宿舍的英语单词是什么? (Windows下stl.h)
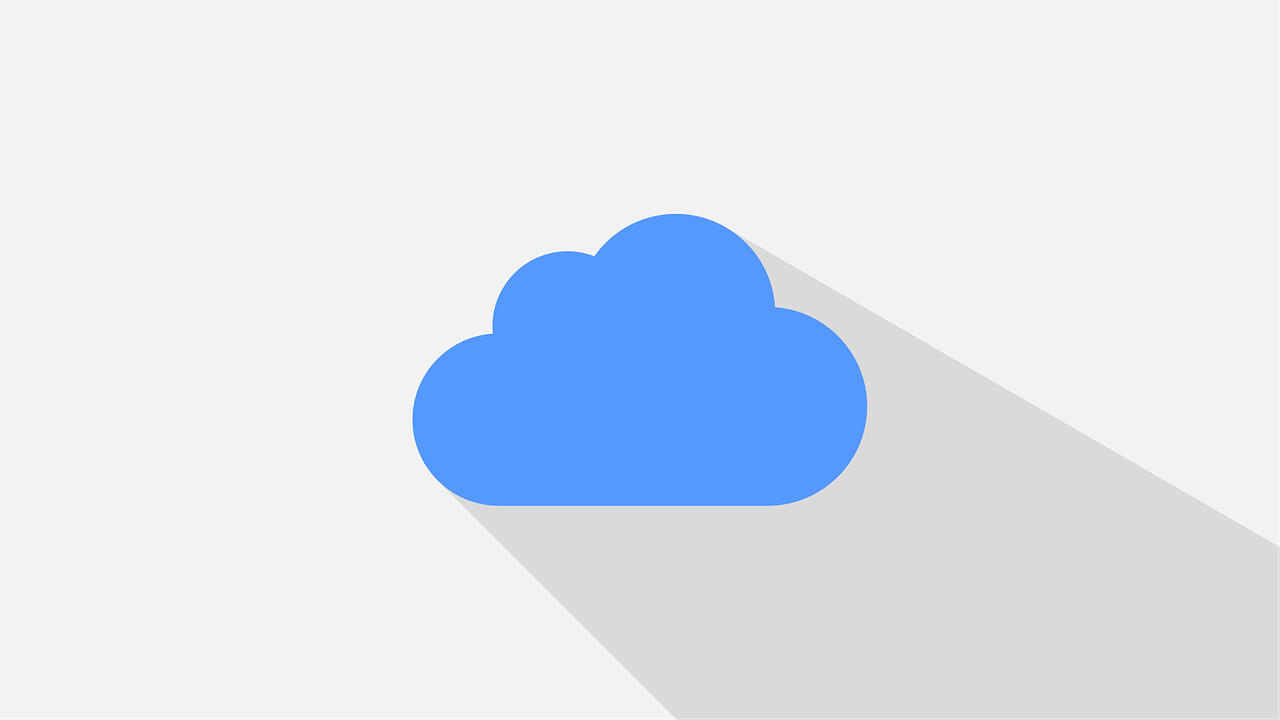
在Windows操作系统中,STL(Standard Template Library,标准模板库)是一个非常重要的编程工具,STL提供了许多常用的数据结构和算法,极大地方便了开发者的工作,本文将探讨如何在Windows环境下使用STL中的set
和map
容器,并展示它们的基本用法及操作。
STL中的set和map
1. set容器
std::set
是一种关联容器,它内部的元素是按顺序排列的,每个元素都是唯一的,不允许重复。set
通常用于存储需要保持有序且不重复的数据。
2. map容器
std::map
也是一种关联容器,它由键值对组成,每个键都映射到一个值。map
会根据键自动排序,因此可以快速查找、插入和删除元素。
基本用法及操作
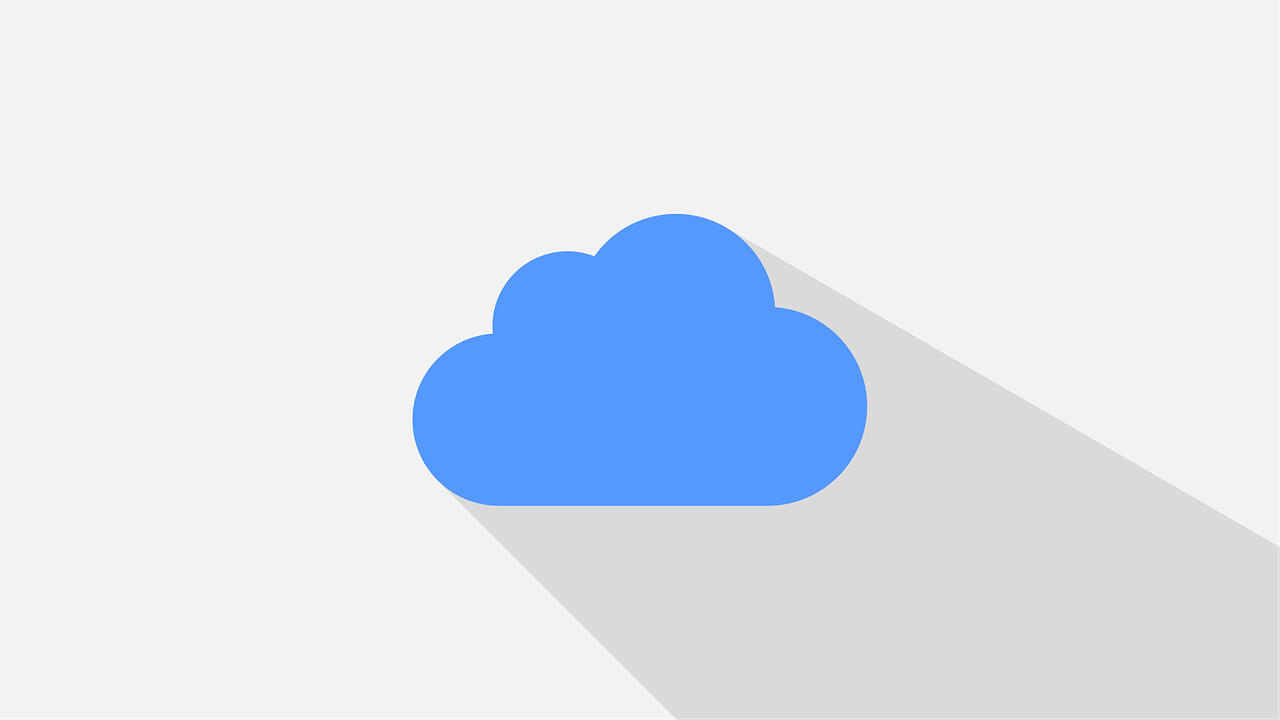
以下是一些常见的操作示例:
1. 创建和使用set
#include <iostream> #include <set> int main() { std::set<int> mySet; // 插入元素 mySet.insert(3); mySet.insert(1); mySet.insert(4); mySet.insert(1); // 重复元素不会被插入 mySet.insert(5); mySet.insert(9); // 遍历集合 std::cout << "Set elements: "; for (auto it = mySet.begin(); it != mySet.end(); ++it) { std::cout << *it << " "; } std::cout << std::endl; return 0; }
输出结果:
Set elements: 1 3 4 5 9
2. 创建和使用map
#include <iostream> #include <map> int main() { std::map<int, std::string> myMap; // 插入元素 myMap[1] = "one"; myMap[3] = "three"; myMap[4] = "four"; myMap[1] = "uno"; // 更新已有键的值 myMap[5] = "five"; myMap[9] = "nine"; // 遍历map std::cout << "Map elements: "; for (auto it = myMap.begin(); it != myMap.end(); ++it) { std::cout << it->first << " => " << it->second << " "; } std::cout << std::endl; return 0; }
输出结果:
Map elements: 1 => uno 3 => three 4 => four 5 => five 9 => nine
高级操作及示例
1. 删除元素
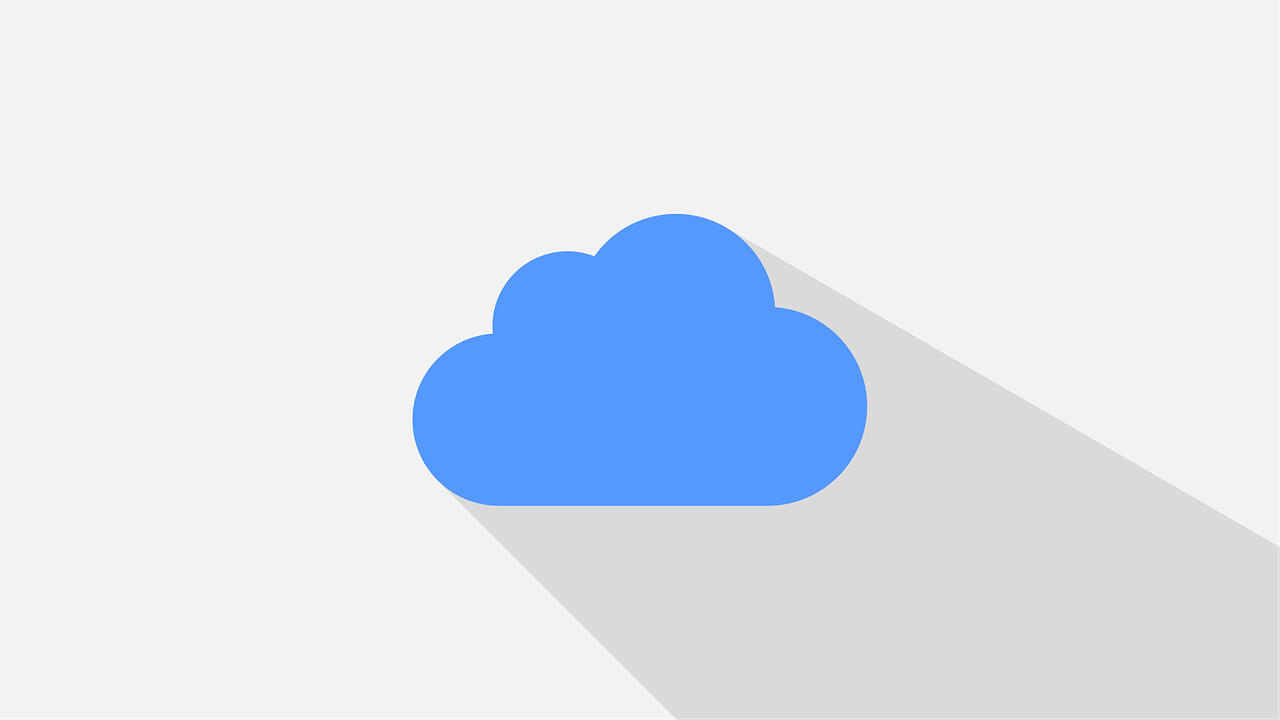
从set
中删除元素:
mySet.erase(3); // 删除键为3的元素
从map
中删除元素:
myMap.erase(3); // 删除键为3的元素及其对应的值
2. 查找元素
在set
中查找元素:
auto it = mySet.find(4); if (it != mySet.end()) { std::cout << "Found element: " << *it << std::endl; } else { std::cout << "Element not found" << std::endl; }
在map
中查找元素:
auto it = myMap.find(4); if (it != myMap.end()) { std::cout << "Found element: " << it->first << " => " << it->second << std::endl; } else { std::cout << "Element not found" << std::endl; }
3. 迭代器操作
使用迭代器遍历set
:
for (auto it = mySet.begin(); it != mySet.end(); ++it) { std::cout << *it << " "; } std::cout << std::endl;
使用迭代器遍历map
:
for (auto it = myMap.begin(); it != myMap.end(); ++it) { std::cout << it->first << " => " << it->second << " "; } std::cout << std::endl;
表格展示常用操作及其效果
操作 | set | map |
