在现代软件开发中,正则表达式(Regular Expressions)是一个强大的工具,用于匹配和操作字符串,Boost库中的正则表达式模块为C++开发者提供了丰富的功能,而JavaScript的正则表达式功能虽然内置于语言之中,但同样强大且灵活,本文将探讨如何在C++中使用Boost库处理正则表达式,以及如何在JavaScript中高效地使用正则表达式。
C++中的Boost正则表达式
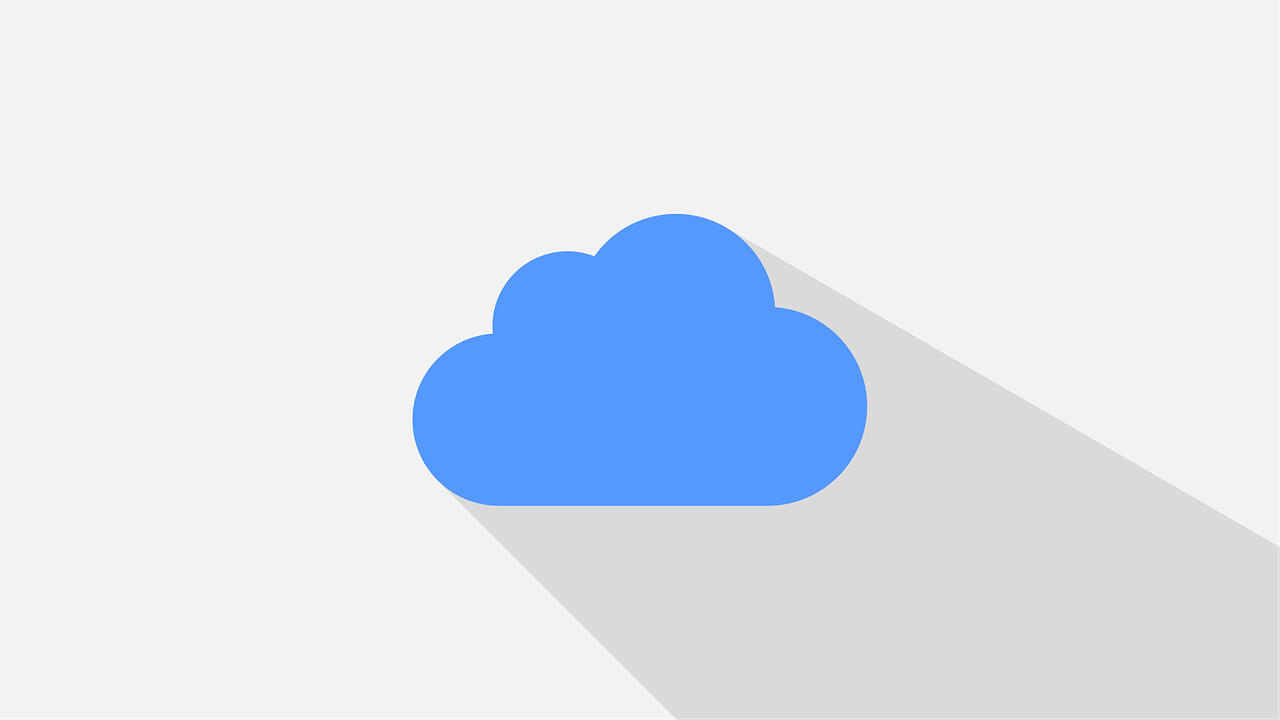
安装与配置
在使用Boost正则表达式之前,首先需要确保已经安装了Boost库,可以通过以下步骤进行安装:
1、下载Boost库:从[Boost官方网站](https://www.boost.org/)下载最新版本的源代码。
2、解压并构建:根据操作系统的不同,执行相应的构建命令,在Linux系统上,可以使用bootstrap.sh
脚本进行构建。
3、配置项目:将Boost库的头文件路径和库文件路径添加到项目的编译器设置中。
基本用法
下面是一个简单的例子,演示如何使用Boost正则表达式来匹配一个字符串:
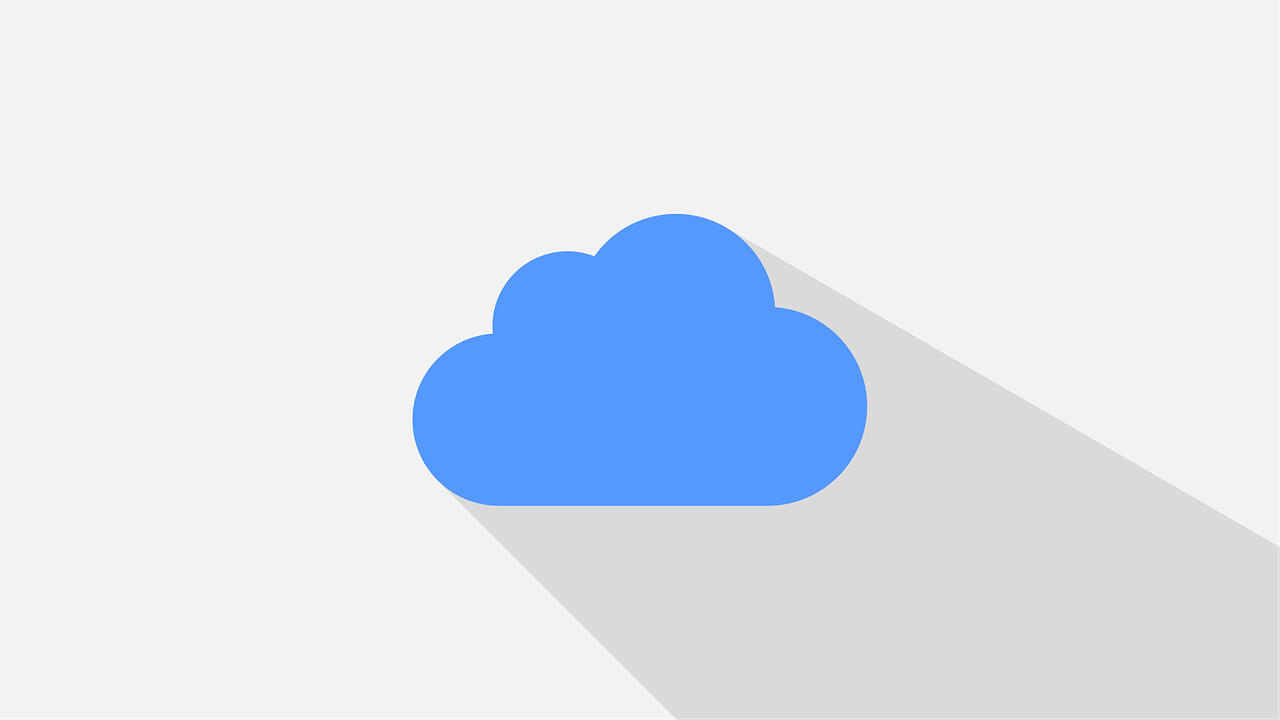
#include <iostream> #include <string> #include <boost/regex.hpp> int main() { std::string text = "Hello, world!"; boost::regex pattern("world"); if (boost::regex_search(text, pattern)) { std::cout << "Pattern found!" << std::endl; } else { std::cout << "Pattern not found." << std::endl; } return 0; }
高级特性
Boost正则表达式模块还支持许多高级特性,如命名捕获组、条件匹配等,以下是一些示例代码:
命名捕获组:
#include <iostream> #include <string> #include <boost/regex.hpp> int main() { std::string text = "John Doe"; boost::regex pattern("(?<first_name> John)(?<last_name> Doe)"); boost::smatch matches; if (boost::regex_search(text, matches, pattern)) { std::cout << "First Name: " << matches["first_name"] << std::endl; std::cout << "Last Name: " << matches["last_name"] << std::endl; } return 0; }
