在当今的软件开发领域,构建高效、可扩展且易于维护的应用程序至关重要,ASP.NET 是一个强大的框架,它提供了丰富的工具和库,帮助开发者快速构建 Web 应用和 API,本文将探讨如何使用 ASP.NET 结合 Entity Framework (EF) 和 MySQL 数据库来创建一个高效的 API。
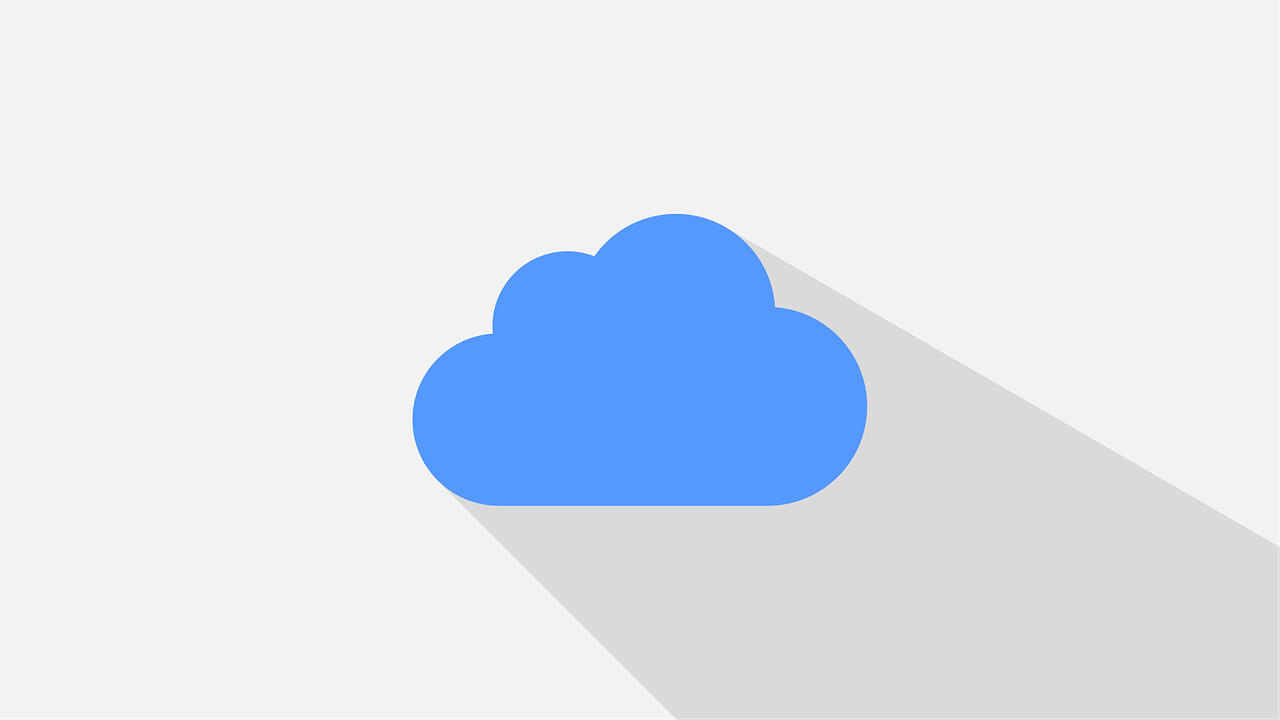
1. 引言
随着互联网的快速发展,Web 应用程序的需求也在不断增长,为了应对这种需求,开发者需要选择适合的工具和技术栈,ASP.NET 是一个流行的框架,它提供了许多功能,如路由、中间件支持和内置的身份验证机制,Entity Framework (EF) 是一个对象关系映射 (ORM) 框架,它允许开发者以面向对象的方式操作数据库,而 MySQL 是一个广泛使用的关系型数据库管理系统,它具有高性能、可靠性和易用性。
2. 环境准备
在开始之前,我们需要确保开发环境中已经安装了以下软件:
Visual Studio(或其他支持 .NET 开发的 IDE)
.NET SDK
MySQL 数据库
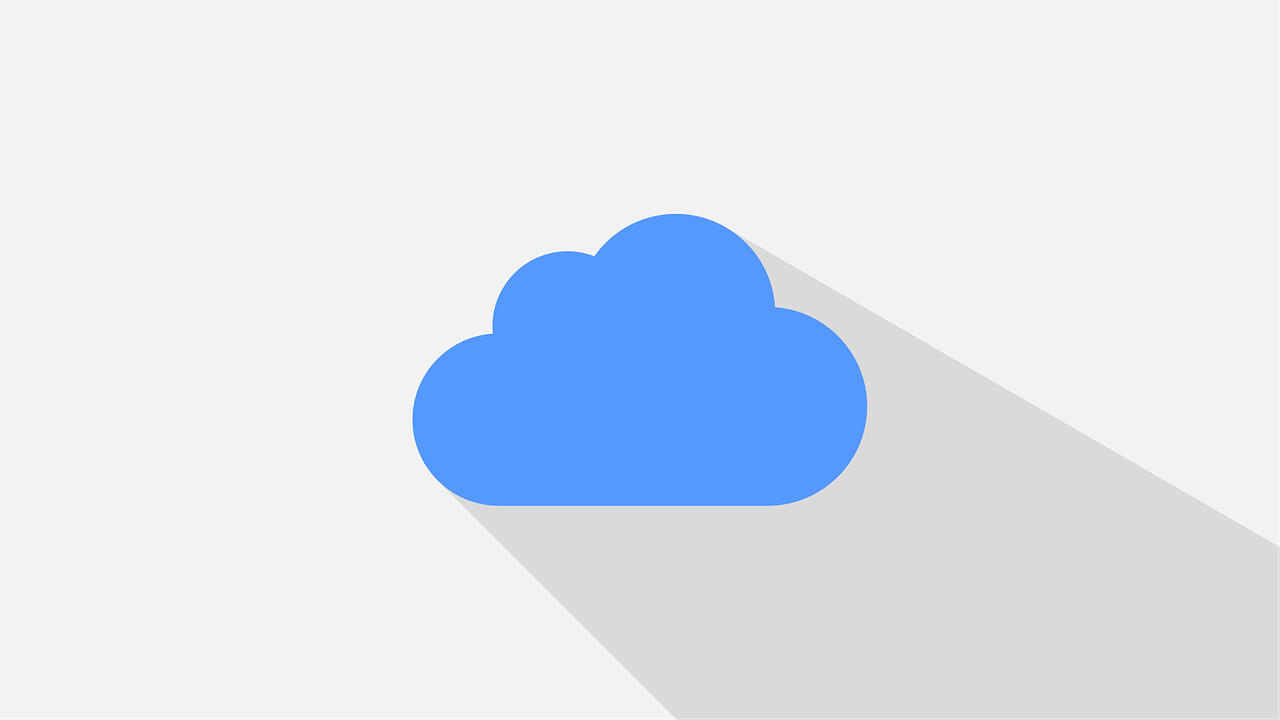
MySQL Workbench(或其他 MySQL 管理工具)
3. 创建 ASP.NET API 项目
我们使用 Visual Studio 创建一个新的 ASP.NET Core Web API 项目,打开 Visual Studio,选择“创建新项目”,然后搜索“ASP.NET Core Web API”,选择一个模板并点击“下一步”,在项目名称中输入一个合适的名称,MyApi”,然后点击“创建”。
4. 配置数据库连接
在创建项目后,我们需要配置数据库连接,在appsettings.json
文件中添加以下配置:
{ "ConnectionStrings": { "DefaultConnection": "Server=localhost;Database=mydatabase;User=root;Password=yourpassword;" } }
请根据实际情况替换服务器地址、数据库名称、用户名和密码。
5. 安装必要的 NuGet 包
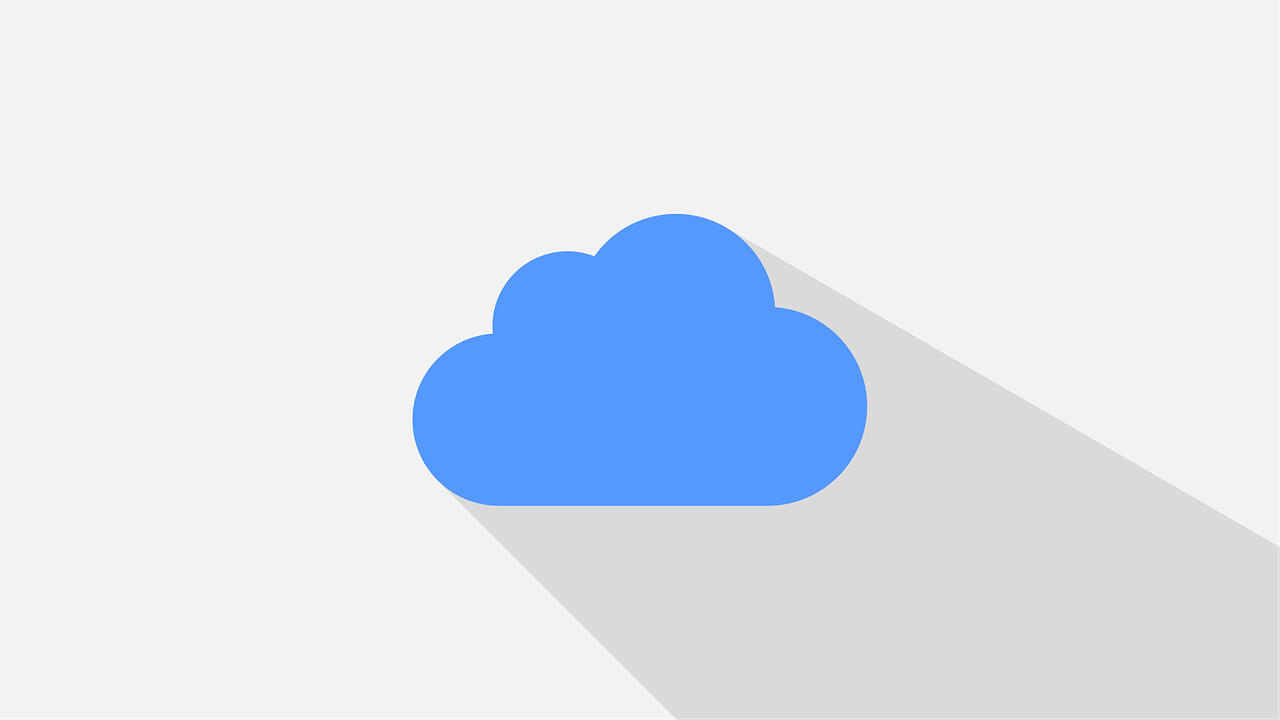
我们需要安装 Entity Framework Core 和 MySql.Data 包,在解决方案资源管理器中右键点击项目名称,选择“管理 NuGet 包”,然后搜索并安装以下包:
Microsoft.EntityFrameworkCore
MySql.Data.EntityFrameworkCore
6. 创建数据模型
现在我们可以开始定义数据模型了,在项目中添加一个新的类文件,命名为Models/Product.cs
,并添加以下代码:
using System; using System.Collections.Generic; namespace MyApi.Models { public class Product { public int Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } } }
7. 创建数据库上下文
我们需要创建一个数据库上下文来管理数据库操作,在项目中添加一个新的类文件,命名为Data/AppDbContext.cs
,并添加以下代码:
