pthread
库创建线程。,“c,#include,void* thread_function(void* arg) {, // 线程代码,},int main() {, pthread_t thread;, pthread_create(&thread, NULL, thread_function, NULL);, pthread_join(thread, NULL);, return 0;,},
“在Linux操作系统中,使用C语言创建和管理线程是一项常见的任务,本文将详细介绍如何使用C语言在Linux环境下创建线程,包括线程的创建、执行、同步以及终止等方面的内容。
线程的基本概念
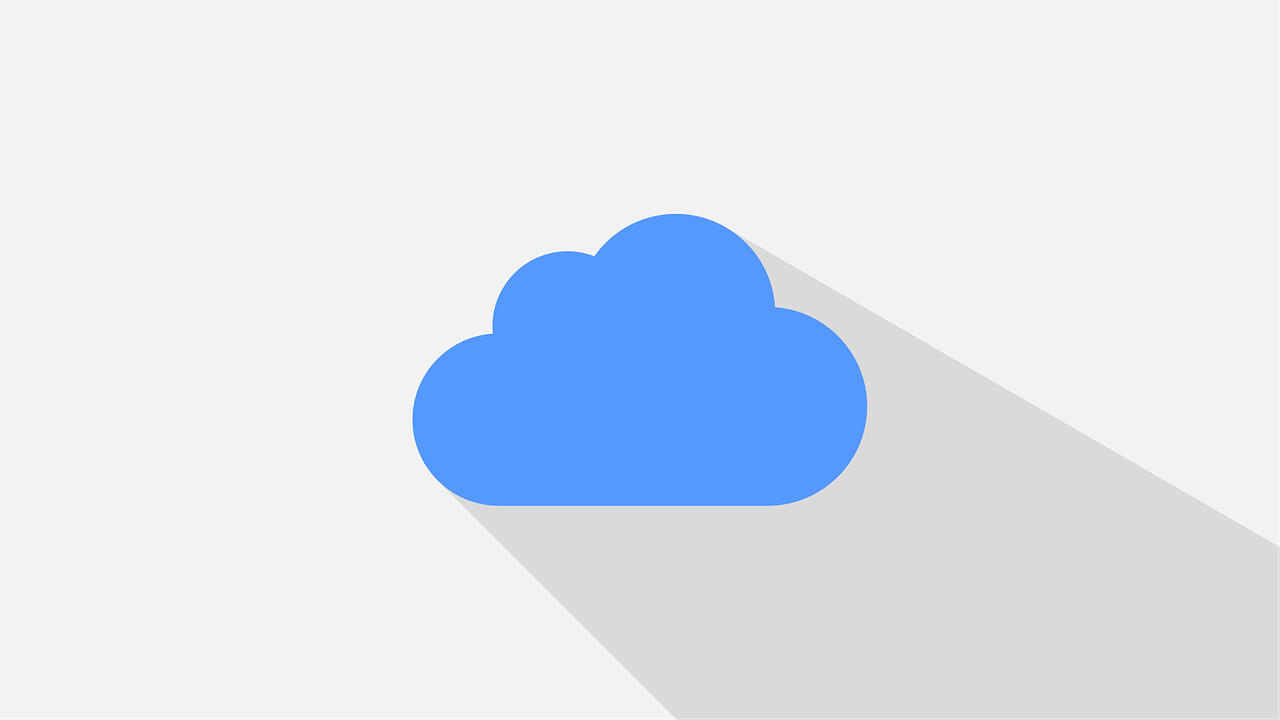
在多线程编程中,线程是程序的最小执行单元,一个进程可以包含多个线程,每个线程共享进程的资源(如内存、文件句柄等),但每个线程都有自己的栈空间和寄存器集合,线程之间可以通过共享数据进行通信,也可以使用同步机制来避免资源竞争。
线程库的选择
在Linux系统中,常用的线程库有POSIX线程(pthread)和NPTL(Native POSIX Thread Library),本文将主要介绍如何使用POSIX线程库创建和管理线程。
创建线程
要使用POSIX线程库创建线程,需要包含头文件<pthread.h>
,并链接相应的库文件,下面是一个创建线程的简单示例:
#include <stdio.h> #include <stdlib.h> #include <pthread.h> // 线程函数 void* thread_func(void* arg) { printf("Hello from new thread! "); return NULL; } int main() { pthread_t tid; int ret; // 创建线程 ret = pthread_create(&tid, NULL, thread_func, NULL); if (ret != 0) { fprintf(stderr, "Failed to create thread "); exit(EXIT_FAILURE); } // 等待线程结束 pthread_join(tid, NULL); printf("Main thread exits "); return 0; }
在这个示例中,我们定义了一个线程函数thread_func
,然后在main
函数中使用pthread_create
函数创建了一个新的线程。pthread_create
函数的第一个参数是指向线程ID的指针,第二个参数是线程属性,通常传递NULL表示使用默认属性,第三个参数是线程函数的指针,第四个参数是传递给线程函数的参数,使用pthread_join
函数等待新创建的线程结束。
线程同步
在多线程编程中,线程之间的同步是非常重要的,POSIX线程库提供了多种同步机制,如互斥锁(mutex)、条件变量(condition variable)和读写锁(read-write lock)等,下面是一个使用互斥锁的示例:
