Button控件检索数据库
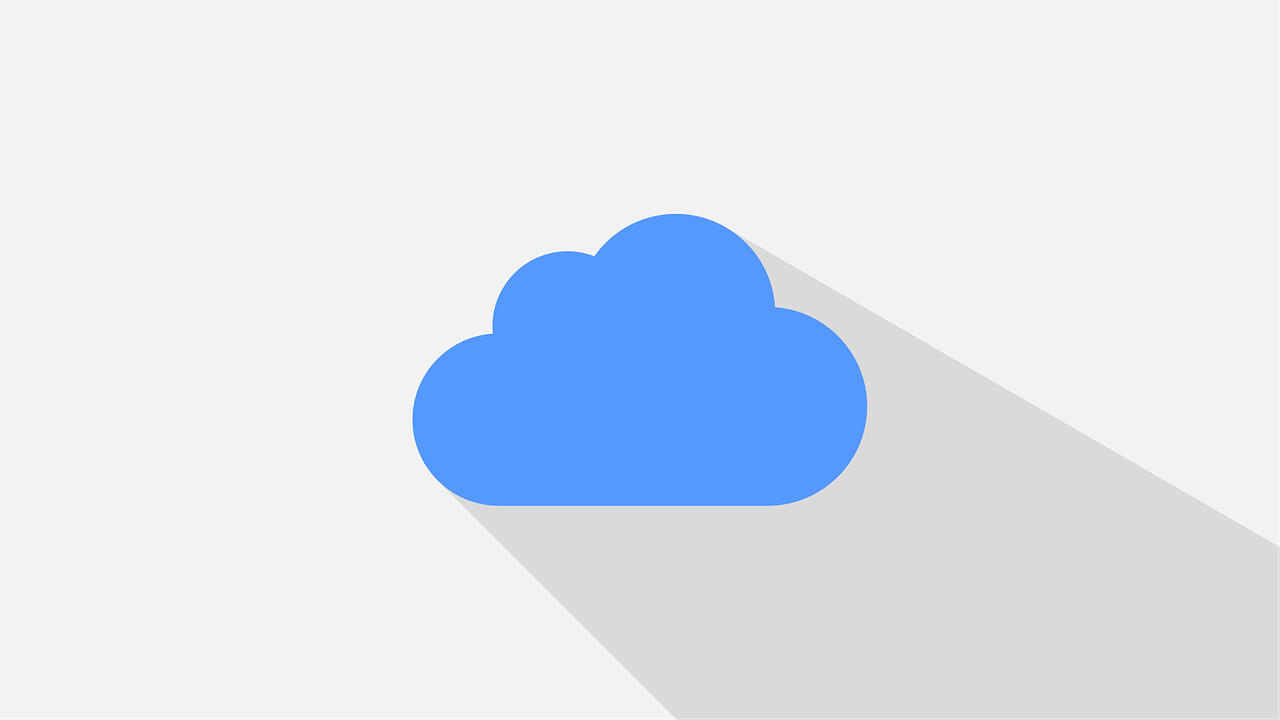
背景介绍
在现代应用程序开发中,用户界面(UI)与数据存储的交互是至关重要的,Button控件作为用户界面的核心组件之一,经常用于触发各种操作,包括从数据库中检索数据,本文将详细介绍如何使用Button控件实现数据库检索功能,包括技术选型、具体步骤和示例代码。
Button控件简介
Button控件是一种常见的用户界面元素,用于触发特定事件或动作,它在不同编程语言和框架中有不同实现方式,但其基本功能一致,在Web开发中,Button控件通常使用HTML的<button>
标签或JavaScript库(如jQuery、React)进行封装;而在桌面应用开发中,Button控件则由GUI框架(如Windows Forms、WPF、Java Swing等)提供。
数据库连接与检索
为了实现Button控件的数据库检索功能,首先需要建立与数据库的连接,并执行SQL查询语句,以下以MySQL数据库为例,介绍如何通过C#语言实现这一过程。
安装必要的库
在使用C#连接MySQL数据库之前,需要安装MySql.Data包,可以通过NuGet进行安装:
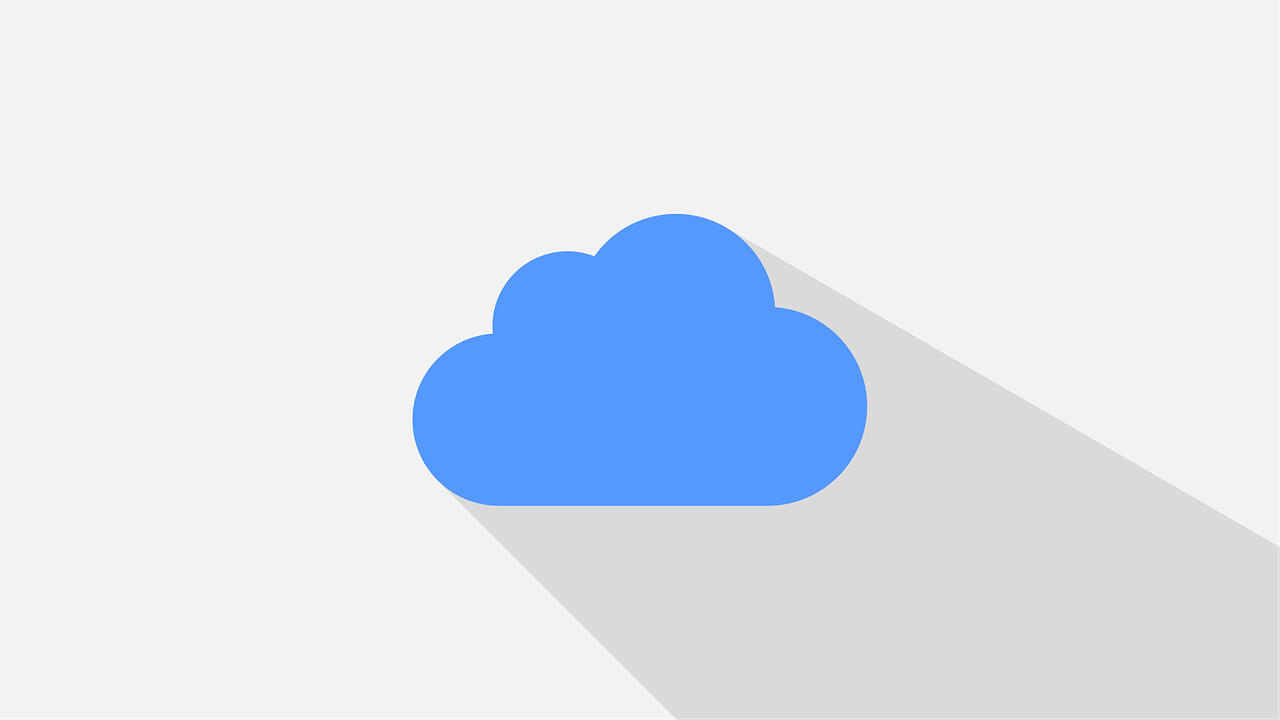
Install-Package MySql.Data
创建数据库连接
在C#中创建一个数据库连接,可以使用MySqlConnection
类,以下是一个简单的示例:
using System; using MySql.Data.MySqlClient; class Program { static void Main() { string connectionString = "Server=localhost;Database=testdb;User ID=root;Password=password;"; using (MySqlConnection conn = new MySqlConnection(connectionString)) { try { conn.Open(); Console.WriteLine("数据库连接成功!"); } catch (Exception ex) { Console.WriteLine("数据库连接失败:" + ex.Message); } } } }
执行SQL查询
一旦建立了数据库连接,就可以执行SQL查询语句来检索数据,以下是一个完整的示例,展示如何在Button点击事件中检索数据库中的数据:
using System; using System.Windows.Forms; using MySql.Data.MySqlClient; namespace WindowsFormsApp { public partial class Form1 : Form { private MySqlConnection _connection; public Form1() { InitializeComponent(); string connectionString = "Server=localhost;Database=testdb;User ID=root;Password=password;"; _connection = new MySqlConnection(connectionString); } private void button1_Click(object sender, EventArgs