csharp,// 上传Word文档,public void UploadDocument(string filePath),{, byte[] fileBytes = File.ReadAllBytes(filePath);, string connectionString = "your_connection_string";, using (SqlConnection conn = new SqlConnection(connectionString)), {, conn.Open();, using (SqlCommand cmd = new SqlCommand("INSERT INTO Documents (Content) VALUES (@Content)", conn)), {, cmd.Parameters.AddWithValue("@Content", fileBytes);, cmd.ExecuteNonQuery();, }, },},,// 显示Word文档,public byte[] GetDocument(int documentId),{, string connectionString = "your_connection_string";, using (SqlConnection conn = new SqlConnection(connectionString)), {, conn.Open();, using (SqlCommand cmd = new SqlCommand("SELECT Content FROM Documents WHERE Id = @Id", conn)), {, cmd.Parameters.AddWithValue("@Id", documentId);, using (SqlDataReader reader = cmd.ExecuteReader()), {, if (reader.Read()), {, return (byte[])reader["Content"];, }, }, }, }, return null;,},
`,,请确保替换
your_connection_string`为实际的数据库连接字符串,并根据需要调整表名和字段名。在ASP.NET中,使用C#语言实现将Word文档上传到SQL数据库并显示出来,是一个涉及文件处理、数据库操作和网页展示的综合任务,以下是详细的步骤和代码示例:
准备工作
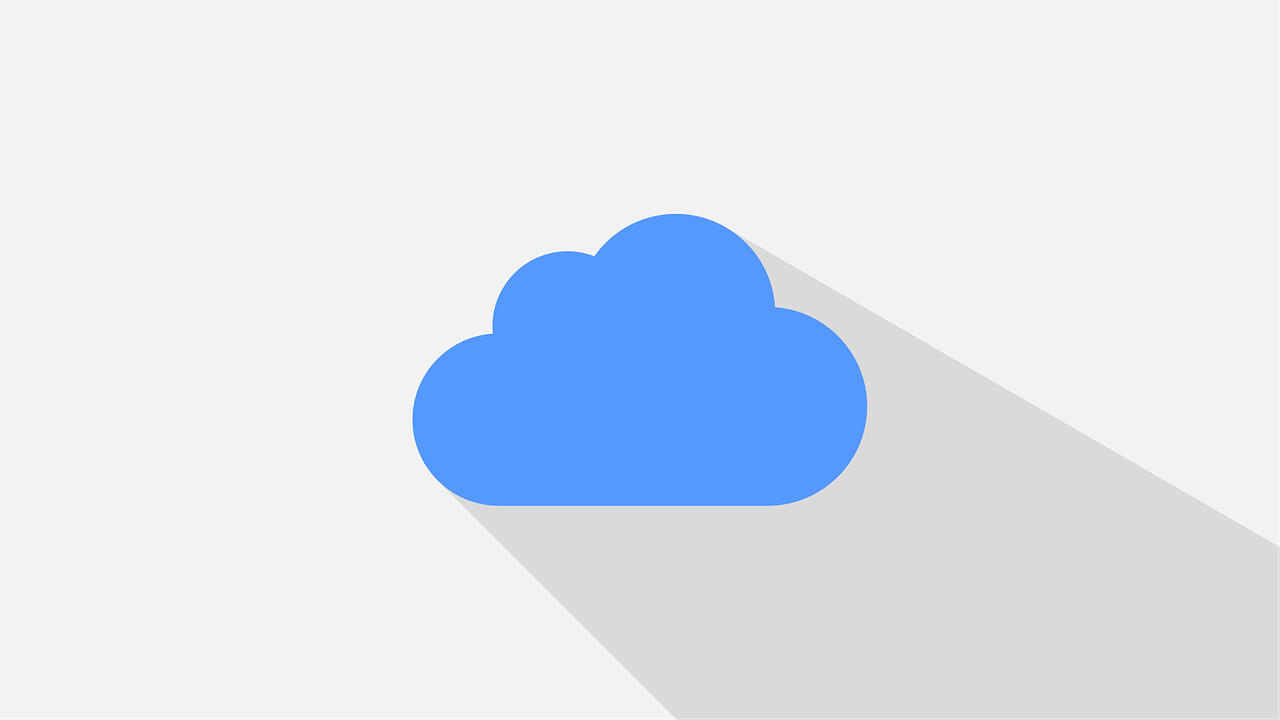
确保你已经安装了以下组件:
.NET Framework(例如4.7或更高版本)
Microsoft SQL Server
Entity Framework(可选,用于简化数据库操作)
创建数据库和表
在SQL Server中创建一个数据库和一个表来存储Word文档的内容。
