在当今大数据时代,MapReduce编程已经成为处理大规模数据的重要工具,本文将通过一个具体的编程实例,详细介绍MapReduce的工作原理及其应用,帮助读者更好地理解和掌握这一技术。
一、MapReduce简介
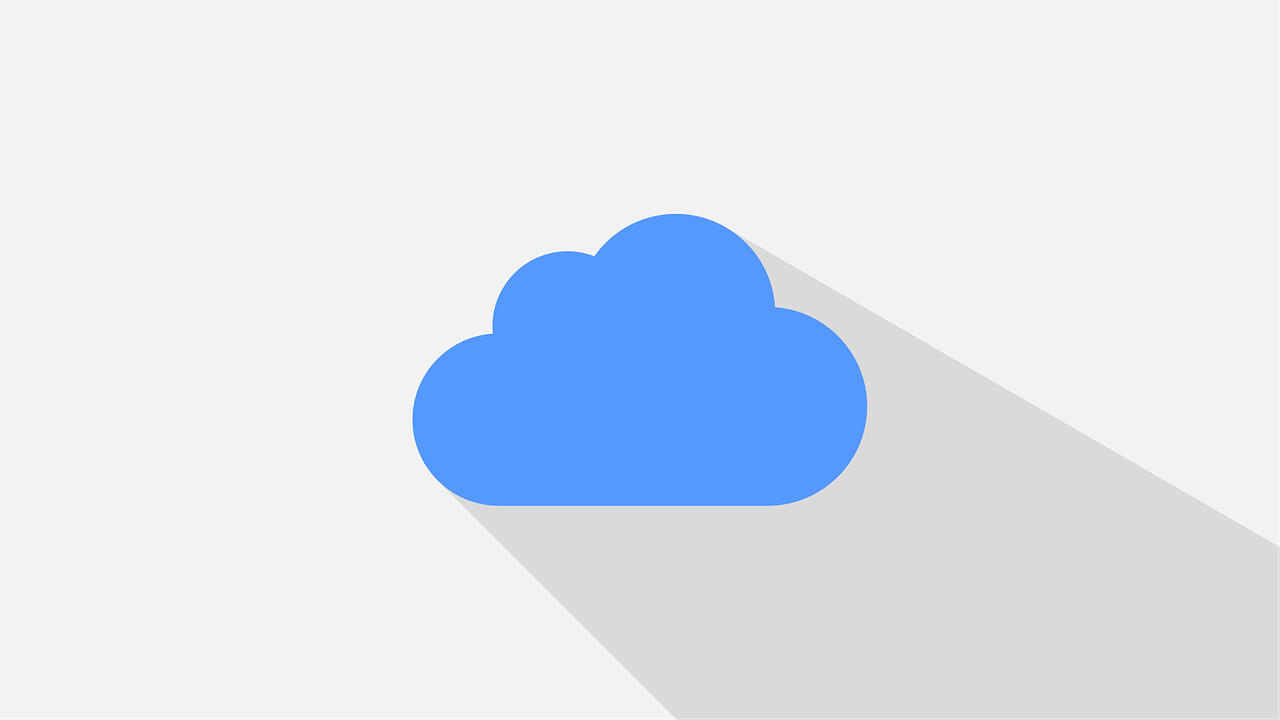
MapReduce是一种用于大规模数据处理的编程模型,由Google提出并广泛应用于大数据分析领域,它主要包括两个步骤:Map(映射)和Reduce(归约),在Map阶段,输入数据被分割成小块,并由多个Mapper并行处理;在Reduce阶段,Mapper输出的中间结果被合并,并由单个或多个Reducer进一步处理,生成最终结果。
二、MapReduce编程实例
为了更好地理解MapReduce的工作原理,我们以一个简单的词频统计为例进行说明,假设我们有一篇英文文章,需要统计每个单词出现的次数。
1. 环境准备
我们需要搭建Hadoop环境,Hadoop是一个开源的分布式计算框架,支持MapReduce编程,可以从Apache Hadoop官网下载并安装Hadoop,然后配置相关环境变量。
2. 编写Mapper类
Mapper类负责处理输入数据,并将结果传递给Reducer,在这个例子中,Mapper的任务是将文章中的每个单词映射为一个键值对,其中键是单词,值是1。
import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.LongWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Mapper; import java.io.IOException; public class WordCountMapper extends Mapper<LongWritable, Text, Text, IntWritable> { private final static IntWritable one = new IntWritable(1); private Text word = new Text(); @Override protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { String[] words = value.toString().split("\s+"); for (String w : words) { word.set(w); context.write(word, one); } } }
