ExecuteScript
方法来执行JavaScript代码,并通过回调函数获取结果。,,以下是一个简单的示例:,,“cpp,#include "include/cef_app.h",#include "include/cef_client.h",#include "include/wrapper/cef_helpers.h",,class MyClient : public CefClient {,public:, MyClient() {},, virtual CefRefPtr GetLifeSpanHandler() OVERRIDE {, return this;, },, void OnAfterCreated(CefRefPtr browser) OVERRIDE {, // Execute JavaScript and get the result, CefRefPtr frame = browser->GetMainFrame();, frame->ExecuteJavaScript("JSON.stringify({key: 'value'})", "", 0);, },, IMPLEMENT_REFCOUNTING(MyClient);,};,,class MyApp : public CefApp, public CefJsDialogHandler {,public:, MyApp() {},, virtual CefRefPtr GetDefaultClient() OVERRIDE {, return new MyClient();, },, virtual bool OnJSDialog(CefRefPtr browser,, const CefString& origin_url,, const CefString& accept_lang,, CefJSDialogType dialog_type,, const CefString& message_text,, const CefString& default_prompt_text,, CefRefPtr callback,, bool& suppress_message) OVERRIDE {, // Handle JavaScript dialog here if needed, return false;, },, IMPLEMENT_REFCOUNTING(MyApp);,};,,int main(int argc, char* argv[]) {, CefMainArgs main_args(argc, argv);, CefRefPtr app(new MyApp());, int exit_code = CefExecuteProcess(main_args, app, nullptr);, if (exit_code >= 0) {, return exit_code;, },, CefSettings settings;, CefInitialize(main_args, settings, app, nullptr);, CefRunMessageLoop();, CefShutdown();, return 0;,},
“,,在这个示例中,当浏览器创建后,会执行一段JavaScript代码,并将结果通过回调函数返回。你可以根据需要修改JavaScript代码和处理逻辑。在现代Web开发中,跨语言的交互变得愈加重要,CEF3(Chromium Embedded Framework)作为一种嵌入式浏览器框架,允许开发者在他们的桌面应用程序中嵌入网页视图,有时需要从JavaScript获取数据并传递到C++代码中,本文将详细介绍如何在CEF3中获取JS返回值的方法和步骤。
准备工作
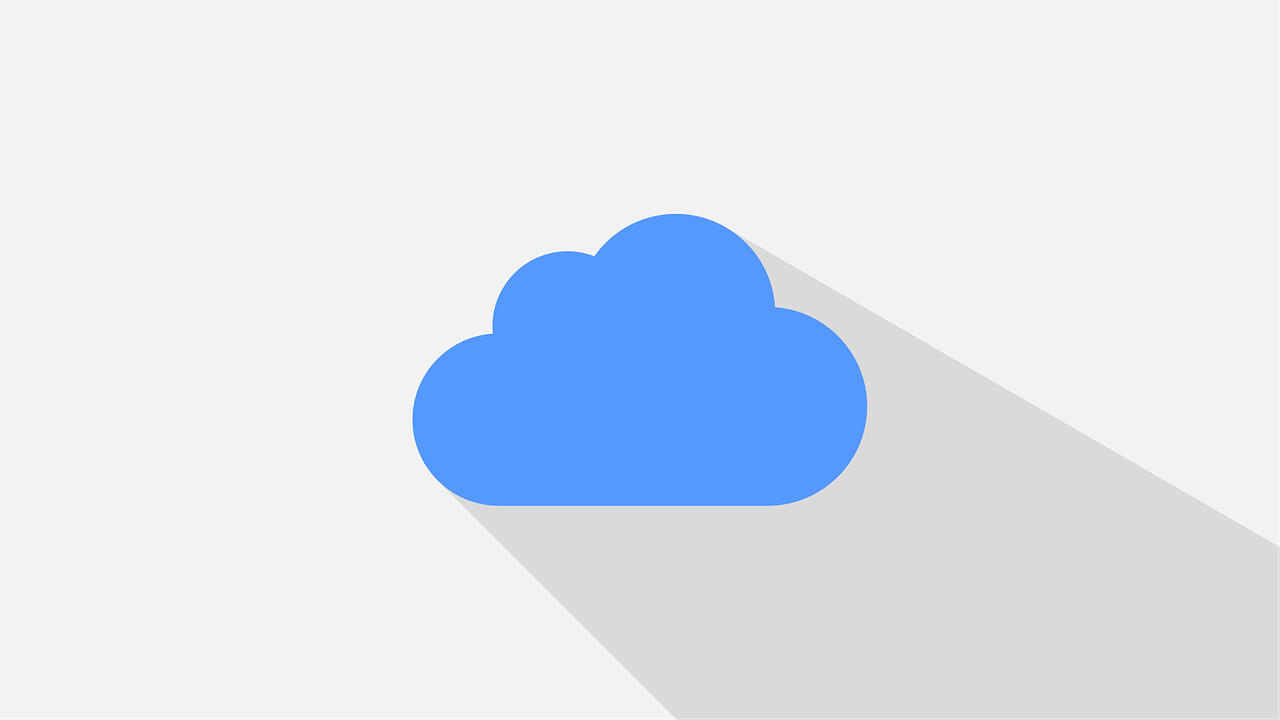
确保你已经安装并配置好CEF3开发环境,如果尚未完成这一步,可以访问[CEF3官方文档](https://bitbucket.org/chromiumembedded/cef/wiki/GeneralUsage)获取详细指南。
2. CEF3与JavaScript的交互基础
