HttpClient
类发送 HTTP 请求,获取响应内容后使用 HtmlDocument
类解析 HTML 数据。在ASP.NET和C#中,抓取页面信息是一项常见的任务,通常用于数据挖掘、网页监控或内容聚合等场景,本文将详细介绍几种常用的方法来抓取页面信息,并附上相关问答FAQs。
方法一:使用HttpClient类
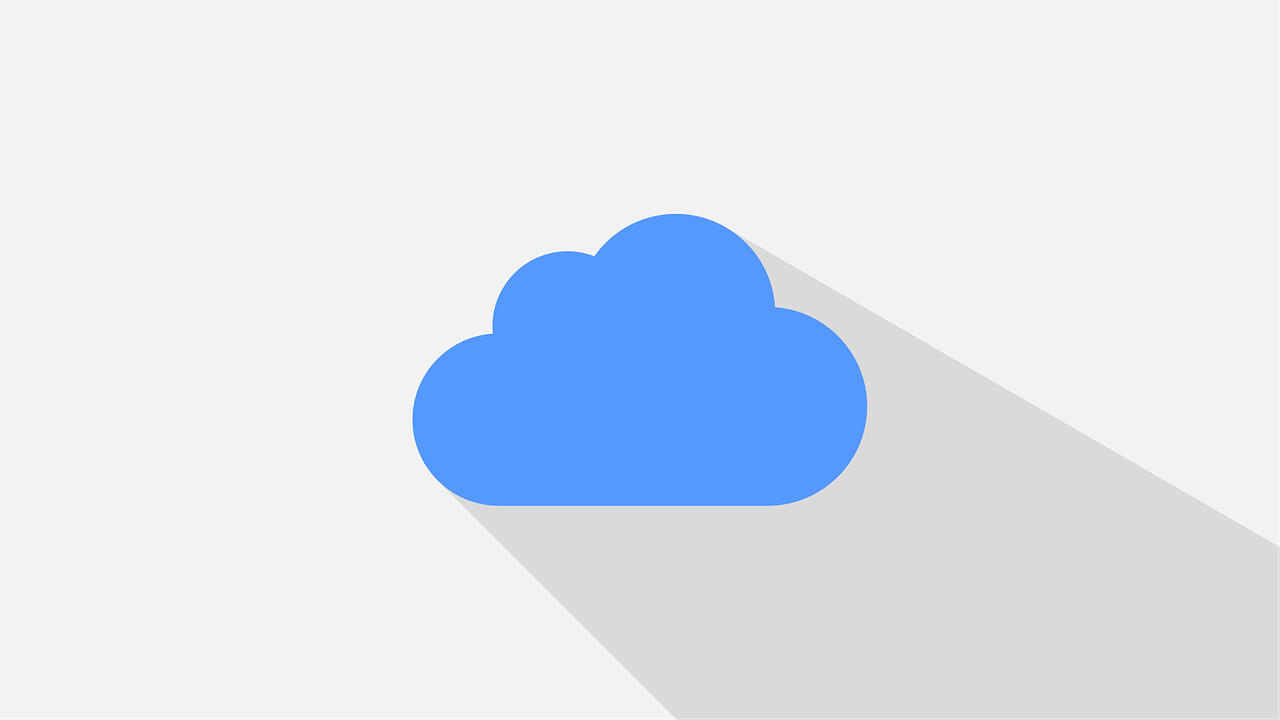
HttpClient
类是.NET框架中用于发送HTTP请求和接收HTTP响应的类,它非常适合用来抓取网页内容,以下是一个简单的示例:
using System; using System.Net.Http; using System.Threading.Tasks; class Program { static async Task Main(string[] args) { using (HttpClient client = new HttpClient()) { try { string url = "http://example.com"; HttpResponseMessage response = await client.GetAsync(url); response.EnsureSuccessStatusCode(); string responseBody = await response.Content.ReadAsStringAsync(); Console.WriteLine(responseBody); } catch (HttpRequestException e) { Console.WriteLine(" Exception Caught!"); Console.WriteLine("Message :{0} ", e.Message); } } } }
方法二:使用WebClient类
WebClient
类是另一个用于发送HTTP请求和接收HTTP响应的类,与HttpClient
相比,WebClient
更为简单,但在功能上稍显不足,以下是一个示例:
using System; using System.Net; class Program { static void Main() { string url = "http://example.com"; WebClient client = new WebClient(); try { string result = client.DownloadString(url); Console.WriteLine(result); } catch (WebException e) { Console.WriteLine(" Exception Caught!"); Console.WriteLine("Message :{0} ", e.Message); } } }
方法三:使用HtmlAgilityPack库
HtmlAgilityPack
是一个强大的HTML解析库,可以用来解析HTML文档并提取所需信息,以下是一个示例:
using System; using System.Net.Http; using HtmlAgilityPack; using System.Threading.Tasks; class Program { static async Task Main(string[] args) { using (HttpClient client = new HttpClient()) { try { string url = "http://example.com"; HttpResponseMessage response = await client.GetAsync(url); response.EnsureSuccessStatusCode(); string responseBody = await response.Content.ReadAsStringAsync(); HtmlDocument doc = new HtmlDocument(); doc.LoadHtml(responseBody); // 示例:获取页面标题 var titleNode = doc.DocumentNode.SelectSingleNode("//title"); if (titleNode != null) { Console.WriteLine("Page Title: " + titleNode.InnerText); } } catch (HttpRequestException e) { Console.WriteLine(" Exception Caught!"); Console.WriteLine("Message :{0} ", e.Message); } } } }
方法四:使用Selenium WebDriver
Selenium WebDriver
是一个自动化测试工具,也可以用来抓取动态生成的内容,以下是一个示例:
using OpenQA.Selenium; using OpenQA.Selenium.Chrome; using System; using System.Threading; class Program { static void Main() { IWebDriver driver = new ChromeDriver(); driver.Navigate().GoToUrl("http://example.com"); Thread.Sleep(5000); // 等待页面加载完成 string pageSource = driver.PageSource; Console.WriteLine(pageSource); driver.Quit(); } }
表格对比各方法特点
方法 | 优点 | 缺点 | 适用场景 |
HttpClient | 功能强大,支持异步操作 | 需要处理更多的细节 | 各种HTTP请求场景 |
WebClient | 简单易用 | 功能有限,不支持异步操作 | 简单的HTTP请求场景 |
HtmlAgilityPack | 强大的HTML解析能力 | 需要额外的库 | HTML解析和数据提取 |
Selenium WebDriver | 能处理动态内容,支持浏览器自动化 | 依赖浏览器驱动,性能开销大 | 动态内容抓取,自动化测试 |
相关问答FAQs
问题1:哪种方法最适合抓取动态生成的内容?
