MapReduce Java API接口介绍
在大数据领域,MapReduce是一种编程模型,用于处理大规模数据集,它由Google提出,并在Hadoop等开源项目中得到了广泛应用,MapReduce的核心思想是将任务分解为两个阶段:Map阶段和Reduce阶段,在Map阶段,输入数据被分割成小块,并由多个Mapper进行处理;在Reduce阶段,Mapper的输出被合并并进一步处理,本文将详细介绍MapReduce Java API接口,帮助开发者更好地理解和使用这个强大的工具。
MapReduce Java API
MapReduce Java API是Hadoop框架的一部分,提供了一组Java类和接口,用于编写MapReduce程序,通过这些API,开发者可以轻松地实现数据的映射和化简操作,从而完成复杂的数据处理任务。
关键组件
1、Job:表示一个完整的MapReduce作业,包括配置信息、Mapper类、Reducer类等。
2、Mapper:负责处理输入数据的映射操作,将输入数据转换为键值对。
3、Reducer:负责处理Mapper输出的化简操作,将相同键的值进行合并或计算。
4、InputFormat:定义了输入数据的格式和读取方式。
5、OutputFormat:定义了输出数据的格式和写入方式。
6、RecordReader:负责从输入源读取数据记录。
7、RecordWriter:负责将数据记录写入输出目标。
8、Partitioner:负责将Mapper输出的数据分配给不同的Reducer。
9、Shuffle and Sort:负责将Mapper输出的数据进行洗牌和排序,以便Reducer进行处理。
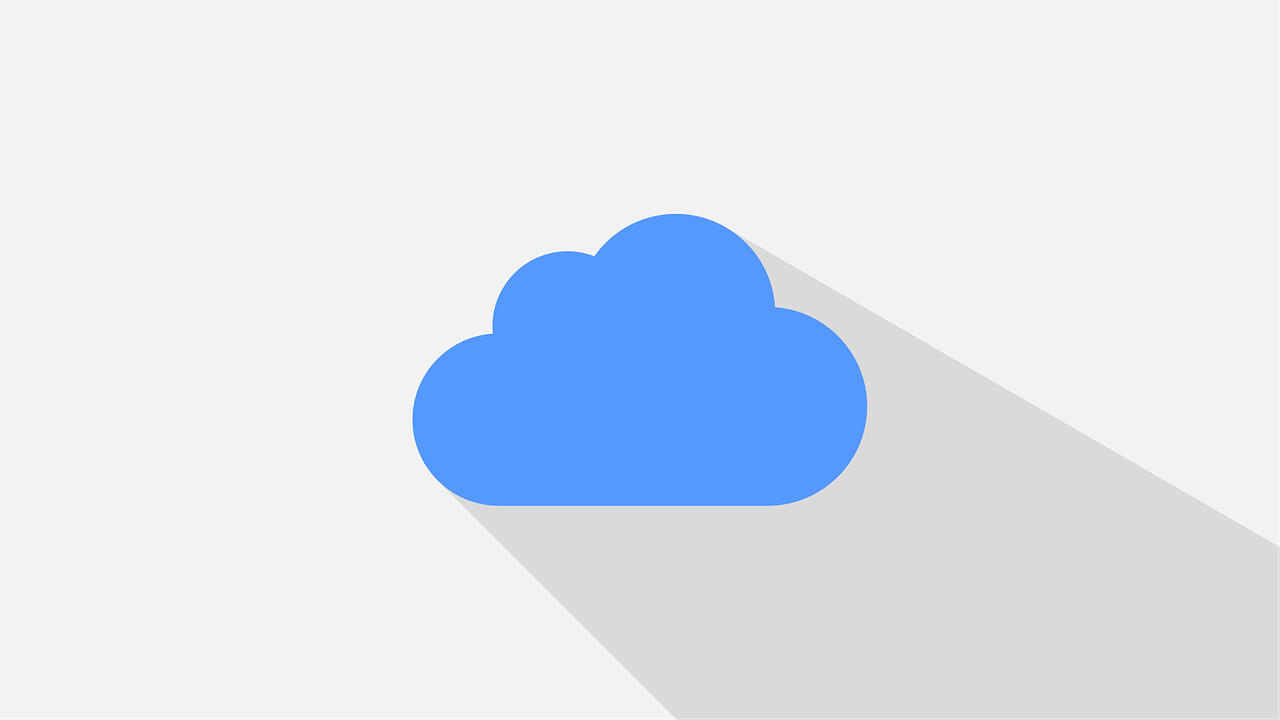
编程步骤
1、创建Job对象,设置作业名称、Jar包路径等信息。
2、配置Mapper类和Reducer类,实现具体的映射和化简逻辑。
3、配置InputFormat和OutputFormat,指定输入输出数据的格式和位置。
4、提交作业到Hadoop集群运行。
5、监控作业运行状态,等待作业完成。
6、获取作业结果,进行分析和展示。
示例代码
以下是一个简单的WordCount示例,展示了如何使用MapReduce Java API实现单词计数功能:
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.Mapper; import org.apache.hadoop.mapreduce.Reducer; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; import java.io.IOException; import java.util.StringTokenizer; public class WordCount { public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> { private final static IntWritable one = new IntWritable(1); private Text word = new Text(); public void map(Object key, Text value, Context context) throws IOException, InterruptedException { StringTokenizer itr = new StringTokenizer(value.toString()); while (itr.hasMoreTokens()) { word.set(itr.nextToken()); context.write(word, one); } } } public static class IntSumReducer extends Reducer<Text, IntWritable, Text, IntWritable> { private IntWritable result = new IntWritable(); public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } result.set(sum); context.write(key, result); } } public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCount.class); job.setMapperClass(TokenizerMapper.class); job.setCombinerClass(IntSumReducer.class); job.setReducerClass(IntSumReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
相关问答FAQs
Q1: MapReduce Java API与Hadoop Streaming有什么区别?
A1: MapReduce Java API是Hadoop框架的一部分,提供了一组Java类和接口,用于编写MapReduce程序,而Hadoop Streaming是一种通用的方法,可以使用任何编程语言来实现MapReduce程序,Streaming允许开发者使用标准输入输出来处理数据,而不需要关心底层的并行执行细节,Streaming更适合于那些不熟悉Java或者希望使用其他编程语言的开发者。
Q2: 如何在MapReduce作业中设置自定义的Partitioner?
A2: 要在MapReduce作业中设置自定义的Partitioner,需要在Job对象上调用setPartitionerClass()方法,并传入自定义Partitioner类的全限定名,job.setPartitionerClass(MyCustomPartitioner.class); 其中MyCustomPartitioner是一个实现了org.apache.hadoop.mapred.Partitioner接口的类,在这个类中,你需要实现getPartition()方法,根据业务需求将Mapper输出的数据分配给不同的Reducer。
小伙伴们,上文介绍了“mapreduce java 编程_MapReduce Java API接口介绍”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1334990.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复