CEF3与JavaScript集成详解
一、介绍
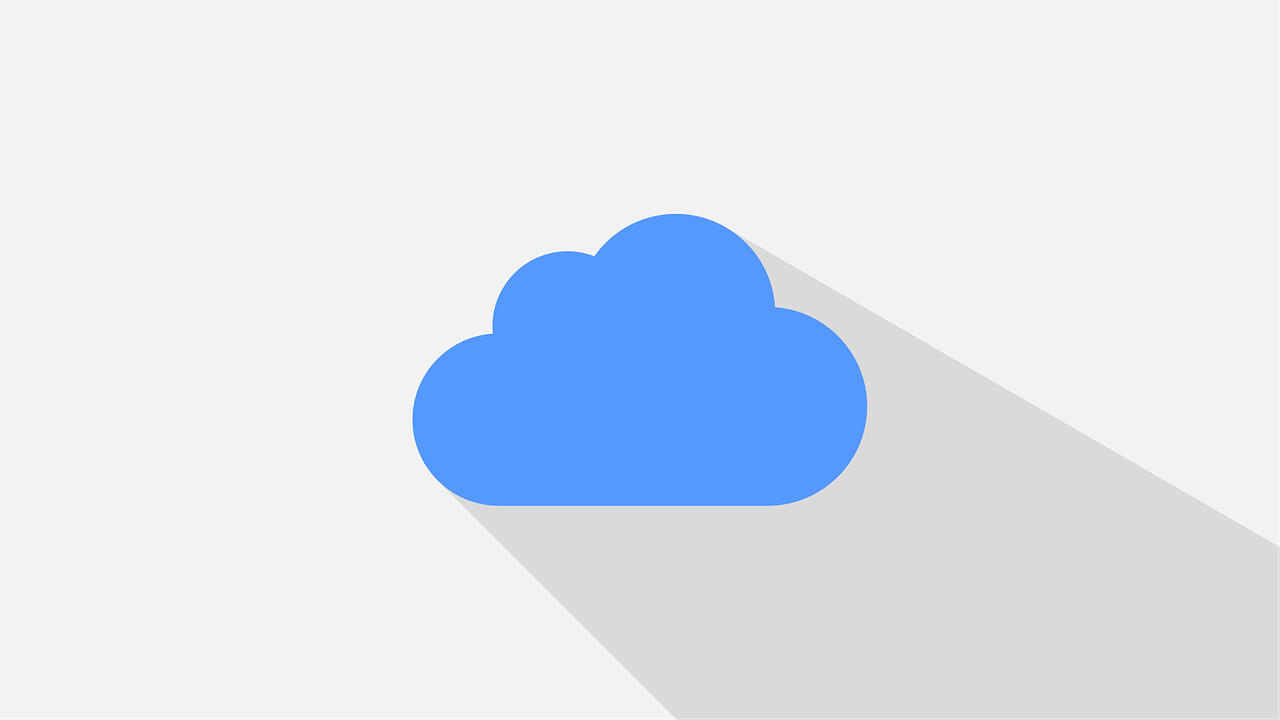
CEF(Chromium Embedded Framework)是一个开源框架,用于在应用程序中嵌入Chromium浏览器引擎,它允许开发者利用现代Web技术来创建丰富的用户界面和交互体验,CEF3是其第三个主要版本,提供了对JavaScript的全面支持,通过V8 JavaScript引擎实现高效的JavaScript执行。
二、执行JavaScript
1. 使用CefFrame::ExecuteJavaScript()函数
在CEF中,执行JavaScript代码最直接的方式是使用CefFrame::ExecuteJavaScript()
函数,该函数可以在浏览器进程和渲染进程中使用,且能够在JS上下文之外安全执行。
CefRefPtr<CefBrowser> browser = ...; CefRefPtr<CefFrame> frame = browser->GetMainFrame(); frame->ExecuteJavaScript("alert('ExecuteJavaScript works!');", frame->GetURL(), 0);
上述代码将在浏览器的主窗口中弹出一个提示框,显示“ExecuteJavaScript works!”,此方法适用于简单的JavaScript代码执行和与页面中的函数或变量进行交互。
2. 回调机制
对于需要从JavaScript返回数据到C++的情况,可以使用回调函数,在C++端定义一个回调处理函数,然后在JavaScript中调用该回调函数并传递数据。
class MyV8Handler : public CefV8Handler { public: MyV8Handler() {} virtual bool Execute(const CefString& name, CefRefPtr<CefV8Value> object, const CefV8ValueList& arguments, CefRefPtr<CefV8Value>& retval, CefString& exception) OVERRIDE { if (name == "getData") { // 返回数据 retval = CefV8Value::CreateString("Hello from C++"); return true; } return false; } }; void BindJavaScriptFunction(CefRefPtr<CefV8Context> context) { CefRefPtr<CefV8Value> global = context->GetGlobal(); CefRefPtr<CefV8Handler> handler = new MyV8Handler(); CefRefPtr<CefV8Value> func = CefV8Value::CreateFunction("getData", handler); global->SetValue("getData", func, V8_PROPERTY_ATTRIBUTE_NONE); }
