ASP.NET 端口转发
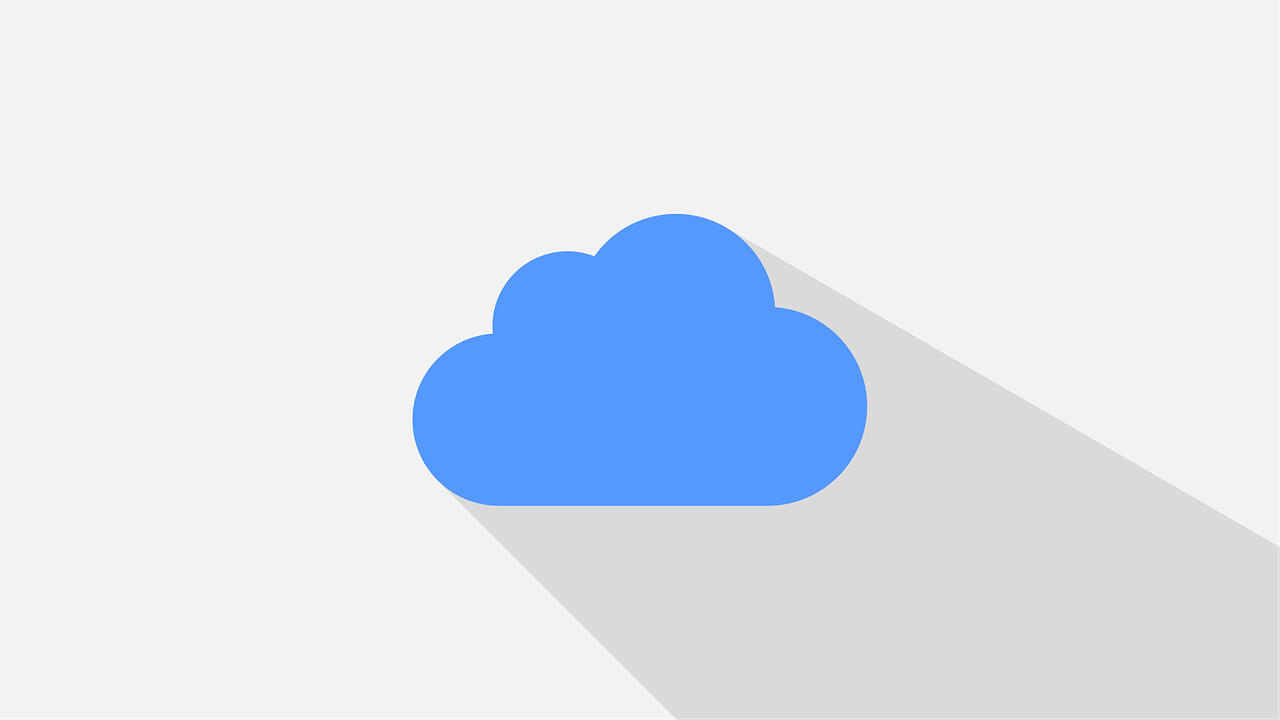
在现代Web开发中,前后端分离的架构越来越普遍,前端应用通常托管在Node.js服务器上,通过Axios等工具访问后端API,有时前端和后端可能运行在不同的服务器或端口上,这就需要进行跨域请求,为了解决这个问题,我们可以使用反向代理来转发请求,本文将详细介绍如何在ASP.NET Core中实现端口转发。
什么是端口转发?
端口转发是一种网络技术,用于将来自一个端口的网络流量重定向到另一个端口,这在开发环境中非常有用,特别是在需要跨域访问资源时,通过端口转发,可以将对特定URL路径的请求转发到指定的内部服务,从而实现跨域资源共享。
为什么使用ASP.NET Core进行端口转发?
