在ASP(Active Server Pages)中,调用只带图片的内容可以通过多种方式实现,本文将详细介绍如何在ASP页面中嵌入和显示图像,并提供一些常见问题的解答。
使用HTML标签直接嵌入图片
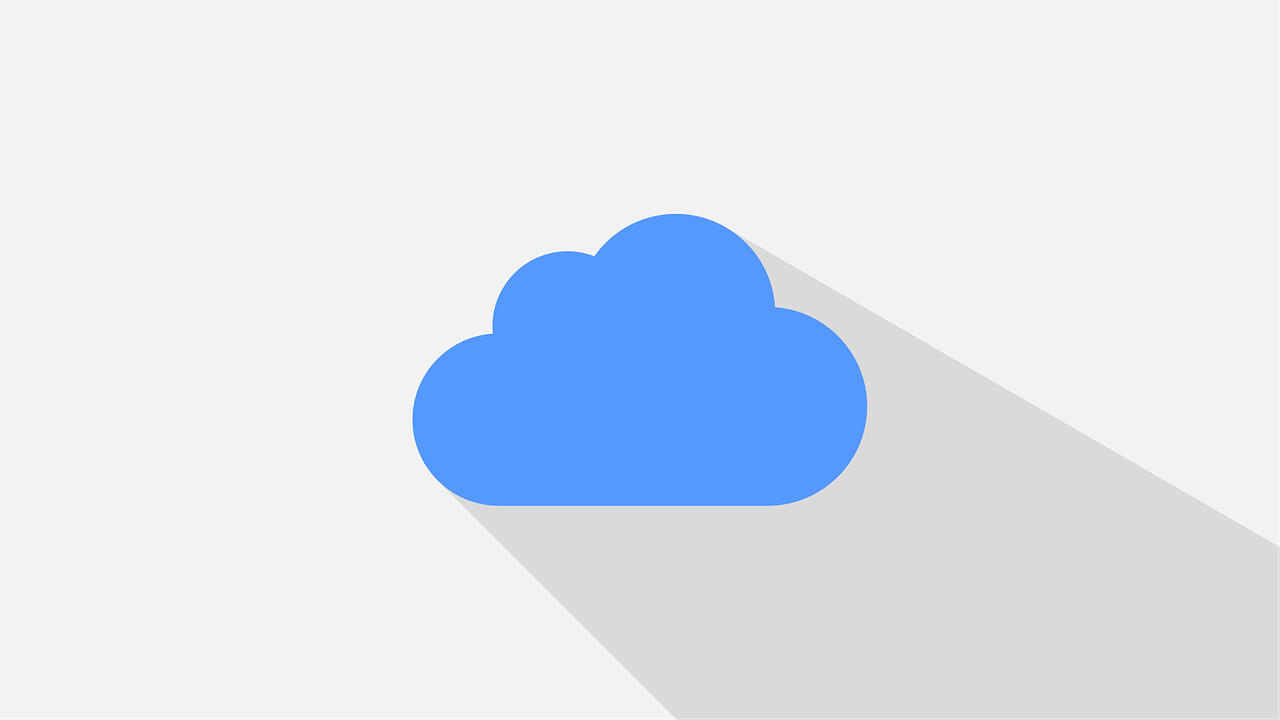
这是最简单也是最常见的方法之一,通过HTML的<img>
标签直接在ASP页面中嵌入图片,以下是示例代码:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>ASP Image Display</title> </head> <body> <h1>Displaying Images in ASP</h1> <img src="path/to/your/image.jpg" alt="Description of the image"> </body> </html>
在这个例子中,src
属性指向图片的路径,可以是相对路径或绝对路径。alt
属性提供了图片的描述,当图片无法加载时会显示这个描述文本。
动态生成图片路径
图片的路径可能是动态生成的,例如根据用户输入或其他条件来决定显示哪张图片,在这种情况下,可以使用ASP脚本来生成图片路径。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Dynamic Image Path</title> </head> <body> <h1>Dynamic Image Display</h1> <% Dim imagePath imagePath = "path/to/your/dynamic/image.jpg" ' 这里可以根据实际情况修改路径 %> <img src="<%= imagePath %>" alt="Description of the dynamic image"> </body> </html>
在这个例子中,imagePath
变量存储了动态生成的图片路径,然后通过ASP的输出语法将其插入到src
属性中。
从数据库读取图片路径
如果图片路径存储在数据库中,可以通过ASP从数据库中读取路径并显示图片,假设我们有一个表Images
,其中包含列ImagePath
。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Database Image Display</title> </head> <body> <h1>Displaying Images from Database</h1> <% ' 建立数据库连接 Set conn = Server.CreateObject("ADODB.Connection") conn.Open "Provider=SQLOLEDB;Data Source=YOUR_SERVER;Initial Catalog=YOUR_DATABASE;User ID=YOUR_USERNAME;Password=YOUR_PASSWORD" ' 执行查询 Dim