c,#include,#include,#include,#include,,int main() {, int serial_port = open("/dev/ttyS0", O_RDWR);, if (serial_port``
Linux 串口通信是嵌入式系统开发中的一个重要方面,广泛应用于设备间的数据交换,在 Linux 操作系统下,串口编程主要通过终端设备文件(如/dev/ttyS0
)进行操作,本文将详细介绍如何在 Linux 环境下进行串口编程,包括打开串口、配置串口参数、读写数据和关闭串口等步骤。
一、打开串口
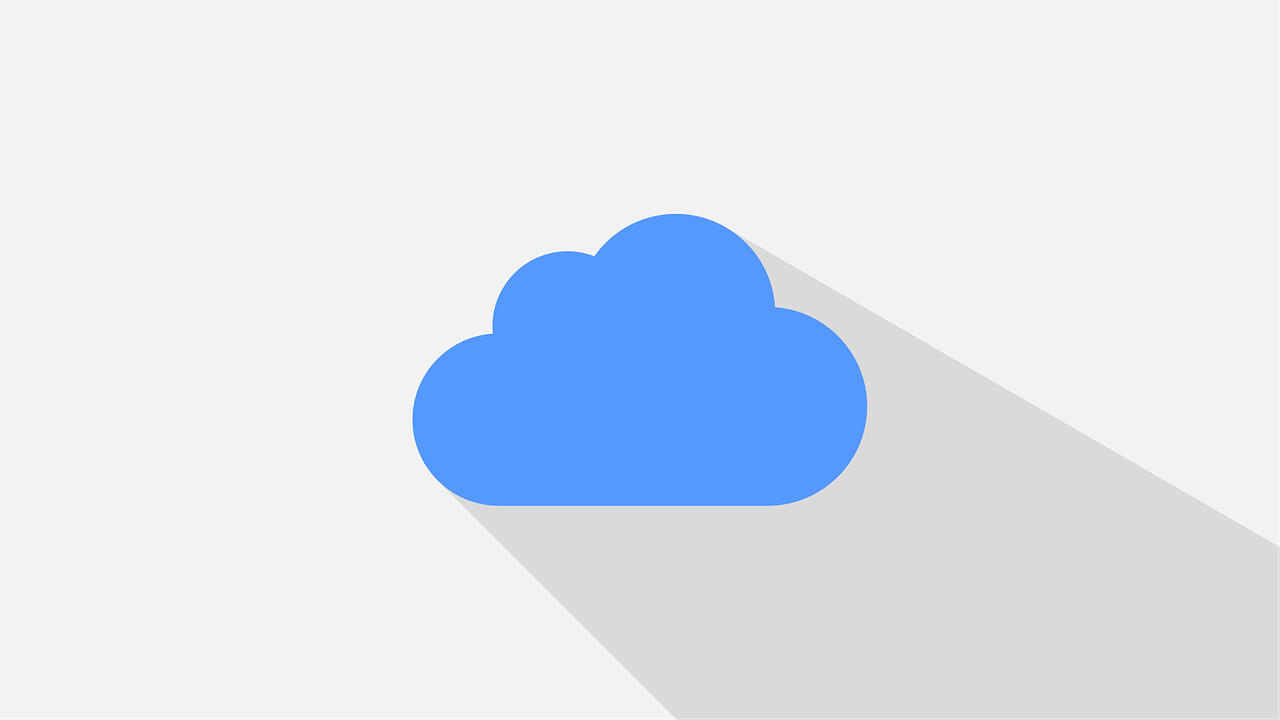
在 Linux 系统中,串口设备通常位于/dev
目录下,例如/dev/ttyS0
表示第一个串口,要打开串口,可以使用标准的 open() 函数:
#include <fcntl.h> #include <unistd.h> #include <stdio.h> #include <string.h> int main() { int serial_port = open("/dev/ttyS0", O_RDWR); if (serial_port < 0) { perror("Error opening serial port"); return -1; } // 后续代码... close(serial_port); return 0; }
二、配置串口参数
配置串口参数需要使用 termios 结构体,并通过 tcgetattr() 和 tcsetattr() 函数获取和设置串口属性,以下是一个例子:
#include <termios.h> struct termios options; // 获取当前串口属性 tcgetattr(serial_port, &options); // 设置波特率为 9600 cfsetispeed(&options, B9600); cfsetospeed(&options, B9600); // 设置字符大小为 8 位,停止位为 1 位,无奇偶校验 options.c_cflag &= ~PARENB; options.c_cflag &= ~CSTOPB; options.c_cflag &= ~CSIZE; options.c_cflag |= CS8; // 应用新的串口属性 tcsetattr(serial_port, TCSANOW, &options);
三、读写数据
读写串口数据可以使用 read() 和 write() 函数,以下是一个简单的例子:
char buffer[256]; int bytes_read = read(serial_port, buffer, sizeof(buffer)); if (bytes_read > 0) { printf("Read %d bytes: %s ", bytes_read, buffer); } else { perror("Error reading from serial port"); } const char *data = "Hello, serial port!"; int bytes_written = write(serial_port, data, strlen(data)); if (bytes_written > 0) { printf("Wrote %d bytes to serial port ", bytes_written); } else { perror("Error writing to serial port"); }
四、关闭串口
完成串口操作后,应该关闭串口以释放资源:
close(serial_port);
五、完整示例代码
以下是一个完整的 Linux 串口编程示例代码:
#include <fcntl.h> #include <unistd.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include <termios.h> int main() { int serial_port = open("/dev/ttyS0", O_RDWR); if (serial_port < 0) { perror("Error opening serial port"); return -1; } struct termios options; tcgetattr(serial_port, &options); cfsetispeed(&options, B9600); cfsetospeed(&options, B9600); options.c_cflag &= ~PARENB; options.c_cflag &= ~CSTOPB; options.c_cflag &= ~CSIZE; options.c_cflag |= CS8; tcsetattr(serial_port, TCSANOW, &options); char buffer[256]; int bytes_read = read(serial_port, buffer, sizeof(buffer)); if (bytes_read > 0) { printf("Read %d bytes: %s ", bytes_read, buffer); } else { perror("Error reading from serial port"); } const char *data = "Hello, serial port!"; int bytes_written = write(serial_port, data, strlen(data)); if (bytes_written > 0) { printf("Wrote %d bytes to serial port ", bytes_written); } else { perror("Error writing to serial port"); } close(serial_port); return 0; }
六、常见问题及解答 (FAQs)
Q1: 如何更改串口的波特率?
