IConfiguration
接口读取配置文件,如appsettings.json。在ASP.NET Core MVC中,配置管理是一个重要的部分,它允许开发者通过配置文件(如appsettings.json)来管理应用程序的各种参数,这些配置可以包括数据库连接字符串、API密钥、应用程序设置等,本文将详细介绍如何在ASP.NET Core MVC中查看和读取配置文件。
一、配置文件的结构
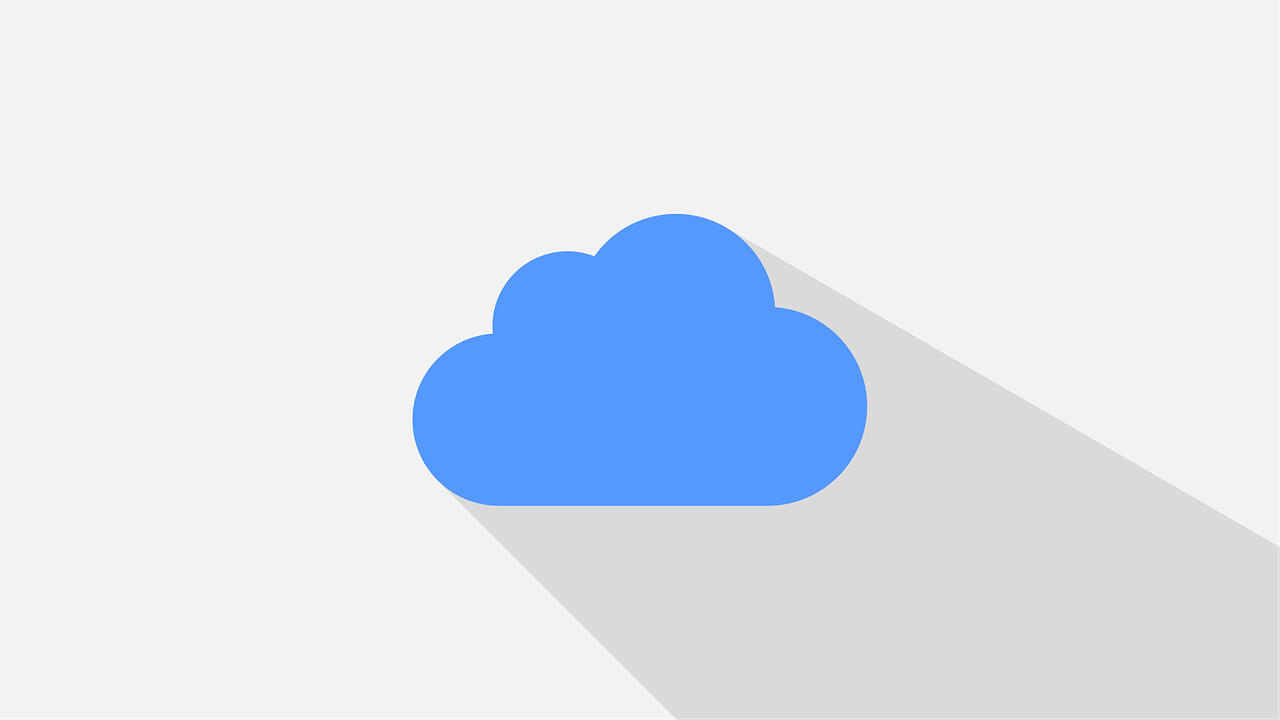
ASP.NET Core MVC使用JSON格式的配置文件,默认情况下是appsettings.json文件,该文件通常位于项目的根目录下,以下是一个典型的appsettings.json文件的例子:
{ "Logging": { "LogLevel": { "Default": "Information", "Microsoft": "Warning", "Microsoft.Hosting.Lifetime": "Information" } }, "AllowedHosts": "*", "ConnectionStrings": { "DefaultConnection": "Server=(localdb)\mssqllocaldb;Database=aspnet-CoreMVC-2d9b3;Trusted_Connection=True;MultipleActiveResultSets=true" }, "AppSettings": { "SecretKey": "your_secret_key" } }
二、读取配置文件的方法
在ASP.NET Core MVC中,读取配置文件主要通过IConfiguration
接口来实现,以下是几种常见的读取配置的方法:
1. 通过键名直接读取
这是最简单的方法,适用于读取简单的键值对,要读取AllowedHosts
的值,可以使用以下代码:
public class HomeController : Controller { private readonly IConfiguration _configuration; public HomeController(IConfiguration configuration) { _configuration = configuration; } public IActionResult Index() { string allowedHosts = _configuration["AllowedHosts"]; return Content(allowedHosts); } }
2. 读取复杂的配置对象
对于复杂的配置结构,可以创建一个与配置节结构相同的实体类,然后使用GetSection
方法将其绑定到该实体类上,要读取ConnectionStrings
节,可以定义一个实体类并绑定:
public class ConnectionStringsConfig { public string DefaultConnection { get; set; } } public class Startup { public void ConfigureServices(IServiceCollection services) { services.Configure<ConnectionStringsConfig>(Configuration.GetSection("ConnectionStrings")); services.AddSingleton<IConnectionStringsConfig>(Configuration.GetSection("ConnectionStrings").Get<ConnectionStringsConfig>()); } }
3. 使用IOptions