#include <stdio.h> #include <stdlib.h> #include <pthread.h> pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; void* thread_func(void* arg) { pthread_mutex_lock(&mutex); printf("Thread %ld has locked the mutex ", pthread_self()); sleep(1); // 模拟一些工作 printf("Thread %ld is unlocking the mutex ", pthread_self()); pthread_mutex_unlock(&mutex); return NULL; } int main() { pthread_t threads[5]; int i; for (i = 0; i < 5; i++) { pthread_create(&threads[i], NULL, thread_func, NULL); } for (i = 0; i < 5; i++) { pthread_join(threads[i], NULL); } printf("All threads have finished execution "); return 0; }
在这个示例中,我们定义了一个全局的互斥锁mutex
,并在线程函数中使用pthread_mutex_lock
和pthread_mutex_unlock
函数来锁定和解锁互斥锁,这样可以确保在同一时间只有一个线程可以访问共享资源。
线程终止
线程可以通过调用pthread_exit
函数或从线程函数返回来终止,当一个线程终止时,它会释放其栈空间和寄存器集合,如果主线程终止,整个进程也会随之终止,通常需要在主线程中等待所有子线程结束后再退出。
常见问题及解答(FAQs)
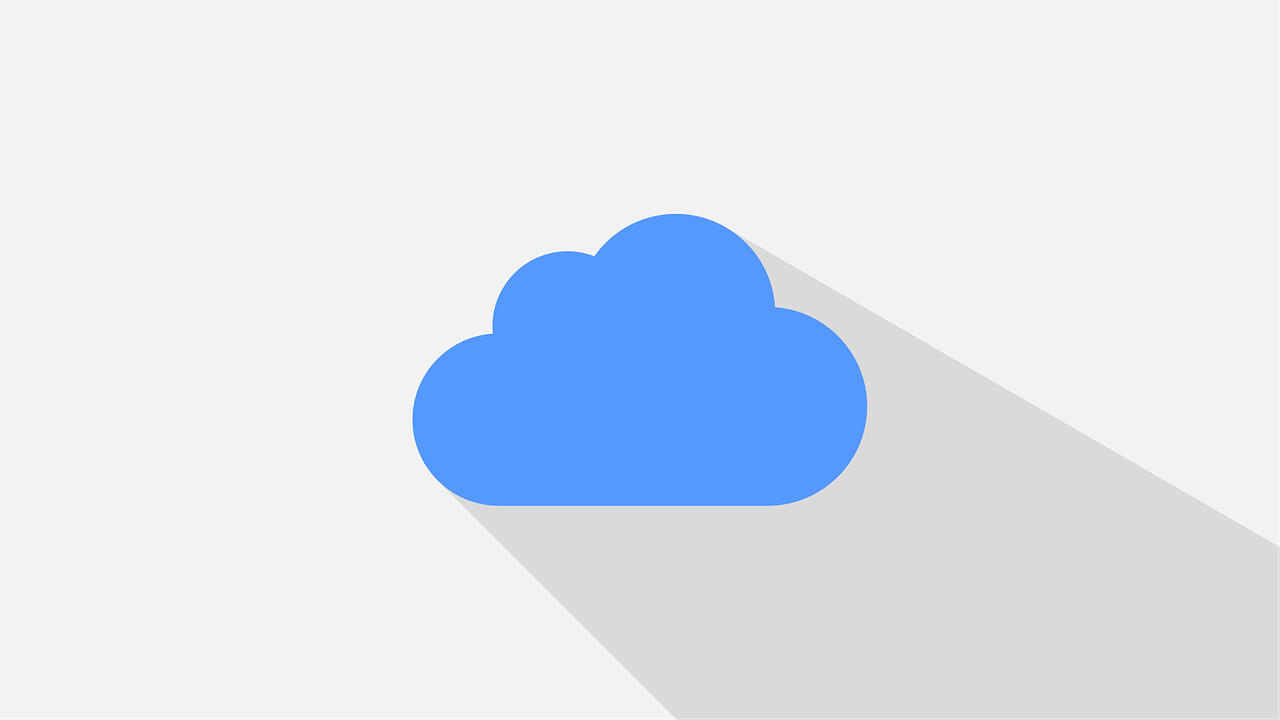
Q1: 如何在C语言中创建线程?
A1: 在C语言中,可以使用POSIX线程库(pthread)来创建线程,首先需要包含头文件<pthread.h>
,然后使用pthread_create
函数创建一个新线程。
pthread_t tid; int ret; ret = pthread_create(&tid, NULL, thread_func, NULL); if (ret != 0) { fprintf(stderr, "Failed to create thread "); exit(EXIT_FAILURE); }
thread_func
是线程函数的指针,NULL
表示使用默认属性,最后一个参数是传递给线程函数的参数。
Q2: 如何实现线程间的同步?
A2: 在多线程编程中,可以使用互斥锁(mutex)、条件变量(condition variable)和读写锁(read-write lock)等同步机制来实现线程间的同步,使用互斥锁可以确保在同一时间只有一个线程可以访问共享资源:
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; pthread_mutex_lock(&mutex); // 临界区代码 pthread_mutex_unlock(&mutex);
小伙伴们,上文介绍了“linux c 创建线程”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1325359.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复