e) { try { // 打开数据库连接 _connection.Open(); // 定义SQL查询语句 string query = "SELECT * FROM Users"; MySqlCommand cmd = new MySqlCommand(query, _connection); // 执行查询并读取数据 MySqlDataReader reader = cmd.ExecuteReader(); while (reader.Read()) { Console.WriteLine($"{reader["Id"]}, {reader["Name"]}, {reader["Age"]}"); } // 关闭读取器和连接 reader.Close(); _connection.Close(); } catch (Exception ex) { MessageBox.Show("发生错误:" + ex.Message, "错误", MessageBoxButtons.OK, MessageBoxIcon.Error); } } } }
完整示例:Windows Forms应用程序
下面是一个完整的Windows Forms应用程序示例,展示如何使用Button控件从数据库中检索数据并在界面上显示。
using System; using System.Windows.Forms; using MySql.Data.MySqlClient; namespace WindowsFormsApp { public partial class Form1 : Form { private MySqlConnection _connection; private ListBox listBox; private TextBox textBox; private Button button; public Form1() { InitializeComponent(); string connectionString = "Server=localhost;Database=testdb;User ID=root;Password=password;"; _connection = new MySqlConnection(connectionString); InitializeControls(); } private void InitializeComponent() { this.listBox = new ListBox(); this.textBox = new TextBox(); this.button = new Button(); this.SuspendLayout(); // // listBox // this.listBox.FormattingEnabled = true; this.listBox.ItemHeight = 16; this.listBox.Location = new System.Drawing.Point(13, 50); this.listBox.Name = "listBox"; this.listBox.Size = new System.Drawing.Size(260, 144); this.listBox.TabIndex = 0; // // textBox // this.textBox.Location = new System.Drawing.Point(13, 13); this.textBox.Name = "textBox"; this.textBox.Size = new System.Drawing.Size(260, 22); this.textBox.TabIndex = 1; // // button // this.button.Location = new System.Drawing.Point(13, 31); this.button.Name = "button"; this.button.Size = new System.Drawing.Size(75, 23); this.button.TabIndex = 2; this.button.Text = "检索数据"; this.button.UseVisualStyleBackColor = true; this.button.Click += new System.EventHandler(this.button1_Click); // // Form1 // this.ClientSize = new System.Drawing.Size(284, 211); this.Controls.Add(this.button); this.Controls.Add(this.textBox); this.Controls.Add(this.listBox); this.Name = "Form1"; this.ResumeLayout(false); } private void button1_Click(object sender, EventArgs e) { try { // 打开数据库连接 _connection.Open(); // 定义SQL查询语句 string query = $"SELECT * FROM Users WHERE Age > @age"; MySqlCommand cmd = new MySqlCommand(query, _connection); cmd.Parameters.AddWithValue("@age", int.Parse(textBox.Text)); // 执行查询并读取数据 MySqlDataReader reader = cmd.ExecuteReader(); while (reader.Read()) { listBox.Items.Add($"{reader["Id"]}, {reader["Name"]}, {reader["Age"]}"); } // 关闭读取器和连接 reader.Close(); _connection.Close(); } catch (Exception ex) { MessageBox.Show("发生错误:" + ex.Message, "错误", MessageBoxButtons.OK, MessageBoxIcon.Error); } } } }
归纳按钮控件检索数据库的过程包括以下几个步骤:建立数据库连接、编写SQL查询语句、执行查询并处理结果,通过以上示例,可以看到Button控件与数据库交互的基本流程,实际应用中,还可以根据需求进行更多优化和完善,例如添加输入验证、异常处理、数据分页等功能。
以上就是关于“button控件检索数据库”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1361357.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复