HttpClient
类来发送请求,并使用 CookieContainer
来管理会话。以下是一个简单的示例代码:,,“csharp,using System;,using System.Net;,using System.Net.Http;,using System.Threading.Tasks;,,public class WebScraper,{, private readonly HttpClient _httpClient;,, public WebScraper(), {, _httpClient = new HttpClient(new HttpClientHandler { CookieContainer = new CookieContainer() });, },, public async Task LoginAndGetContentAsync(string loginUrl, string targetUrl, string username, string password), {, var loginData = new FormUrlEncodedContent(new[], {, new KeyValuePair("username", username),, new KeyValuePair("password", password), });,, // Send login request, var loginResponse = await _httpClient.PostAsync(loginUrl, loginData);, if (!loginResponse.IsSuccessStatusCode), {, throw new Exception("Login failed");, },, // Get content from the target page after login, var contentResponse = await _httpClient.GetAsync(targetUrl);, if (!contentResponse.IsSuccessStatusCode), {, throw new Exception("Failed to get content");, },, return await contentResponse.Content.ReadAsStringAsync();, },},
`,,这个示例展示了如何创建一个
WebScraper 类,该类包含一个方法
LoginAndGetContentAsync`,用于登录并获取目标页面的内容。在ASP.NET和C#中,采集需要登录页面的数据通常涉及模拟用户登录并获取会话信息,以下是实现这一目标的基本原理及代码示例:
实现原理
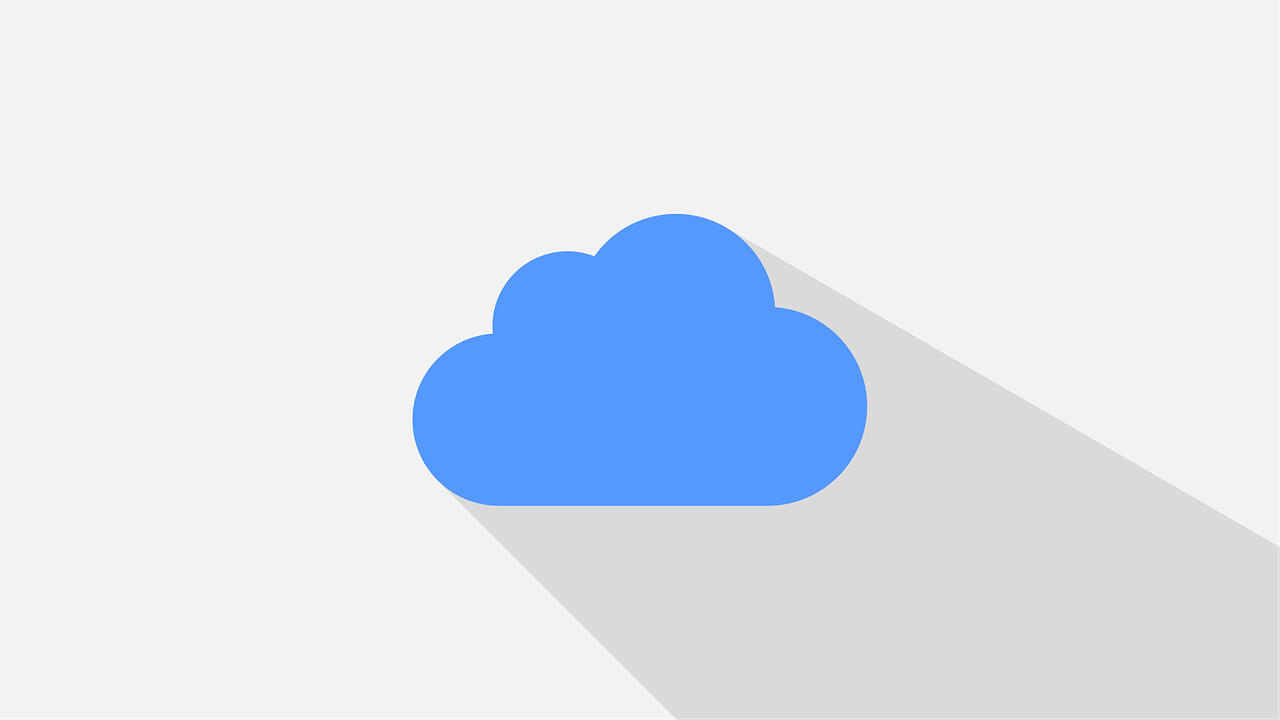
1、模拟登录请求:通过发送HTTP请求到登录页面,提交用户的登录凭证(如用户名和密码)。
2、处理响应:接收服务器返回的响应,通常会包含一个会话Cookie或其他形式的会话标识符。
3、保存会话信息:将获得的会话信息保存下来,以便后续请求中使用,保持登录状态。
4、发起后续请求:使用保存的会话信息发起对目标页面的请求,从而采集所需数据。
代码示例
以下是一个使用C#和HttpClient类实现上述步骤的简化代码示例:
using System; using System.Net.Http; using System.Net.Http.Headers; using System.Threading.Tasks; class Program { static async Task Main(string[] args) { string loginUrl = "https://example.com/login"; string targetUrl = "https://example.com/protected-page"; string username = "yourUsername"; string password = "yourPassword"; using (HttpClient client = new HttpClient()) { // Step 1: Create a form to hold login credentials var loginData = new FormUrlEncodedContent(new[] { new KeyValuePair<string, string>("username", username), new KeyValuePair<string, string>("password", password) }); // Step 2: Send the login request and receive the response HttpResponseMessage loginResponse = await client.PostAsync(loginUrl, loginData); if (loginResponse.IsSuccessStatusCode) { // Step 3: Save the session cookie from the login response var cookies = loginResponse.Headers.ToList(); client.DefaultRequestHeaders.Add("Cookie", cookies.ToString()); // Step 4: Use the saved session information to access the protected page HttpResponseMessage targetResponse = await client.GetAsync(targetUrl); if (targetResponse.IsSuccessStatusCode) { string pageContent = await targetResponse.Content.ReadAsStringAsync(); Console.WriteLine(pageContent); } else { Console.WriteLine("Failed to access the protected page. Status code: " + targetResponse.StatusCode); } } else { Console.WriteLine("Login failed. Status code: " + loginResponse.StatusCode); } } } }
相关问答FAQs
Q1: 如果目标网站使用了JavaScript进行登录验证,上述方法是否还适用?