插入元素 | mySet.insert(value); | myMap[key] = value; |
删除元素 | mySet.erase(value); | myMap.erase(key); |
查找元素 | auto it = mySet.find(value); | auto it = myMap.find(key); |
遍历容器 | for (auto it = mySet.begin(); it != mySet.end(); ++it) | for (auto it = myMap.begin(); it != myMap.end(); ++it) |
更新元素 | mySet.insert(new_value); | myMap[key] = new_value; |
获取元素个数 | mySet.size(); | myMap.size(); |
判断是否为空 | mySet.empty(); | myMap.empty(); |
相关问答FAQs
问:如何判断一个元素是否存在于set中?
答:可以使用find
方法来判断元素是否存在于set
中。
if (mySet.find(3) != mySet.end()) { std::cout << "Element exists in the set." << std::endl; } else { std::cout << "Element does not exist in the set." << std::endl; }
问:如何在map中根据键值对进行排序?
答:map
容器会自动根据键进行排序,如果需要自定义排序方式,可以使用std::map
的模板参数传入自定义的比较函数。
struct CustomCompare { bool operator()(const int& lhs, const int& rhs) const { return lhs > rhs; // 降序排序 } }; std::map<int, std::string, CustomCompare> myMap; myMap[1] = "one"; myMap[3] = "three"; // ...其他操作...
到此,以上就是小编对于“宿舍的英语单词是什么?(windows下stl.h)”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1347207.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复