sort
函数用于对列表进行排序,默认按升序排列,可以通过参数设置降序或其他自定义排序方式。C++中的sort函数详解与应用
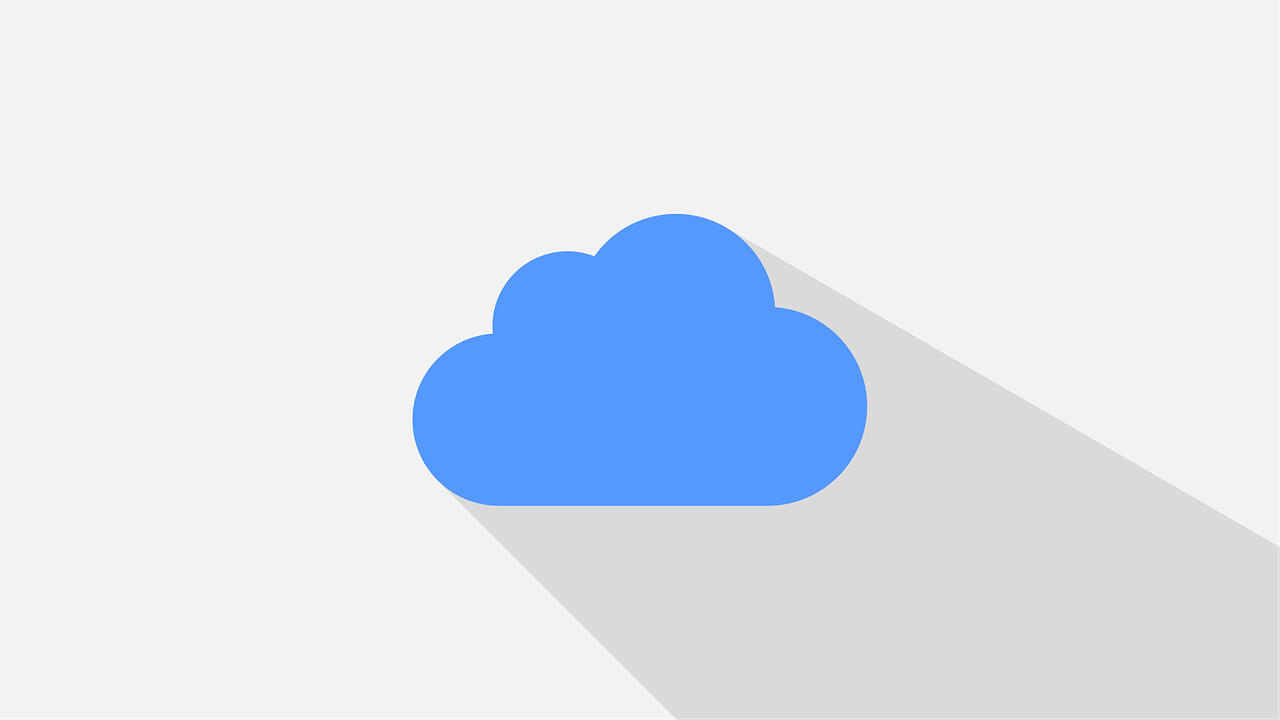
背景和简介
C++中的sort
函数是一个强大的工具,用于对容器或数组中的元素进行排序,它位于标准模板库(STL)的<algorithm>
头文件中,提供了高效且灵活的排序功能,默认情况下,sort
函数使用升序排序,但通过提供自定义的比较函数,可以实现降序或其他复杂的排序需求。
sort
函数的基本用法
包含必要头文件
要使用sort
函数,首先需要包含<algorithm>
头文件。
#include <algorithm>
基本语法
void sort(RandomAccessIterator first, RandomAccessIterator last); void sort(RandomAccessIterator first, RandomAccessIterator last, Compare comp);
first: 指向待排序区间的第一个元素。
last: 指向待排序区间最后一个元素的下一个位置。
comp: 可选参数,用于指定排序规则的比较函数对象,如果省略,则默认为升序排序。
示例代码
以下是一个简单的示例,演示如何使用sort
函数对整数数组进行升序排序:
#include <iostream> #include <algorithm> using namespace std; int main() { int arr[] = {9, 6, 3, 8, 5, 2, 7, 4, 1, 0}; sort(arr, arr + 10); // 对整个数组进行升序排序 for(int i = 0; i < 10; i++) { cout << arr[i] << " "; } return 0; }
输出结果:
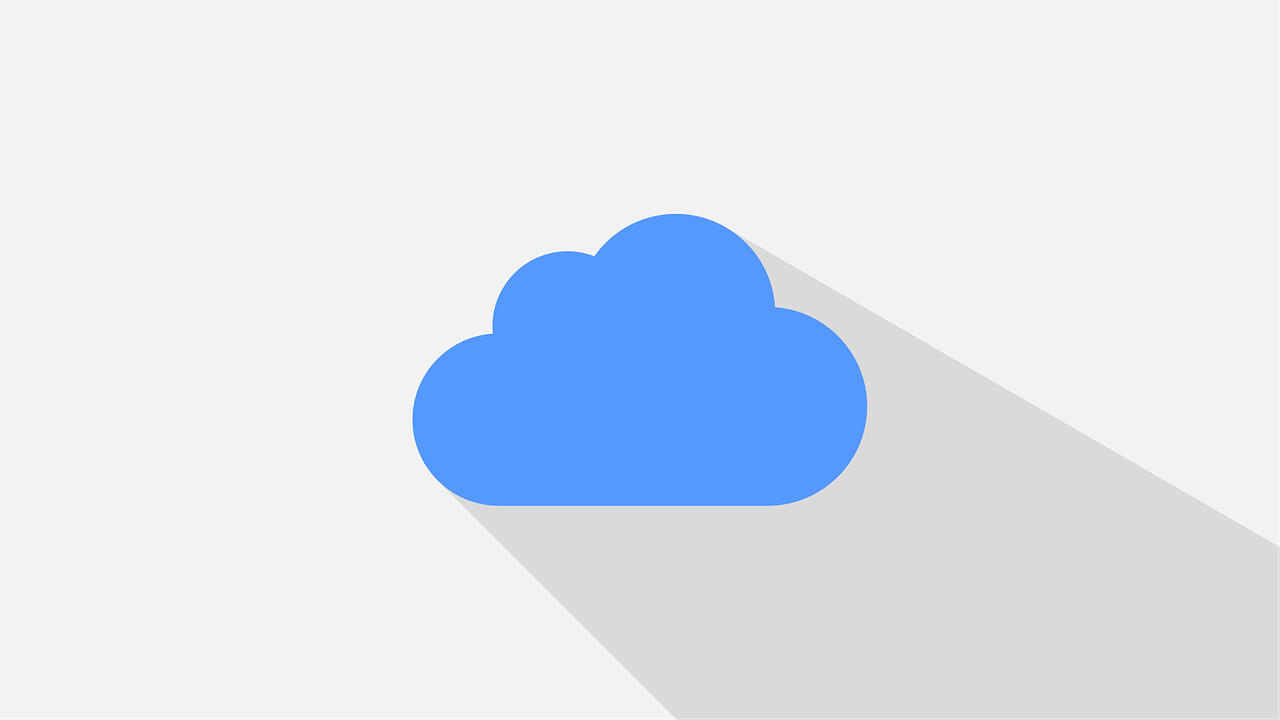
0 1 2 3 4 5 6 7 8 9
高级用法
降序排序
要实现降序排序,可以使用标准库提供的greater<>
函数对象:
#include <iostream> #include <algorithm> using namespace std; int main() { int arr[] = {9, 6, 3, 8, 5, 2, 7, 4, 1, 0}; sort(arr, arr + 10, greater<int>()); // 使用greater进行降序排序 for(int i = 0; i < 10; i++) { cout << arr[i] << " "; } return 0; }
