在构建动态网站时,站内信系统是一种常见的功能,它允许用户之间进行私密通信,ASP(Active Server Pages)作为一种服务器端脚本编写环境,可以方便地实现这一功能,本文将详细介绍如何使用ASP技术构建一个基本的站内信系统,包括数据库设计、前后端代码实现以及一些常见问题的解答。
一、数据库设计
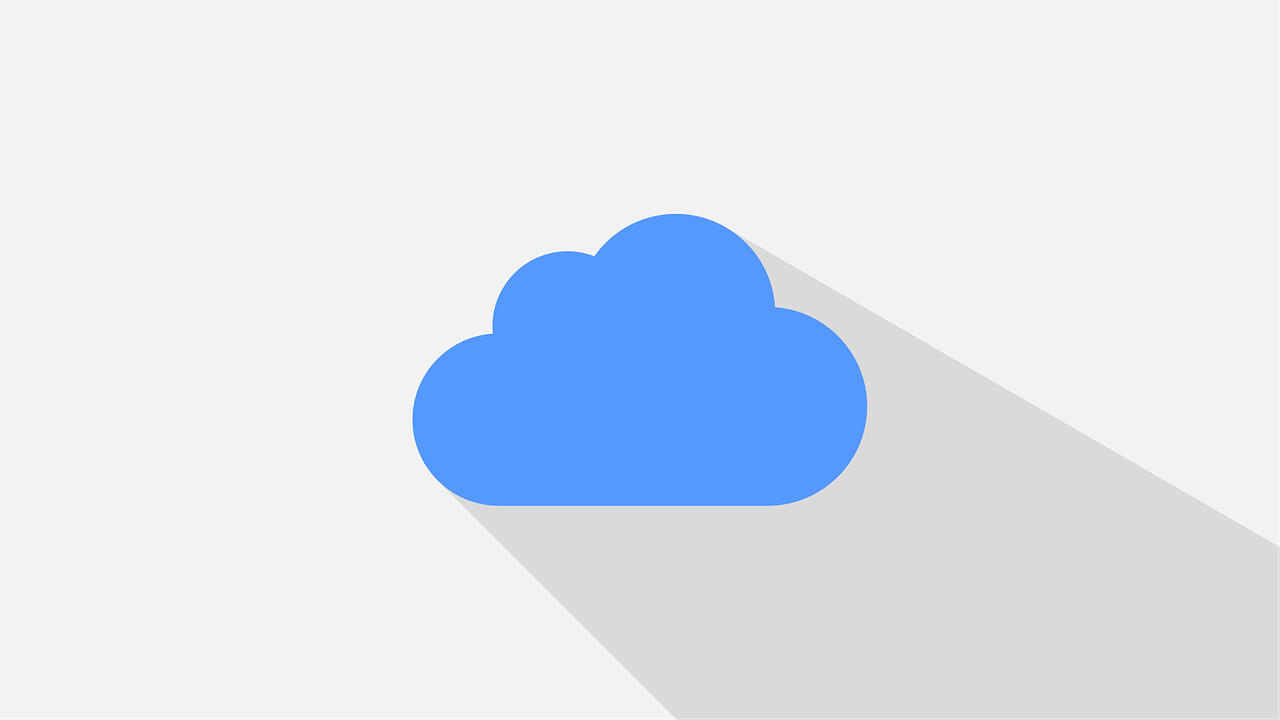
为了存储站内信的数据,我们需要设计一个数据库表来保存相关信息,以下是一个示例的表结构:
字段名 | 数据类型 | 长度 | 是否主键 | 说明 |
MessageID | INT | 4 | 是 | 消息ID |
SenderID | INT | 4 | 否 | 发送者ID |
RecipientID | INT | 4 | 否 | 接收者ID |
Subject | NVARCHAR | 255 | 否 | 主题 |
Body | NTEXT | 否 | 消息内容 | |
Timestamp | DATETIME | 否 | 发送时间 |
二、前端页面设计
1. 登录页面 (login.asp)
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <title>登录</title> </head> <body> <h2>登录</h2> <form action="checklogin.asp" method="post"> <label for="username">用户名:</label> <input type="text" id="username" name="username"><br><br> <label for="password">密码:</label> <input type="password" id="password" name="password"><br><br> <input type="submit" value="登录"> </form> </body> </html>
2. 主页面 (index.asp)
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <title>站内信</title> </head> <body> <h2>站内信</h2> <a href="sendmessage.asp">发送消息</a><br> <a href="inbox.asp">收件箱</a><br> <a href="logout.asp">登出</a> </body> </html>
3. 发送消息页面 (sendmessage.asp)
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <title>发送消息</title> </head> <body> <h2>发送消息</h2> <form action="sendmsg.asp" method="post"> <label for="recipient">接收者:</label> <input type="text" id="recipient" name="recipient"><br><br> <label for="subject">主题:</label> <input type="text" id="subject" name="subject"><br><br> <label for="body">内容:</label> <textarea id="body" name="body"></textarea><br><br> <input type="submit" value="发送"> </form> </body> </html>