在JavaScript中,可以直接调用绑定的函数:
let data = getData(); console.log(data); // 输出 "Hello from C++"
三、窗口绑定
窗口绑定允许客户端应用程序将值附加到窗口的window对象上,以便在JavaScript中访问,这通常通过实现CefRenderProcessHandler::OnContextCreated()
函数来实现。
void MyRenderProcessHandler::OnContextCreated(CefRefPtr<CefBrowser> browser, CefRefPtr<CefFrame> frame, CefRefPtr<CefV8Context> context) { // 获取window对象 CefRefPtr<CefV8Value> windowObject = context->GetGlobal(); // 创建一个JS字符串值 CefRefPtr<CefV8Value> str = CefV8Value::CreateString("My Value!"); // 将字符串添加到window对象作为"window.myval" windowObject->SetValue("myval", str, V8_PROPERTY_ATTRIBUTE_NONE); }
在JavaScript中,可以这样访问绑定的值:
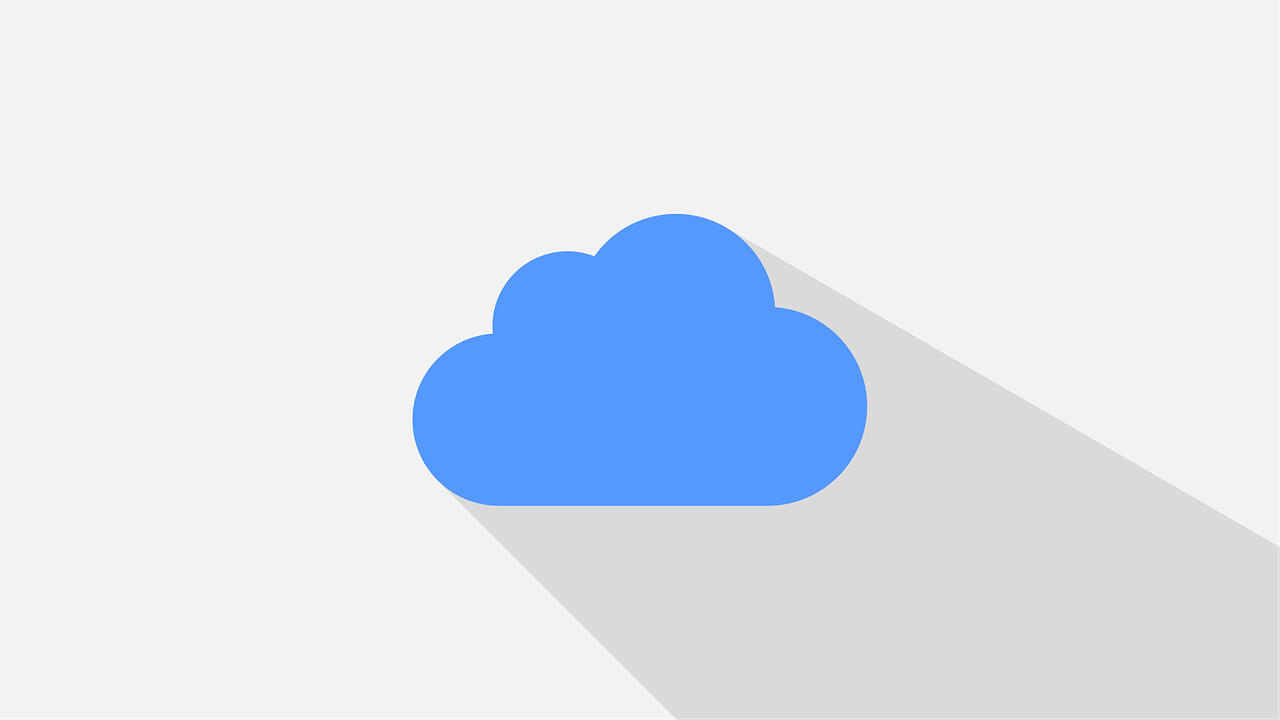
alert(window.myval); // 显示一个带有"My Value!"的提示框
四、扩展
扩展类似于窗口绑定,但它是在每个框架的上下文中加载,并且一旦加载就不能修改,扩展应该在CefRenderProcessHandler::OnWebKitInitialized()
函数中使用CefRegisterExtension
函数注册。
void MyRenderProcessHandler::OnWebKitInitialized() { std::string extensionCode = R"(var test; if (!test) test = {}; (function() { test.myval = 'My Value!'; })();)"; CefRegisterExtension("v8/test", extensionCode, NULL); }
在JavaScript中,可以这样访问扩展中的值:
alert(test.myval); // 显示一个带有"My Value!"的提示框
五、基本JS类型
CEF支持多种基本的JavaScript数据类型,包括undefined, null, bool, int, double, date和string,这些类型可以通过CefV8Value::Create*()
