在大数据时代,MapReduce作为一种高效的分布式计算模型,被广泛应用于各种数据处理任务中,单词统计是MapReduce的经典应用之一,本文将详细介绍如何使用MapReduce进行单词统计,并通过一个具体的样例程序来展示其实现过程。
MapReduce 概念简介
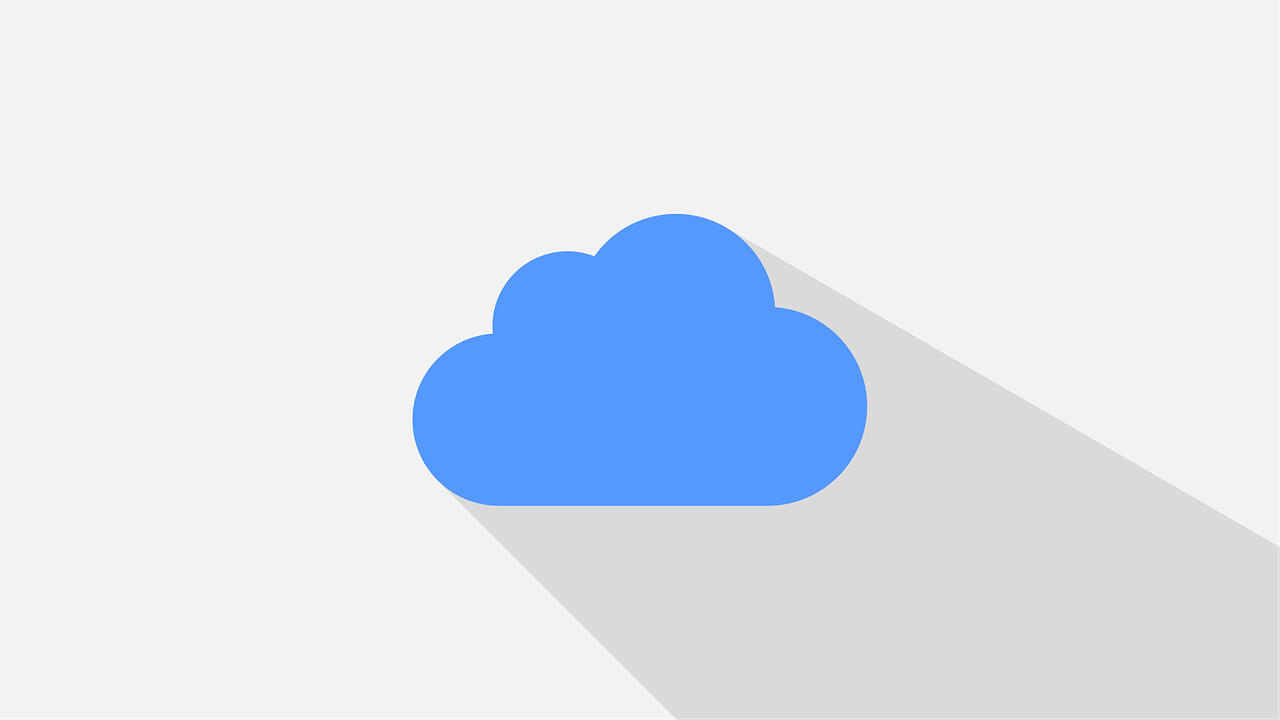
MapReduce是一种编程模型,用于处理和生成大规模数据集,它主要包括两个阶段:Map(映射)阶段和Reduce(归约)阶段。
Map阶段:输入数据被分割成独立的块,每个块由多个map任务并行处理,Map函数接收输入的键值对,并产生一系列的中间键值对。
Reduce阶段:所有具有相同键的中间键值对会被传递给同一个reduce任务,Reduce函数接收这些中间键值对,并进行合并操作,最终输出结果。
MapReduce 单词统计样例程序
假设我们有一个文本文件input.txt
如下:
Hello world Hello MapReduce MapReduce is powerful I love MapReduce
我们希望统计该文件中每个单词的出现次数,以下是使用MapReduce进行单词统计的步骤和代码示例。
1. 环境配置
需要确保已经安装了Hadoop,并且HDFS服务正在运行。
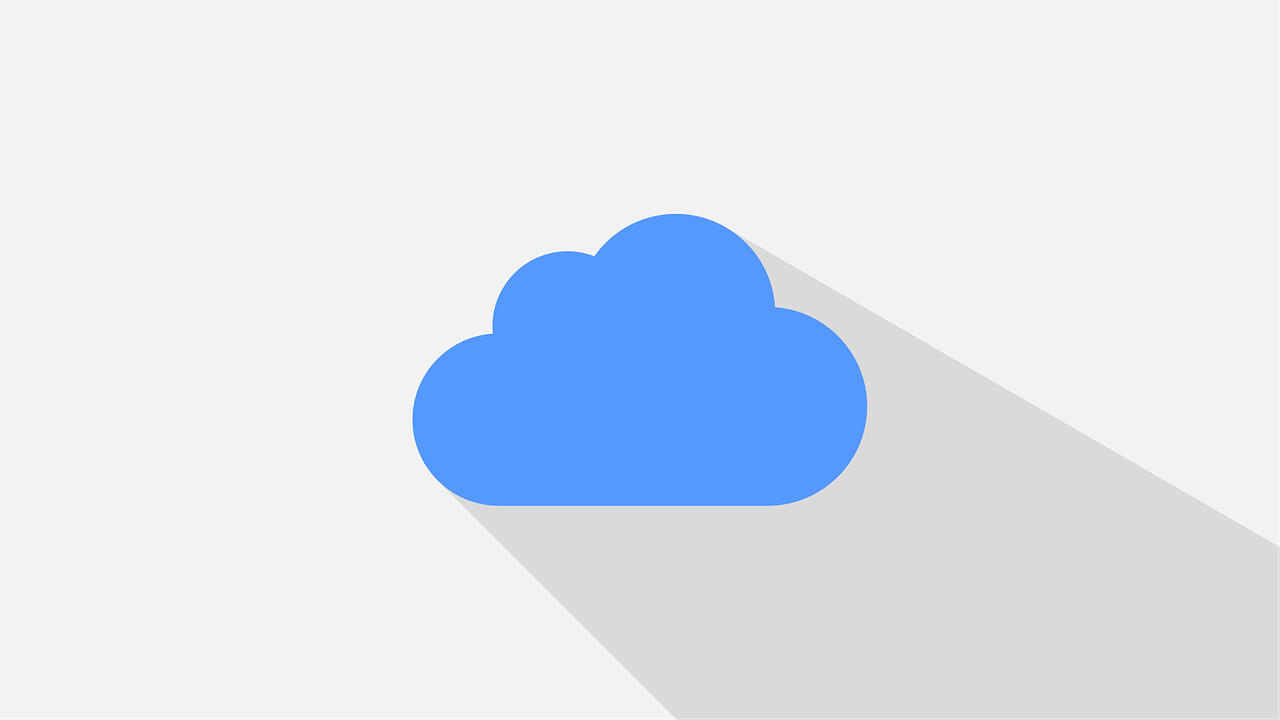
2. 编写Mapper类
Mapper类负责读取输入数据并生成中间键值对,在这个例子中,Mapper将每一行文本拆分成单词,并输出单词及其初始计数值1。
import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.LongWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Mapper; import java.io.IOException; import java.util.StringTokenizer; public class WordCountMapper extends Mapper<LongWritable, Text, Text, IntWritable> { private final static IntWritable one = new IntWritable(1); private Text word = new Text(); @Override protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { StringTokenizer itr = new StringTokenizer(value.toString()); while (itr.hasMoreTokens()) { word.set(itr.nextToken()); context.write(word, one); } } }
