OAuth 服务器搭建
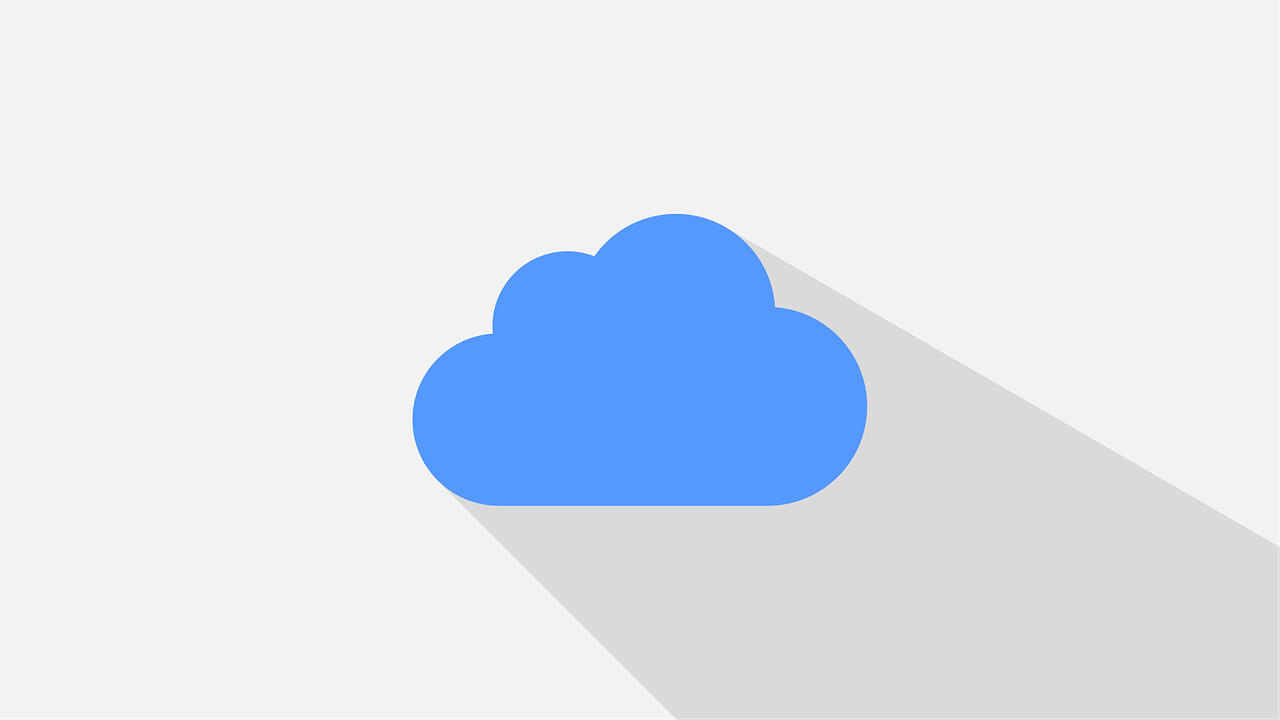
OAuth是一种开放标准,允许用户在不共享他们的用户名和密码的情况下,让第三方应用程序访问他们在特定网站上的私密资源,通过OAuth,用户能够授权第三方应用在一定时间内访问其存储在服务提供商上的资源,而无需直接暴露自己的登录凭证,本文将详细介绍如何使用Spring Boot搭建一个基本的OAuth2认证服务器,包括必要的配置步骤、代码示例以及常见问题解答。
一、环境准备
1、开发工具:推荐使用IntelliJ IDEA或Eclipse等IDE。
2、JDK版本:需要Java 8及以上版本。
3、构建工具:Maven 3.2+ 或 Gradle 4.x+。
4、数据库:MySQL 5.7+ 或其他兼容的关系型数据库。
5、Spring Boot版本:2.3.x 或更高版本(确保兼容Spring Security 5.3.x)。
6、依赖管理:Maven或Gradle,用于项目依赖管理和构建。
二、创建Spring Boot项目
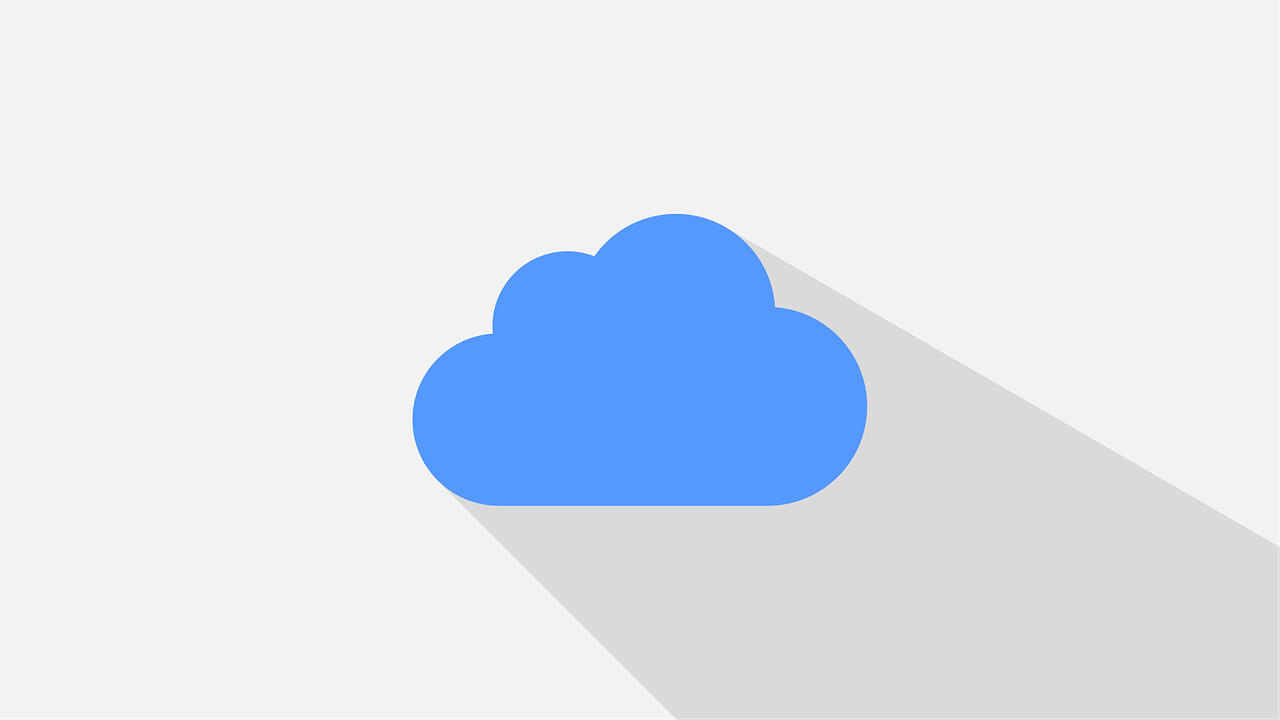
可以使用Spring Initializr来快速创建一个Spring Boot项目,选择以下依赖项:
Spring Web
Spring Security
OAuth2 Authorization Server(可选)
三、配置数据库连接
在application.properties
或application.yml
中添加数据库连接配置:
spring.datasource.url=jdbc:mysql://localhost:3306/oauth_db?useSSL=false&serverTimezone=UTC&characterEncoding=utf8 spring.datasource.username=root spring.datasource.password=yourpassword spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5Dialect
四、创建数据库表结构
OAuth2需要几个关键的表来存储客户端信息、用户信息、访问令牌等,可以使用以下SQL脚本创建这些表:
CREATE TABLE oauth_client_details ( client_id VARCHAR(256) PRIMARY KEY, resource_ids VARCHAR(256), client_secret VARCHAR(256), scope VARCHAR(256), authorized_grant_types VARCHAR(256), web_server_redirect_uri VARCHAR(256), authorities VARCHAR(256), access_token_validity INTEGER, refresh_token_validity INTEGER, additional_information VARCHAR(4096), autoapprove VARCHAR(256) ); CREATE TABLE oauth_client_token ( token_id VARCHAR(256), token LONG VARBINARY, authentication_id VARCHAR(256) PRIMARY KEY, user_name VARCHAR(256), client_id