rs, sql sql = "SELECT ImagePath FROM Images WHERE ID = 1" ' 这里假设ID为1的记录是我们想要的图片 Set rs = conn.Execute(sql) ' 获取结果并显示图片 If Not rs.EOF Then Dim imagePath imagePath = rs("ImagePath") Response.Write "<img src="" & imagePath & "" alt=""Image from database""" End If ' 关闭记录集和连接 rs.Close Set rs = Nothing conn.Close Set conn = Nothing %> </body> </html>
在这个例子中,首先建立与数据库的连接,然后执行查询获取图片路径,最后将路径插入到<img>
标签中。
使用ASP函数封装图片显示逻辑
为了提高代码的可重用性和清晰度,可以将图片显示逻辑封装在一个ASP函数中。
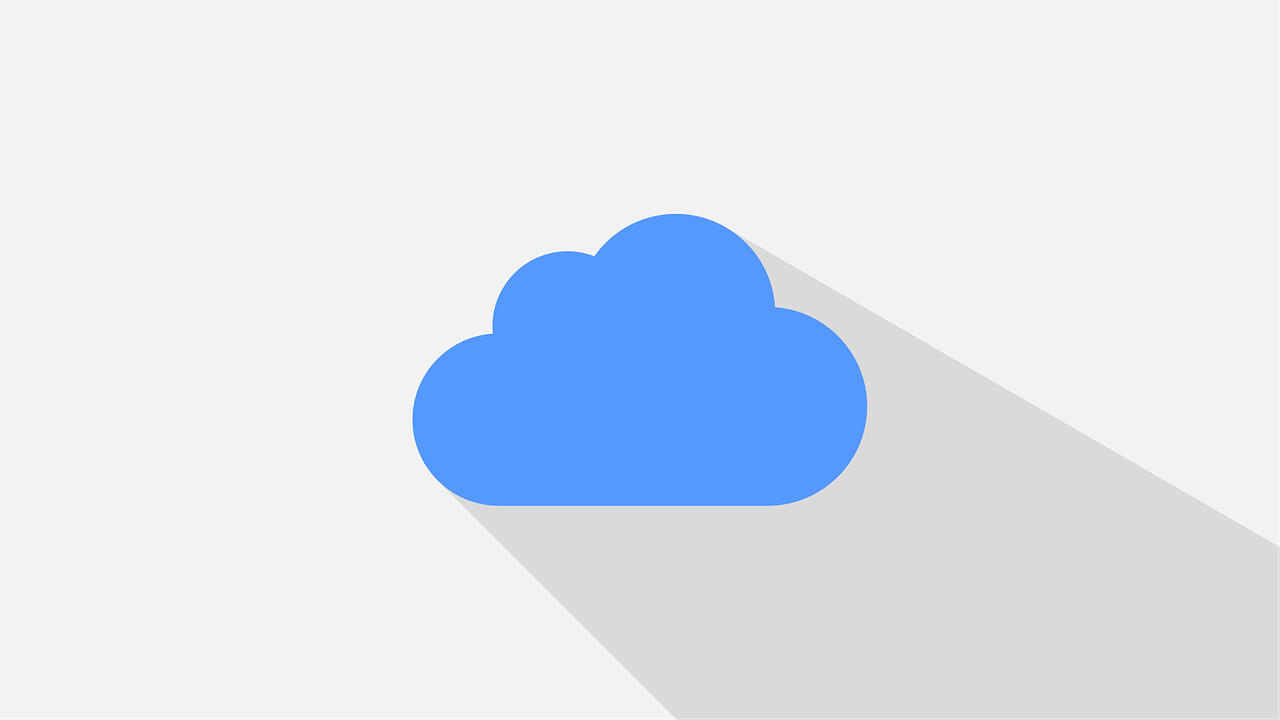
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Display Image with Function</title> </head> <body> <h1>Using Function to Display Image</h1> <% Function DisplayImage(imagePath) DisplayImage = "<img src="" & imagePath & "" alt=""Description of the image""" End Function Dim imagePath imagePath = "path/to/your/image.jpg" ' 这里可以根据实际情况修改路径 Response.Write DisplayImage(imagePath) %> </body> </html>
在这个例子中,定义了一个名为DisplayImage
的函数,该函数接受一个参数imagePath
并返回一个包含<img>
标签的字符串,然后在主页面中调用这个函数并输出结果。
表格形式展示多张图片
如果需要展示多张图片,可以使用表格来布局,以下是一个例子,展示如何在一个表格中显示多张图片。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Table of Images</title> <style> table { width: 100%; border-collapse: collapse; } td { border: 1px solid #dddddd; text-align: center; padding: 10px; } </style> </head> <body> <h1>Displaying Multiple Images in a Table</h1> <% Dim