在当今的Web开发领域,动态网页的需求日益增加,ASP(Active Server Pages)作为一种服务器端脚本环境,广泛用于构建交互式和数据驱动的Web应用程序,日期日历功能是许多Web应用中常见的组件之一,它允许用户选择日期,进行日期范围的选择等操作,本文将详细介绍如何在ASP中实现一个功能齐全的日期日历组件,并提供相关代码示例和常见问题解答。
一、ASP简介
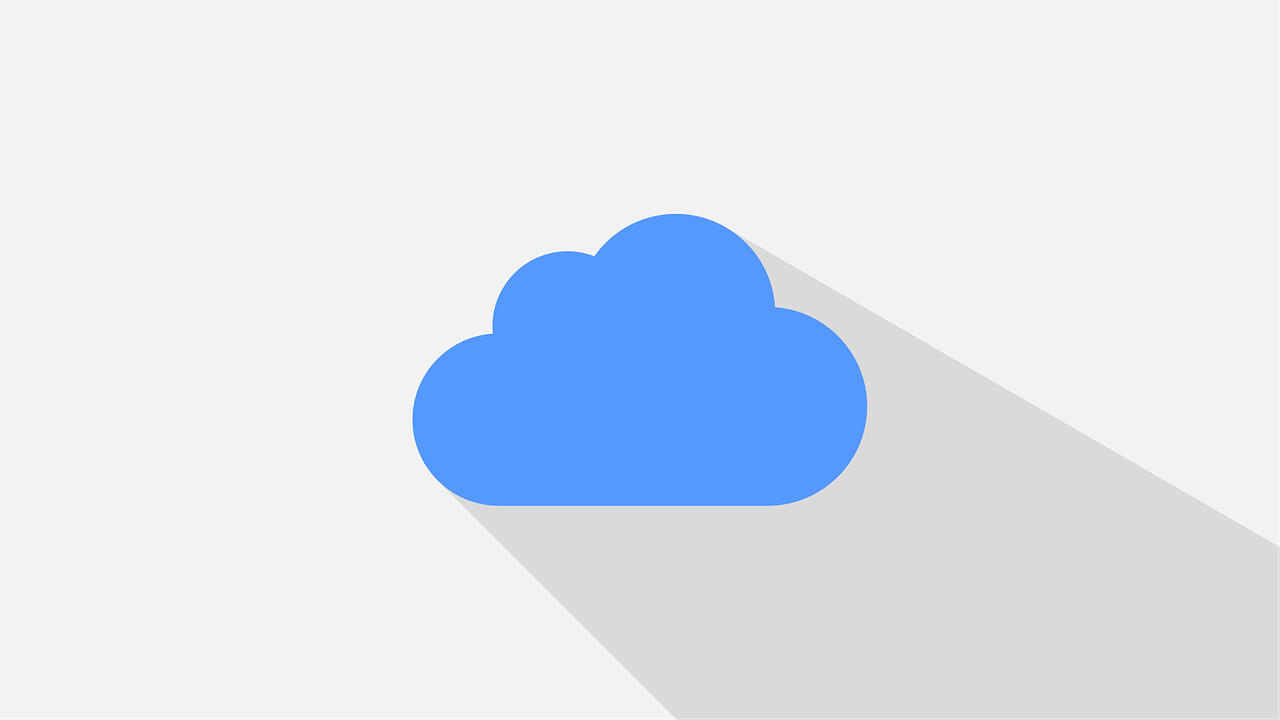
ASP是一种由微软开发的服务器端脚本技术,用于创建动态网页,ASP文件通常包含HTML标记、CSS样式、JavaScript脚本以及ASP特定的脚本标记(<% … %>),当用户请求一个ASP页面时,服务器会执行其中的脚本,生成动态内容并将其发送到客户端浏览器。
二、日期日历的基本需求
在实现日期日历功能之前,我们需要明确一些基本需求:
1、显示当前月份的日历:包括所有天数和对应的星期几。
2、支持导航:用户可以点击箭头按钮或年份/月份下拉菜单来切换到其他月份或年份。
3、选择日期:用户可以点击某一天来选择该日期。
4、高亮显示选中的日期:被选中的日期应该以不同的颜色或样式显示。
5、支持禁用特定日期:例如周末或特定的节假日。
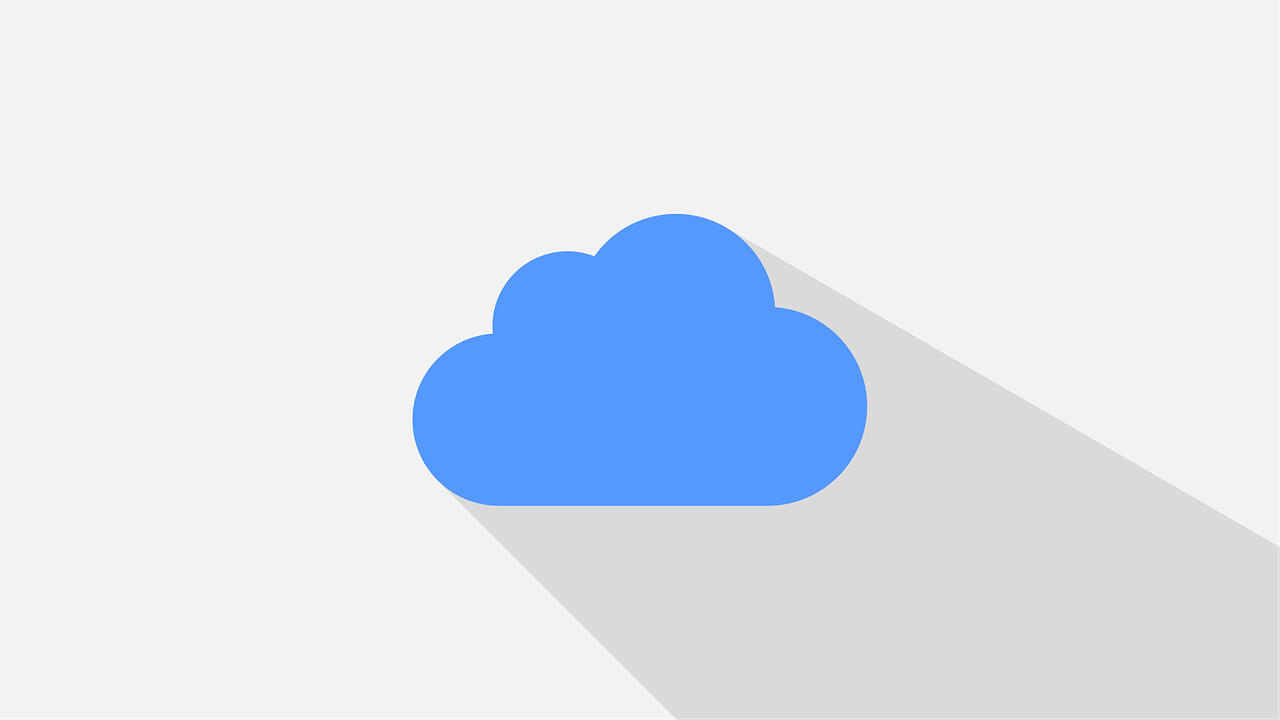
6、响应式设计:确保在不同设备上都能良好显示。
三、实现步骤
1. 创建基本的HTML结构
我们需要创建一个基本的HTML结构来容纳我们的日期日历组件,以下是一个简单的例子:
<!DOCTYPE html> <html lang="zh-CN"> <head> <meta charset="UTF-8"> <title>日期日历</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div id="calendar-container"></div> <script src="calendar.js"></script> </body> </html>
2. 使用CSS进行样式设置
我们为日期日历添加一些基本的样式,在styles.css
文件中,我们可以定义如下样式:
#calendar-container { width: 300px; margin: 0 auto; font-family: Arial, sans-serif; } .calendar-header { display: flex; justify-content: space-between; align-items: center; padding: 10px; background-color: #f0f0f0; } .calendar-header button { background: none; border: none; cursor: pointer; font-size: 20px; } .calendar-days, .calendar-dates { display: grid; grid-template-columns: