python,import mysql.connector,,# 创建连接,conn = mysql.connector.connect(, host="localhost",, user="yourusername",, password="yourpassword",, database="yourdatabase",),,# 检查连接是否成功,if conn.is_connected():, print("Successfully connected to the database"),else:, print("Failed to connect to the database"),
“在现代软件开发中,数据库连接是至关重要的一环,MySQL 作为一种流行的关系型数据库管理系统,广泛应用于各种项目和应用程序中,本文将详细介绍如何通过代码链接到 MySQL 数据库,并展示一些常见的使用场景和技巧。
一、MySQL 链接数据库的基本步骤
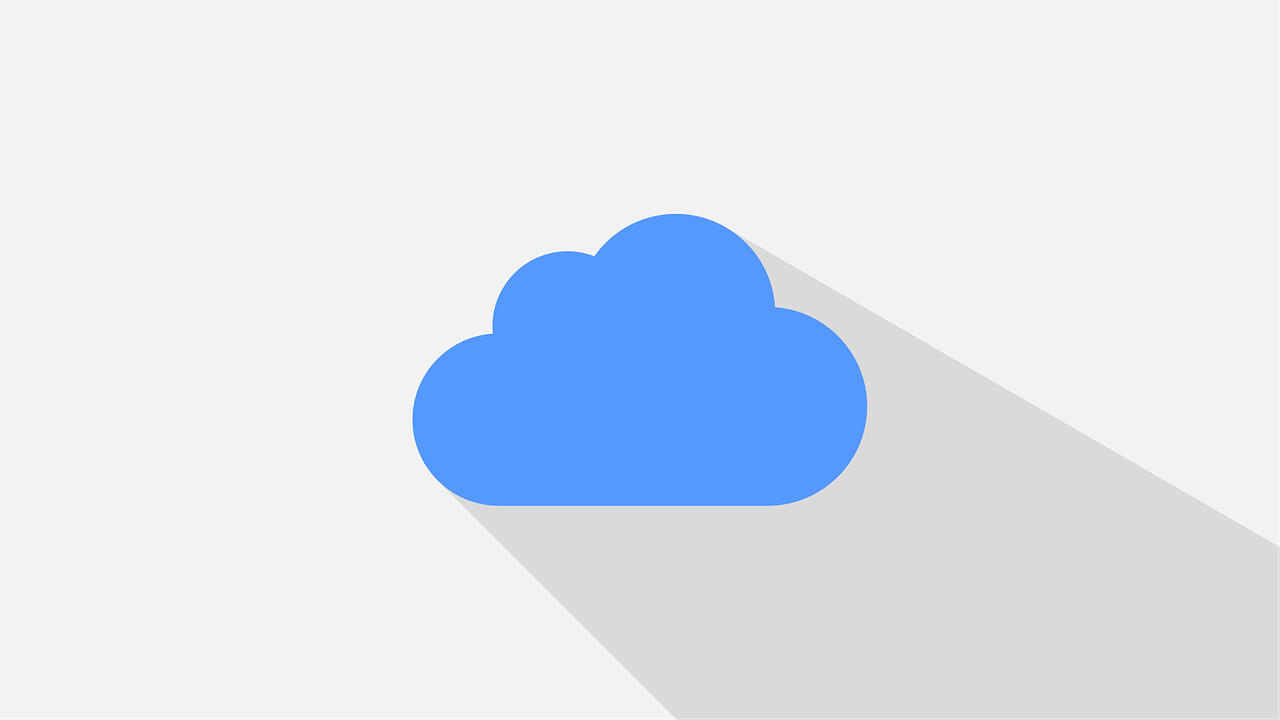
1、安装 MySQL 驱动:首先需要确保你的开发环境中安装了 MySQL 驱动程序,如果你使用的是 Java,可以通过 Maven 添加 MySQL Connector/J 依赖。
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.26</version> </dependency>
2、导入相关包:在你的代码中导入必要的包和类。
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement;
3、注册 JDBC 驱动程序:尽管现代的 JDBC 驱动程序会自动注册,但手动注册可以确保兼容性。
try { Class.forName("com.mysql.cj.jdbc.Driver"); } catch (ClassNotFoundException e) { e.printStackTrace(); }
4、创建数据库连接:使用DriverManager.getConnection
方法创建数据库连接,你需要提供数据库的 URL、用户名和密码。
String url = "jdbc:mysql://localhost:3306/yourDatabase"; String user = "yourUsername"; String password = "yourPassword"; Connection connection = null; try { connection = DriverManager.getConnection(url, user, password); System.out.println("Database connected!"); } catch (SQLException e) { e.printStackTrace(); }
5、执行 SQL 语句:通过连接对象创建Statement
或PreparedStatement
对象,并执行 SQL 查询或更新操作。
try { Statement statement = connection.createStatement(); String sql = "SELECT * FROM yourTable"; ResultSet resultSet = statement.executeQuery(sql); while (resultSet.next()) { System.out.println("Column1: " + resultSet.getString("column1")); System.out.println("Column2: " + resultSet.getInt("column2")); } } catch (SQLException e) { e.printStackTrace(); } finally { try { if (connection != null) { connection.close(); } } catch (SQLException e) { e.printStackTrace(); } }