3. 编写Reducer类
Reducer类负责接收Mapper生成的中间键值对,并对其进行归约操作,在这个例子中,Reducer将累加每个单词的计数值。
import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Reducer; import java.io.IOException; public class WordCountReducer extends Reducer<Text, IntWritable, Text, IntWritable> { @Override protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } context.write(key, new IntWritable(sum)); } }
4. 编写Driver类
Driver类负责设置作业的配置信息,包括输入输出路径、Mapper和Reducer类等。
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat; public class WordCountDriver { public static void main(String[] args) throws Exception { if (args.length != 2) { System.err.println("Usage: WordCount <input path> <output path>"); System.exit(-1); } Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCountDriver.class); job.setMapperClass(WordCountMapper.class); job.setCombinerClass(WordCountReducer.class); job.setReducerClass(WordCountReducer.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
5. 运行程序
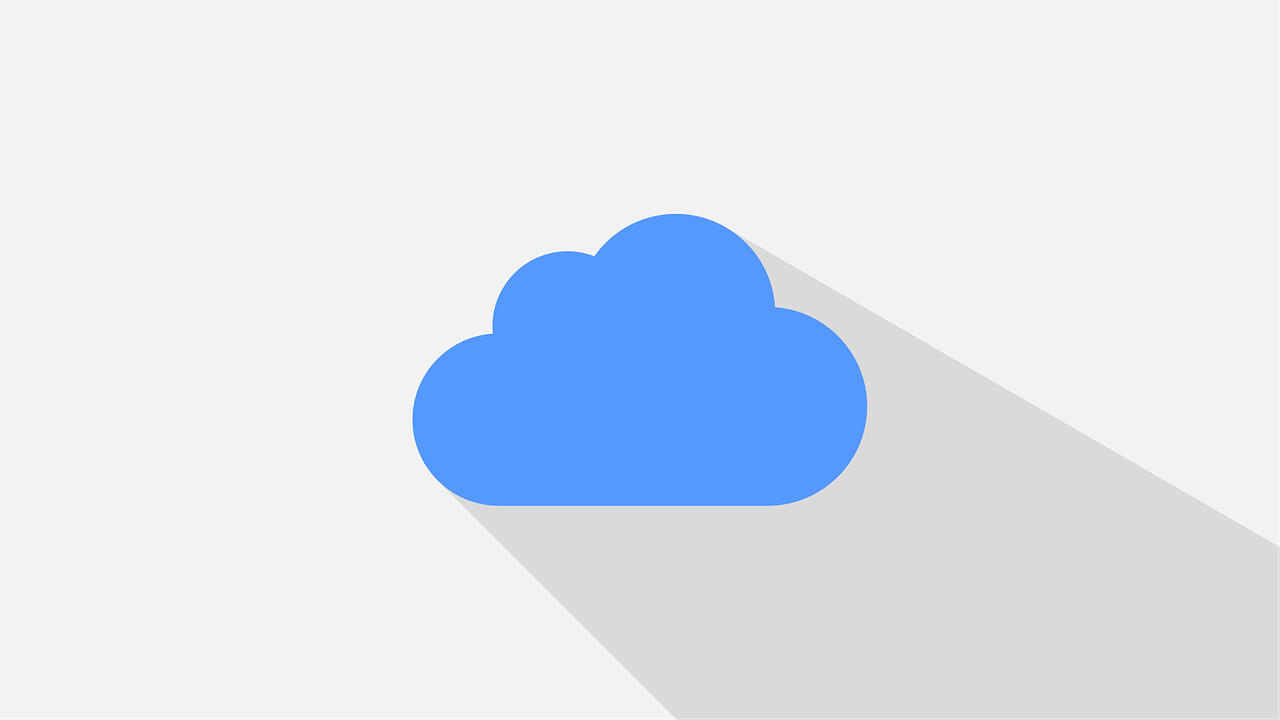
编译并打包上述Java代码,然后将其提交到Hadoop集群执行,假设输入文件位于HDFS的/user/hadoop/input
目录下,输出结果将存储在/user/hadoop/output
目录下。
hadoop jar wordcount.jar WordCountDriver /user/hadoop/input /user/hadoop/output
6. 查看结果
执行完成后,可以通过以下命令查看输出结果:
hdfs dfs -cat /user/hadoop/output/part-r-00000
输出结果应该类似于:
I 1 Hello 3 is 1 love 1 MapReduce 2 world 1 powerful 1
相关问答FAQs
Q1: MapReduce中的Combiner是什么?它有什么作用?
A1: Combiner是MapReduce中的一个优化工具,它是一个本地的Reduce任务,通常与Mapper在同一个节点上运行,Combiner的作用是在Map阶段对中间结果进行局部汇总,减少传输到Reducer的数据量,从而提高整个作业的性能,在单词统计的例子中,Combiner可以对每个Mapper产生的中间结果进行初步汇总,减少网络传输的开销。
Q2: 如果输入数据非常大,MapReduce作业是否会因为内存不足而失败?如何优化?
A2: 如果输入数据非常大,确实可能会导致MapReduce作业因为内存不足而失败,以下是几种常见的优化方法:
1、增加节点数:通过增加集群中的节点数量,可以分散数据和计算压力。
2、调整Map和Reduce任务的数量:合理设置Map和Reduce任务的数量,避免单个任务处理过多数据。
3、压缩中间数据:启用中间数据的压缩,可以显著减少网络传输的数据量。
4、优化Mapper和Reducer的逻辑:确保Mapper和Reducer的逻辑尽可能高效,避免不必要的计算和内存消耗。
5、使用外部排序:对于非常大的数据集,可以使用外部排序来处理中间数据,减少内存占用。
通过以上优化措施,可以有效提升MapReduce作业的性能和稳定性。
小伙伴们,上文介绍了“mapreduce 单词统计_MapReduce统计样例程序”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1332125.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复