repeat(7, 1fr); gap: 1px; } .calendar-days div, .calendar-dates div { padding: 10px; text-align: center; background-color: #fff; } .calendar-dates div { cursor: pointer; } .calendar-dates div.selected { background-color: #007bff; color: white; }
3. 编写JavaScript逻辑
我们来实现日期日历的核心逻辑,在calendar.js
文件中,我们将编写以下代码:
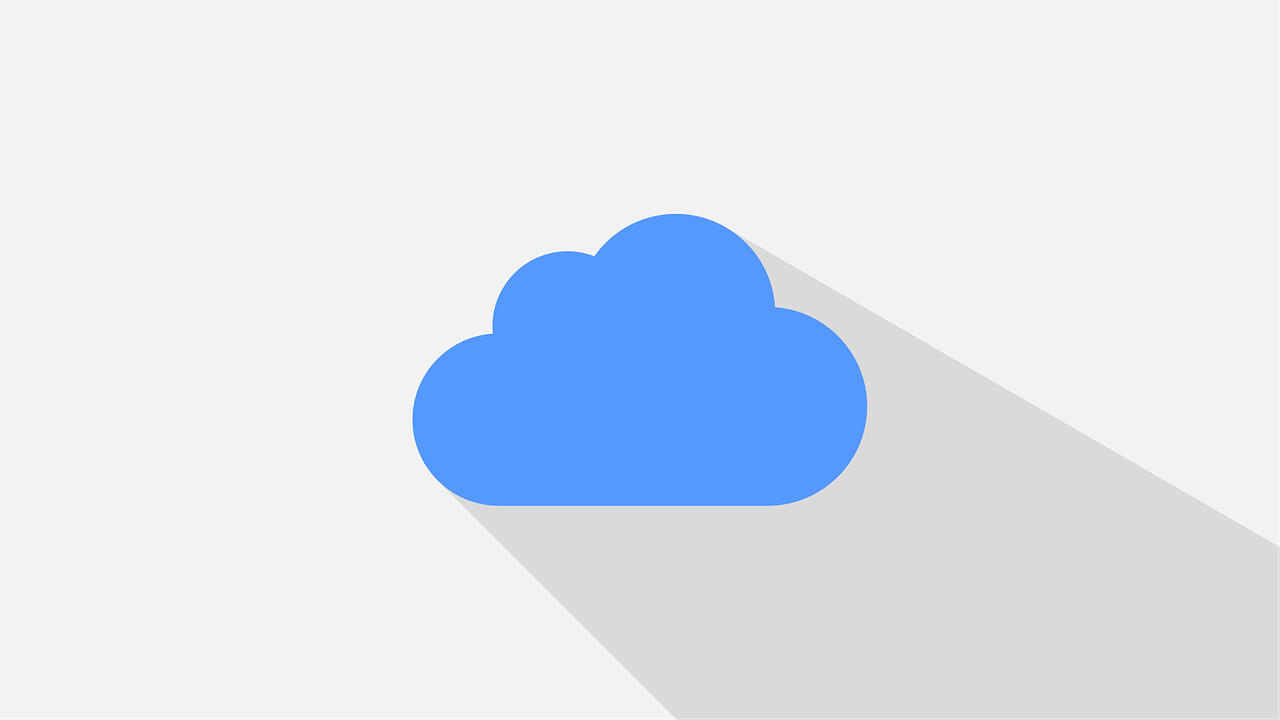
document.addEventListener('DOMContentLoaded', function() {
const calendarContainer = document.getElementById('calendar-container');
const currentDate = new Date();
renderCalendar(currentDate, calendarContainer);
});
function renderCalendar(date, container) {
container.innerHTML = ''; // 清空容器内容
const header = document.createElement('div');
header.className = 'calendar-header';
header.innerHTML = `
<button onclick="prevMonth()"><</button>
${date.toLocaleDateString('zh-CN', { year: 'numeric', month: 'long' })}
<button onclick="nextMonth()">></button>
`;
container.appendChild(header);
const daysOfWeek = ['日', '一', '二', '三', '四', '五', '六'];
const daysContainer = document.createElement('div');
daysContainer.className = 'calendar-days';
daysOfWeek.forEach(day => {
const dayElement = document.createElement('div');
dayElement.textContent = day;
daysContainer.appendChild(dayElement);
});
container.appendChild(daysContainer);
const datesContainer = document.createElement('div');