二、常见使用场景及示例代码
1、插入数据:向数据库表中插入一条记录。
try { String insertSql = "INSERT INTO yourTable (column1, column2) VALUES ('value1', 'value2')"; int rowsInserted = statement.executeUpdate(insertSql); if (rowsInserted > 0) { System.out.println("A new row was inserted successfully!"); } } catch (SQLException e) { e.printStackTrace(); } finally { try { if (statement != null) { statement.close(); } } catch (SQLException e) { e.printStackTrace(); } }
2、更新数据:更新数据库表中的一条或多条记录。
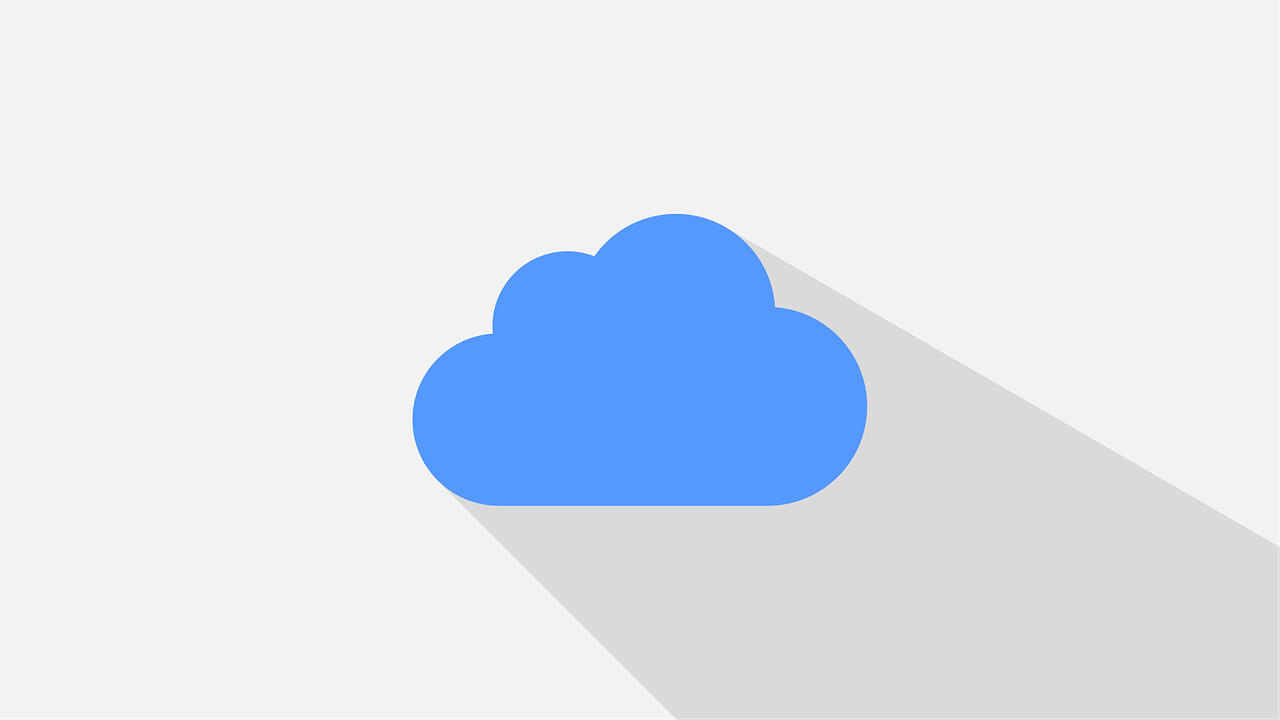
try { String updateSql = "UPDATE yourTable SET column1 = 'newValue' WHERE column2 = 'someValue'"; int rowsUpdated = statement.executeUpdate(updateSql); if (rowsUpdated > 0) { System.out.println("Rows were updated successfully!"); } } catch (SQLException e) { e.printStackTrace(); } finally { try { if (statement != null) { statement.close(); } } catch (SQLException e) { e.printStackTrace(); } }
3、删除数据:从数据库表中删除一条或多条记录。
try { String deleteSql = "DELETE FROM yourTable WHERE column1 = 'someValue'"; int rowsDeleted = statement.executeUpdate(deleteSql); if (rowsDeleted > 0) { System.out.println("Rows were deleted successfully!"); } } catch (SQLException e) { e.printStackTrace(); } finally { try { if (statement != null) { statement.close(); } } catch (SQLException e) { e.printStackTrace(); } }
4、事务处理:确保一组 SQL 操作要么全部成功,要么全部失败。
try { connection.setAutoCommit(false); // Start transaction // Perform some operations... connection.commit(); // Commit transaction } catch (SQLException e) { try { connection.rollback(); // Rollback transaction on error } catch (SQLException ex) { ex.printStackTrace(); } } finally { try { if (connection != null) { connection.close(); } } catch (SQLException e) { e.printStackTrace(); } }
三、常见问题解答(FAQs)
问题1:如何更改数据库连接的超时时间?
