gettid()
系统调用来获取当前线程的线程 ID。这个函数定义在 ` 头文件中,并且可以通过以下方式使用:,,
`c,#include,#include,#include,,pid_t tid = syscall(SYS_gettid);,
“,,这段代码会返回当前线程的线程 ID。在Linux操作系统中,线程是轻量级进程,它们共享相同的地址空间和资源,为了管理和调试多线程程序,有时需要获取线程的ID(TID),本文将详细介绍如何在Linux环境下获取线程ID的方法。
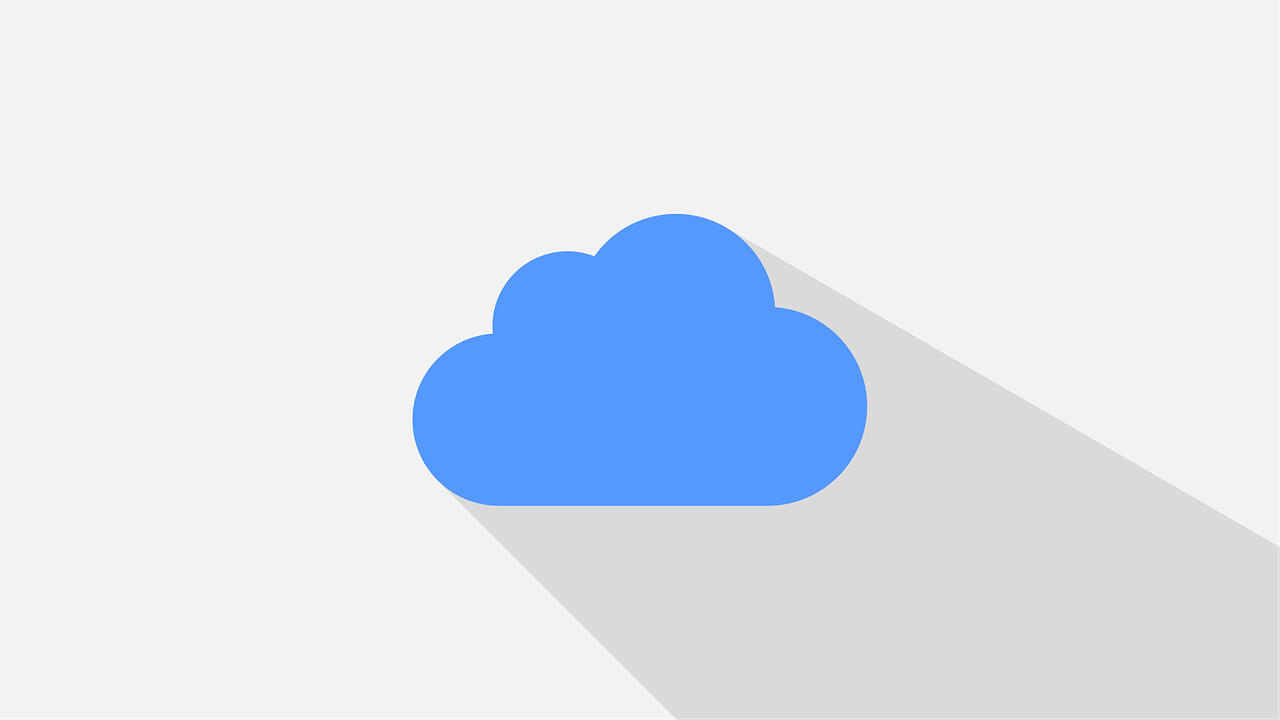
方法一:使用pthread_self()
pthread_self()
是一个POSIX线程库函数,用于返回调用线程的线程ID,该函数定义在<pthread.h>
头文件中。
示例代码
#include <stdio.h> #include <pthread.h> void* thread_function(void* arg) { printf("Thread ID: %lu ", pthread_self()); return NULL; } int main() { pthread_t thread; pthread_create(&thread, NULL, thread_function, NULL); pthread_join(thread, NULL); return 0; }
解释
pthread_self()
返回当前线程的线程ID。
pthread_create()
创建一个新线程,并执行thread_function
。
pthread_join()
等待新创建的线程结束。
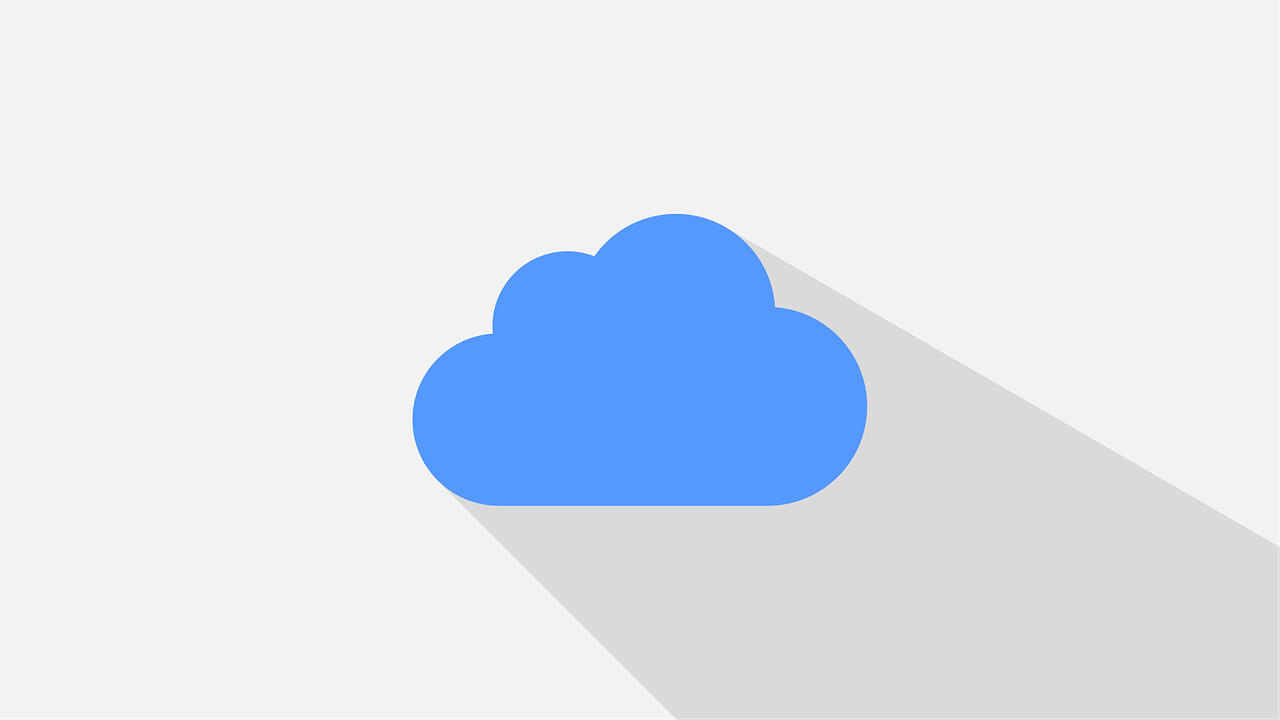
方法二:使用getpid()
和/proc
文件系统
每个线程都有一个唯一的PID(进程ID),可以通过访问/proc
文件系统中的/proc/[pid]/task/[tid]
目录来获取线程ID。
示例代码