4. 收件箱页面 (inbox.asp)
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <title>收件箱</title> </head> <body> <h2>收件箱</h2> <% Dim conn, rs, sql Set conn = Server.CreateObject("ADODB.Connection") conn.Open "Provider=SQLOLEDB;Data Source=(local);Initial Catalog=YourDatabase;User ID=yourusername;Password=yourpassword" sql = "SELECT * FROM Messages WHERE RecipientID=" & Session("UserID") & " ORDER BY Timestamp DESC" Set rs = conn.Execute(sql) %> <table border="1"> <tr> <th>发件人</th> <th>主题</th> <th>内容</th> <th>时间</th> </tr> <% Do While Not rs.EOF %> <tr> <td><%= rs("SenderID") %></td> <td><%= rs("Subject") %></td> <td><%= rs("Body") %></td> <td><%= rs("Timestamp") %></td> </tr> <% rs.MoveNext Loop %> </table> <% rs.Close Set rs = Nothing conn.Close Set conn = Nothing %> </body> </html>
三、后端逻辑实现
1. 登录验证 (checklogin.asp)
<% Dim conn, rs, username, password, userID username = Request.Form("username") password = Request.Form("password") Set conn = Server.CreateObject("ADODB.Connection") conn.Open "Provider=SQLOLEDB;Data Source=(local);Initial Catalog=YourDatabase;User ID=yourusername;Password=yourpassword" sql = "SELECT * FROM Users WHERE Username='" & username & "' AND Password='" & password & "'" Set rs = conn.Execute(sql) If Not rs.EOF Then userID = rs("UserID") Session("UserID") = userID Response.Redirect("index.asp") Else Response.Write "用户名或密码错误" End If rs.Close Set rs = Nothing conn.Close Set conn = Nothing %>