条件匹配:
#include <iostream> #include <string> #include <boost/regex.hpp> int main() { std::string text = "age=25"; boost::regex pattern("age=(\d+)"); boost::smatch match; if (boost::regex_search(text, match, pattern)) { int age = std::stoi(match[1].str()); if (age >= 18) { std::cout << "Adult" << std::endl; } else { std::cout << "Minor" << std::endl; } } return 0; }
JavaScript中的正则表达式
JavaScript内置了对正则表达式的支持,通过RegExp
对象可以方便地进行各种字符串匹配和替换操作,以下是一些常见的用法:
基本用法
let text = "Hello, world!"; let pattern = /world/; if (pattern.test(text)) { console.log("Pattern found!"); } else { console.log("Pattern not found."); }
高级特性
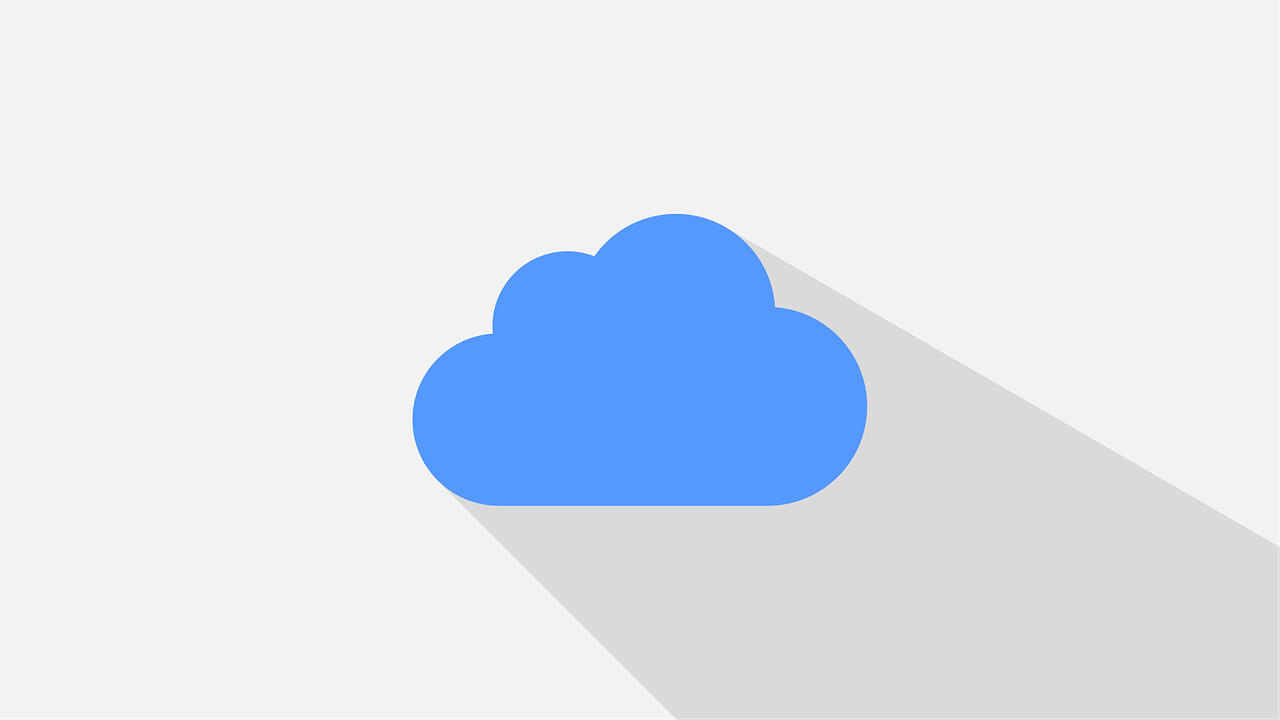
捕获组:
let text = "John Doe"; let pattern = /(John) (Doe)/; let match = text.match(pattern); if (match) { console.log("First Name: " + match[1]); console.log("Last Name: " + match[2]); }
替换:
let text = "The quick brown fox jumps over the lazy dog."; let newText = text.replace(/quick/, "slow"); console.log(newText); // The slow brown fox jumps over the lazy dog.
全局匹配:
let text = "apple banana apple grape"; let pattern = /apple/g; let matches = text.match(pattern); console.log(matches); // ["apple", "apple"]
