Linux CAN总线详解
Linux系统中的CAN(Controller Area Network)是一种广泛用于自动控制、嵌入式设备和汽车领域的工业总线技术,它由德国BOSCH公司开发,并最终成为国际标准ISO 11898-1,在Linux系统中,CAN接口被当作网络设备进行统一管理,这使得CAN总线通信近似于以太网通信,应用程序开发接口更加通用和灵活,本文将详细介绍如何在Linux系统中配置和使用CAN总线接口,包括接口配置、应用程序开发以及常见问题解答。
一、Linux系统中CAN接口配置
在Linux系统中,CAN总线接口设备作为网络设备被系统进行统一管理,可以使用与以太网类似的命令来配置CAN总线,使用ifconfig
命令可以查看当前系统中的所有网络设备,包括CAN总线接口(如can0和can1)。
ifconfig -a
要设置CAN总线的位速率,可以使用ip
命令。
sudo ip link set can0 type can bitrate 500000
这条命令将can0设备的位速率设置为500 kbps,完成设置后,可以通过以下命令使能或禁用can0设备:
sudo ifconfig can0 up # 使能can0设备 sudo ifconfig can0 down # 禁用can0设备
二、Linux系统中CAN接口应用程序开发
初始化SocketCAN套接字
SocketCAN是Linux提供的一套用于实现CAN应用开发的套接字接口,首先需要创建一个套接字并将其绑定到指定的CAN接口,以下是一个简单的示例代码:
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <sys/socket.h> #include <linux/can.h> #include <linux/can/raw.h> #include <net/if.h> #include <sys/ioctl.h> int main() { int s; struct sockaddr_can addr; struct ifreq ifr; // 创建SocketCAN套接字 s = socket(PF_CAN, SOCK_RAW, CAN_RAW); if (s < 0) { perror("Error while opening socket"); return -1; } // 指定CAN接口 strcpy(ifr.ifr_name, "can0" ); ioctl(s, SIOCGIFINDEX, &ifr); addr.can_family = AF_CAN; addr.can_ifindex = ifr.ifr_ifindex; // 绑定套接字到指定的CAN接口 if (bind(s, (struct sockaddr *)&addr, sizeof(addr)) < 0) { perror("Error in socket bind"); return -2; } printf("Socket bound to CAN interface "); return 0; }
数据发送和接收
发送和接收数据时,需要构造can_frame
结构体,以下是发送和接收数据的示例代码:
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <sys/socket.h> #include <linux/can.h> #include <linux/can/raw.h> #include <net/if.h> #include <sys/ioctl.h> int main() { int s; struct sockaddr_can addr; struct ifreq ifr; struct can_frame frame; int nbytes; // 创建SocketCAN套接字并绑定到指定的CAN接口(同上) // ... // 构造一个数据帧 frame.can_id = 0x123; // 标识符为0x123的标准帧 frame.can_dlc = 1; // 数据长度为1字节 frame.data[0] = 0xAB; // 数据内容为0xAB // 发送数据帧 nbytes = write(s, &frame, sizeof(struct can_frame)); if (nbytes != sizeof(struct can_frame)) { fprintf(stderr, "Failed to write frame "); } else { printf("Frame sent "); } // 接收数据帧 nbytes = read(s, &frame, sizeof(struct can_frame)); if (nbytes > 0) { printf("Received a frame with ID=%X DLC=%d Data=%.