2. 发送消息处理 (sendmsg.asp)
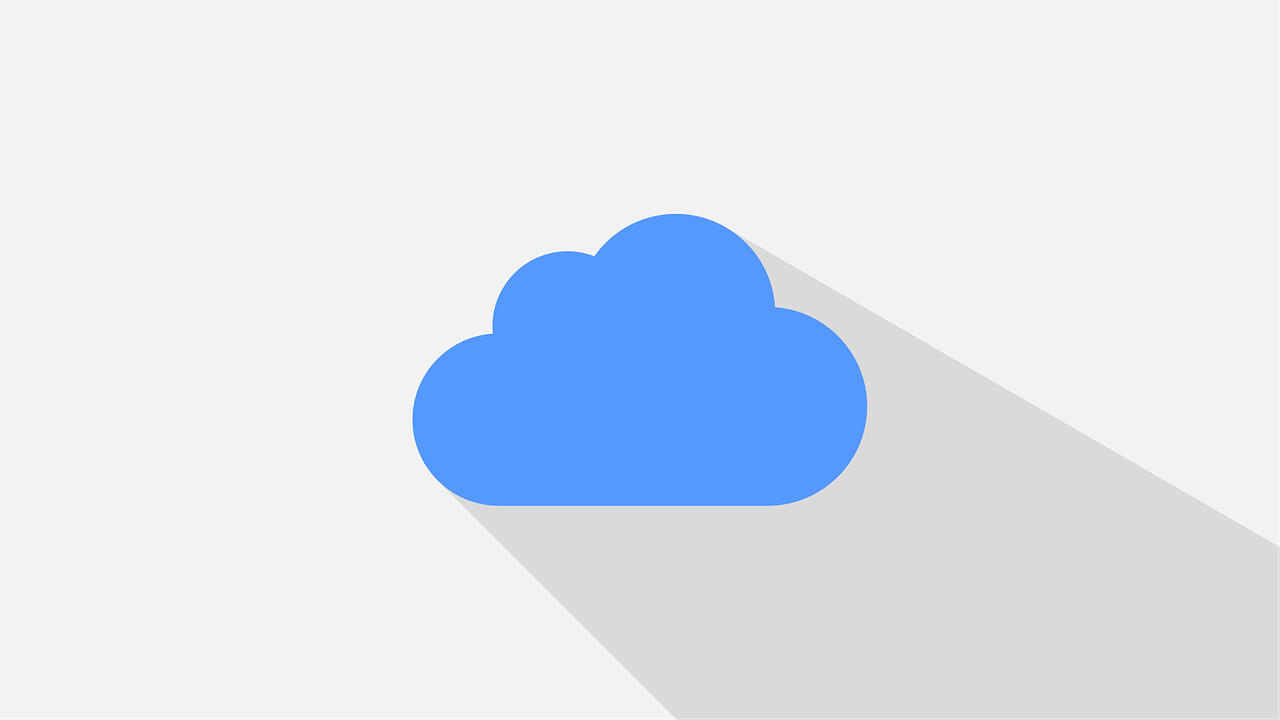
<% Dim conn, recipientID, subject, body, senderID, sql recipientID = Request.Form("recipient") subject = Request.Form("subject") body = Request.Form("body") senderID = Session("UserID") Set conn = Server.CreateObject("ADODB.Connection") conn.Open "Provider=SQLOLEDB;Data Source=(local);Initial Catalog=YourDatabase;User ID=yourusername;Password=yourpassword" sql = "INSERT INTO Messages (SenderID, RecipientID, Subject, Body, Timestamp) VALUES (" & senderID & ", " & recipientID & ", '" & subject & "', '" & body & "', GETDATE())" conn.Execute(sql) Response.Redirect("index.asp") conn.Close Set conn = Nothing %>
四、FAQs
问题1:如何更改站内信的主题?
答:要更改站内信的主题,可以在发送消息时修改表单中的subject
字段值,在sendmessage.asp
页面中,将<input type="text" id="subject" name="subject">
的值改为你想要的新主题即可,然后在sendmsg.asp
中,这个新的主题会被插入到数据库中。
问题2:如何删除收到的消息?
答:要删除收到的消息,可以在inbox.asp
页面中添加一个删除链接或按钮,当用户点击该链接或按钮时,可以调用一个ASP脚本来执行删除操作,可以在inbox.asp
中添加如下代码:
<% Dim messageID, conn, sql messageID = Request.QueryString("id") Set conn = Server.CreateObject("ADODB.Connection") conn.Open "Provider=SQLOLEDB;Data Source=(local);Initial Catalog=YourDatabase;User ID=yourusername;Password=yourpassword" sql = "DELETE FROM Messages WHERE MessageID=" & messageID conn.Execute(sql) Response.Redirect("inbox.asp") conn.Close Set conn = Nothing %>
这样,当用户点击删除链接时,会传递当前消息的ID给上述脚本,然后该脚本会从数据库中删除对应的记录。
到此,以上就是小编对于“asp 站内信”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1349085.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复