CREATE DATABASE DocumentDB; USE DocumentDB; CREATE TABLE Documents ( Id INT PRIMARY KEY IDENTITY(1,1), FileName NVARCHAR(255), Content VARBINARY(MAX) );
创建ASP.NET Web应用程序
打开Visual Studio,创建一个新的ASP.NET Web应用程序项目。
添加必要的引用和命名空间
在你的项目中,添加对以下命名空间的引用:
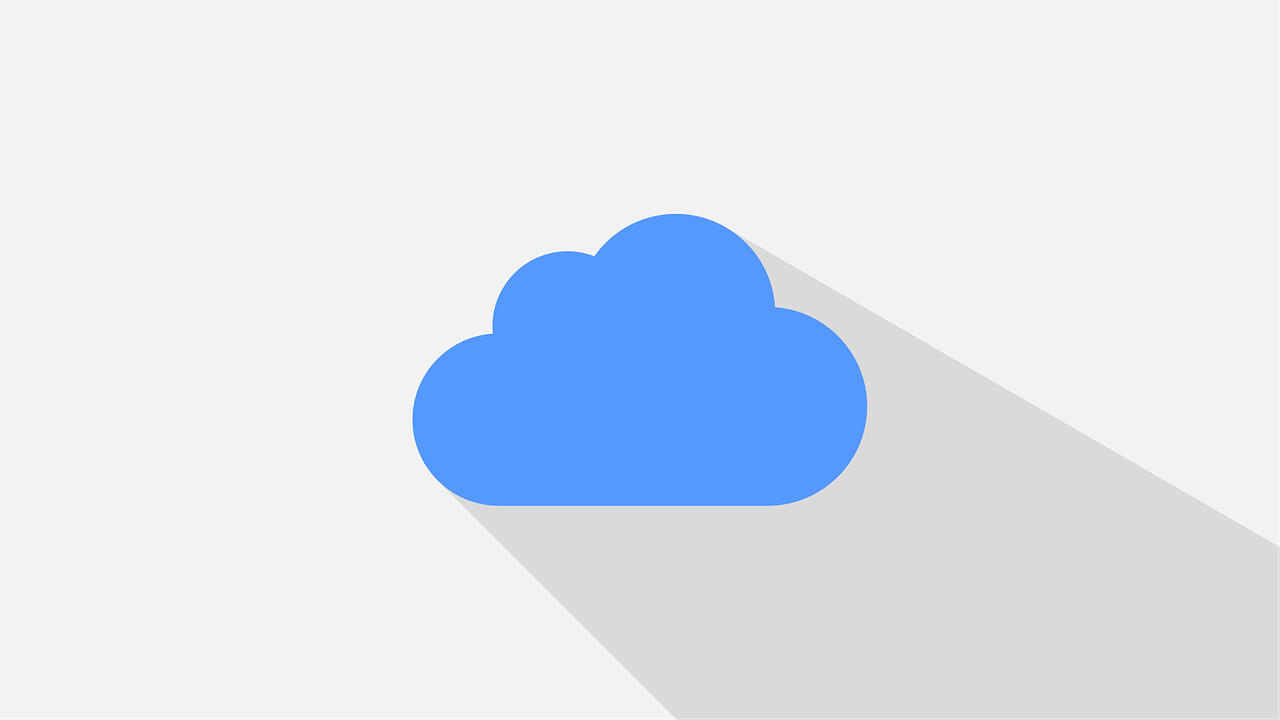
using System; using System.Data; using System.Data.SqlClient; using System.IO; using System.Web.UI; using System.Web.UI.WebControls;
设计上传界面
在Default.aspx
页面中,添加一个文件上传控件和一个按钮:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title>上传Word文档</title> </head> <body> <form id="form1" runat="server"> <div> 选择文件: <asp:FileUpload ID="FileUpload1" runat="server" /> <br /> <asp:Button ID="Button1" runat="server" Text="上传" OnClick="Button1_Click" /> </div> </form> </body> </html>
实现上传功能
在Default.aspx.cs
文件中,编写上传按钮的事件处理程序:
protected void Button1_Click(object sender, EventArgs e) { if (FileUpload1.HasFile) { try { // 获取文件名和内容 string fileName = FileUpload1.FileName; byte[] content = FileUpload1.FileBytes; // 连接到数据库并插入数据 using (SqlConnection connection = new SqlConnection("your_connection_string")) { connection.Open(); string query = "INSERT INTO Documents (FileName, Content) VALUES (@FileName, @Content)"; using (SqlCommand command = new