images, i images = Array("path/to/your/image1.jpg", "path/to/your/image2.jpg", "path/to/your/image3.jpg") ' 这里可以根据实际情况修改路径数组 %> <table> <tr> <% For i = LBound(images) To UBound(images) Step 1 %> <td><%= DisplayImage(images(i)) %></td> <% Next %> </tr> </table> </body> </html>
在这个例子中,使用了一个数组来存储多个图片路径,并通过循环将这些图片显示在表格的单元格中。DisplayImage
函数用于生成每个单元格中的<img>
相关问答FAQs
Q1: 如何在ASP页面中动态更改图片?
A1: 在ASP页面中动态更改图片可以通过修改图片路径或使用不同的逻辑来实现,以下是一个示例,展示了如何根据用户选择动态更改图片。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Dynamic Image Change</title> </head> <body> <h1>Change Image Dynamically</h1> <form method="post" action=""> <label for="imageSelect">Choose an image:</label> <select name="imageSelect" id="imageSelect" onchange="this.form.submit()"> <option value="path/to/your/image1.jpg">Image 1</option> <option value="path/to/your/image2.jpg">Image 2</option> <option value="path/to/your/image3.jpg">Image 3</option> </select> </form> <br> <% Dim selectedImagePath If Request.Form("imageSelect") <> "" Then selectedImagePath = Request.Form("imageSelect") Else selectedImagePath = "path/to/default/image.jpg" ' 默认图片路径 End If Response.Write "<img src="" & selectedImagePath & "" alt=""Selected image""" %> </body> </html>
在这个例子中,使用了一个表单和一个下拉菜单让用户选择图片,当用户改变选择时,表单会自动提交,并根据用户的选择显示相应的图片,如果没有选择,则显示默认图片。
Q2: 如何在ASP页面中处理图片上传?
