浮动购物车 JavaScript 实现
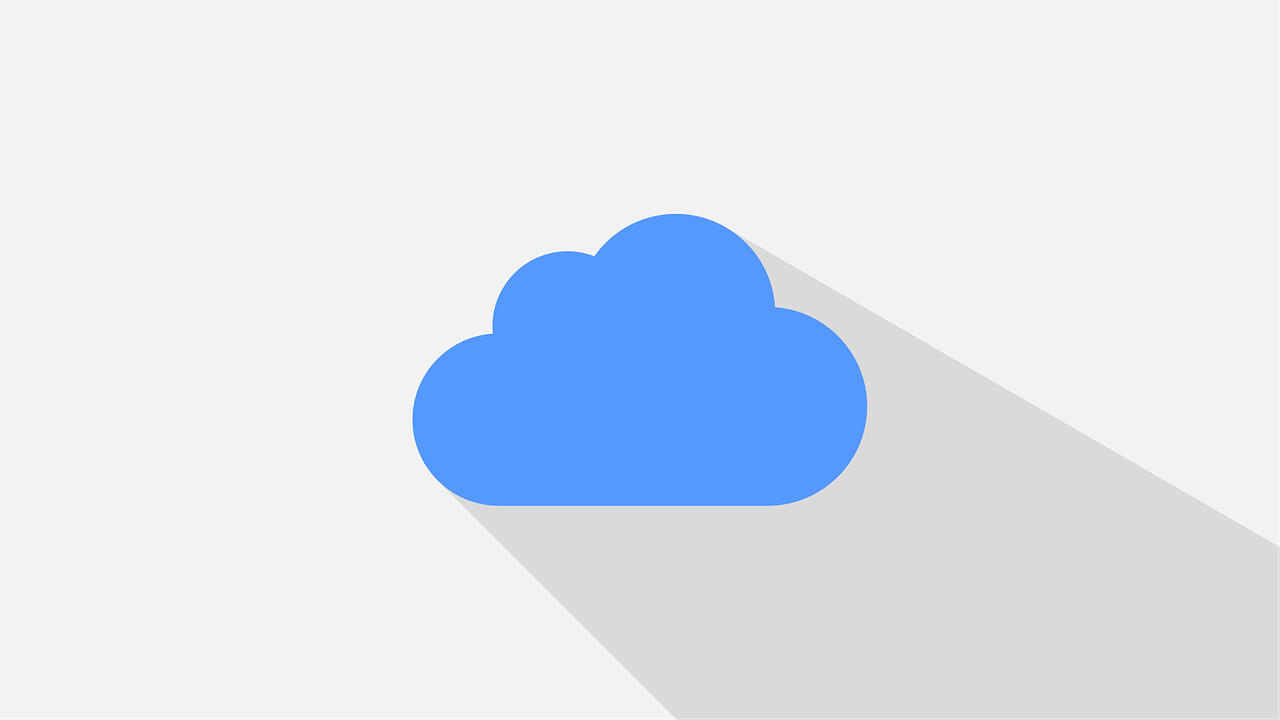
在现代网页设计中,浮动购物车是一种常见的用户界面元素,它允许用户在浏览网站时随时查看他们添加到购物车中的商品,这种功能不仅提高了用户体验,还有助于提高转化率,本文将介绍如何使用 HTML、CSS 和 JavaScript 实现一个基本的浮动购物车。
H3: 准备工作
在开始编写代码之前,我们需要准备以下文件:
1、index.html
主页面文件
2、styles.css
样式文件
3、script.js
JavaScript 文件
H3: 创建 HTML 结构
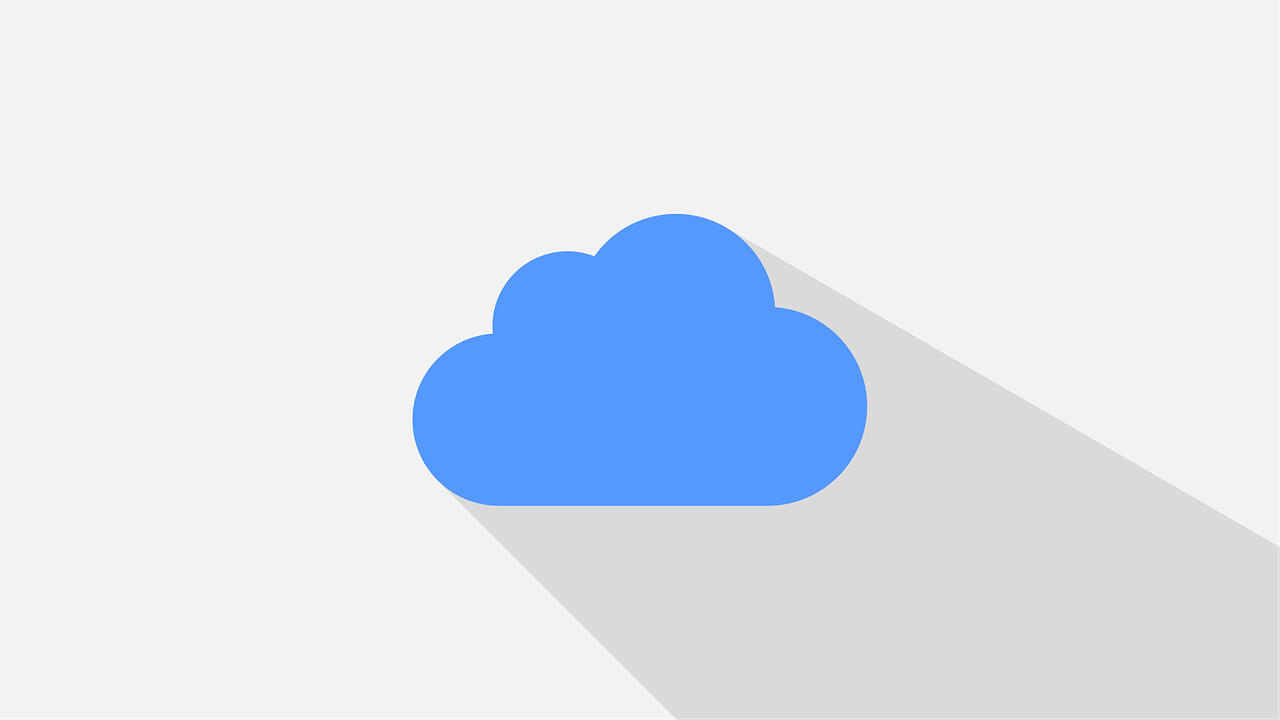
我们在index.html
文件中创建一个基本的 HTML 结构。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>浮动购物车示例</title> <link rel="stylesheet" href="styles.css"> </head> <body> <header> <h1>在线商店</h1> </header> <main> <div class="product" data-id="1" data-name="商品1" data-price="100"> <h2>商品1</h2> <p>价格: $100</p> <button class="add-to-cart">加入购物车</button> </div> <div class="product" data-id="2" data-name="商品2" data-price="200"> <h2>商品2</h2> <p>价格: $200</p> <button class="add-to-cart">加入购物车</button> </div> </main> <div id="floating-cart" class="hidden"> <h3>购物车</h3> <table> <thead> <tr> <th>商品名称</th> <th>数量</th> <th>单价</th> <th>总价</th> <th>操作</th> </tr> </thead> <tbody id="cart-items"></tbody> </table> <p id="total-price">总价: $0</p> </div> <script src="script.js"></script> </body> </html>
H3: 添加 CSS 样式
我们在styles.css
文件中添加一些基本的样式。