输出结果:
9 8 7 6 5 4 3 2 1 0
自定义排序准则
用户还可以定义自己的比较函数来实现特定的排序需求,按绝对值大小排序:
#include <iostream> #include <algorithm> #include <cmath> // for abs function using namespace std; bool compare(int a, int b) { return abs(a) < abs(b); // 按绝对值升序排序 } int main() { int arr[] = {-9, -6, -3, -8, -5, -2, -7, -4, -1, -0}; sort(arr, arr + 10, compare); // 使用自定义比较函数 for(int i = 0; i < 10; i++) { cout << arr[i] << " "; } return 0; }
输出结果:
-1 -2 -3 -4 -5 -6 -7 -8 -9
结构体排序
对于结构体数组,可以通过自定义比较函数来排序,按学生的成绩从高到低排序,如果成绩相同则按姓名字典序排序:
#include <iostream> #include <algorithm> #include <string> using namespace std; struct Student { string name; int score; }; bool compareStudents(const Student &a, const Student &b) { if (a.score != b.score) return a.score > b.score; // 成绩从高到低 return a.name < b.name; // 成绩相同按姓名字典序 } int main() { Student students[] = {{"Alice", 90}, {"Bob", 90}, {"Charlie", 85}}; sort(students, students + 3, compareStudents); // 使用自定义比较函数排序 for(const auto &student : students) { cout << student.name << " " << student.score << endl; } return 0; }
输出结果:
Bob 90 Alice 90 Charlie 85
相关问答FAQs
Q1:sort
函数的时间复杂度是多少?