A1: 不适用,上述方法适用于传统的表单提交登录,如果目标网站使用JavaScript进行登录验证,可能需要使用更高级的工具或库,如Selenium或Puppeteer,这些工具可以模拟浏览器行为,包括执行JavaScript。
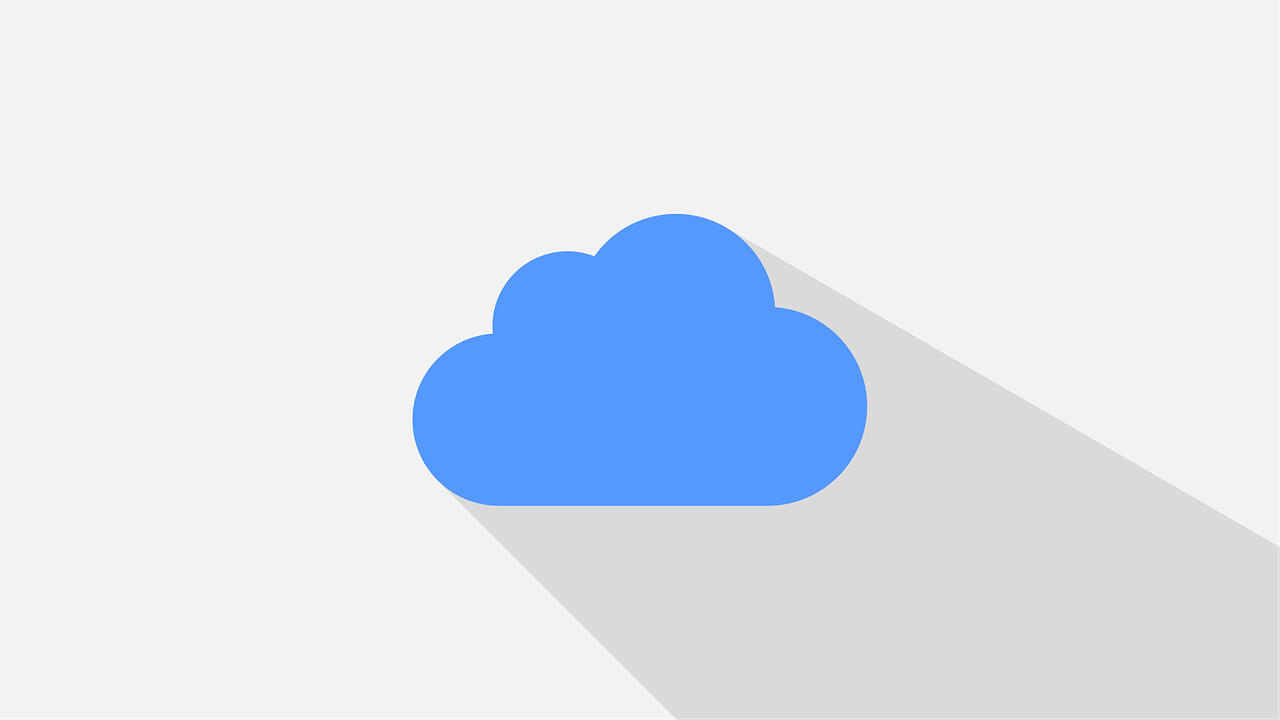
Q2: 如何处理HTTPS协议下的登录和数据采集?
A2: 在使用HttpClient时,默认情况下它会自动处理HTTPS请求,如果目标网站使用了自签名证书或需要客户端证书验证,则需要配置HttpClientHandler来信任这些证书或提供必要的客户端证书,这可以通过设置HttpClient的ServerCertificateCustomValidationCallback来实现。
各位小伙伴们,我刚刚为大家分享了有关“asp.net c#采集需要登录页面的实现原理及代码”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1372765.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复