CEF3提供了一些接口,使得C++代码能够调用JavaScript函数,并处理JavaScript返回的结果,这主要通过CefV8context
类来实现。
2.1 创建CefV8上下文
在使用CEF3时,首先需要创建一个CefV8context
对象,这是所有JavaScript执行的基础。
#include "include/cef_v8.h" // 假设你已经有一个有效的browser实例 CefRefPtr<CefBrowser> browser; // 获取主帧的V8上下文 CefRefPtr<CefV8context> v8Context = browser->GetMainFrame()->GetV8Context();
2.2 定义JavaScript函数
在HTML页面中定义一个JavaScript函数,该函数将返回你需要的数据。
<!DOCTYPE html> <html> <head> <title>Sample Page</title> <script type="text/javascript"> function getData() { return "Hello from JavaScript!"; } </script> </head> <body> <h1>Check the console for output</h1> </body> </html>
3. 从C++调用JavaScript并获取返回值
使用C++代码调用这个JavaScript函数,并获取其返回值,以下是详细的步骤:
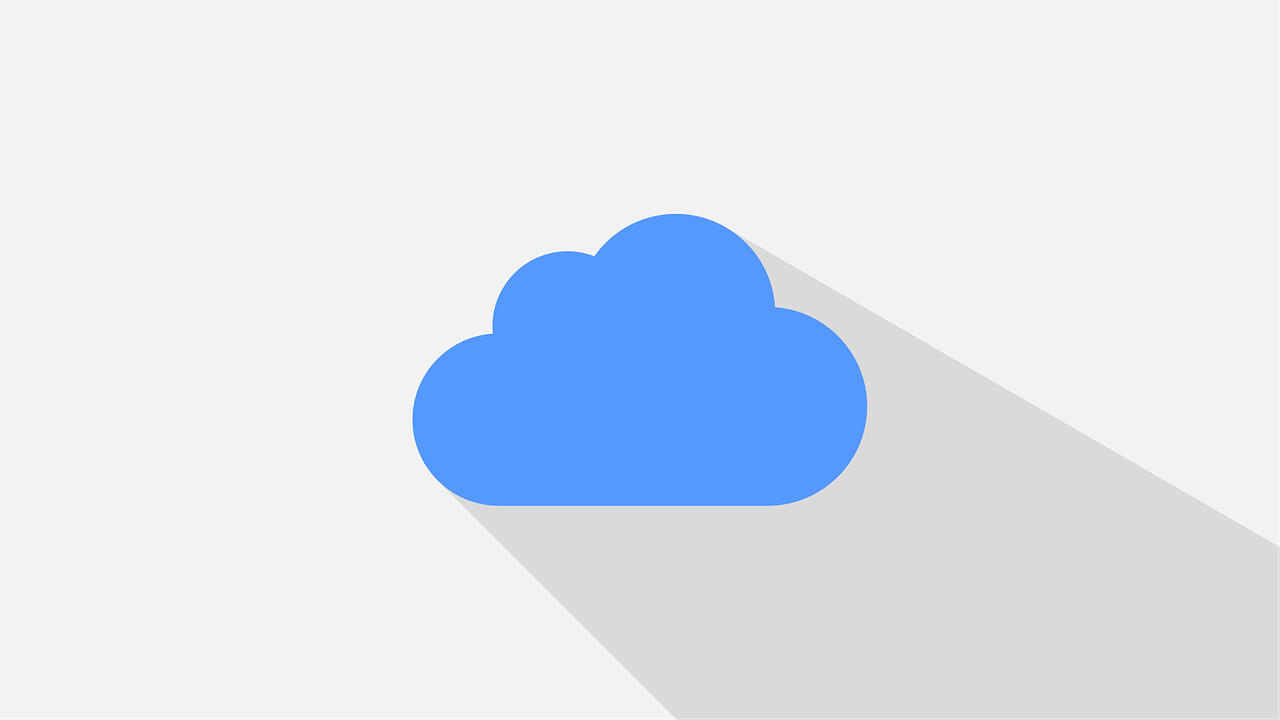
3.1 创建访问器对象
为了访问JavaScript中的函数,我们需要创建一个访问器对象:
#include "include/cef_v8value.h" // 定义一个回调类来接收JavaScript函数的返回值 class JSAccessor : public CefV8Accessor { public: explicit JSAccessor(CefRefPtr<CefV8context> context) : context_(context) {} CefRefPtr<CefV8Value> GetReturnValue() { return return_value_; } private: CefRefPtr<CefV8context> context_; CefRefPtr<CefV8Value> return_value_; };
3.2 调用JavaScript函数
使用访问器对象调用JavaScript函数,并获取返回值:
bool result = false; CefRefPtr<CefV8Value> arg(context->GetException()); CefRefPtr<CefV8Value> func = context->GetFunction("getData"); if (func.get()) { CefRefPtr<JSAccessor> accessor(new JSAccessor(context)); result = accessor->ExecuteFunctionWithContext(NULL, func, arg, 1); }
3.3 处理返回值
处理从JavaScript返回的值:
if (result && accessor && accessor->GetReturnValue().get()) { CefString returned_value; accessor->GetReturnValue()->GetString().ToWString(&returned_value); std::wcout << L"JavaScript returned: " << returned_value.c_str() << std::endl; } else { std::wcout << L"Failed to call JavaScript function or no return value." << std::endl; }
完整示例代码