*s ", frame.can_id, frame.can_dlc, frame.can_dlc, frame.data); } else { fprintf(stderr, "Failed to read frame "); } close(s); return 0; }
错误处理和过滤规则设置
当接收到帧后,可以通过判断can_id
中的CAN_ERR_FLAG
位来判断是否为错误帧,如果是错误帧,可以通过其他符号位来判断错误的具体原因,还可以设置过滤规则,只接收符合规则的报文,以下是一个简单的过滤规则设置示例:
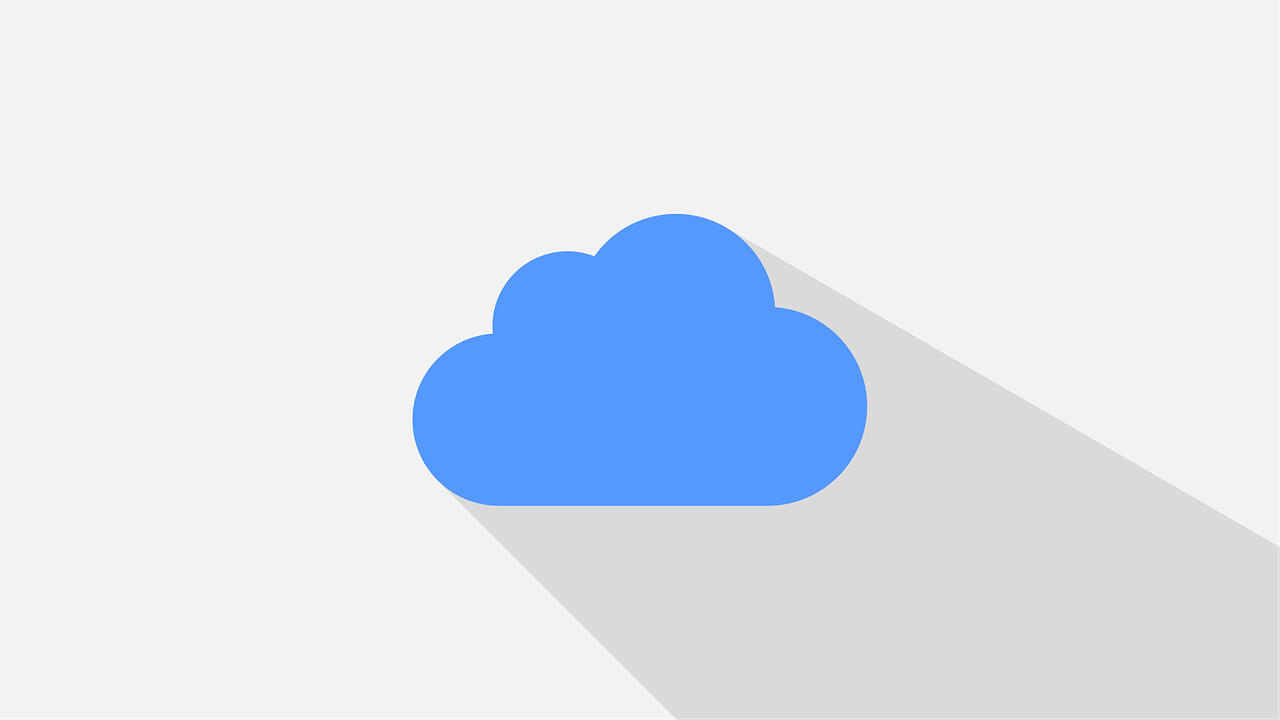
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <sys/socket.h> #include <linux/can.h> #include <linux/can/raw.h> #include <net/if.h> #include <sys/ioctl.h> int main() { int s; struct sockaddr_can addr; struct ifreq ifr; struct can_frame frame; struct can_filter rfilter[1]; int nbytes; // 创建SocketCAN套接字并绑定到指定的CAN接口(同上) // ... // 设置过滤规则,只接收标识符为0x123的帧 rfilter[0].can_id = 0x123; rfilter[0].can_mask = CAN_SFF_MASK; setsockopt(s, SOL_CAN_RAW, CAN_RAW_FILTER, rfilter, sizeof(rfilter)); // 接收数据帧(只会接收符合条件的帧) nbytes = read(s, &frame, sizeof(struct can_frame)); if (nbytes > 0) { printf("Filtered frame received with ID=%X DLC=%d Data=%.*s ", frame.can_id, frame.can_dlc, frame.can_dlc, frame.data); } else { fprintf(stderr, "Failed to read frame or no matching frame received "); } close(s); return 0; }
三、常见问题解答(FAQs)
Q1: 如何更改CAN总线的位速率?
A1: 可以使用ip
命令来更改CAN总线的位速率,要将can0设备的位速率设置为500 kbps,可以使用以下命令:
sudo ip link set can0 type can bitrate 500000
Q2: 如何判断接收到的帧是否为错误帧?
A2: 可以通过检查can_frame
结构体中的can_id
字段来判断,如果can_id
的CAN_ERR_FLAG
位被置位,则表示该帧为错误帧,可以通过以下方式判断:
if (frame.can_id & CAN_ERR_FLAG) { printf("Received an error frame "); } else { printf("Received a normal frame "); }
以上内容就是解答有关“linux can总线”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1340047.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复