datesContainer.className = 'calendar-dates';
const firstDayOfMonth = new Date(date.getFullYear(), date.getMonth(), 1);
const lastDayOfMonth = new Date(date.getFullYear(), date.getMonth() + 1, 0);
const startDay = firstDayOfMonth.getDay();
let dateIndex = 1 startDay; // 调整到本月第一天的位置
while (dateIndex <= lastDayOfMonth.getDate()) {
const dayElement = document.createElement('div');
dayElement.textContent = dateIndex;
dayElement.onclick = () => selectDate(new Date(date.getFullYear(), date.getMonth(), dateIndex));
if (dateIndex === 0 || dateIndex > lastDayOfMonth.getDate()) {
dayElement.style.visibility = 'hidden'; // 隐藏非本月的日期
} else {
dayElement.classList.add('available'); // 本月日期可点击
}
datesContainer.appendChild(dayElement);
dateIndex++;
}
container.appendChild(dateContainer);
}
function prevMonth() {
const currentDate = new Date();
const newDate = new Date(currentDate.getFullYear(), currentDate.getMonth() 1);
renderCalendar(newDate, document.getElementById('calendar-container'));
}
function nextMonth() {
const currentDate = new Date();
const newDate = new Date(currentDate.getFullYear(), currentDate.getMonth() + 1);
renderCalendar(newDate, document.getElementById('calendar-container'));
}
function selectDate(date) {
// 在这里处理日期选择的逻辑,例如保存到数据库或更新UI
alert(Selected date: ${date.toLocaleDateString('zh-CN')}