VARCHAR(256) ); CREATE TABLE oauth_access_token ( token_id VARCHAR(256), token LONG VARBINARY, authentication_id VARCHAR(256) PRIMARY KEY, user_name VARCHAR(256), client_id VARCHAR(256), authentication LONG VARBINARY, refresh_token LONG VARBINARY ); CREATE TABLE oauth_refresh_token ( token_id VARCHAR(256), token LONG VARBINARY, authentication LONG VARBINARY );
五、配置OAuth2认证服务器
在Spring Security配置文件中启用OAuth2认证服务器功能,并配置客户端详情服务、授权管理器、令牌增强器等,以下是一个简单的配置示例:
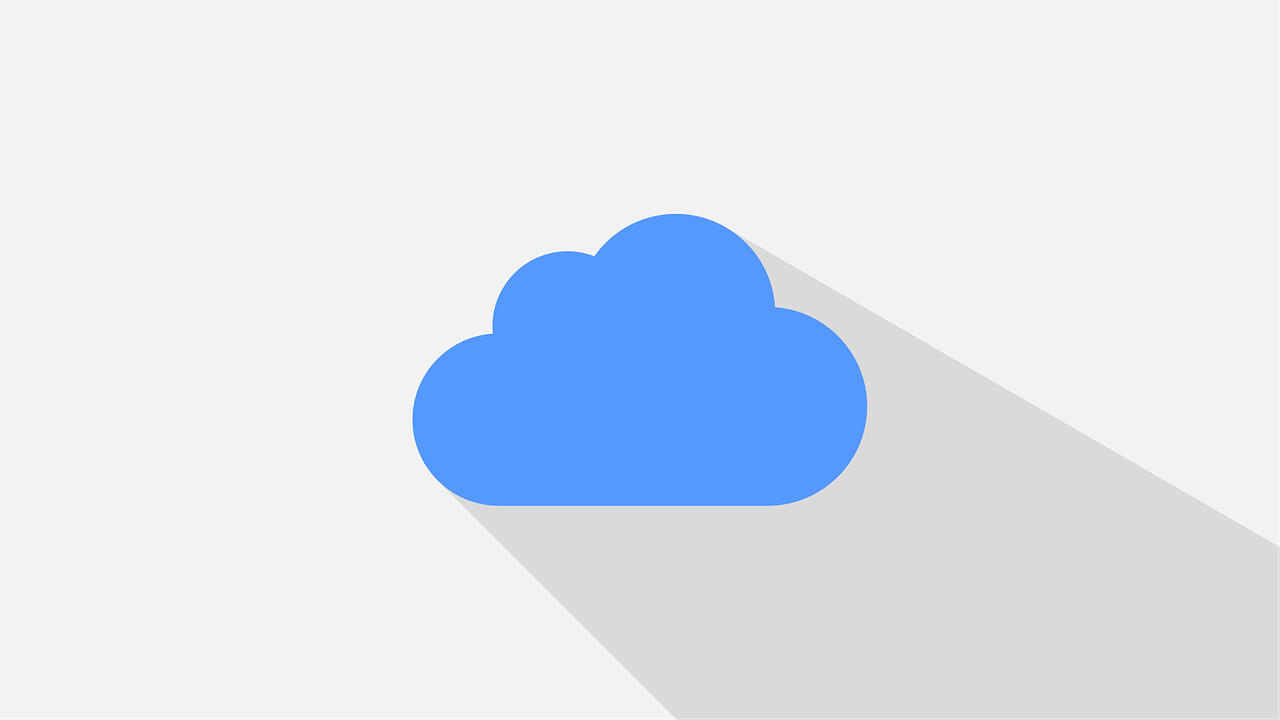
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; import org.springframework.security.crypto.password.PasswordEncoder; import org.springframework.security.oauth2.config.annotation.configurers.ClientDetailsServiceConfigurer; import org.springframework.security.oauth2.config.annotation.web.configuration.AuthorizationServerConfigurerAdapter; import org.springframework.security.oauth2.config.annotation.web.configuration.EnableAuthorizationServer; import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer; import org.springframework.security.oauth2.provider.ClientDetailsUserDetailsService; import org.springframework.security.oauth2.provider.client.JdbcClientDetailsService; import org.springframework.security.oauth2.provider.code.AuthorizationCodeServices; import org.springframework.security.oauth2.provider.code.JdbcAuthorizationCodeServices; import org.springframework.security.oauth2.provider.token.TokenEnhancerChain; import org.springframework.security.oauth2.provider.token.store.JdbcTokenStore; import