在处理PHP字符串时,从其中提取特定数据是一个常见需求,无论是解析用户输入、处理日志文件,还是操作API响应,掌握有效的字符串处理技术都是非常重要的,本文将详细介绍如何在PHP中从字符串中提取特定数据的方法,包括正则表达式、字符串函数以及一些实用的技巧和示例。
使用正则表达式提取数据
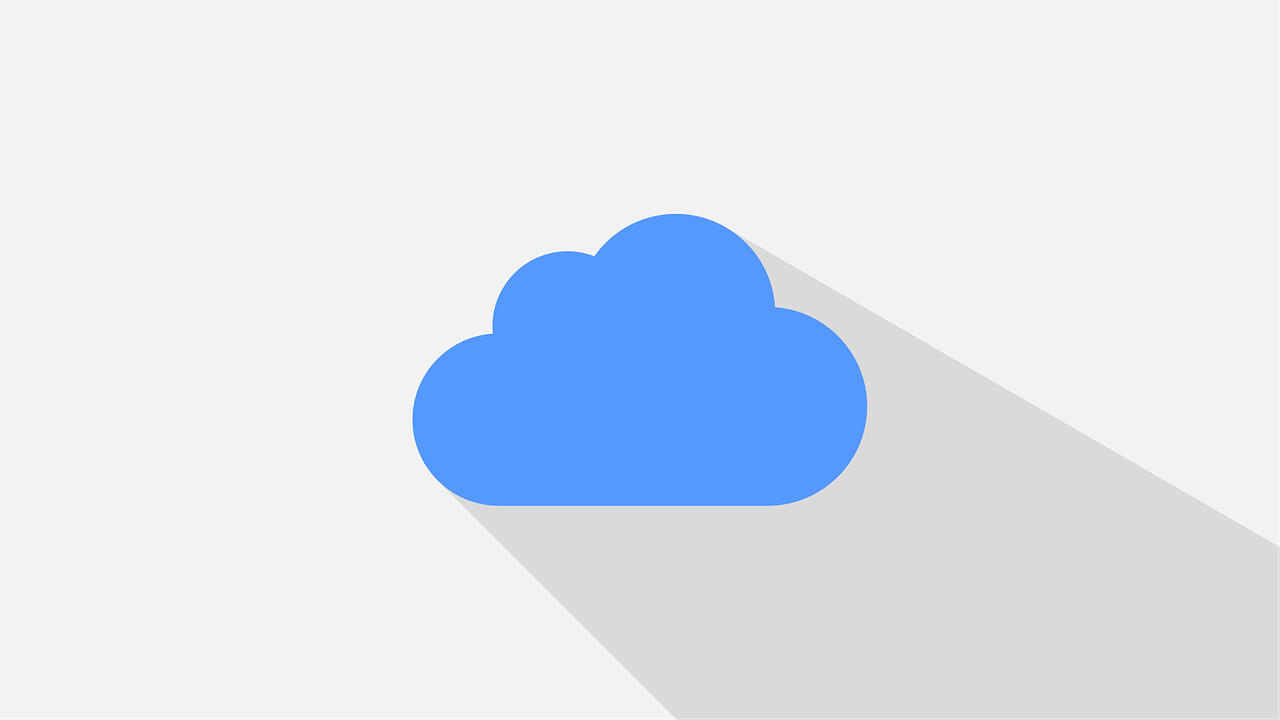
正则表达式是一种强大的文本处理工具,可以用于匹配和提取复杂的字符串模式,PHP提供了preg_match()
和preg_match_all()
函数来执行正则表达式匹配。
示例:提取电子邮件地址
$text = "请联系 support@example.com 或 sales@example.com"; $pattern = '/[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,6}/'; preg_match_all($pattern, $text, $matches); print_r($matches[0]); // 输出: Array ( [0] => support@example.com [1] => sales@example.com )
示例:提取URL
$text = "访问我们的网站 http://www.example.com 或 https://secure.example.com"; $pattern = '/https?://[a-zA-Z0-9.-]+.[a-zA-Z]{2,6}/i'; preg_match_all($pattern, $text, $matches); print_r($matches[0]); // 输出: Array ( [0] => http://www.example.com [1] => https://secure.example.com )
使用字符串函数提取数据
PHP还提供了许多内置的字符串函数,可以用来处理和提取字符串中的特定数据。strpos()
、substr()
、str_replace()
等都是常用的函数。
示例:从固定格式的字符串中提取数据
假设有一个固定格式的字符串"Name: John Doe, Age: 30"
,我们需要提取名字和年龄。
$string = "Name: John Doe, Age: 30"; $name = substr($string, strpos($string, ":") + 2, strpos($string, ",") strpos($string, ":") 2); $age = substr($string, strpos($string, ":") + 4); echo "Name: $name, Age: $age"; // 输出: Name: John Doe, Age: 30
结合使用正则表达式和字符串函数
有时,单一的处理方法可能无法满足需求,这时可以结合使用正则表达式和字符串函数来达到目的。
示例:从复杂文本中提取多个字段
$text = "订单号: 123456, 商品: 书籍, 数量: 2, 总价: 100元"; $orderPattern = '/订单号:s*(d+)/'; $productPattern = '/商品:s*([^,]+)/'; $quantityPattern = '/数量:s*(d+)/'; $totalPricePattern = '/总价:s*(d+.?d*)元/'; preg_match($orderPattern, $text, $orderMatches); preg_match($productPattern, $text, $productMatches); preg_match($quantityPattern, $text, $quantityMatches); preg_match($totalPricePattern, $text, $totalPriceMatches); $data = [ 'order_id' => $orderMatches[1], 'product' => $productMatches[1], 'quantity' => $quantityMatches[1], 'total_price' => $totalPriceMatches[1] ]; print_r($data); // 输出: Array ( [order_id] => 123456 [product] => 书籍 [quantity] => 2 [total_price] => 100 )
方法 | 描述 | 示例代码 |
preg_match() | 进行正则表达式匹配 | preg_match('/pattern/', $string, $matches) |
preg_match_all() | 进行全局正则表达式匹配 | preg_match_all('/pattern/', $string, $matches) |
strpos() | 查找子字符串的位置 | $pos = strpos($string, 'search') |
substr() | 获取子字符串 | $substring = substr($string, $start, $length) |
str_replace() | 替换字符串 | $newString = str_replace('old', 'new', $string) |
explode() | 分割字符串 | $array = explode(',', $string) |
implode() | 连接数组为字符串 | $string = implode(',', $array) |
trim() | 去除字符串两端空白 | $cleanString = trim($string) |
FAQs
Q1: 如何从包含HTML标签的字符串中提取纯文本?