答案:可以通过在数据库 URL 中添加参数来设置连接超时时间,将超时时间设置为 30 秒:
String url = "jdbc:mysql://localhost:3306/yourDatabase?connectTimeout=30000";
也可以在DriverManager
获取连接时传递一个Properties
对象来设置超时时间和其他连接属性:
Properties properties = new Properties(); properties.put("user", "yourUsername"); properties.put("password", "yourPassword"); properties.put("connectTimeout", "30000"); Connection connection = DriverManager.getConnection(url, properties);
问题2:如何处理 SQL 注入攻击?
答案:为了防止 SQL 注入攻击,应始终使用PreparedStatement
而不是Statement
。PreparedStatement
允许你使用占位符(如?
)来表示参数,从而避免直接拼接 SQL 字符串。
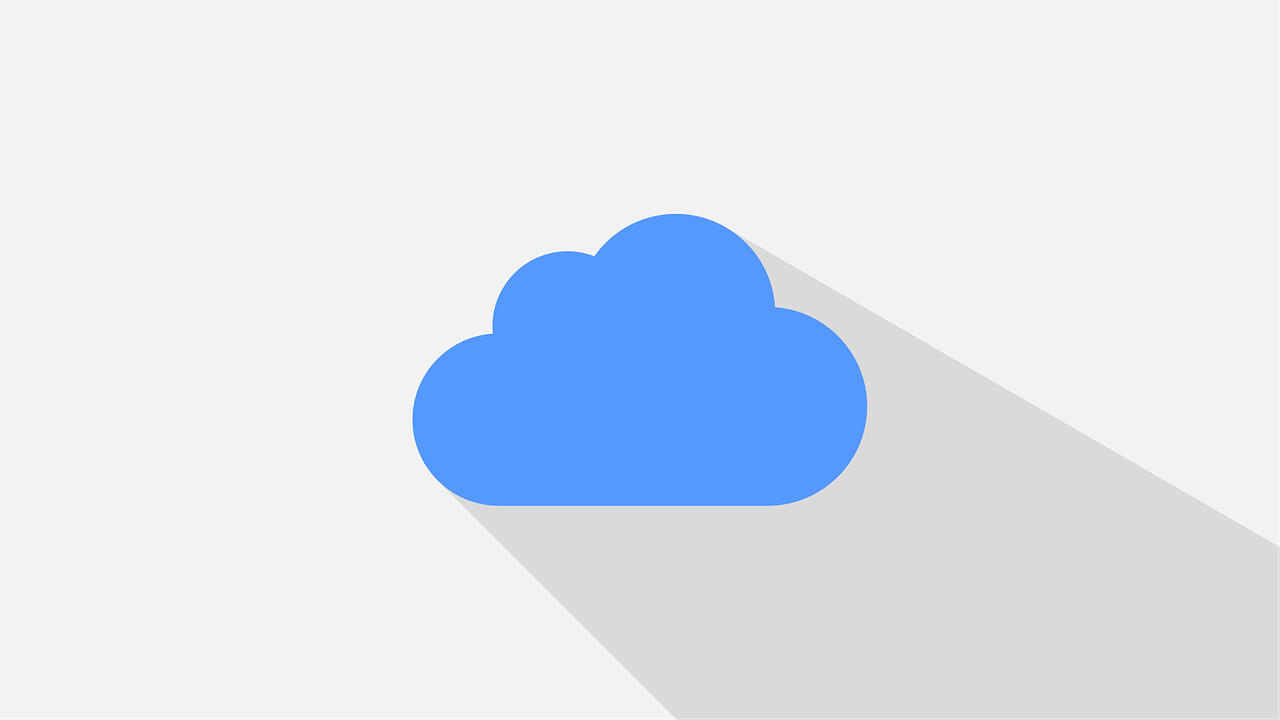
String insertSql = "INSERT INTO yourTable (column1, column2) VALUES (?, ?)"; PreparedStatement preparedStatement = connection.prepareStatement(insertSql); preparedStatement.setString(1, "value1"); preparedStatement.setString(2, "value2"); int rowsInserted = preparedStatement.executeUpdate();
各位小伙伴们,我刚刚为大家分享了有关“mysql链接数据库的代码_链接”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1357307.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复