);
}
4. 测试和调试
保存所有文件后,打开浏览器查看效果,你应该能够看到一个基本的日期日历组件,可以点击箭头按钮切换月份,并选择日期,如果有任何问题,请检查控制台日志以获取错误信息,并进行相应的调试。
四、常见问题解答(FAQs)
Q1: 如何使日期日历组件支持多语言?
A1: 要使日期日历组件支持多语言,可以在JavaScript中使用国际化API(Intl)来格式化日期和星期几的名称,可以使用Intl.DateTimeFormat
对象来获取本地化的星期几名称,还可以通过传递语言参数来动态切换语言。
Q2: 如何禁用周末或特定日期?
A2: 要在日期日历中禁用周末或特定日期,可以在渲染日期时添加条件判断,可以在循环中检查当前日期是否为周末,如果是则跳过该日期或将其设置为不可点击状态,同样地,可以将特定日期存储在一个数组中,并在渲染时检查当前日期是否在该数组中,如果是则禁用该日期,具体实现可以参考以下代码片段:
const disabledDates = [/* 特定日期数组 */]; ... while (dateIndex <= lastDayOfMonth.getDate()) { const dayElement = document.createElement('div'); dayElement.textContent = dateIndex; dayElement.onclick = () => selectDate(new Date(date.getFullYear(), date.getMonth(), dateIndex)); if (dateIndex === 0 || dateIndex > lastDayOfMonth.getDate() || disabledDates.includes(new Date(date.getFullYear(), date.getMonth(), dateIndex).toISOString().split('T')[0])) { dayElement.style.visibility = 'hidden'; // 隐藏非本月的日期或禁用特定日期 } else { dayElement.classList.add('available'); // 本月日期可点击 } datesContainer.appendChild(dayElement); dateIndex++; }
通过以上步骤和示例代码,你可以在ASP项目中实现一个功能齐全的日期日历组件,根据实际需求,你可以进一步扩展和优化这个组件,例如添加更多的样式、支持更多的功能(如选择日期范围)等,希望这篇文章对你有所帮助!
以上内容就是解答有关“asp 日期日历”的详细内容了,我相信这篇文章可以为您解决一些疑惑,有任何问题欢迎留言反馈,谢谢阅读。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1339142.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复