System.out.println()
或System.out.print()
方法。,,“java,System.out.println("Hello, World!"); // 输出并换行,System.out.print("Hello, World!"); // 输出不换行,
“在Java编程中,输出语句扮演着至关重要的角色,它们不仅用于向用户展示程序的运行结果,还能帮助开发者调试代码,本文将深入探讨Java中的各类输出语句及其应用,包括标准输出、格式化输出以及文件输出等。
一、标准输出
1.System.out.print()
与System.out.println()
这两个方法用于将文本输出到控制台。System.out.print()
不会在输出后换行,而System.out.println()
则会在输出后添加一个换行符。
public class Main { public static void main(String[] args) { System.out.print("Hello, "); System.out.println("World!"); } }
上述代码将在控制台输出:
Hello, World!
2.System.out.printf()
System.out.printf()
提供了一种格式化输出的方式,类似于C语言中的printf
函数,它允许我们指定占位符来格式化字符串,并插入变量的值。
public class Main { public static void main(String[] args) { int num = 5; String str = "apples"; System.out.printf("I have %d %s. ", num, str); } }
上述代码将在控制台输出:
I have 5 apples.
3.System.out.format()
System.out.format()
与System.out.printf()
类似,但不会在末尾自动添加换行符,如果需要在末尾添加换行符,可以手动添加%n
作为格式说明符的一部分。
public class Main { public static void main(String[] args) { int year = 2023; System.out.format("Current year: %d%n", year); } }
上述代码将在控制台输出:
Current year: 2023
二、文件输出
1.FileWriter
类
FileWriter
类用于将数据写入文件,它提供了多种构造方法,可以根据需要创建新文件或追加到现有文件中。
import java.io.FileWriter; import java.io.IOException; public class Main { public static void main(String[] args) { try (FileWriter writer = new FileWriter("output.txt")) { writer.write("Hello, file!"); } catch (IOException e) { e.printStackTrace(); } } }
上述代码将在当前目录下创建一个名为output.txt
的文件,并写入“Hello, file!”。
2.BufferedWriter
类
BufferedWriter
类提供了缓冲功能,可以提高写入效率,它通常与FileWriter
一起使用。
import java.io.BufferedWriter; import java.io.FileWriter; import java.io.IOException; public class Main { public static void main(String[] args) { try (BufferedWriter writer = new BufferedWriter(new FileWriter("output.txt"))) { writer.write("Hello, buffered file!"); } catch (IOException e) { e.printStackTrace(); } } }
上述代码同样会在output.txt