body { font-family: Arial, sans-serif; } header { background-color: #4CAF50; color: white; padding: 1em; text-align: center; } .product { border: 1px solid #ccc; margin: 1em; padding: 1em; text-align: center; } .add-to-cart { background-color: #4CAF50; color: white; border: none; padding: 0.5em 1em; cursor: pointer; } #floating-cart { position: fixed; top: 50%; right: 20px; transform: translateY(-50%); background-color: white; border: 1px solid #ccc; padding: 1em; width: 300px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } #floating-cart.hidden { display: none; } table { width: 100%; border-collapse: collapse; } th, td { border: 1px solid #ccc; padding: 0.5em; text-align: left; }
H3: 编写 JavaScript 逻辑
我们在script.js
文件中编写 JavaScript 逻辑来实现浮动购物车的功能。
document.addEventListener('DOMContentLoaded', () => {
const cart = document.getElementById('floating-cart');
const cartItemsTableBody = document.getElementById('cart-items');
const totalPriceElement = document.getElementById('total-price');
const products = document.querySelectorAll('.product');
let cartItems = [];
let totalPrice = 0;
function updateCartUI() {
cartItemsTableBody.innerHTML = '';
cartItems.forEach(item => {
const row = document.createElement('tr');
row.innerHTML = `
<td>${item.name}</td>
<td>${item.quantity}</td>
<td>$${item.price}</td>
<td>$${(item.price * item.quantity).toFixed(2)}</td>
<td><button class="remove-item" data-id="${item.id}">移除</button></td>
`;
cartItemsTableBody.appendChild(row);
});