#include <stdio.h> #include <unistd.h> #include <sys/types.h> #include <dirent.h> #include <string.h> void print_thread_ids(pid_t pid) { char path[40]; sprintf(path, "/proc/%d/task/", pid); DIR* dir = opendir(path); if (dir == NULL) { perror("opendir"); return; } struct dirent* entry; while ((entry = readdir(dir)) != NULL) { if (entry->d_type == DT_DIR && isdigit(entry->d_name[0])) { printf("Thread ID: %s ", entry->d_name); } } closedir(dir); } int main() { pid_t pid = getpid(); print_thread_ids(pid); return 0; }
解释
getpid()
返回当前进程的PID。
sprintf(path, "/proc/%d/task/", pid)
构建指向线程目录的路径。
opendir()
打开线程目录。
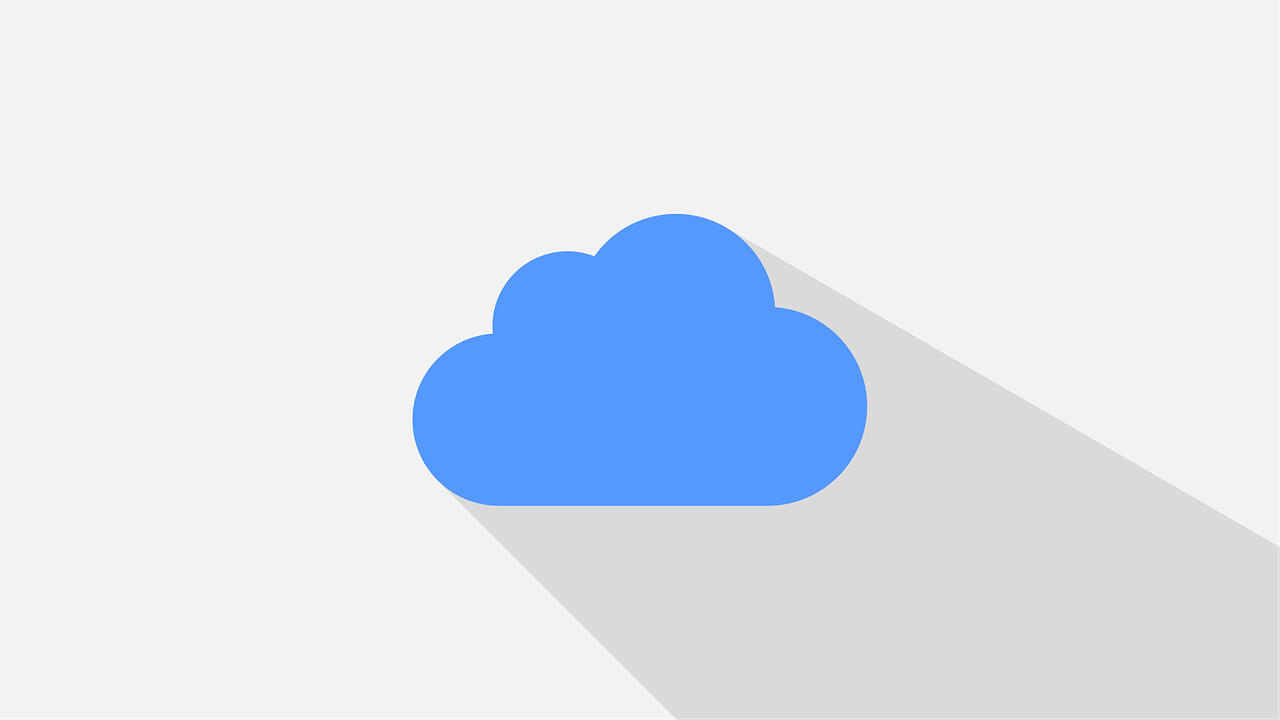
readdir()
读取目录中的条目,检查是否为目录且名称为数字,即为线程ID。
closedir()
关闭目录。
方法三:使用/proc/[pid]/as
/proc/[pid]/as
文件包含了进程的地址空间信息,其中也包含了线程的信息,通过解析该文件,可以提取线程ID。
示例代码
#include <stdio.h> #include <stdlib.h> #include <string.h> void parse_as_file(pid_t pid) { char path[40], line[256]; sprintf(path, "/proc/%d/as", pid); FILE* file = fopen(path, "r"); if (file == NULL) { perror("fopen"); return; } while (fgets(line, sizeof(line), file) != NULL) { char* tid_str = strtok(line, " "); if (tid_str != NULL && isdigit(tid_str[0])) { printf("Thread ID: %s ", tid_str); } } fclose(file); } int main() { pid_t pid = getpid(); parse_as_file(pid); return 0; }