文件中写入“Hello, buffered file!”,但由于使用了缓冲机制,写入速度更快。
三、表格输出
在Java中,虽然没有内置的表格输出功能,但我们可以通过循环和格式化字符串来实现简单的表格输出。
public class Main { public static void main(String[] args) { String[] headers = {"ID", "Name", "Age"}; String[][] data = { {"1", "Alice", "23"}, {"2", "Bob", "30"}, {"3", "Charlie", "25"} }; for (String header : headers) { System.out.printf("%-8s", header); } System.out.println(); for (String[] row : data) { for (String cell : row) { System.out.printf("%-8s", cell); } System.out.println(); } } }
上述代码将在控制台输出一个简单的表格:
ID Name Age 1 Alice 23 2 Bob 30 3 Charlie 25
这里使用了%-8s
作为格式说明符,确保每个单元格占用8个字符的宽度,并对齐到左侧。
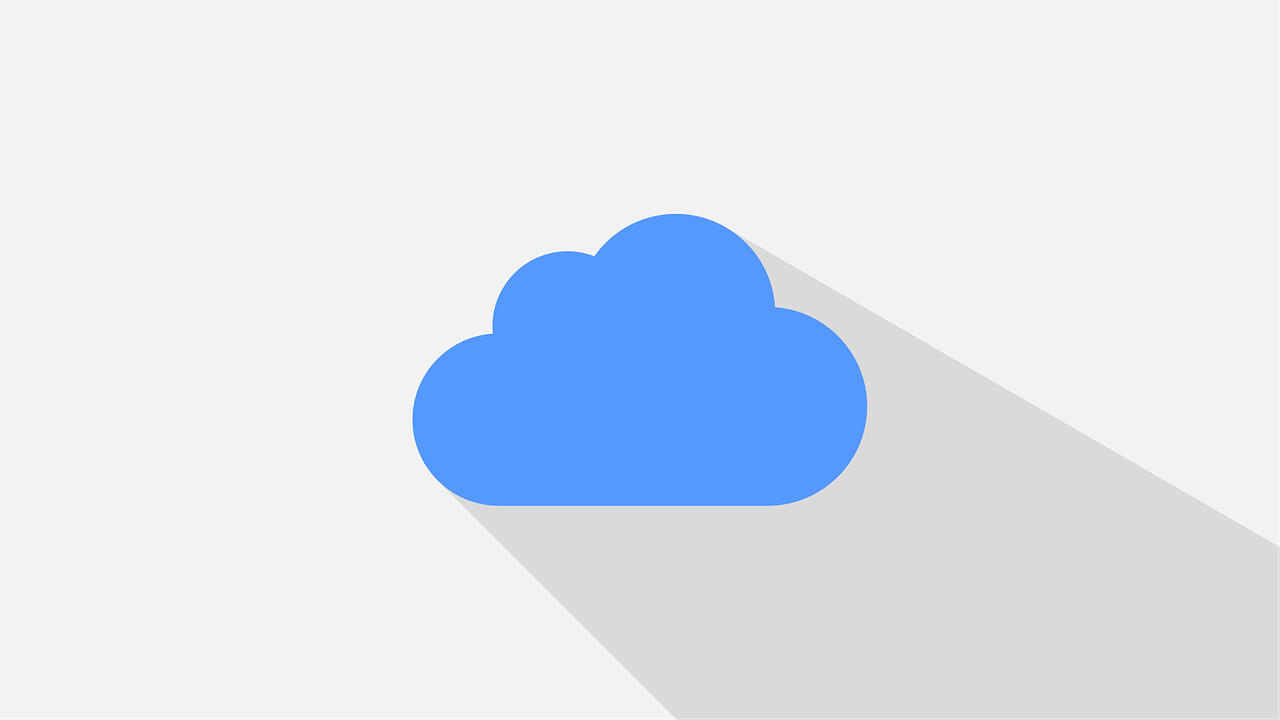
四、FAQs
Q1: 如何在Java中更改输出语句的颜色?
A1: Java本身不支持直接更改控制台输出颜色的功能,可以使用第三方库如Jansi或JLine来实现这一功能,使用Jansi库可以这样实现:
import org.fusesource.jansi.Ansi; import org.fusesource.jansi.AnsiConsole; import org.fusesource.jansi.AnsiOutputStream; public class Main { public static void main(String[] args) { Ansi ansi = Ansi.get(); AnsiConsole.wrapSystemOut(); // Wrap standard output with ANSI capabilities System.out.println(ansi.fg(Ansi.Color.RED).a("This is red text").reset()); System.out.println(ansi.fg(Ansi.Color.GREEN).a("This is green text").reset()); } }
上述代码将在控制台输出红色和绿色的文本,需要注意的是,这需要终端支持ANSI转义序列。
Q2: 如何在Java中捕获并处理输出语句的异常?
A2: 在Java中,大多数输出操作都可能抛出IOException
,为了捕获和处理这些异常,可以使用try-catch语句块。
import java.io.BufferedWriter; import java.io.FileWriter; import java.io.IOException; public class Main { public static void main(String[] args) { try (BufferedWriter writer = new BufferedWriter(new FileWriter("output.txt"))) { writer.write("Trying to write this line..."); } catch (IOException e) { System.err.println("An error occurred while writing to the file: " + e.getMessage()); } } }
上述代码尝试将一行文本写入文件,并在发生异常时捕获并处理该异常,通过这种方式,可以确保程序的健壮性和稳定性。
小伙伴们,上文介绍了“java输出语句”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1366183.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复