html,,,,,Simple Rich Text Editor,, #editor { border: 1px solid #ccc; padding: 10px; width: 500px; height: 300px; }, .toolbar button { margin-right: 5px; },,,,,Bold,Italic,Underline,Bullet List,Numbered List,Link,Image,,,,, function execCmd(command, value = null) {, document.execCommand(command, false, value);, },,,,
`,,这个简单的富文本编辑器包含以下功能:,加粗 (
bold),斜体 (
italic),下划线 (
underline),无序列表 (
insertUnorderedList),有序列表 (
insertOrderedList),插入链接 (
createLink),插入图片 (
insertImage`),,通过点击工具栏上的按钮,可以执行相应的命令来编辑内容。在这篇文章中,我们将探讨如何使用不到200行的JavaScript代码实现一个基本的富文本编辑器,这个编辑器将包括一些常见的功能,如文字加粗、斜体、下划线、字体颜色和背景颜色的更改,为了简化实现,我们会使用HTML和CSS来构建用户界面,而JavaScript则用于处理逻辑和事件。
HTML结构
我们定义HTML结构,我们需要一个<textarea>
元素作为编辑区域,以及几个按钮来触发不同的格式化操作。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>富文本编辑器</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="editor"> <div class="toolbar"> <button onclick="format('bold')">Bold</button> <button onclick="format('italic')">Italic</button> <button onclick="format('underline')">Underline</button> <button onclick="format('color')">Color</button> <input type="color" id="colorPicker" onchange="setColor(this.value)"> <button onclick="format('background')">Background Color</button> <input type="color" id="bgColorPicker" onchange="setBackgroundColor(this.value)"> </div> <textarea id="textArea"></textarea> </div> <script src="script.js"></script> </body> </html>
CSS样式
我们添加一些基本的CSS样式来美化编辑器。
/* styles.css */ body { font-family: Arial, sans-serif; } .editor { border: 1px solid #ccc; padding: 10px; margin: 20px; } .toolbar { margin-bottom: 10px; } .toolbar button { margin-right: 5px; } #textArea { width: 100%; height: 200px; }
JavaScript逻辑
我们编写JavaScript代码来实现富文本编辑器的功能,这段代码将不到200行。
// script.js document.addEventListener('DOMContentLoaded', () => { const textArea = document.getElementById('textArea'); const formatButtons = document.querySelectorAll('.toolbar button'); const colorPicker = document.getElementById('colorPicker'); const bgColorPicker = document.getElementById('bgColorPicker'); function format(command) { document.execCommand(command, false, null); } function setColor(color) { document.execCommand('foreColor', false, color); } function setBackgroundColor(color) { document.execCommand('backColor', false, color); } formatButtons.forEach(button => { button.addEventListener('click', () => { format(button.getAttribute('onclick').split("(")[1].split("'")[1]); }); }); });
完整代码整合
下面是完整的HTML文件,包含所有部分:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>富文本编辑器</title> <style> body { font-family: Arial, sans-serif; } .editor { border: 1px solid #ccc; padding: 10px; margin: 20px; } .toolbar { margin-bottom: 10px; } .toolbar button { margin-right: 5px; } #textArea { width: 100%; height: 200px; } </style> </head> <body> <div class="editor"> <div class="toolbar"> <button onclick="format('bold')">Bold</button> <button onclick="format('italic')">Italic</button> <button onclick="format('underline')">Underline</button> <button onclick="format('color')">Color</button> <input type="color" id="colorPicker" onchange="setColor(this.value)"> <button onclick="format('background')">Background Color</button> <input type="color" id="bgColorPicker" onchange="setBackgroundColor(this.value)"> </div> <textarea id="textArea"></textarea> </div> <script> document.addEventListener('DOMContentLoaded', () => { const textArea = document.getElementById('textArea'); const formatButtons = document.querySelectorAll('.toolbar button'); const colorPicker = document.getElementById('colorPicker'); const bgColorPicker = document.getElementById('bgColorPicker'); function format(command) { document.execCommand(command, false, null); } function setColor(color) { document.execCommand('foreColor', false, color); } function setBackgroundColor(color) { document.execCommand('backColor', false, color); } formatButtons.forEach(button => { button.addEventListener('click', () => { format(button.getAttribute('onclick').split("(")[1].split("'")[1]); }); }); }); </script> </body> </html>
FAQs
Q1: 这个富文本编辑器支持撤销和重做操作吗?