SqlCommand(query, connection)) { command.Parameters.AddWithValue("@FileName", fileName); command.Parameters.AddWithValue("@Content", content); command.ExecuteNonQuery(); } } Response.Write("文件上传成功!"); } catch (Exception ex) { Response.Write("文件上传失败:" + ex.Message); } } else { Response.Write("请选择一个文件。"); } }
注意:将your_connection_string
替换为你的实际数据库连接字符串。
显示上传的文档
为了从数据库中检索并显示Word文档,你需要创建一个新页面或在同一页面上添加一个新部分,这里我们假设你创建了一个名为ViewDocument.aspx
的新页面。
在ViewDocument.aspx
页面中,添加一个按钮和一个标签来显示文档内容:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title>查看文档</title> </head> <body> <form id="form1" runat="server"> <div> <asp:Button ID="Button1" runat="server" Text="查看文档" OnClick="Button1_Click" /> <br /> <asp:Label ID="Label1" runat="server" Text=""></asp:Label> </div> </form> </body> </html>
在ViewDocument.aspx.cs
文件中,编写按钮的事件处理程序:
protected void Button1_Click(object sender, EventArgs e) { try { // 连接到数据库并检索数据 using (SqlConnection connection = new SqlConnection("your_connection_string")) { connection.Open(); string query = "SELECT * FROM Documents"; using (SqlCommand command = new SqlCommand(query, connection)) { using (SqlDataReader reader = command.ExecuteReader()) { if (reader.Read()) { // 获取文件名和内容 string fileName = reader["FileName"].ToString(); byte[] content = (byte[])reader["Content"]; // 保存到临时文件并显示 string tempPath = Path.Combine(Path.GetTempPath(), fileName); File.WriteAllBytes(tempPath, content); Label1.Text = "<a href='" + tempPath + "' target='_blank'>" + fileName + "</a>"; } else { Label1.Text = "没有找到文档。"; } } } } } catch (Exception ex) { Label1.Text = "查看文档失败:" + ex.Message; } }
注意:同样需要将your_connection_string
替换为你的实际数据库连接字符串。
运行应用程序
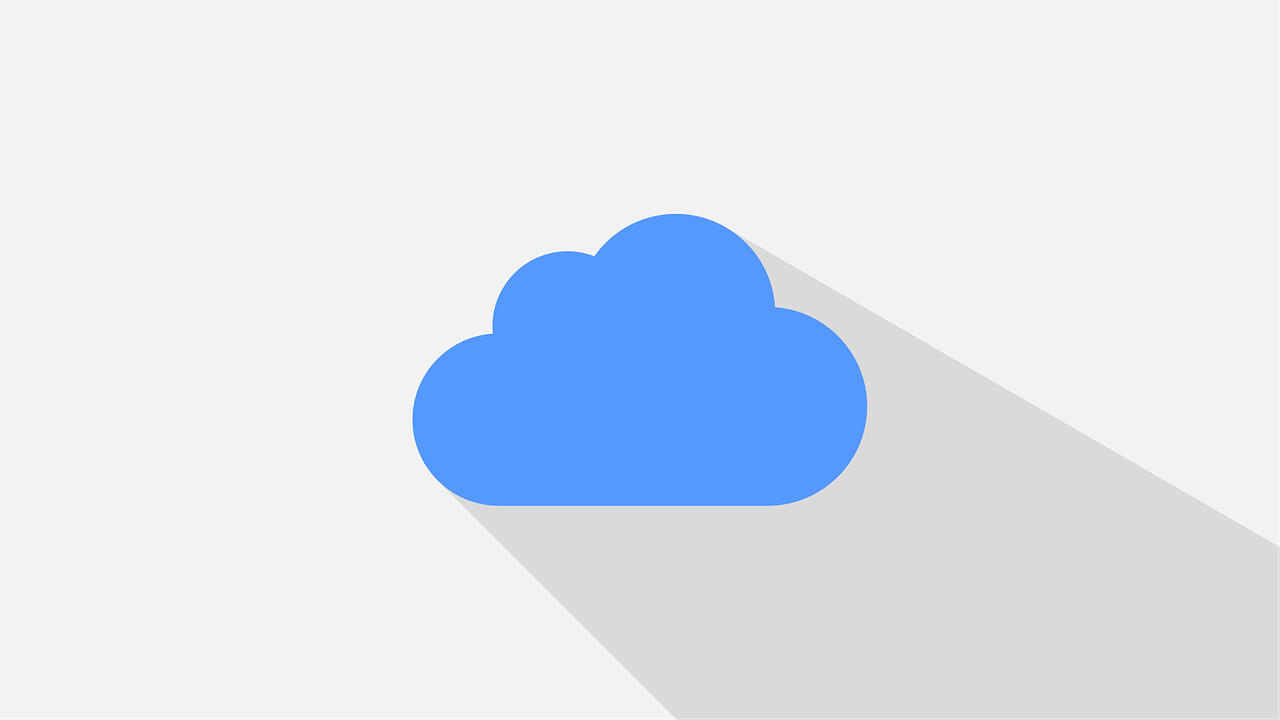
现在你可以运行你的应用程序了,在浏览器中访问Default.aspx
页面,选择一个Word文档进行上传,上传成功后,访问ViewDocument.aspx
页面查看上传的文档。
相关问答FAQs
Q1: 如何更改数据库连接字符串?
A1: 你可以在Web.config文件中设置数据库连接字符串,然后在代码中使用ConfigurationManager.ConnectionStrings["your_connection_string_name"].ConnectionString
来获取它。
<configuration> <connectionStrings> <add name="MyDbConnectionString" connectionString="your_connection_string" providerName="System.Data.SqlClient" /> </connectionStrings> </configuration>
然后在代码中使用:
string connectionString = ConfigurationManager.ConnectionStrings["MyDbConnectionString"].ConnectionString;
Q2: 如果上传的文件很大,如何处理?
A2: 对于大文件上传,可以考虑以下方法:
增加服务器的文件上传大小限制(例如在web.config中设置<httpRuntime maxRequestLength="..."/>
)。
使用分块上传技术,将文件分成多个小块分别上传。
优化数据库设计,考虑将文件存储在文件系统而非数据库中,仅在数据库中存储文件路径和元数据。
各位小伙伴们,我刚刚为大家分享了有关“asp.net c#上传word文档到sql数据库并显示出来”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1371874.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复