org.springframework.security.provisioning.JdbcUserDetailsManager; import org.springframework.security.web.util.matcher.RequestMatchers; import javax.sql.DataSource; @Configuration @EnableAuthorizationServer public class OAuth2ServerConfig extends AuthorizationServerConfigurerAdapter { @Autowired private DataSource dataSource; @Override public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception { // 配置令牌存储策略为JDBC TokenEnhancerChain tokenEnhancerChain = new TokenEnhancerChain(); JdbcTokenStore jdbcTokenStore = new JdbcTokenStore(dataSource); tokenEnhancerChain.setTokenStore(jdbcTokenStore); endpoints.tokenStore(jdbcTokenStore) .authorizationCodeServices(new JdbcAuthorizationCodeServices(dataSource)) .tokenEnhancer(tokenEnhancerChain); } @Override public void configure(ClientDetailsServiceConfigurer clients) throws Exception { // 配置客户端详情服务为JDBC JdbcClientDetailsService jdbcClientDetailsService = new JdbcClientDetailsService(dataSource); clients.withClientDetails(jdbcClientDetailsService); } }
六、配置Web安全和用户认证
配置Spring Security以拦截所有请求,并通过自定义用户详情服务进行用户认证,以下是一个简单的配置示例:
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.authentication.AuthenticationManager; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.provisioning.JdbcUserDetailsManager; import org.springframework.security.web.util.matcher.RequestMatchers; import javax.sql.DataSource; @Configuration @EnableWebSecurity public class WebSecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private DataSource dataSource; @Bean @Override public UserDetailsService