3. 编写Reducer类
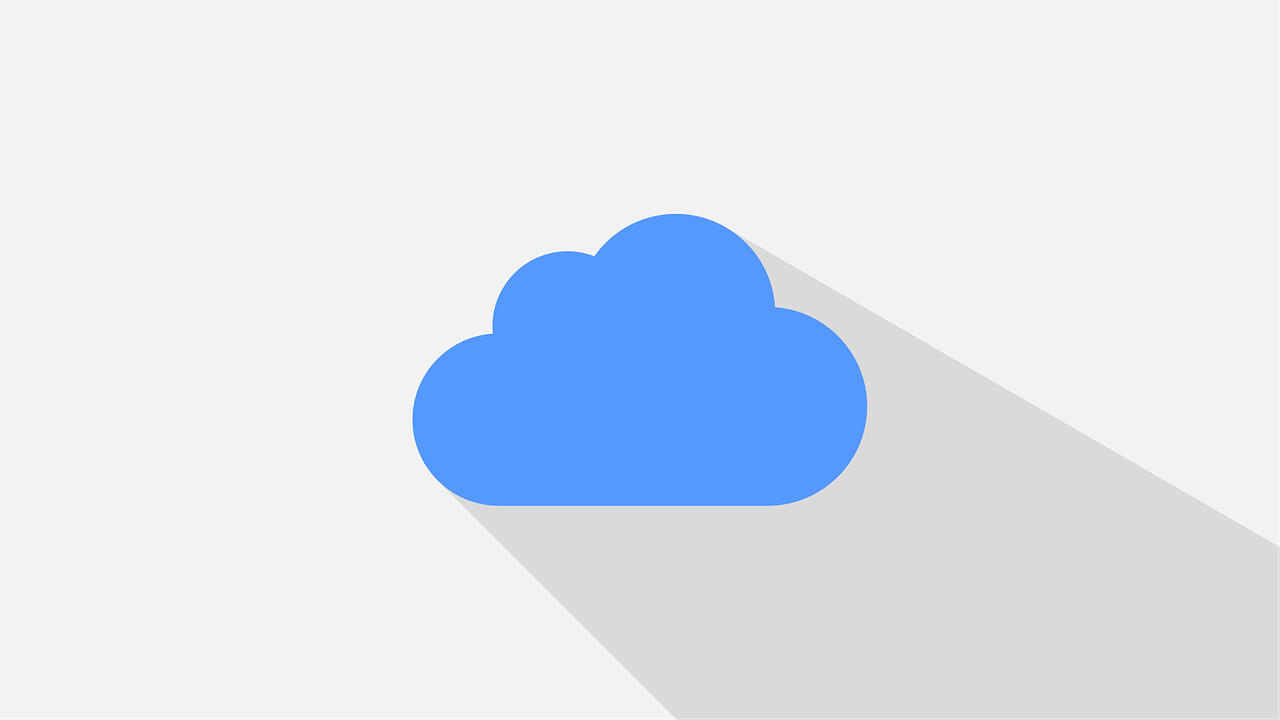
Reducer类负责接收Mapper的输出,并进行汇总处理,在这个例子中,Reducer的任务是将相同单词的计数相加,得到每个单词的总次数。
import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Reducer; import java.io.IOException; public class WordCountReducer extends Reducer<Text, IntWritable, Text, IntWritable> { @Override protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } context.write(key, new IntWritable(sum)); } }
4. 编写主程序
主程序负责设置作业参数,指定Mapper和Reducer类,并启动作业。
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; public class WordCount { public static void main(String[] args) throws Exception { if (args.length != 2) { System.err.println("Usage: WordCount <input path> <output path>"); System.exit(-1); } Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCount.class); job.setMapperClass(WordCountMapper.class); job.setCombinerClass(WordCountReducer.class); job.setReducerClass(WordCountReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
