MapReduce是一种用于处理和生成大规模数据集的编程模型,它通过将任务分解成更小的部分来并行处理数据,从而实现高效的计算,以下是关于MapReduce的详细介绍:
MapReduce的核心概念
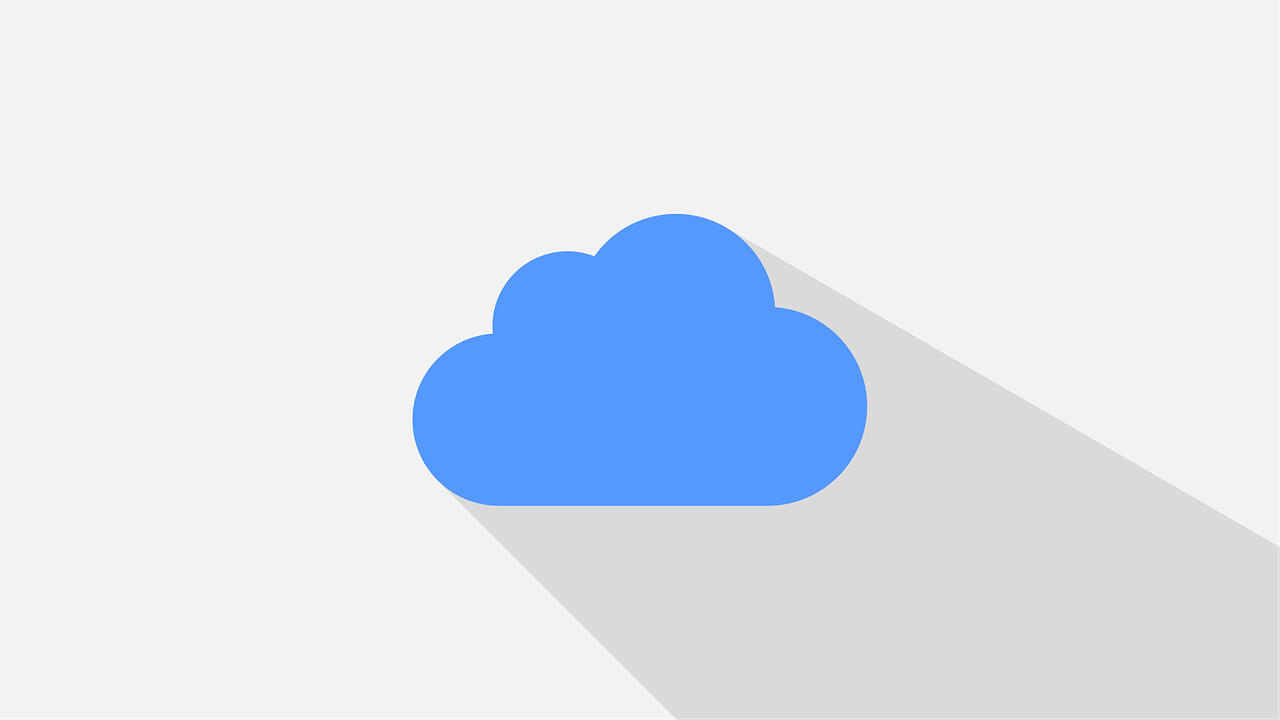
1、Map(映射)阶段:在这个阶段,输入数据被分成多个小块,并由多个map任务并行处理,每个map任务会将输入数据转换为一系列的中间键值对。
2、Shuffle and Sort(洗牌和排序)阶段:在map阶段产生的中间键值对会被按键进行分组,并将相同键的所有值收集在一起,这个过程通常伴随着数据的排序。
3、Reduce(归约)阶段:在这个阶段,每个reduce任务会对分组后的中间键值对进行处理,并生成最终的输出结果。
MapReduce实例:Word Count
一个经典的MapReduce实例是单词计数(Word Count),其目标是统计文本文件中每个单词出现的次数,以下是一个使用Java编写的简单Word Count程序示例:
import java.io.IOException; import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.LongWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.Mapper; import org.apache.hadoop.mapreduce.Reducer; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; public class WordCount { public static class TokenizerMapper extends Mapper<LongWritable, Text, Text, IntWritable> { private final static IntWritable one = new IntWritable(1); private Text word = new