ASP.NET Core是一个高性能、模块化且跨平台的框架,非常适合构建现代Web应用程序,它提供了强大的中间件模式,可以轻松地拦截和处理HTTP请求,通过定义自定义中间件,我们可以实现灵活的请求转发逻辑。
如何实现端口转发
1. 创建检测约定URL的接口与实现
我们需要定义一个接口IUrlRewriter
,用于检测URL是否有对应的前缀,并生成新的URL地址。
public interface IUrlRewriter { Task<Uri> RewriteUri(HttpContext context); }
实现这个接口:
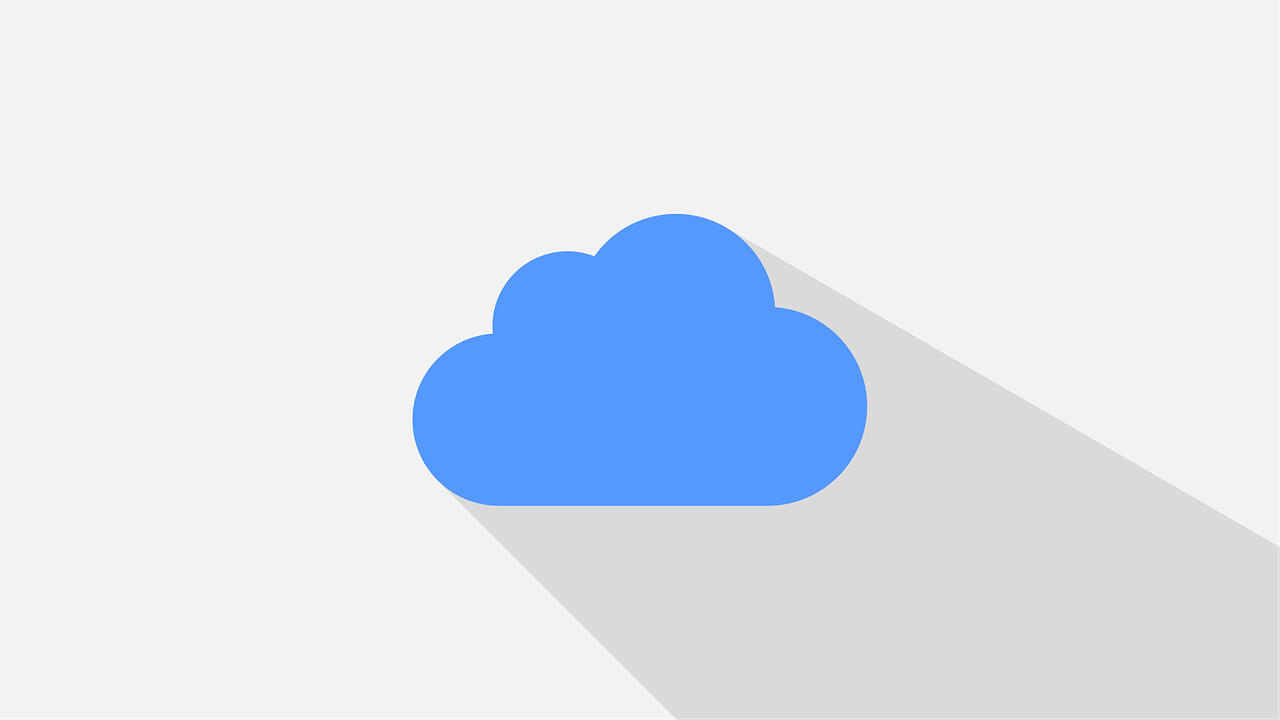
public class PrefixRewriter : IUrlRewriter { private readonly PathString _prefix; // 前缀值 private readonly string _newHost; // 转发的地址 public PrefixRewriter(PathString prefix, string newHost) { _prefix = prefix; _newHost = newHost; } public Task<Uri> RewriteUri(HttpContext context) { if (context.Request.Path.StartsWithSegments(_prefix)) // 判断访问是否含有前缀 { var newUri = context.Request.Path.Value.Remove(0, _prefix.Value.Length) + context.Request.QueryString; var targetUri = new Uri(_newHost + newUri); return Task.FromResult(targetUri); } return Task.FromResult((Uri)null); } }
2. 创建代理转发需要的ProxyHttpClient
创建一个独立的ProxyHttpClient
,用于区分代理转发的HTTP客户端,方便后期添加日志或其他处理。
public class ProxyHttpClient { public HttpClient Client { get; private set; } public ProxyHttpClient(HttpClient httpClient) { Client = httpClient; } }
3. 创建代理转发的中间件
创建代理转发的中间件ProxyMiddleware
,中间件的主要功能是拦截访问,检测前缀,并进行转发。
public class ProxyMiddleware { private const string CDN_HEADER_NAME = "Cache-Control"; private static readonly string[] NotForwardedHttpHeaders = new[] { "Connection", "Host" }; private readonly RequestDelegate _next; private readonly ILogger<ProxyMiddleware> _logger; public ProxyMiddleware(RequestDelegate next, ILogger<ProxyMiddleware> logger) { _next = next; _logger = logger; } public async Task Invoke(HttpContext context, IUrlRewriter urlRewriter, ProxyHttpClient proxyHttpClient) { var targetUri = await urlRewriter.RewriteUri(context); if (targetUri != null) { // 发送请求到新的目标地址 var response = await proxyHttpClient.Client.SendAsync(new HttpRequestMessage(new HttpMethod(context.Request.Method), targetUri)); // 将响应写回原始请求者 context.Response.StatusCode = (int)response.StatusCode; using (var responseStream = await response.Content.ReadAsStreamAsync()) { await responseStream.CopyToAsync(context.Response.Body); } } else { await _next(context); } } }