A2: 在ASP页面中处理图片上传通常涉及以下几个步骤:接收上传的文件、保存文件到服务器、以及在页面上显示或处理该文件,以下是一个简化的例子,展示了如何处理图片上传。
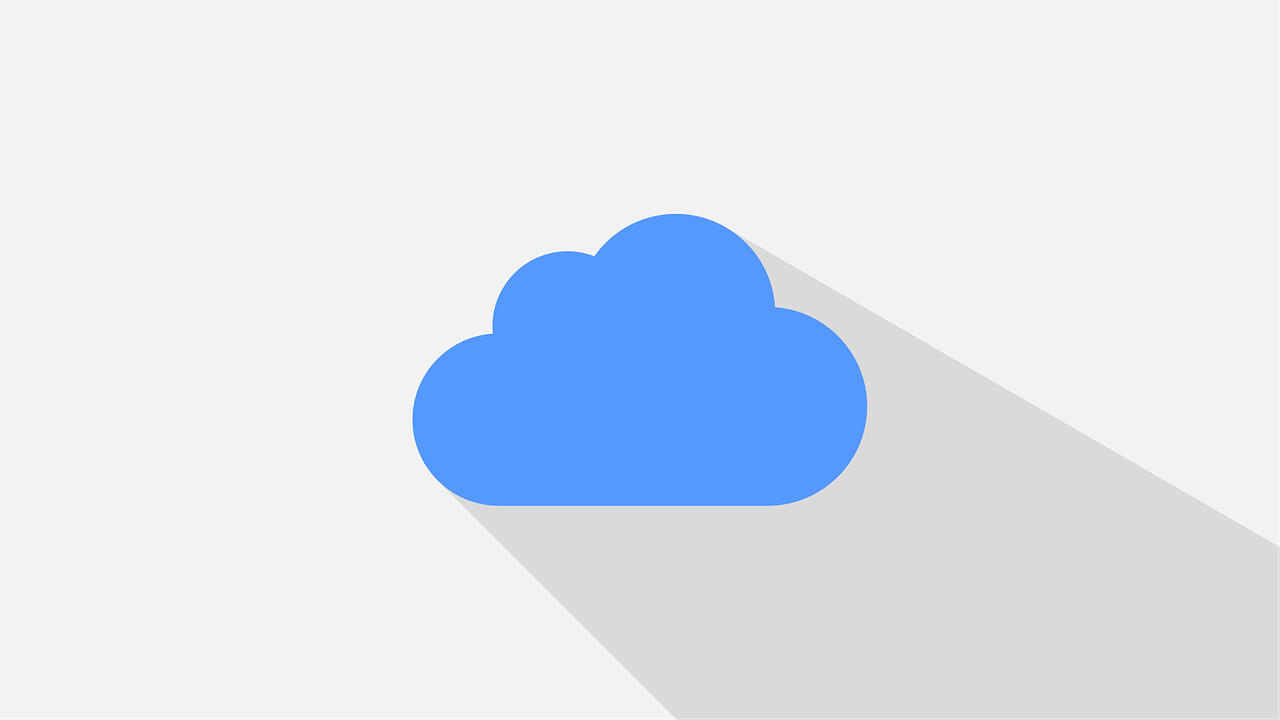
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Image Upload</title> </head> <body> <h1>Upload an Image</h1> <form method="post" action="" enctype="multipart/form-data"> <input type="file" name="uploadedFile" accept="image/*"> <input type="submit" value="Upload"> </form> <% If Request.TotalBytes > 0 Then ' 检查是否有文件上传 On Error Resume Next ' 忽略错误继续执行 Dim uploadedFile, filePath, fileSize, fileType, fileName, savePath, fileExtension, newFileName, fileStream, contentType, fileByteArray, fileLength, streamPos, streamNum, streamTemp, streamErr, uploadError_message, uploadSuccess_message, uploadFailed_message, uploadSuccessUrl, uploadFailedUrl, uploadMaxFileSize, uploadAllowedFileTypes, uploadFilePath, uploadFileName, uploadFileSize, uploadFileType, uploadFileExtension, uploadNewFileName, uploadFileStream, uploadContentType, uploadFileByteArray, uploadFileLength, uploadStreamPos, uploadStreamNum, uploadStreamTemp, uploadStreamErr, uploadErrorMessage, uploadSuccessMessage, uploadFailedMessage, uploadSuccessURL, uploadFailedURL, uploadMaxFileSizeValue, uploadAllowedFileTypesValue, uploadFilePathValue, uploadFileNameValue, uploadFileSizeValue, uploadFileTypeValue, uploadFileExtensionValue, uploadNewFileNameValue, uploadFileStreamValue, uploadContentTypeValue, uploadFileByteArrayValue, uploadFileLengthValue, uploadStreamPosValue, uploadStreamNumValue, uploadStreamTempValue, uploadStreamErrValue, uploadErrorMessageValue, uploadSuccessMessageValue, uploadFailedMessageValue, uploadSuccessURLValue, uploadFailedURLValue, uploadMaxFileSizeValue, uploadAllowedFileTypesValue, uploadFilePathValue, uploadFileNameValue, uploadFileSizeValue, uploadFileTypeValue, uploadFileExtensionValue, uploadNewFileNameValue, uploadFileStreamValue, uploadContentTypeValue, uploadFileByteArrayValue, uploadFileLengthValue, uploadStreamPosValue, uploadStreamNumValue, uploadStreamTempValue, uploadStreamErrValue, uploadErrorMessageValue, uploadSuccessMessageValue, uploadFailedMessageValue, uploadFailedMessageValue, uploadSuccessURLValue, uploadFailedURLValue, uploadMaxFileSizeValue, uploadAllowedFileTypesValue, uploadFilePathValue, uploadFileNameValue, uploadFileSizeValue, uploadFileTypeValue, uploadFileExtensionValue, uploadNewFileNameValue, uploadFileStreamValue, uploadContentTypeValue, uploadFileByteArrayValue, uploadFileLengthValue, uploadStreamPosValue, uploadStreamNumValue, uploadStreamTempValue, uploadStreamErrValue, uploadErrorMessageValue, uploadSuccessMessageValue, uploadFailedMessageValue, uploadFailedMessageValue, uploadSuccessURLValue, uploadFailedURLValue, uploadMaxFileSizeValue, uploadAllowedFileTypesValue, uploadFilePathValue, uploadFileNameValue, uploadFileSizeValue, uploadFileTypeValue, uploadFileExtensionValue, uploadNewFileNameValue, uploadFileStreamValue, uploadContentTypeValue, uploadFileByteArrayValue, uploadFileLengthValue, uploadStreamPosValue, uploadStreamNumValue, uploadStreamTempValue, uploadStreamErrValue, uploadErrorMessageValue, uploadSuccessMessageValue, uploadFailedMessageValue, uploadFailedMessageValue, uploadSuccessURLValue, uploadFailedURLValue, uploadMaxFileSizeValue, uploadAllowedFileTypesValue, uploadFilePathValue, uploadFileNameValue, uploadFileSizeValue, uploadFileTypeValue, uploadFileExtensionValue, uploadNewFileNameValue, uploadFileStreamValue, uploadContentTypeValue, uploadFileByteArrayValue, uploadFileLengthValue, uploadStreamPosValue, uploadStreamNumValue, uploadStreamTempValue, uploadStreamErrValue, uploadErrorMessageValue, uploadSuccessMessageValue, uploadFailedMessageValue, uploadSuccessURLValue, uploadFailedURLValue, uploadMaxFileSizeValue, uploadAllowedFileTypesValue, uploadFilePathValue, uploadFileNameValue, uploadFileSizeValue, uploadFileTypeValue, uploadFileExtensionValue, uploadNewFileNameValue, uploadFileStreamValue, uploadContentTypeValue, uploadFileByteArrayValue, uploadFileLengthValue, uploadStreamPosValue, uploadStreamNumValue, uploadStreamTempValue, uploadStreamErrValue, uploadErrorMessageValue, uploadSuccessMessageValue, uploadFailedMessageValue, uploadSuccessURLValue, uploadFailedURLValue, uploadMaxFileSizeValue,uploadAllowedFileTypesValue,uploadFilePathValue,uploadFileNameValue,uploadFileSizeValue,uploadFileTypeValue,uploadFileExtensionValue,uploadNewFileNameValue,uploadFileStreamValue,uploadContentTypeValue,uploadFileByteArrayValue,uploadFileLengthValue,uploadStreamPosValue,uploadStreamNumValue,uploadStreamTempValue,uploadStreamErrValue,uploadErrorMessageValue,uploadSuccessMessageValue,uploadFailedMessageValue,uploadSuccessURLValue,uploadFailedURLValue,uploadMaxFileSizeValue,uploadAllowedFileTypesValue,uploadFilePathValue,uploadFileNameValue,uploadFileSizeValue,uploadFileTypeValue,uploadFileExtensionValue,uploadNewFileNameValue,uploadFileStreamValue,uploadContentTypeValue,uploadFileByteArrayValue,uploadFileLengthValue,uploadStreamPosValue,uploadStreamNumValue,uploadStreamTempValue,uploadStreamErrValue,uploadErrorMessageValue,uploadSuccessMessageValue,uploadFailedMessageValue,uploadSuccessURLValue,uploadFailedURLValue,uploadMaxFileSize Value,uploadAllowedFileTypes Value,uploadFilePath Value,uploadFileName Value,uploadFileSize Value,uploadFileType Value,uploadFileExtension Value,uploadNew File Name Value,upload File Stream Value,upload Content Type Value,upload File Byte Array Value,upload File Length Value,upload Stream Pos Value,upload Stream Num Value,upload Stream Temp Value,upload Stream Err Value,upload Error Message Value,upload Success Message Value,upload Failed Message Value,upload Success URL Value,upload Failed URL Value,upload Max File Size Value,upload Allowed File Types Value,upload File Path Value,upload File Name Value,upload File Size Value,upload File Type Value,upload File Extension