解释
sprintf(path, "/proc/%d/as", pid)
构建指向地址空间文件的路径。
fopen()
打开地址空间文件。
fgets()
逐行读取文件内容。
strtok()
分割字符串,提取线程ID。
fclose()
关闭文件。
方法四:使用 `gdb` 调试器
gdb
是一个强大的调试工具,可以用来调试多线程程序并获取线程ID。
示例命令
gdb -p [pid]
步骤
1、启动gdb
并附加到目标进程。
2、使用info threads
命令查看所有线程的信息,包括线程ID。
3、使用thread [tid]
命令切换到指定线程。
相关问答FAQs
问题1: 如何在C程序中获取当前线程的ID?
答: 在C程序中,可以使用pthread_self()
函数获取当前线程的ID。
#include <stdio.h> #include <pthread.h> void* thread_function(void* arg) { printf("Thread ID: %lu ", pthread_self()); return NULL; } int main() { pthread_t thread; pthread_create(&thread, NULL, thread_function, NULL); pthread_join(thread, NULL); return 0; }
这个程序会输出当前线程的ID。
问题2: 如何在Linux命令行中获取某个进程的所有线程ID?
答: 可以使用以下命令获取某个进程的所有线程ID:
ps -eLf | grep [pid]
要获取PID为1234的进程的所有线程ID,可以运行:
ps -eLf | grep 1234
这将列出所有与该进程相关的线程及其详细信息。
到此,以上就是小编对于“linux 获取 线程 id”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1324399.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复