userDetailsService() { // 配置用户详情服务为JDBC,从数据库中加载用户信息 JdbcUserDetailsManager jdbcUserDetailsManager = new JdbcUserDetailsManager(); jdbcUserDetailsManager.setDataSource(dataSource); return jdbcUserDetailsManager; } @Bean @Override public AuthenticationManager authenticationManagerBean() throws Exception { return super.authenticationManagerBean(); } @Override protected void configure(HttpSecurity http) throws Exception { http .requestMatchers(RequestMatchers.anyRequest()) .authorizeRequests() .antMatchers("/css/", "/js/", "/images/", "/webjars/").permitAll() .anyRequest().authenticated() .and() .formLogin().permitAll() .and() .logout().permitAll(); } }
七、启动与测试
完成以上配置后,可以启动Spring Boot应用,并通过浏览器或Postman等工具测试OAuth2认证流程,可以通过以下步骤测试密码模式获取令牌:
1、发送POST请求到/oauth/token
端点,包含以下参数:
grant_type=password
username=<你的用户名>
password=<你的密码>
scope=read write
(根据需要)
2、验证响应中的访问令牌,并使用该令牌访问受保护的资源。
八、相关FAQs问答
Q1: OAuth2支持哪些授权模式?如何在Spring Boot中配置它们?A1: OAuth2支持四种主要的授权模式:授权码模式(Authorization Code)、简化模式(Implicit)、密码模式(Resource Owner Password Credentials)、客户端模式(Client Credentials),在Spring Boot中,可以通过HttpSecurity
配置类来配置不同的授权模式,可以在configure(HttpSecurity http)
方法中添加相应的过滤器链和授权请求处理器来处理不同的授权模式,对于授权码模式,可以添加authorizationCode()
方法调用;对于密码模式,可以添加resourceOwnerPassword()
方法调用等,还可以通过自定义AuthorizationServerConfigurer
来实现更复杂的授权逻辑,需要注意的是,不同的授权模式适用于不同的应用场景和需求,因此在选择时应根据具体情况进行权衡和决策,为了确保系统的安全性,还应对各种授权模式进行适当的安全配置和防护措施。
小伙伴们,上文介绍了“OAuth 服务器搭建”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1308567.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复