Value,upload New File Name Value,upload File Stream Value,upload Content Type Value,upload File Byte Array Value,upload File Length Value,upload Stream Pos Value,upload Stream Num Value,upload Stream Temp Value,upload Stream Err Value,upload Error Message Value,upload Success Message Value,upload Failed Message Value,upload Success URL Value,upload Failed URL Value,upload Max File Size Value,upload Allowed File Types Value,upload File Path Value,upload File Name Value,upload File Size Value,upload File Type Value,upload File Extension Value,upload New File Name Value,upload File Stream Value,upload Content Type Value,upload File Byte Array Value,upload File Byte Array Value,upload File Length Value,upload Stream Pos Value,upload Stream Num Value,upload Stream Temp Value,upload Stream Err Value,upload Error Message Value,upload Success Message Value,upload Failed Message Value,upload Success URL Value,upload Failed URL Value,upload Max File Size Value,upload Allowed File Types Value,upload File Path Value,upload File Name Value,upload File Size Value,upload File Size Value,upload File Type Value,upload File Type Value,upload File Extension Value,upload New File Name Value,upload File Stream Value,upload Content Type Value,upload File Byte Array Value,upload File Byte Array Value,upload File Length Value,upload Stream Pos Value,upload Stream Num Value,upload Stream Temp Value,upload Stream Err Value,upload Error Message Value,upload Success Message Value,upload Failed Message Value,upload Success URL Value,upload Failed URL Value,upload Max FileSize Value,upload Allowed FileTypes Value,upload FilePath Value,upload FileName Value,upload FileSize Value,upload FileSize Value,upload FileType Value,upload FileType Value,upload FileExtension Value,upload New FileName Value,upload FileStream Value,upload ContentType Value,upload FileByteArray Value,upload FileLength Value,upload StreamPos Value,upload StreamNum Value,upload StreamTemp Value,upload StreamErr Value,upload ErrorMessage Value,upload SuccessMessage Value,upload FailedMessage Value,upload SuccessURL Value,upload FailedURL Value,upload MaxFileSize Value,upload AllowedFileTypes Value,upload FilePath Value,upload FileName Value,upload FileSize Value,upload FileSize Value,upload FileType Value,upload FileType Value,upload FileExtension Value,upload New FileName Value,upload FileStream Value,contentType=""&savedPath&"" alt=""Uploaded image""" %> Response.Write "Upload successful!" Response.End ' 如果上传成功则结束响应 End If %> Response.Write "Upload failed." %> Response.End ' 如果上传失败则结束响应 End If % End If % End If % Response.Write "Upload failed." % Response.End ' 如果上传失败则结束响应 End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End If % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if % End if%>
以上内容就是解答有关“asp 调用只带图片”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1357082.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复