表格对比
特性 | C++ Boost 正则表达式 | JavaScript 正则表达式 |
基础匹配 | boost::regex_search | pattern.test |
命名捕获组 | (? | 不支持 |
条件匹配 | 支持 | 不支持 |
替换 | boost::regex_replace | string.replace |
全局匹配 | boost::smatch with global flag | pattern.exec in a loop |
分组引用 | (?:...) | (...) |
非贪婪匹配 | *? ,+? ,?? | *? ,+? ,?? |
零宽断言 | (?=...) ,(?!...) | (?=...) ,(?!...) |
FAQs
Q1: 如何在C++中使用Boost正则表达式进行不区分大小写的匹配?
A1: 在C++中使用Boost正则表达式进行不区分大小写的匹配,可以在正则表达式模式前添加(?i)
修饰符。
#include <iostream> #include <string> #include <boost/regex.hpp> int main() { std::string text = "Hello, World!"; boost::regex pattern("(?i)hello"); if (boost::regex_search(text, pattern)) { std::cout << "Pattern found!" << std::endl; } else { std::cout << "Pattern not found." << std::endl; } return 0; }
Q2: 如何在JavaScript中创建一个动态的正则表达式?
A2: 在JavaScript中,可以使用RegExp
构造函数来创建动态的正则表达式。
let dynamicPattern = new RegExp("world", "i"); // 'i'表示不区分大小写 let text = "Hello, World!"; if (dynamicPattern.test(text)) { console.log("Pattern found!"); } else { console.log("Pattern not found."); }
到此,以上就是小编对于“boost正则表达式js”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1348087.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复