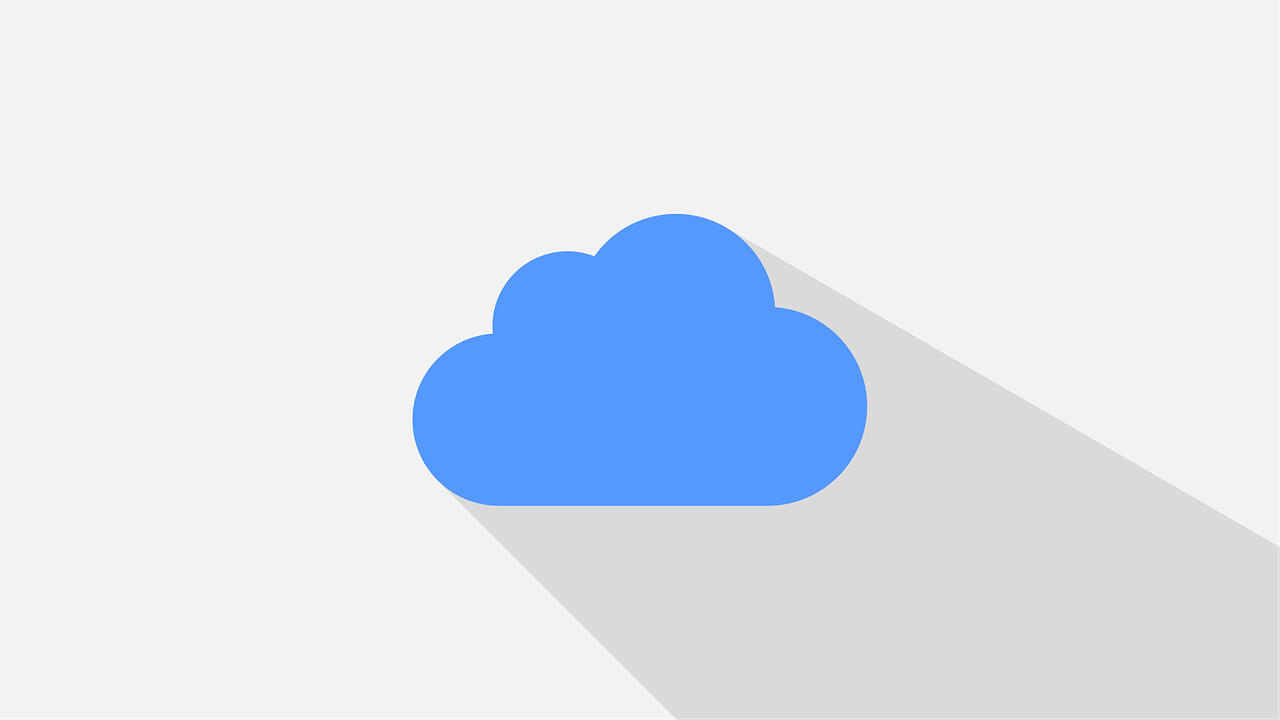
A1: 可以使用PHP的DOMDocument类来解析HTML并提取其中的文本内容。
$html = '<div>Hello <b>World</b></div>'; $dom = new DOMDocument(); @$dom->loadHTML($html, LIBXML_NOERROR | LIBXML_NOWARNING); $textContent = strip_tags($dom->textContent); echo $textContent; // 输出: Hello World
Q2: 如果需要从CSV格式的字符串中提取每一列的数据怎么办?
A2: 可以使用str_getcsv()
函数将CSV字符串转换为数组,然后遍历数组提取数据。
$csvString = "John,Doe,30 Jane,Smith,25"; $rows = str_getcsv($csvString, " "); foreach ($rows as $row) { $columns = str_getcsv($row, ","); list($firstName, $lastName, $age) = $columns; echo "Name: $firstName $lastName, Age: $age "; } // 输出: // Name: John Doe, Age: 30 // Name: Jane Smith, Age: 25
小编有话说
从PHP字符串中提取特定数据是开发过程中经常遇到的问题,通过掌握正则表达式、字符串函数以及它们的组合使用,我们可以高效地完成各种复杂的数据提取任务,希望本文提供的方法和示例能够帮助你在实际项目中更加得心应手地处理字符串,如果你有任何疑问或需要进一步的帮助,请随时留言交流!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1379263.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复