totalPriceElement.textContent =总价: $${totalPrice.toFixed(2)}
;
}
products.forEach(product => {
product.querySelector('.add-to-cart').addEventListener('click', () => {
const id = product.getAttribute('data-id');
const name = product.getAttribute('data-name');
const price = parseFloat(product.getAttribute('data-price'));
const existingItem = cartItems.find(item => item.id === id);
if (existingItem) {
existingItem.quantity++;
} else {
cartItems.push({ id, name, price, quantity: 1 });
}
totalPrice += price;
updateCartUI();
});
});
document.addEventListener('click', event => {
if (event.target.classList.contains('remove-item')) {
const id = event.target.getAttribute('data-id');
const index = cartItems.findIndex(item => item.id === id);
if (index > -1) {
const removedItem = cartItems.splice(index, 1)[0];
totalPrice -= removedItem.price * removedItem.quantity;
updateCartUI();
}
} else if (!event.target.closest('#floating-cart')) {
cart.classList.add('hidden');
} else {
cart.classList.remove('hidden');
}
});
});
H3: 归纳
通过以上步骤,我们实现了一个简单的浮动购物车功能,用户可以点击“加入购物车”按钮将商品添加到购物车,并实时查看购物车中的商品和总价,还可以通过点击“移除”按钮从购物车中删除商品,这个示例展示了如何使用 HTML、CSS 和 JavaScript 构建一个交互式的用户界面组件。
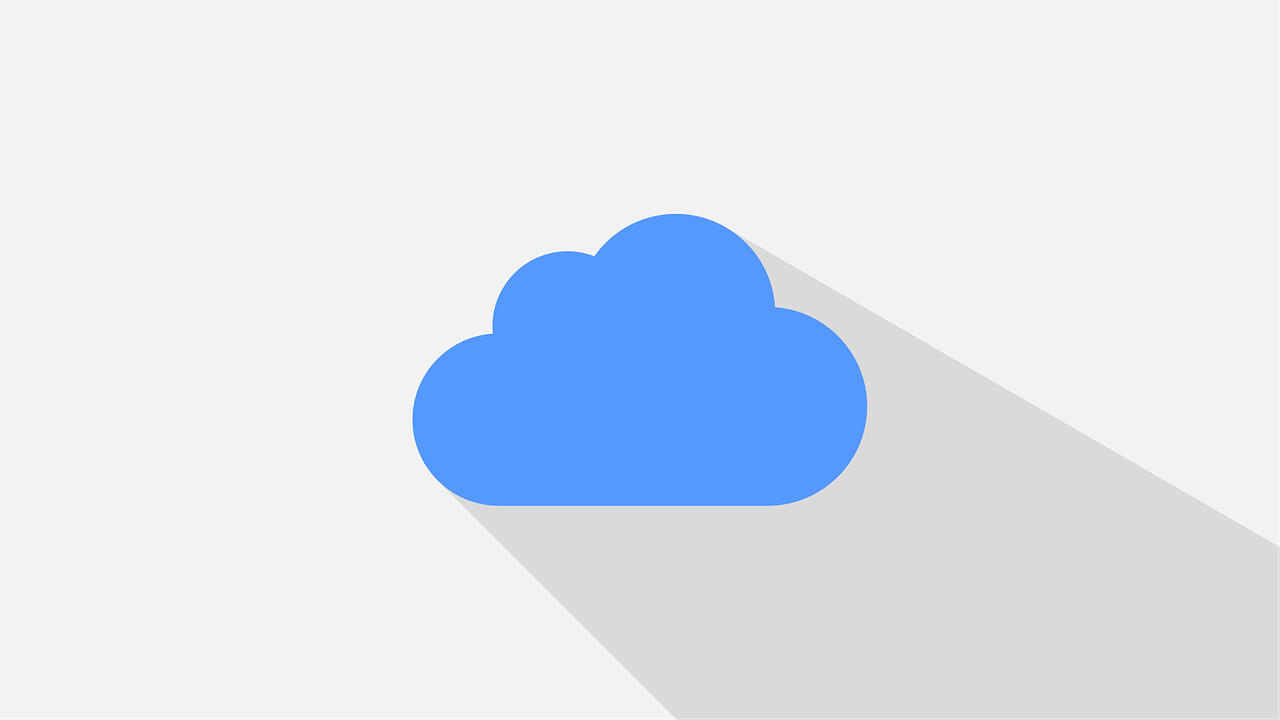
小伙伴们,上文介绍了“浮动购物车js”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1355866.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复