsolutePath()); } public static void main(String[] args) { try { downloadFile("https://example.com/file.txt", "/path/to/download"); } catch (IOException ex) { ex.printStackTrace(); } } }
如果需要下载网页内容并解析HTML,可以使用Jsoup
库,以下是一个示例代码:
import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import java.io.FileWriter; import java.io.IOException; public class WebPageDownloader { public static void downloadWebPage(String url, String saveDir) throws IOException { Document doc = Jsoup.connect(url).get(); try (FileWriter writer = new FileWriter(saveDir)) { writer.write(doc.html()); System.out.println("Web page downloaded: " + saveDir); } } public static void main(String[] args) { try { downloadWebPage("https://example.com", "/path/to/download/webpage.html"); } catch (IOException ex) { ex.printStackTrace(); } } }
相关问答FAQs
Q1: 如何指定下载文件的保存路径?
A1: 在上述所有示例代码中,saveDir
参数即为下载文件的保存路径,你可以根据需要修改该参数的值来指定具体的保存位置。
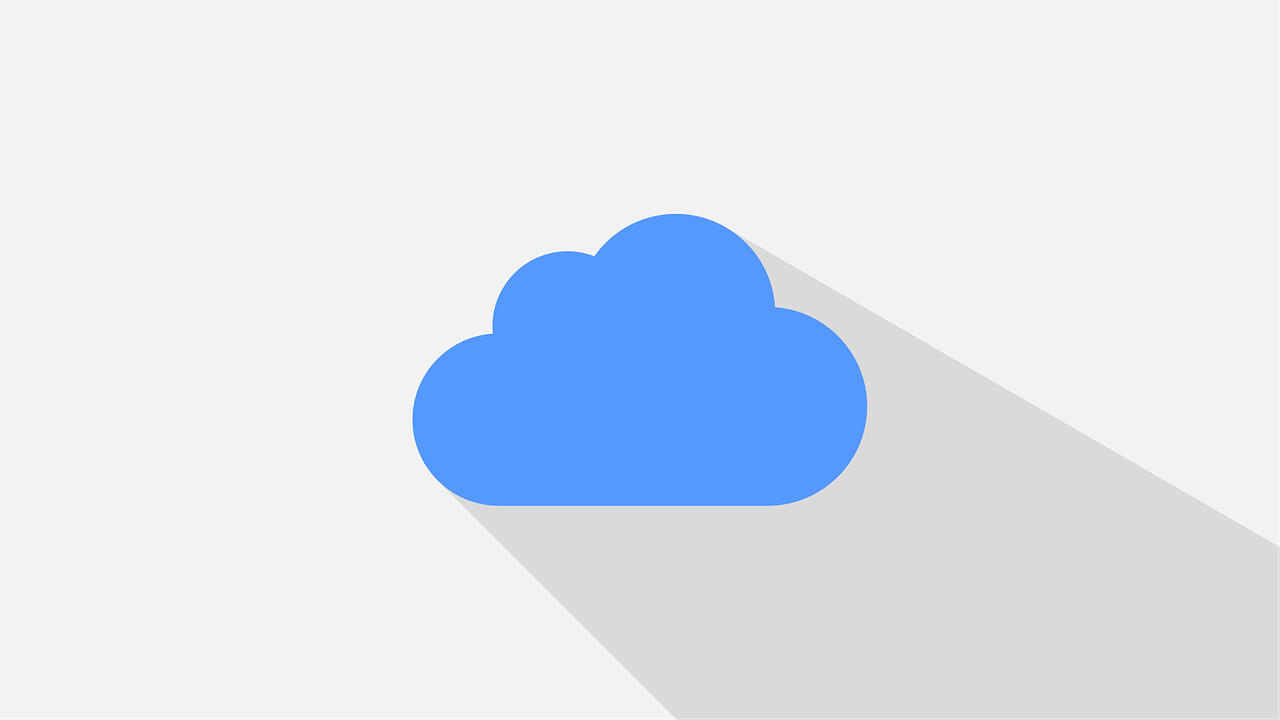
downloadFile("https://example.com/file.txt", "/path/to/download");
这将把文件下载到/path/to/download
目录下。
Q2: 如果下载的文件已经存在,如何处理?
A2: 你可以在下载文件之前检查目标文件是否存在,并根据需要选择覆盖或跳过下载,以下是一个示例代码:
import java.io.File; import java.io.IOException; import java.net.HttpURLConnection; import java.net.URL; public class FileDownloaderWithCheck { public static void downloadFile(String fileURL, String saveDir) throws IOException { File file = new File(saveDir); if (file.exists()) { System.out.println("File already exists: " + saveDir); return; // Skip downloading if file already exists } URL url = new URL(fileURL); HttpURLConnection httpConn = (HttpURLConnection) url.openConnection(); int responseCode = httpConn.getResponseCode(); if (responseCode == HttpURLConnection.HTTP_OK) { // Download logic here... } else { System.out.println("No file to download. Server replied HTTP code: " + responseCode); } httpConn.disconnect(); } }
在这个示例中,如果目标文件已存在,程序将输出提示信息并跳过下载过程,否则,它将继续执行下载逻辑。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1382587.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复