A1: 要更改串口的波特率,可以使用 cfsetispeed() 和 cfsetospeed() 函数来设置输入和输出波特率,将波特率设置为 115200:
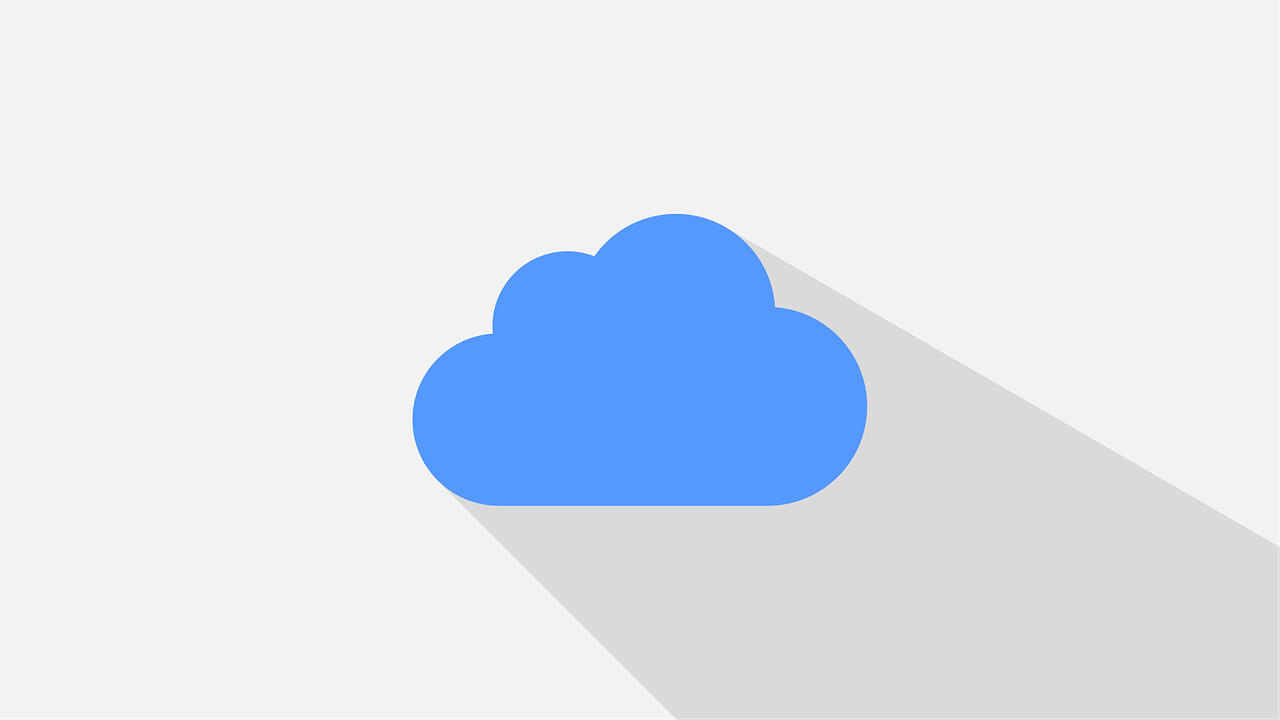
cfsetispeed(&options, B115200); cfsetospeed(&options, B115200);
然后使用 tcsetattr() 函数应用新的串口属性。
Q2: 如果串口设备文件不存在怎么办?
A2: 如果串口设备文件不存在,可能是因为串口设备没有正确初始化或者设备文件被误删除,可以尝试以下步骤:
1、确保串口设备已连接并且驱动程序已加载。
2、检查/dev
目录下是否有其他串口设备文件,例如/dev/ttyS1
。
3、如果设备文件丢失,可以尝试重新创建设备文件(需要管理员权限):
sudo mknod /dev/ttyS0 c 4 64
到此,以上就是小编对于“linux串口代码”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1332384.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复