Linux C Map
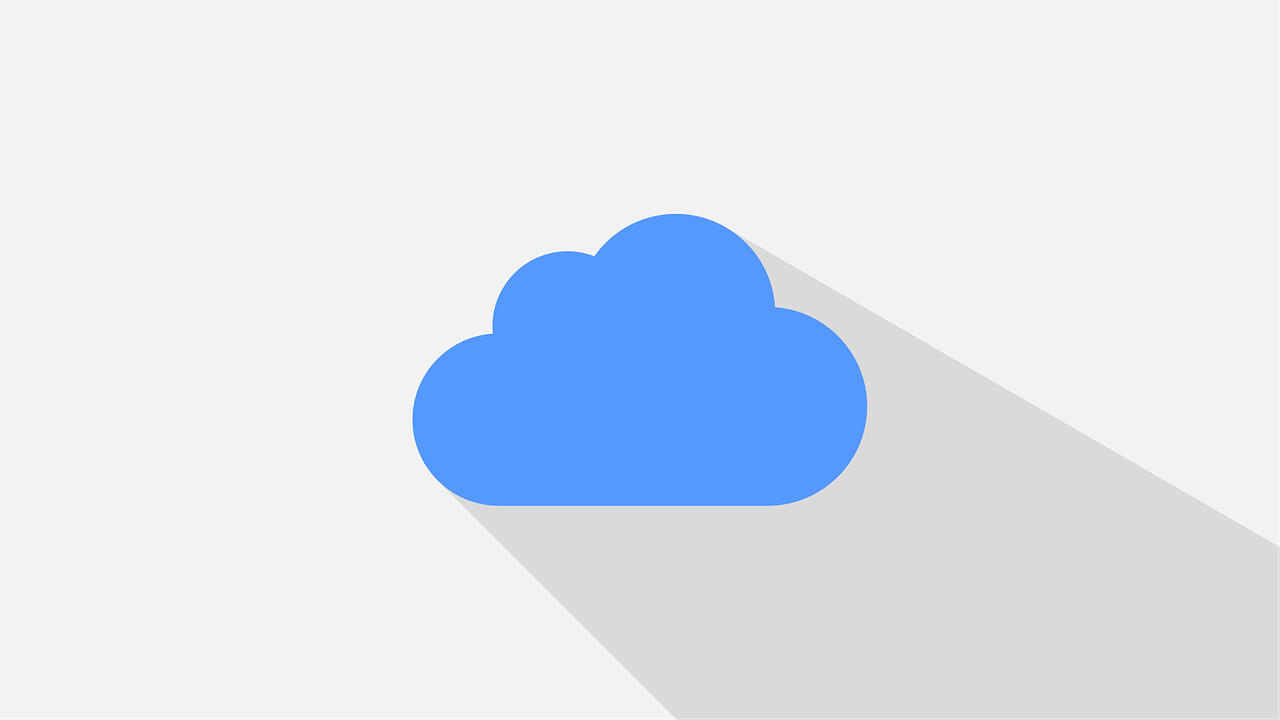
在Linux系统编程中,mmap
函数是一个强大的工具,用于将文件或设备映射到内存地址空间,这使得进程能够像访问普通内存一样访问文件或设备,从而提高了I/O操作的效率,本文将详细介绍mmap
函数的使用方法、注意事项以及相关的示例代码。
什么是 mmap?