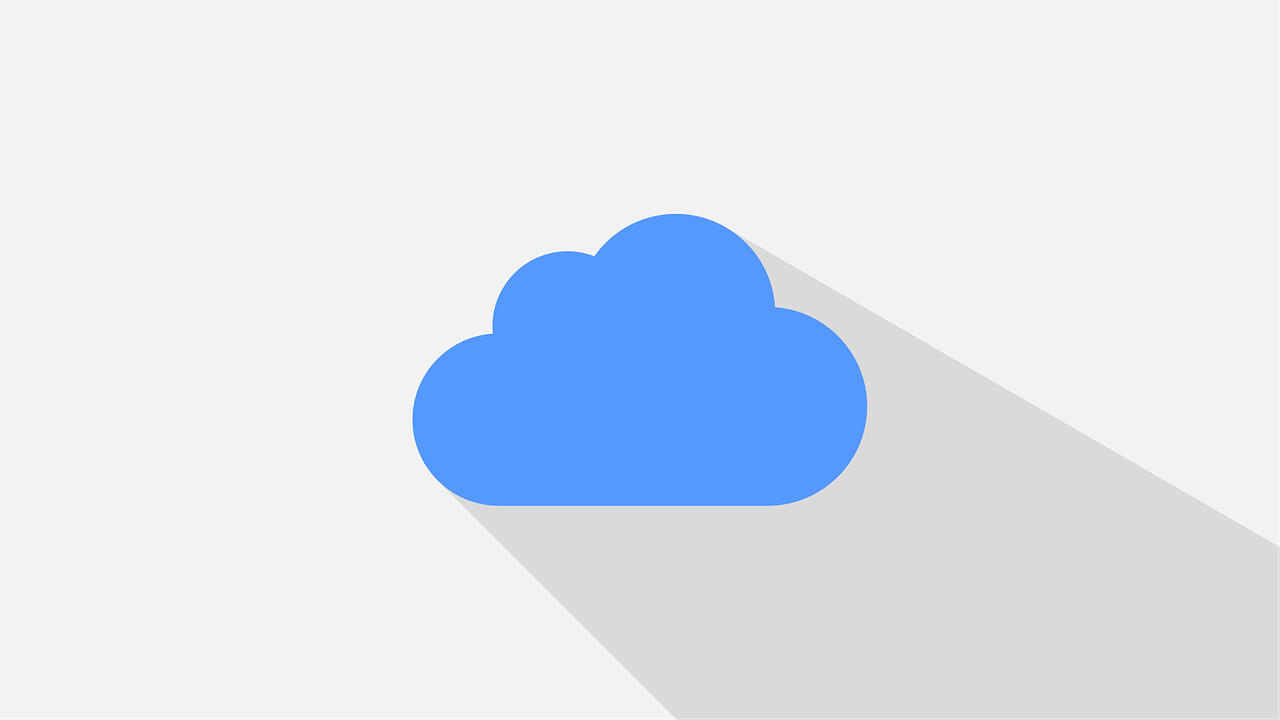
A1:sort
函数使用的是混合了快速排序、插入排序和堆排序的算法,平均时间复杂度为O(n log n),其中n是待排序元素的数量,在最坏情况下,时间复杂度也是O(n log n),由于其高效的性能,sort
函数通常是排序操作的首选。
Q2: 为什么在使用sort
函数时需要包含<algorithm>
头文件?
A2:<algorithm>
头文件包含了STL中所有算法相关的函数声明和模板,包括sort
函数,包含这个头文件是为了确保编译器能够识别并正确处理sort
函数的调用,如果不包含该头文件,编译器将无法找到sort
函数的定义,导致编译错误,在使用sort
函数之前,必须包含<algorithm>
头文件。
以上就是关于“sort函数”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1377181.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复