以下是一个完整的示例代码,展示了如何从C++代码中获取JavaScript返回的值:
#include "include/cef_app.h" #include "include/cef_client.h" #include "include/cef_browser.h" #include "include/cef_frame.h" #include "include/cef_v8.h" #include "include/cef_v8value.h" #include <iostream> // 简单的客户端实现 class SimpleClient : public CefClient, public CefLifeSpanHandler { public: SimpleClient() {} virtual ~SimpleClient() {} // 实现必要的方法... }; int main(int argc, char* argv[]) { CefMainArgs main_args(argc, argv); int exit_code = CefExecuteProcess(main_args, nullptr, nullptr); if (exit_code >= 0) { return exit_code; } // 初始化CEF CefSettings settings; CefInitialize(main_args, settings, app.get(), nullptr); CefWindowInfo window_info; CefBrowserSettings browser_settings; SimpleClient client; CefBrowserHost::CreateBrowser(window_info, client, "http://localhost/test.html", browser_settings, nullptr, nullptr); // 获取主帧的V8上下文 CefRefPtr<CefBrowser> browser = client.GetBrowser(); CefRefPtr<CefFrame> frame = browser->GetMainFrame(); CefRefPtr<CefV8context> v8Context = frame->GetV8Context(); // 创建访问器对象 CefRefPtr<JSAccessor> accessor(new JSAccessor(v8Context)); // 调用JavaScript函数并获取返回值 CefRefPtr<CefV8Value> func = v8Context->GetFunction("getData"); if (func.get()) { bool result = accessor->ExecuteFunctionWithContext(nullptr, func, v8Context->GetException(), 1); if (result && accessor->GetReturnValue().get()) { CefString returned_value; accessor->GetReturnValue()->GetString().ToWString(&returned_value); std::wcout << L"JavaScript returned: " << returned_value.c_str() << std::endl; } else { std::wcout << L"Failed to call JavaScript function or no return value." << std::endl; } } else { std::wcout << L"JavaScript function 'getData' not found." << std::endl; } // 清理并退出CEF CefShutdown(); return 0; }
相关FAQs
Q1: 如果JavaScript函数没有返回值怎么办?