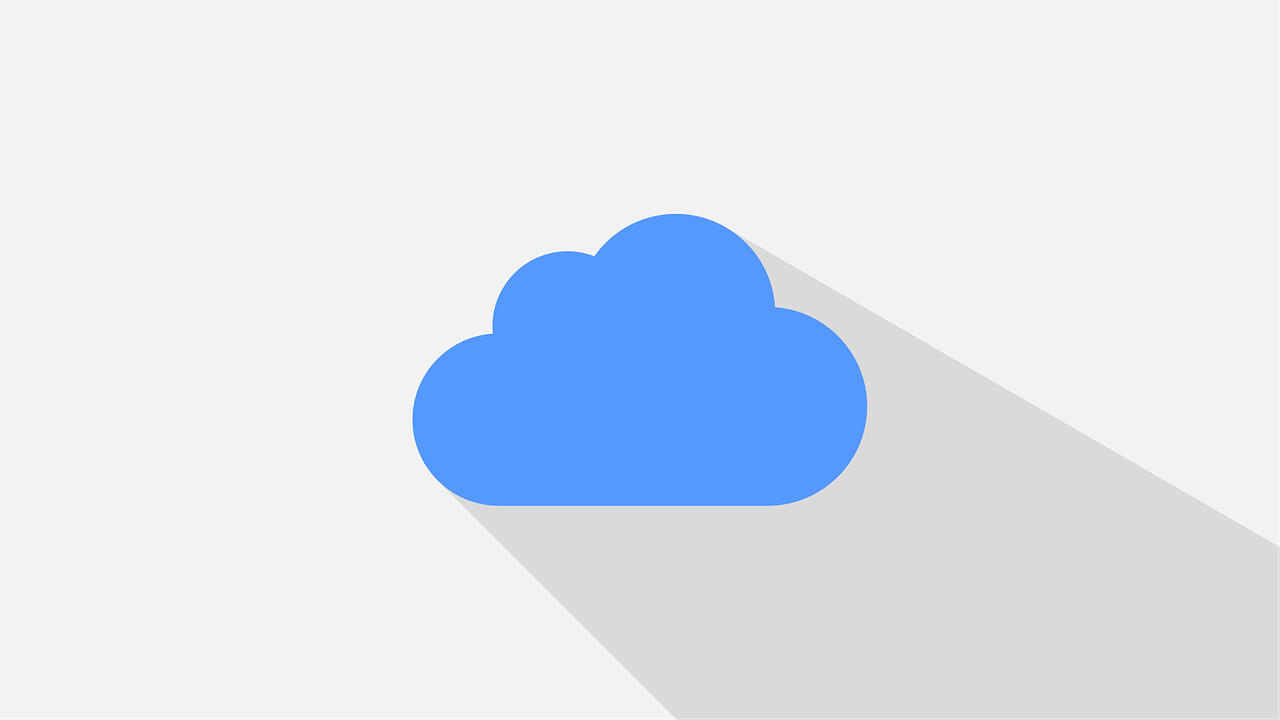
回答:Selenium WebDriver
最适合抓取动态生成的内容,因为它可以模拟用户行为,等待JavaScript执行完毕后再获取页面内容,但需要注意的是,这种方法的性能开销较大。
问题2:如何选择合适的抓取方法?
回答:选择抓取方法时需要考虑以下几个因素:目标网站的类型、抓取内容的复杂度、是否需要处理动态内容、性能要求以及开发难度,对于简单的静态页面,可以选择HttpClient
或WebClient
;对于需要解析HTML的情况,可以选择HtmlAgilityPack
;对于动态内容较多的网站,可以考虑使用Selenium WebDriver
。
小伙伴们,上文介绍了“asp.net c# 抓取页面信息方法介绍”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1371648.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复