系列静态函数创建,创建一个JS字符串:
CefRefPtr<CefV8Value> str = CefV8Value::CreateString("My Value!");
检测值类型可以使用Is*()
系列函数:
CefRefPtr<CefV8Value> val = ...; if (val.IsString()) { // The value is a string. }
六、JS数组和对象
1. JS数组
使用CefV8Value::CreateArray()
函数并传递一个长度参数来创建数组,数组只能在上下文内部创建和使用:
CefRefPtr<CefV8Value> arr = CefV8Value::CreateArray(2); arr->SetValue(0, CefV8Value::CreateString("My First String!")); arr->SetValue(1, CefV8Value::CreateString("My Second String!"));
检查是否为数组以及获取数组长度:
bool isArray = arr->IsArray(); int length = arr->GetArrayLength();
2. JS对象
使用CefV8Value::CreateObject()
函数创建对象,并通过SetValue()
方法添加属性:
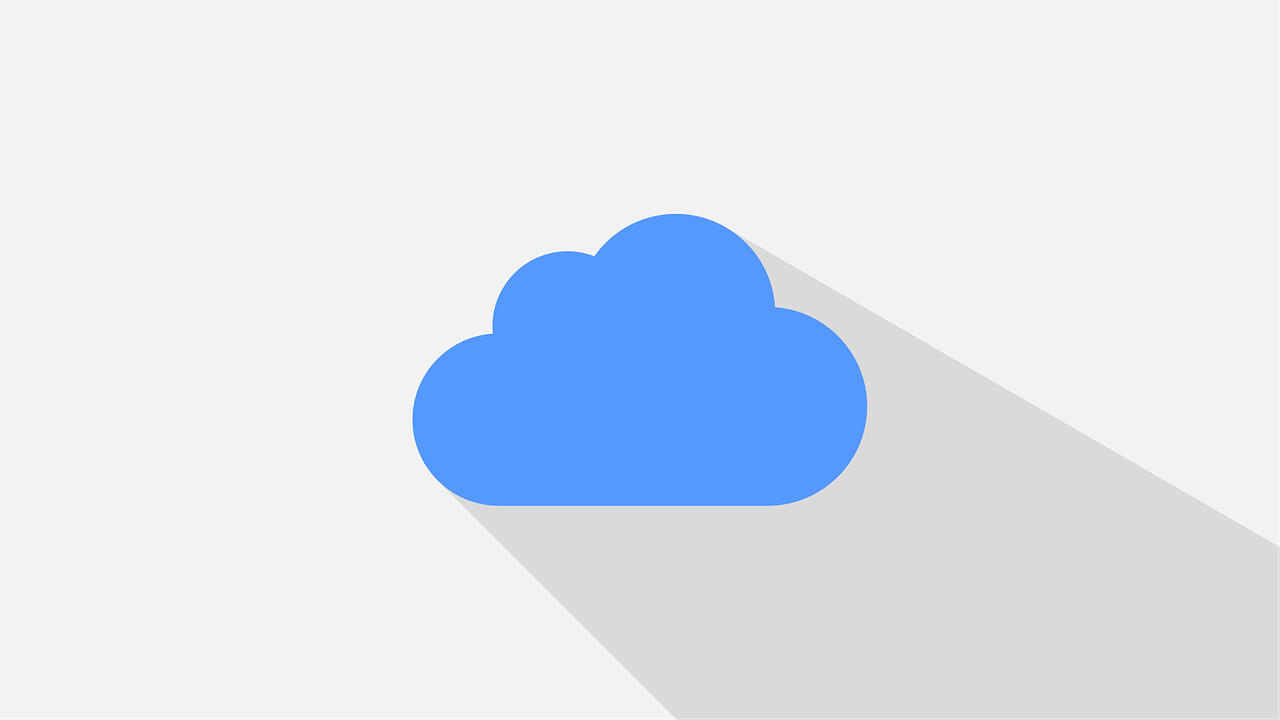
CefRefPtr<CefV8Value> obj = CefV8Value::CreateObject(); obj->SetValue("key", CefV8Value::CreateString("value"), V8_PROPERTY_ATTRIBUTE_NONE);
七、JavaScript与C++交互
除了直接调用JavaScript函数外,还可以通过消息机制在JavaScript和C++之间传递消息,这通常涉及在C++端设置消息处理回调,并在JavaScript中发送消息。
// C++端设置消息处理回调 browser_->SendProcessMessage(PID_BROWSER, message); // JavaScript端发送消息 window.postMessage("Hello from JS", "*");
CEF3通过V8 JavaScript引擎实现了强大的JavaScript支持,允许开发者在客户端应用程序中灵活地执行JavaScript代码、与页面进行交互以及扩展浏览器功能,通过掌握本文介绍的各种方法和技术,开发者可以充分利用CEF3的潜力,构建出功能强大且用户体验优异的应用程序。
以上内容就是解答有关“cef3 js”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1378711.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复