4. 配置中间件管道
在Startup.cs
文件中,配置中间件管道以使用我们定义的ProxyMiddleware
。
public void Configure(IApplicationBuilder app, IHostingEnvironment env) { app.UseMiddleware<ProxyMiddleware>(); // 其他中间件配置... }
示例代码
以下是一个完整的示例代码,展示了如何使用上述组件实现端口转发。
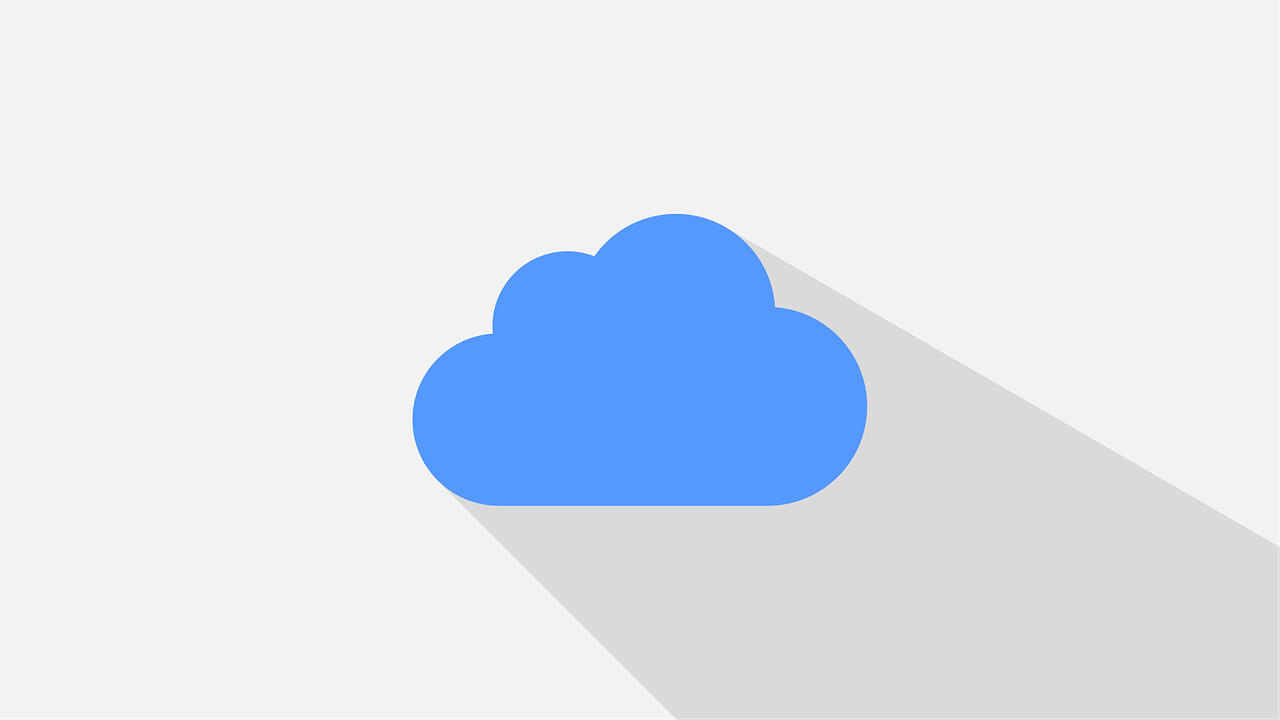
Startup.cs
using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Logging; using System; using System.IO; using System.Threading.Tasks; using Microsoft.AspNetCore.Http; using Microsoft.Extensions.Logging.Abstractions; using Microsoft.Extensions.Options; using Newtonsoft.Json.Serialization; using Microsoft.AspNetCore.Mvc.Formatters; using Microsoft.AspNetCore.Mvc; using System.Net.Http; using Microsoft.Extensions.DependencyInjection.Extensions; public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } public void ConfigureServices(IServiceCollection services) { services.AddMvc(); services.TryAddSingleton<IHttpContextAccessor, HttpContextAccessor>(); services.AddLogging(builder => builder.AddConsole()); services.AddScoped<IUrlRewriter, PrefixRewriter>(); services.AddScoped<ProxyHttpClient>(provider => new ProxyHttpClient(new HttpClient())); } public void Configure(IApplicationBuilder app, IHostingEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseHsts(); } app.UseMiddleware<ProxyMiddleware>(); app.UseMvc(); } }
Program.cs
using Microsoft.AspNetCore; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Logging; using System; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.PlatformAbstractions; namespace AspNetPortForwardingExample { public class Program { public static void Main(string[] args) { BuildWebHost(args).Run(); } public static IWebHost BuildWebHost(string[] args) => WebHost.CreateDefaultBuilder(args) .UseStartup<Startup>() .Build(); } }
通过以上步骤,我们实现了一个简单的ASP.NET Core端口转发机制,这种机制可以帮助我们在开发过程中解决跨域请求的问题,同时也为微服务架构中的服务间通信提供了便利,希望本文能对你有所帮助!
小伙伴们,上文介绍了“asp 端口转发”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1349652.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复