5. 运行程序
将上述代码编译打包,并上传到Hadoop集群,然后通过以下命令运行程序:
hadoop jar wordcount.jar WordCount /input/path /output/path
/input/path
是输入文件所在的目录,/output/path
是输出结果保存的目录。
三、结果分析
运行完成后,可以在Hadoop Web界面上查看作业的执行情况和输出结果,输出结果将包含每个单词及其出现的次数,格式如下:
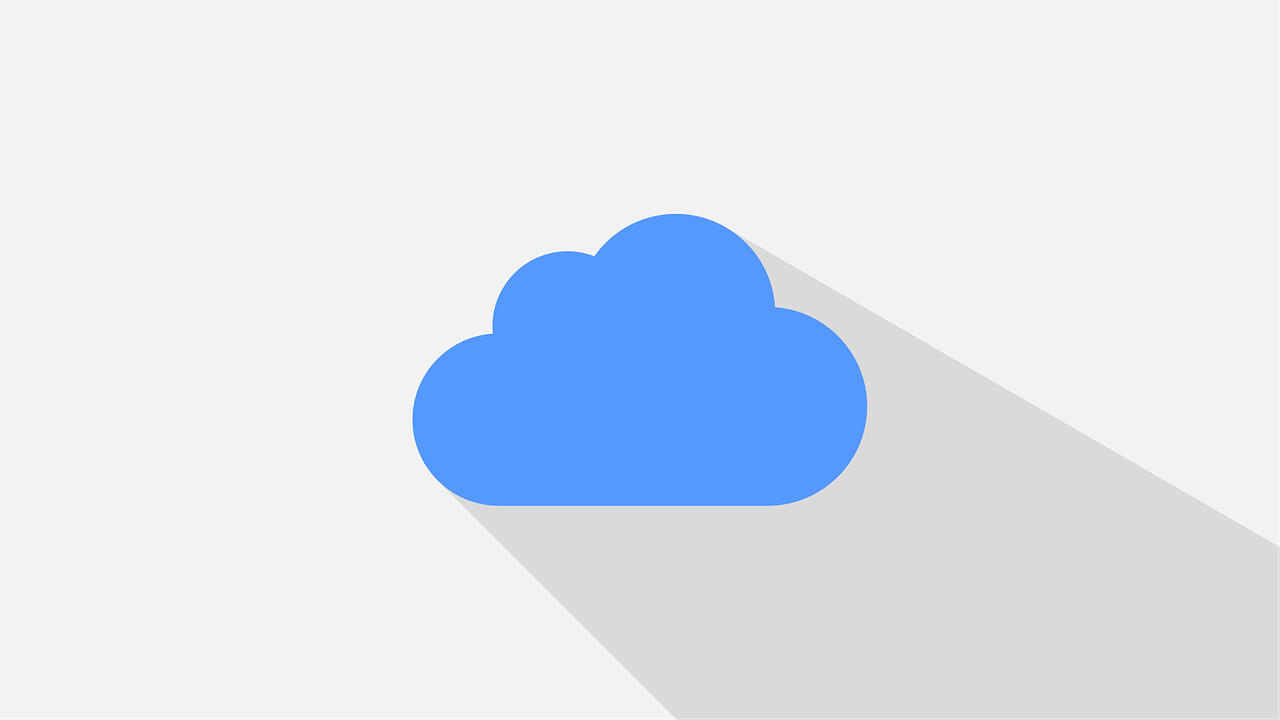
hello 1 world 1 this 1 is 1 a 1 test 1 ...
四、FAQs
Q1: MapReduce中的Combiner是什么?
A1: Combiner是MapReduce中的一个优化工具,用于在Mapper端进行局部汇总,减少传输到Reducer的数据量,在本例中,Combiner和Reducer使用相同的逻辑,即对相同单词的计数进行累加,这样可以显著提高作业的性能。
Q2: MapReduce如何处理数据倾斜问题?
A2: 数据倾斜是指在MapReduce作业中,某些Reducer接收到的数据量远大于其他Reducer,导致作业执行时间延长,为了解决数据倾斜问题,可以采取以下措施:
使用自定义分区器,确保数据均匀分布到各个Reducer。
增加Reducer的数量,分散处理负载。
对输入数据进行预处理,避免极端不均匀的数据分布。
通过以上内容,相信读者对MapReduce编程有了更深入的了解,MapReduce作为一种强大的数据处理工具,不仅适用于简单的词频统计,还可以扩展到更复杂的数据分析任务中,希望本文能为您的学习和工作带来帮助。
小伙伴们,上文介绍了“mapreduce编程_编程实例”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1335298.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复