mmap
(memory map)是一种将文件或设备的内容映射到进程的虚拟地址空间的技术,通过这种方式,进程可以直接读取和写入文件内容,而无需进行传统的文件读写操作,这种技术广泛应用于数据库系统、文件系统、共享内存等场景。
使用 mmap 的步骤
1、打开文件:使用open
函数打开需要映射的文件。
2、创建内存映射:使用mmap
函数将文件映射到内存。
3、访问文件内容:通过指针直接访问内存映射区域。
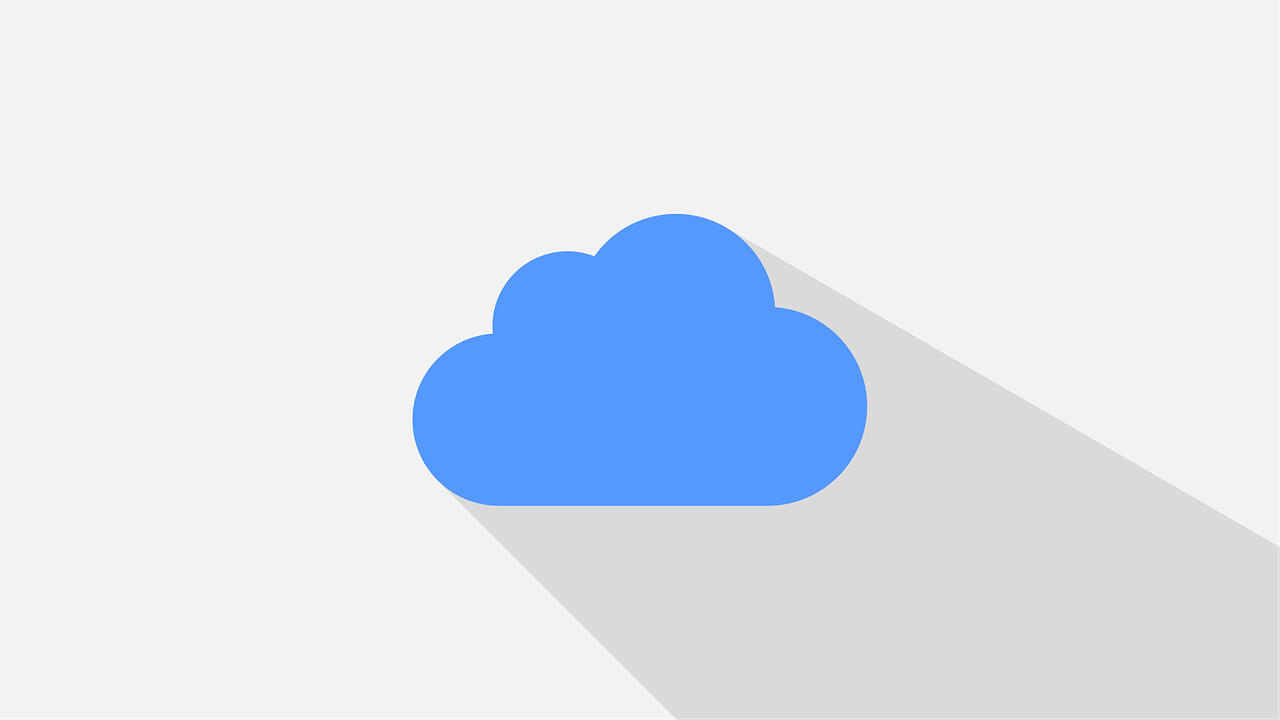
4、解除映射:使用munmap
函数解除内存映射。
5、关闭文件:使用close
函数关闭文件。
以下是一个简单的示例代码,展示了如何使用mmap
函数将一个文本文件映射到内存并读取其内容。
#include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <sys/mman.h> #include <sys/stat.h> #include <unistd.h> int main() { const char *filepath = "example.txt"; // 打开文件 int fd = open(filepath, O_RDONLY); if (fd == -1) { perror("Error opening file"); exit(EXIT_FAILURE); } // 获取文件大小 struct stat sb; if (fstat(fd, &sb) == -1) { perror("Error getting the file size"); close(fd); exit(EXIT_FAILURE); } // 将文件内容映射到内存 void *mapped = mmap(NULL, sb.st_size, PROT_READ, MAP_PRIVATE, fd, 0); if (mapped == MAP_FAILED) { perror("Error mmapping the file"); close(fd); exit(EXIT_FAILURE); } // 读取文件内容 printf("%s", (char *)mapped); // 解除内存映射 if (munmap(mapped, sb.st_size) == -1) { perror("Error un-mmapping the file"); close(fd); exit(EXIT_FAILURE); } // 关闭文件 close(fd); return 0; }
参数解析
addr
:指向希望开始映射的地址,通常设置为NULL
,让内核自动选择。
length
:映射区域的字节数。
prot
:映射区域的保护标志,如PROT_READ
(只读)、PROT_WRITE
(可写)等。
flags
:影响映射行为的标志,如MAP_PRIVATE
(私有拷贝)、MAP_SHARED
(共享)等。
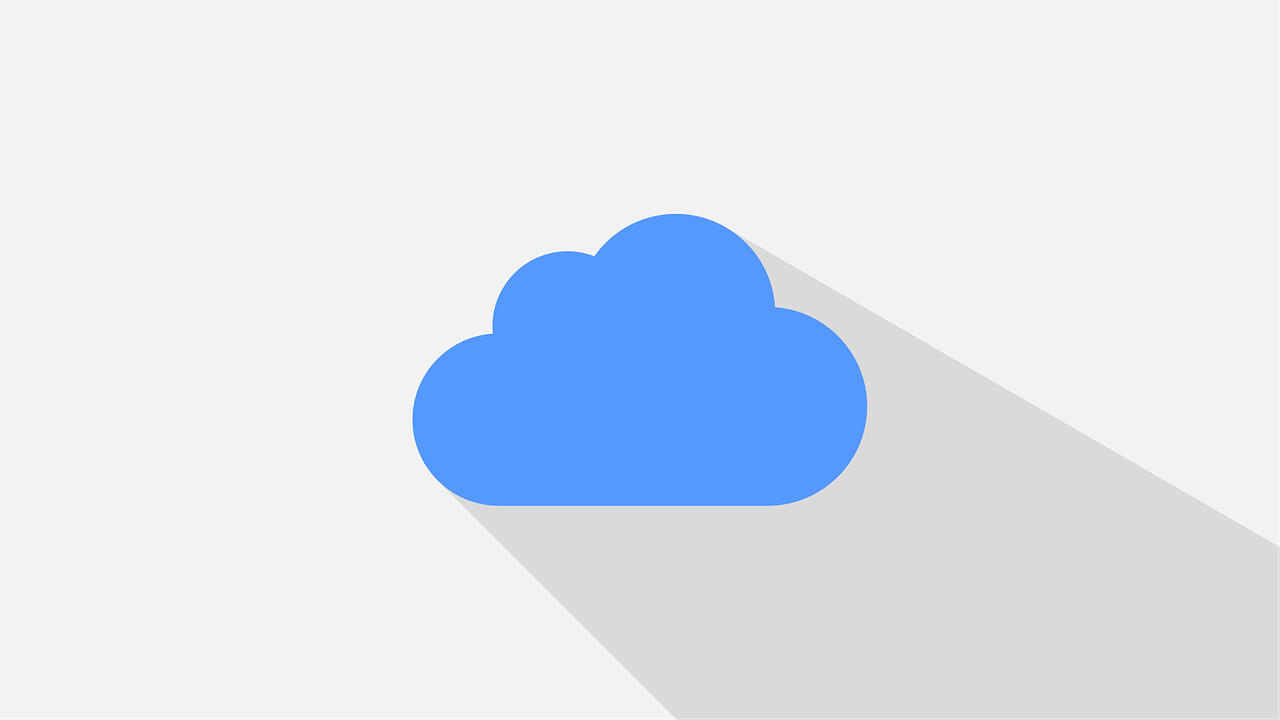
fd
:文件描述符。
offset
:文件中的偏移量,通常设置为0
。
注意事项
1、文件描述符有效性:确保在调用mmap
之前已经成功打开了文件,并且在使用完毕后正确关闭文件。
2、权限检查:确保进程具有足够的权限来访问文件,如果没有足够的权限,mmap
可能会失败。
3、错误处理:始终检查mmap
和munmap
的返回值,以处理可能的错误情况。
4、内存同步:对于MAP_SHARED
映射,修改后的数据会自动同步回文件;对于MAP_PRIVATE
映射,则不会。
5、内存泄漏:确保在不再需要时调用munmap
解除映射,避免内存泄漏。
6、对齐要求:某些系统可能要求映射的地址和长度满足特定的对齐要求,具体可以参考系统的文档。
相关问答FAQs
Q1: 如何更改 mmap 映射区域的保护属性?