Text(); public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { String[] words = value.toString().split("\s+"); for (String str : words) { word.set(str); context.write(word, one); } } } public static class IntSumReducer extends Reducer<Text, IntWritable, Text, IntWritable> { public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } context.write(key, new IntWritable(sum)); } } public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCount.class); job.setMapperClass(TokenizerMapper.class); job.setCombinerClass(IntSumReducer.class); job.setReducerClass(IntSumReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
在这个例子中,TokenizerMapper
类负责将输入文本拆分成单词,并为每个单词生成一个键值对(单词,1)。IntSumReducer
类则负责将所有相同单词的值相加,从而得到每个单词的总计数。
MapReduce的优势与应用场景
MapReduce的优势在于它能够处理大规模数据集,并且具有良好的扩展性和容错性,它适用于以下场景:
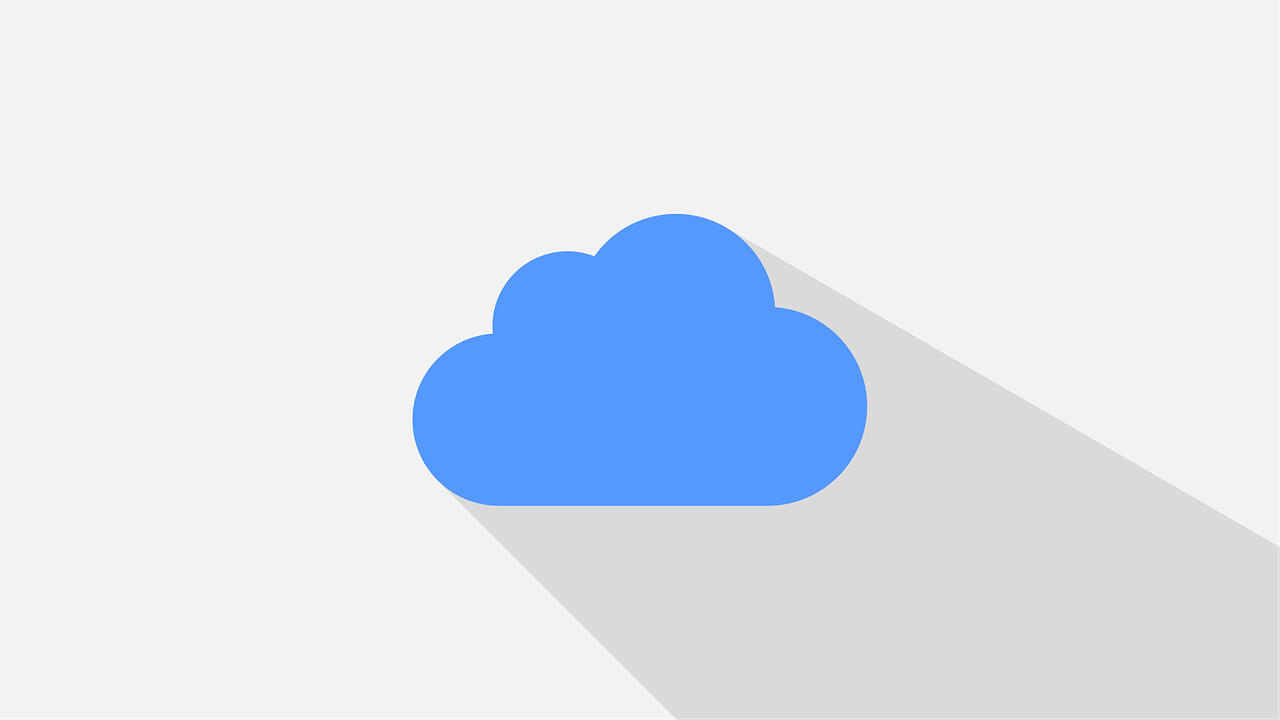
日志分析:可以用于分析大规模的日志文件,提取有用的信息。
数据挖掘:可以从大量数据中发现模式和趋势。
索引构建:可以用于构建搜索引擎的索引。
机器学习:可以用于训练大规模的机器学习模型。
常见问题解答(FAQs)
Q1: MapReduce中的map和reduce函数分别完成什么任务?