A1: 如果JavaScript函数没有显式返回值,它将默认返回undefined
,在C++代码中,你可以通过检查accessor->GetReturnValue()
是否为空来判断是否有返回值,如果没有返回值,你可以采取适当的措施,比如记录日志或显示错误信息。
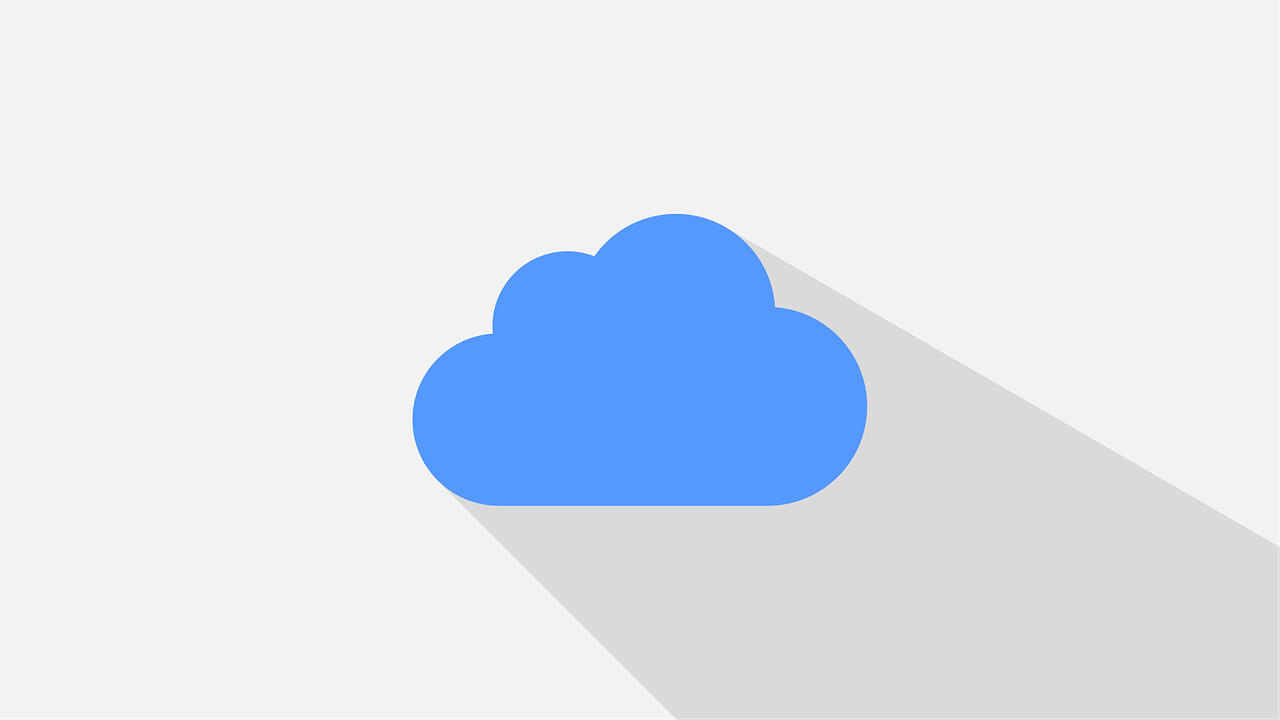
Q2: 如何处理JavaScript函数抛出的异常?
A2: 当JavaScript函数抛出异常时,你可以通过context->GetException()
获取异常对象,并进行相应的处理,可以记录异常信息或者显示错误对话框,确保在捕获异常后进行适当的清理操作,以防止内存泄漏或其他资源问题。
小编有话说
通过上述步骤,我们详细介绍了如何在CEF3中从C++代码获取JavaScript返回值的方法,这个过程涉及多个步骤,包括创建V8上下文、定义JavaScript函数、调用JavaScript函数以及处理返回值,希望这篇文章能帮助你在项目中实现跨语言的交互,提升开发效率和应用的功能丰富度,如果你有任何疑问或需要进一步的帮助,欢迎随时提问!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1381946.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复