在大数据领域,MapReduce 是一种编程模型和关联实现,用于处理和生成大规模数据集,编写 MapReduce 测试程序是确保数据处理逻辑正确性的关键步骤,本文将详细介绍如何编写一个有效的 MapReduce 测试程序,涵盖从环境搭建到具体测试用例的设计,并提供一些常见问题及其解答。
一、环境搭建
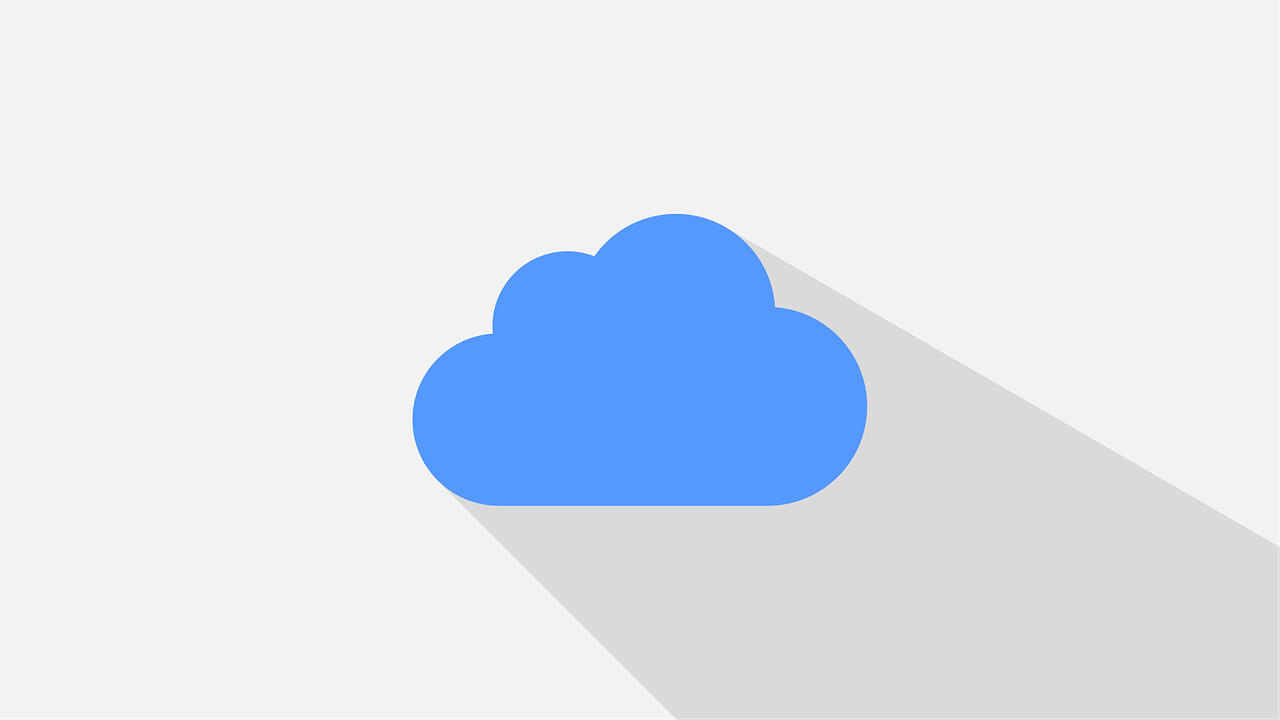
1、安装 Hadoop:
下载 Hadoop 二进制文件:https://hadoop.apache.org/releases.html
解压并配置环境变量:
tar -xzf hadoop-3.3.1.tar.gz export HADOOP_HOME=~/hadoop-3.3.1 export PATH=$HADOOP_HOME/bin:$PATH
2、启动 Hadoop 集群:
格式化 NameNode:
hdfs namenode -format
启动 HDFS:
start-dfs.sh
启动 Yarn:
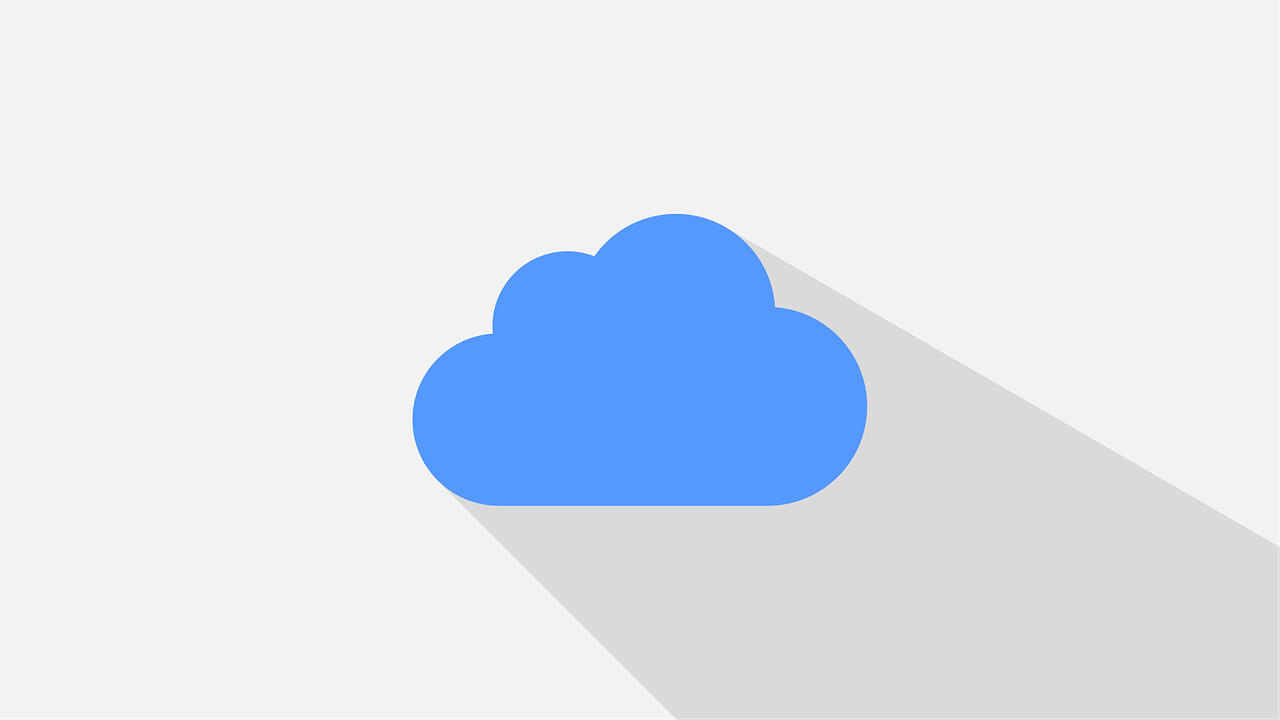
start-yarn.sh
二、编写 MapReduce 程序
1、创建 Maven 项目:
使用 Apache Maven 创建一个新的 Java 项目。
添加 Hadoop 依赖项到pom.xml
文件中:
<dependency> <groupId>org.apache.hadoop</groupId> <artifactId>hadoop-common</artifactId> <version>3.3.1</version> </dependency> <dependency> <groupId>org.apache.hadoop</groupId> <artifactId>hadoop-mapreduce-client-core</artifactId> <version>3.3.1</version> </dependency>
2、编写 Mapper 类:
创建一个继承自Mapper
类的 Java 类。
重写map
方法以处理输入数据并生成键值对。