模式注入配置
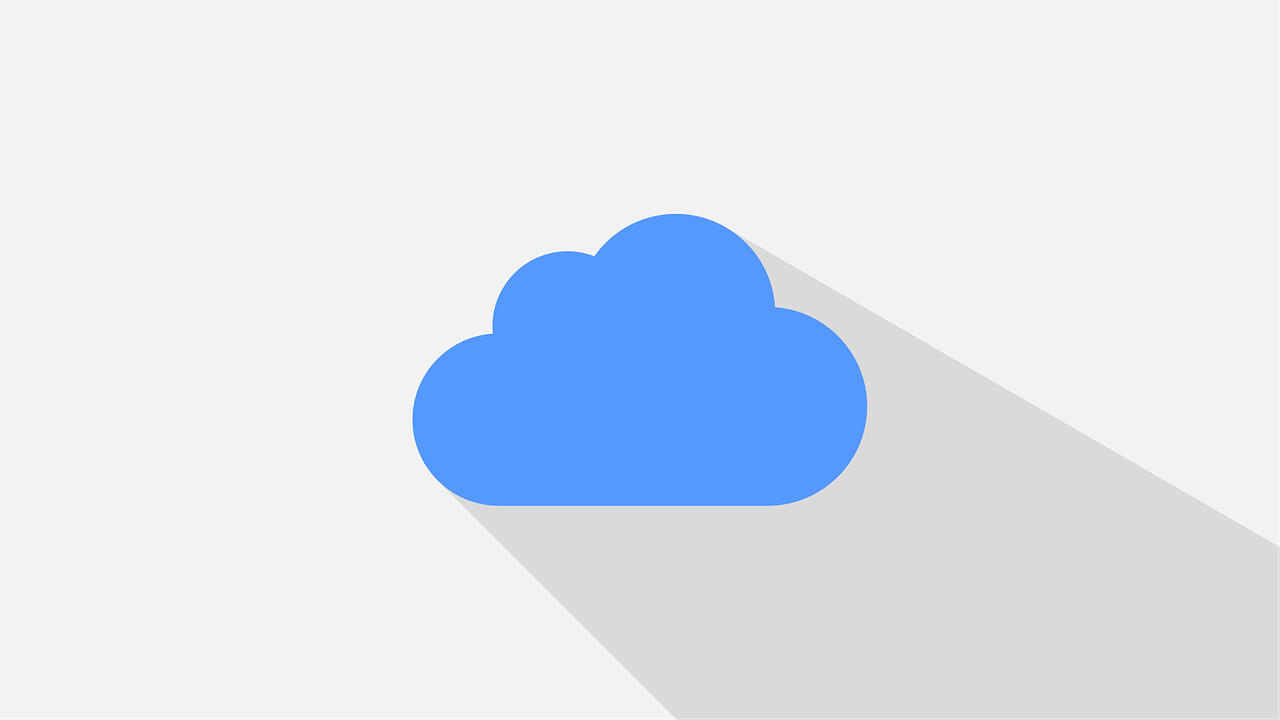
ASP.NET Core还提供了一种更优雅的方式来处理配置,即使用IOptions
模式,在Startup.cs
中配置服务:
services.Configure<MyOptions>(Configuration.GetSection("MyOptions"));
创建一个MyOptions
类:
public class MyOptions { public string Name { get; set; } public string Value { get; set; } }
在需要使用配置的地方注入IOptions<MyOptions>
:
public class HomeController : Controller { private readonly MyOptions _myOptions; public HomeController(IOptions<MyOptions> myOptions) { _myOptions = myOptions.Value; } public IActionResult Index() { string name = _myOptions.Name; return Content(name); } }
三、动态读取不同环境的配置
在开发过程中,经常需要根据不同的环境(如开发、测试、生产)加载不同的配置文件,ASP.NET Core支持这种功能,可以通过设置环境变量来指定要加载的配置文件,可以在launchSettings.json
中配置不同的环境变量:
{ "profiles": { "IIS Express": { "commandName": "IISExpress", "launchBrowser": true, "environmentVariables": { "ASPNETCORE_ENVIRONMENT": "Development" } }, "Production": { "commandName": "Project", "dotnetRunMessages": "true", "launchBrowser": true, "applicationUrl": "https://localhost:5001;http://localhost:5000", "environmentVariables": { "ASPNETCORE_ENVIRONMENT": "Production" } } } }
然后在Program.cs
中根据环境变量加载相应的配置文件:
public class Program { public static void Main(string[] args) { CreateWebHostBuilder(args).Build().Run(); } public static IWebHostBuilder CreateWebHostBuilder(string[] args) => WebHost.CreateDefaultBuilder(args) .ConfigureAppConfiguration((hostingContext, config) => { var env = hostingContext.HostingEnvironment; config.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true) .AddJsonFile($"appsettings.{env.EnvironmentName}.json", optional: true, reloadOnChange: true); }); }
四、相关问答FAQs
问:如何在ASP.NET Core MVC中更改配置文件的路径?