using Microsoft.EntityFrameworkCore; using MyApi.Models; namespace MyApi.Data { public class AppDbContext : DbContext { public AppDbContext(DbContextOptions<AppDbContext> options) : base(options) { } public DbSet<Product> Products { get; set; } } }
8. 配置依赖注入和服务
在Startup.cs
文件中配置依赖注入和服务,打开Startup.cs
文件,修改ConfigureServices
方法如下:
public void ConfigureServices(IServiceCollection services) { services.AddControllers(); services.AddDbContext<AppDbContext>(options => options.UseMySQL(Configuration.GetConnectionString("DefaultConnection"))); }
9. 创建控制器
我们需要创建一个控制器来处理 API 请求,在项目中添加一个新的控制器文件,命名为Controllers/ProductsController.cs
,并添加以下代码:
using Microsoft.AspNetCore.Mvc; using Microsoft.EntityFrameworkCore; using MyApi.Data; using MyApi.Models; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; namespace MyApi.Controllers { [Route("api/[controller]")] [ApiController] public class ProductsController : ControllerBase { private readonly AppDbContext _context; public ProductsController(AppDbContext context) { _context = context; } // GET: api/Products [HttpGet] public async Task<ActionResult<IEnumerable<Product>>> GetProducts() { return await _context.Products.ToListAsync(); } // GET: api/Products/5 [HttpGet("{id}")] public async Task<ActionResult<Product>> GetProduct(int id) { var product = await _context.Products.FindAsync(id); if (product == null) { return NotFound(); } return product; } // PUT: api/Products/5 [HttpPut("{id}")] public async Task<IActionResult> PutProduct(int id, Product