public class WordCountMapper extends Mapper<LongWritable, Text, Text, IntWritable> { private final static IntWritable one = new IntWritable(1); private Text word = new Text(); @Override protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { String[] words = value.toString().split("\s+"); for (String str : words) { if (str.length() > 0) { word.set(str); context.write(word, one); } } } }
3、编写 Reducer 类:
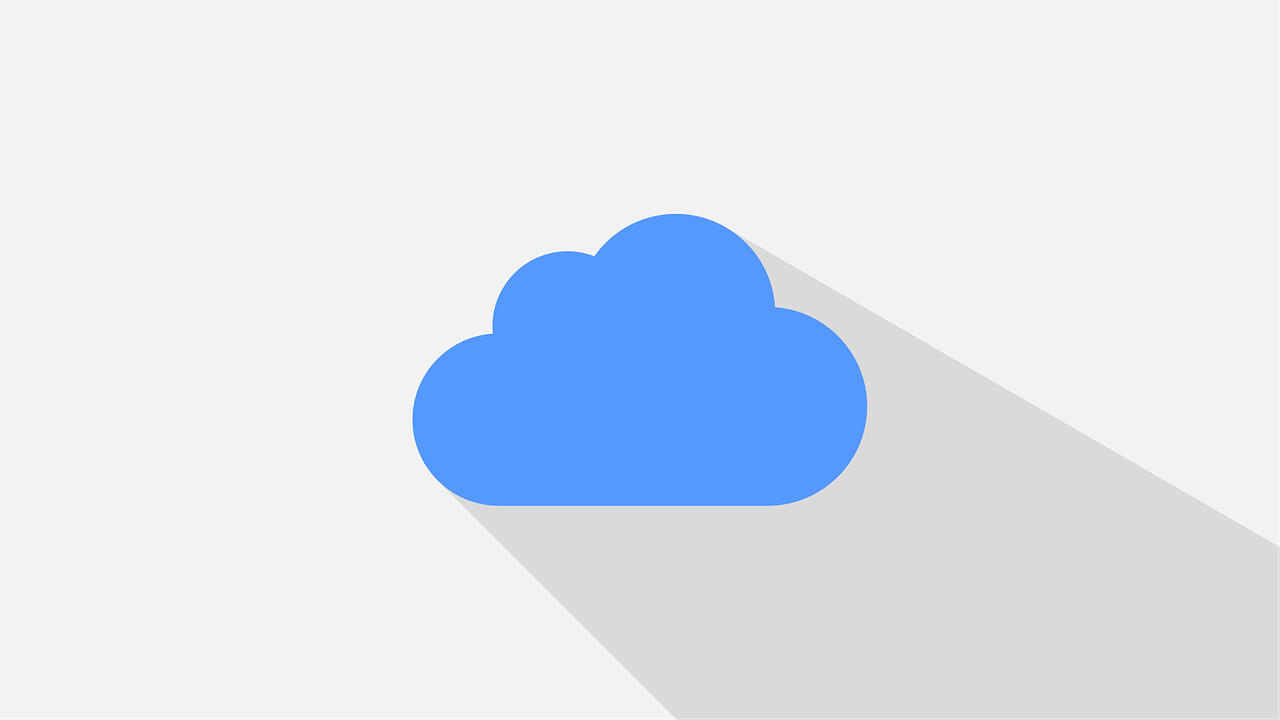
创建一个继承自Reducer
类的 Java 类。
重写reduce
方法以聚合键值对。
public class WordCountReducer extends Reducer<Text, IntWritable, Text, IntWritable> { @Override protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } context.write(key, new IntWritable(sum)); } }
4、编写驱动程序:
创建一个主类来运行 MapReduce 作业。
设置输入输出路径,并提交作业。
public class WordCountDriver { public static void main(String[] args) throws Exception { if (args.length != 2) { System.err.println("Usage: WordCount <input path> <output path>"); System.exit(-1); } Job job = Job.getInstance(new