答:可以通过在Program.cs
中使用ConfigureAppConfiguration
方法来更改配置文件的路径。
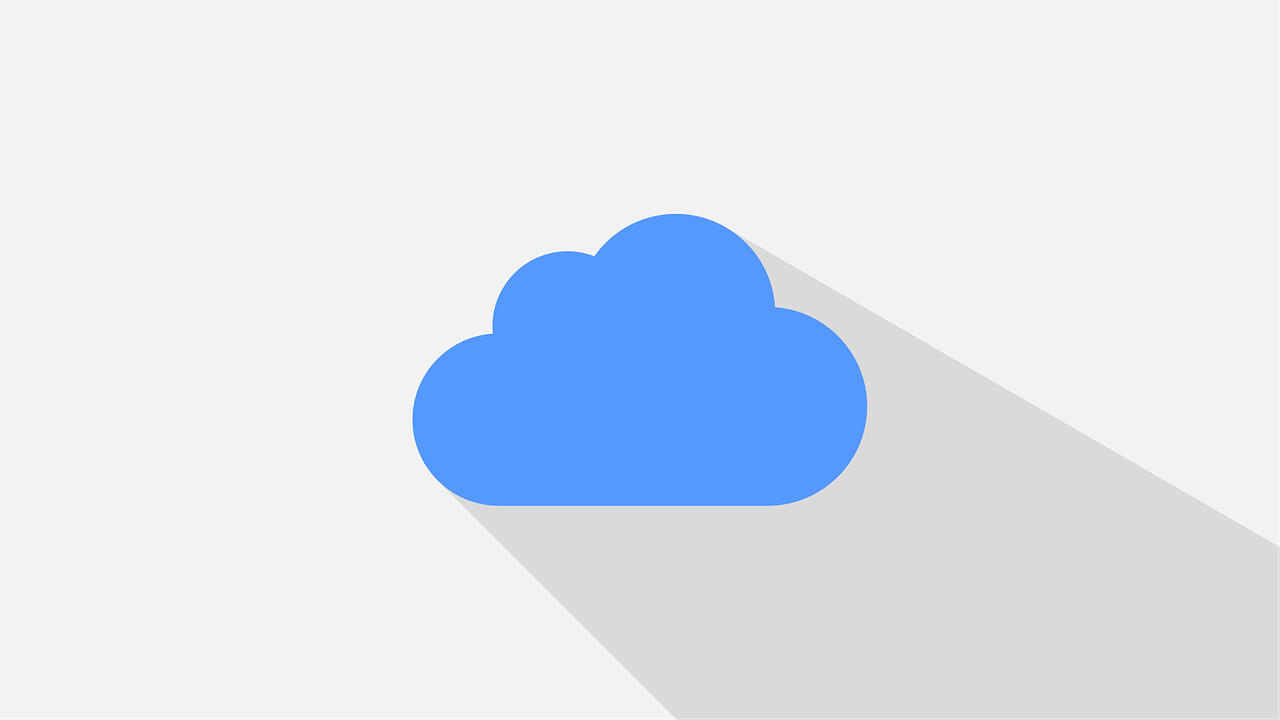
public static IWebHostBuilder CreateWebHostBuilder(string[] args) => WebHost.CreateDefaultBuilder(args) .ConfigureAppConfiguration((hostingContext, config) => { var env = hostingContext.HostingEnvironment; config.SetBasePath(Path.Combine(env.ContentRootPath, "CustomConfigFolder")) .AddJsonFile("appsettings.json", optional: false, reloadOnChange: true); });
这样就会从CustomConfigFolder
文件夹中加载配置文件。
问:如何在运行时重新加载配置文件?
答:ASP.NET Core支持在配置文件更改时自动重新加载配置,只需在添加配置文件时将reloadOnChange
参数设置为true
即可:
config.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true);
这样,当appsettings.json
文件发生变化时,配置将自动重新加载。
ASP.NET Core MVC通过IConfiguration
接口和IOptions
模式提供了灵活且强大的配置管理功能,使得开发者能够轻松地管理和读取应用程序的各种配置,无论是简单的键值对还是复杂的配置对象,都可以通过上述方法进行有效的管理。
以上内容就是解答有关“ASP.NET Core MVC如何看配置”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1372593.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复