A1: 可以使用mprotect
函数来更改内存映射区域的保护属性,可以将一个只读的映射区域更改为可读写,以下是一个简单的示例代码:
#include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <sys/mman.h> #include <sys/stat.h> #include <unistd.h> int main() { const char *filepath = "example.txt"; // 打开文件 int fd = open(filepath, O_RDONLY); if (fd == -1) { perror("Error opening file"); exit(EXIT_FAILURE); } // 获取文件大小 struct stat sb; if (fstat(fd, &sb) == -1) { perror("Error getting the file size"); close(fd); exit(EXIT_FAILURE); } // 将文件内容映射到内存 void *mapped = mmap(NULL, sb.st_size, PROT_READ, MAP_PRIVATE, fd, 0); if (mapped == MAP_FAILED) { perror("Error mmapping the file"); close(fd); exit(EXIT_FAILURE); } // 更改映射区域的保护属性为可读写 if (mprotect(mapped, sb.st_size, PROT_READ | PROT_WRITE) == -1) { perror("Error changing protection"); munmap(mapped, sb.st_size); close(fd); exit(EXIT_FAILURE); } // 修改映射区域的内容 ((char *)mapped)[0] = 'A'; // 解除内存映射 if (munmap(mapped, sb.st_size) == -1) { perror("Error un-mmapping the file"); close(fd); exit(EXIT_FAILURE); } // 关闭文件 close(fd); return 0; }
在这个示例中,我们首先将文件映射为只读,然后使用mprotect
函数将其更改为可读写,最后修改了映射区域的内容并解除映射,需要注意的是,不是所有的系统都支持更改映射区域的保护属性。
A2: 要将两个文件的内容合并到一个映射区域中,可以分别映射这两个文件,然后将它们的内容复制到一个新的内存区域中,以下是一个示例代码:
#include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <sys/mman.h> #include <sys/stat.h> #include <unistd.h> #include <string.h> int main() { const char *filepath1 = "file1.txt"; const char *filepath2 = "file2.txt"; // 打开第一个文件 int fd1 = open(filepath1, O_RDONLY); if (fd1 == -1) { perror("Error opening file1"); exit(EXIT_FAILURE); } // 获取第一个文件的大小 struct stat sb1; if (fstat(fd1, &sb1) == -1) { perror("Error getting the size of file1"); close(fd1); exit(EXIT_FAILURE); } // 打开第二个文件 int fd2 = open(filepath2, O_RDONLY); if (fd2 == -1) { perror("Error opening file2"); close(fd1); exit(EXIT_FAILURE); } // 获取第二个文件的大小 struct stat sb2; if (fstat(fd2, &sb2) == -1) { perror("Error getting the size of file2"); close(fd1); close(fd2); exit(EXIT_FAILURE); } // 计算总大小 size_t total_size = sb1.st_size + sb2.st_size; // 分配内存以存储两个文件的内容 void *combined = malloc(total_size); if (combined == NULL) { perror("Error allocating memory"); close(fd1); close(fd2); exit(EXIT_FAILURE); } // 映射第一个文件到内存 void *mapped1 = mmap(NULL, sb1.st_size, PROT_READ, MAP_PRIVATE, fd1, 0); if (mapped1 == MAP_FAILED) { perror("Error mmapping file1"); free(combined); close(fd1); close(fd2); exit(EXIT_FAILURE); } // 复制第一个文件的内容到新的内存区域 memcpy(combined, sb1.st_size, mapped1, sb1.st_size); munmap(mapped1, sb1.st_size); // 解除第一个文件的映射 // 映射第二个文件到内存 void *mapped2 = mmap(NULL, sb2.st_size, PROT_READ, MAP_PRIVATE, fd2, 0); if (mapped2 == MAP_FAILED) { perror("Error mmapping file2"); free(combined); close(fd1); close(fd2); exit(EXIT_FAILURE); } // 复制第二个文件的内容到新的内存区域,紧跟第一个文件的内容之后 memcpy((char *)combined + sb1.st_size, mapped2, sb2.st_size); munmap(mapped2, sb2.st_size); // 解除第二个文件的映射 // 打印合并后的内容(假设是文本文件) printf("%s ", combined); free(combined); // 释放分配的内存 close(fd1); // 关闭第一个文件 close(fd2); // 关闭第二个文件 return 0;}
到此,以上就是小编对于“linux c map”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1305336.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复