A1: 是的,浏览器自带的document.execCommand
方法支持撤销(undo)和重做(redo)操作,可以通过调用document.execCommand('undo')
和document.execCommand('redo')
来实现这些功能,你可以在工具栏中添加相应的按钮,并为它们绑定事件处理器来调用这两个命令。
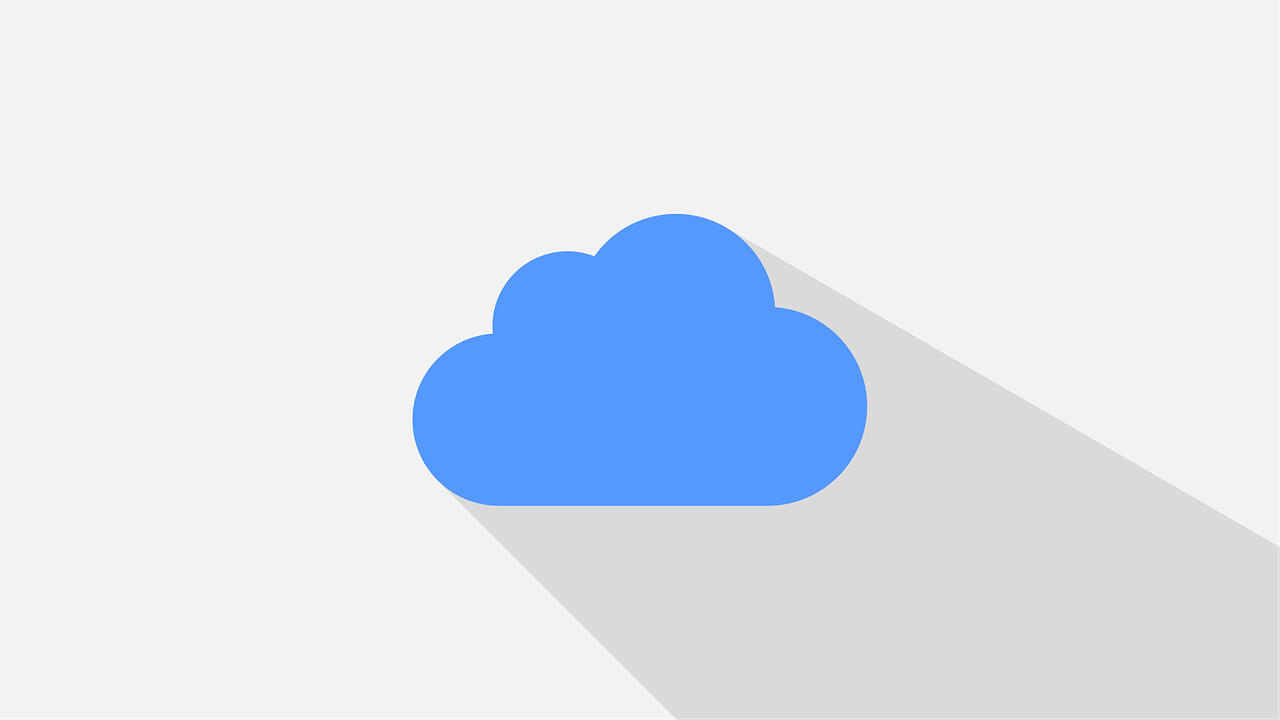
Q2: 如何扩展这个编辑器以支持更多的功能?
A2: 你可以通过添加更多的按钮和相应的事件处理器来扩展这个编辑器,添加一个“插入链接”按钮,并在点击时调用document.execCommand('createLink', false, prompt('URL:'))
,你还可以实现自定义的命令,通过修改文档的DOM结构或样式来实现更复杂的功能。
以上就是关于“不到200行 JavaScript 代码实现富文本编辑器的方法”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1366218.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复