org.apache.hadoop.conf.Configuration()); job.setJarByClass(WordCountDriver.class); job.setMapperClass(WordCountMapper.class); job.setCombinerClass(WordCountReducer.class); job.setReducerClass(WordCountReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
三、编写测试程序
1、准备测试数据:
创建一个简单的文本文件作为输入数据,例如input.txt
如下:
Hello Hadoop MapReduce is powerful Let's learn MapReduce together
2、运行 MapReduce 作业:
使用以下命令打包并运行 MapReduce 作业:
mvn clean package hadoop jar target/wordcount-1.0-SNAPSHOT.jar input.txt output
3、验证输出结果:
检查输出目录中的文件,确保结果正确,输出应该是:
Let 1 Hadoop 1 Hello 1 MapReduce 1 is 1 Lets 1 learn 1 powerful 1 together 1
四、常见问题及解答(FAQs)
1、问题:如何调试 MapReduce 程序?
答:可以使用 Hadoop 的日志系统查看详细的错误信息,可以在本地模式下运行 MapReduce 作业进行调试,
hadoop jar target/wordcount-1.0-SNAPSHOT.jar input.txt output -local
2、问题:如何处理大数据集时的性能优化?
答:可以通过以下几种方式优化性能:
使用 Combiner 减少数据传输量。
调整并行度(mapred.reduce.tasks)。
优化数据序列化格式,如使用 Avro 或 Protocol Buffers。
确保硬件资源充足,包括 CPU、内存和磁盘 I/O。
到此,以上就是小编对于“mapreduce测试程序_编写测试程序”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1334228.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复