product) { if (id != product.Id) { return BadRequest(); } _context.Entry(product).State = EntityState.Modified; try { await _context.SaveChangesAsync(); } catch (DbUpdateConcurrencyException) { if (!ProductExists(id)) { return NotFound(); } else { throw; } } return NoContent(); } // POST: api/Products [HttpPost] public async Task<ActionResult<Product>> PostProduct(Product product) { _context.Products.Add(product); await _context.SaveChangesAsync(); return CreatedAtAction(nameof(GetProduct), new { id = product.Id }, product); } // DELETE: api/Products/5 [HttpDelete("{id}")] public async Task<IActionResult> DeleteProduct(int id) { var product = await _context.Products.FindAsync(id); if (product == null) { return NotFound(); } _context.Products.Remove(product); await _context.SaveChangesAsync(); return NoContent(); } private bool ProductExists(int id) { return _context.Products.Any(e => e.Id == id); } } }
10. 运行应用程序
我们已经完成了 API 的基本设置,可以通过运行 Visual Studio 来启动应用程序,并测试 API 是否工作正常,默认情况下,应用程序将在 http://localhost:5000/ 上运行,你可以使用 Postman 或其他 API 客户端工具来测试 API 的各个端点。
FAQs
Q1: 如何更改数据库连接字符串?
A1: 你可以通过修改appsettings.json
文件中的ConnectionStrings
部分来更改数据库连接字符串,确保新的连接字符串包含正确的服务器地址、数据库名称、用户名和密码。
Q2: 如果我想添加更多的实体模型,应该怎么做?
A2: 你可以通过在Models
文件夹中添加新的类文件来定义更多的实体模型,然后在AppDbContext
中添加相应的DbSet
属性,并在OnModelCreating
方法中配置实体之间的关系(如果有),根据需要更新控制器以处理新的实体。
以上就是关于“asp.net api ef mysql”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1363671.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复