A1: Map函数负责将输入数据转换为一系列的中间键值对,而reduce函数则负责将这些中间键值对归并为最终的输出结果,map函数处理的是原始输入数据,而reduce函数处理的是由map函数生成的中间键值对。
Q2: MapReduce是如何实现并行处理的?
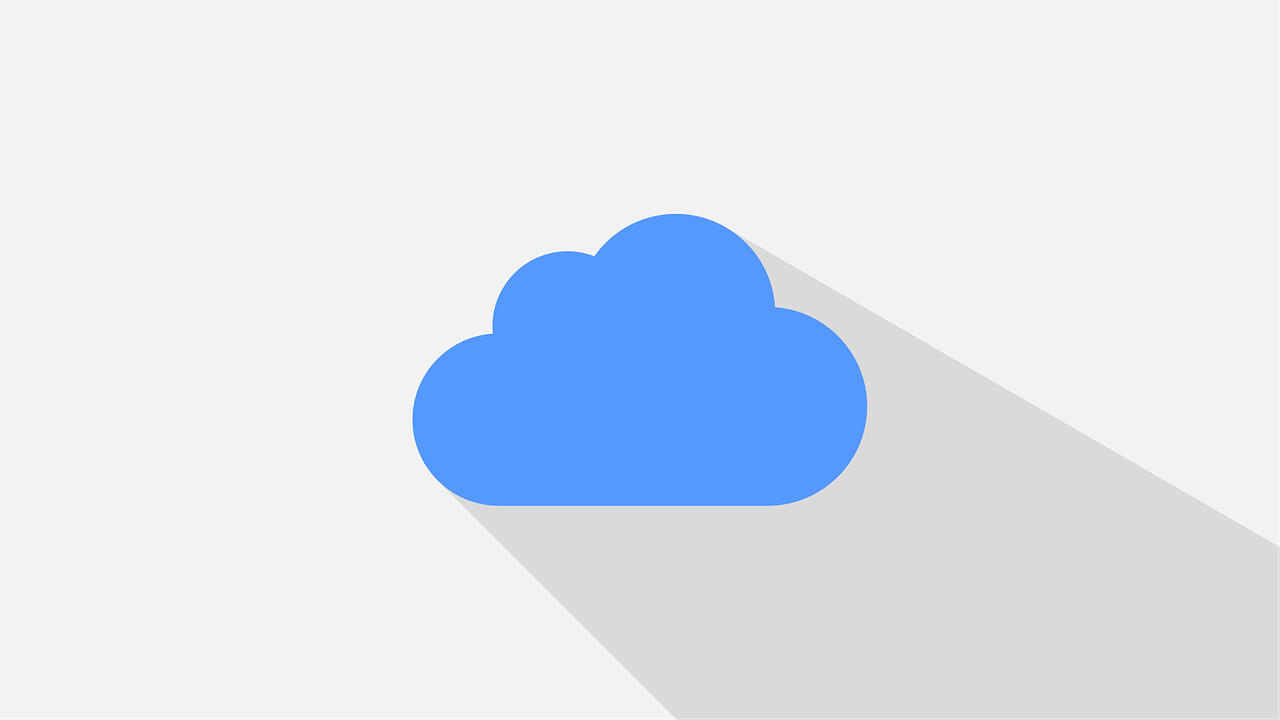
A2: MapReduce通过将输入数据分成多个小块,并为每个小块分配一个map任务来实现并行处理,这些map任务可以在不同的节点上并行执行,从而提高了处理效率,在shuffle and sort阶段,系统会对map任务的输出进行排序和分组,为reduce任务的执行做准备,reduce任务会对分组后的数据进行处理,生成最终的输出结果,整个过程中,MapReduce框架会自动处理数据的分发、任务调度和容错等问题。
到此,以上就是小编对于“mapreduce的实例_MapReduce”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1338435.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复