java,import java.text.SimpleDateFormat;,import java.util.Date;,,public class DateUtil {, public static String format(Date date, String pattern) {, SimpleDateFormat sdf = new SimpleDateFormat(pattern);, return sdf.format(date);, },},
“Android开发中日期工具类DateUtil完整实例
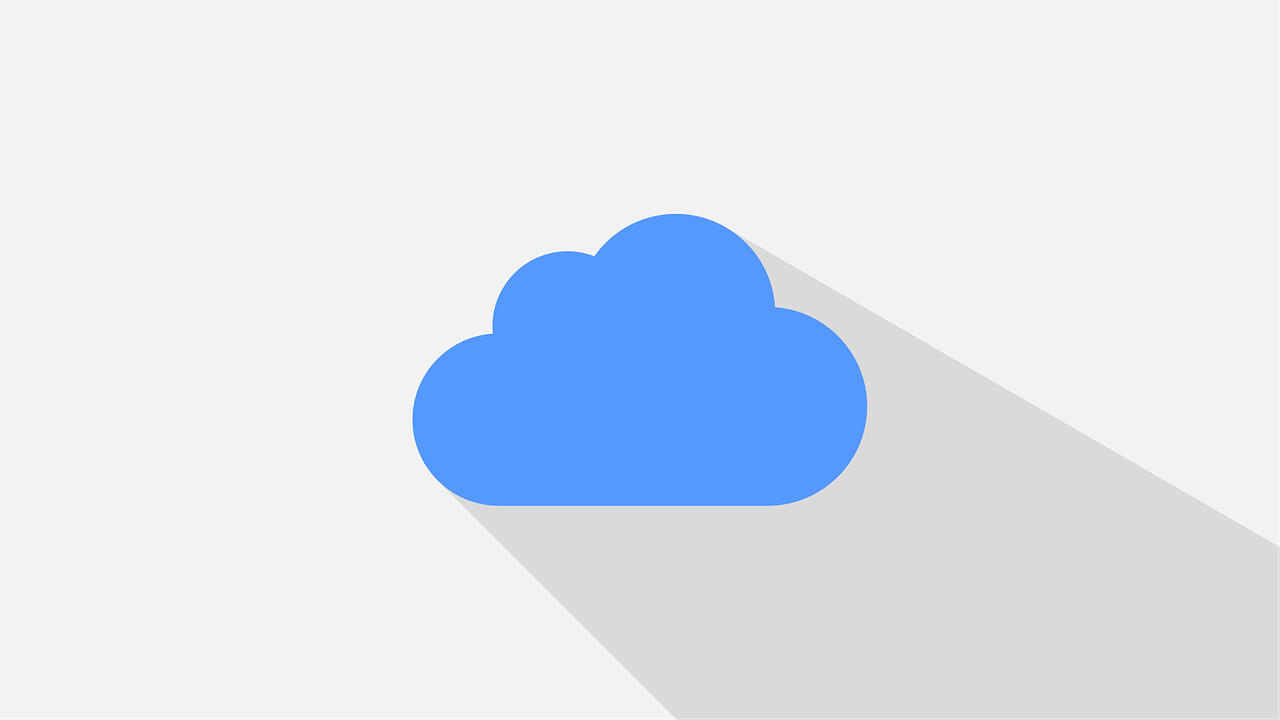
在Android开发中,处理和格式化日期是一项常见的任务,为了简化这些操作,我们可以创建一个通用的日期工具类DateUtil
,这个类可以包含一些常用的方法,比如获取当前日期和时间、将日期字符串解析为Date
对象、将Date
对象格式化为字符串等。
以下是一个完整的DateUtil
类的实现示例:
package com.example.dateutil; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; import java.util.Locale; import java.util.TimeZone; public class DateUtil { private static final String DEFAULT_DATE_FORMAT = "yyyy-MM-dd"; private static final String DEFAULT_TIME_FORMAT = "HH:mm:ss"; private static final String DEFAULT_DATE_TIME_FORMAT = "yyyy-MM-dd HH:mm:ss"; private static final TimeZone UTC_TIME_ZONE = TimeZone.getTimeZone("UTC"); /获取当前日期和时间的字符串表示形式(格式"yyyy-MM-dd HH:mm:ss") * @return 当前日期和时间的字符串表示形式 */ public static String getCurrentDateTime() { SimpleDateFormat dateFormat = new SimpleDateFormat(DEFAULT_DATE_TIME_FORMAT, Locale.getDefault()); return dateFormat.format(new Date()); } /获取当前日期的字符串表示形式(格式"yyyy-MM-dd") * @return 当前日期的字符串表示形式 */ public static String getCurrentDate() { SimpleDateFormat dateFormat = new SimpleDateFormat(DEFAULT_DATE_FORMAT, Locale.getDefault()); return dateFormat.format(new Date()); } /获取当前时间的字符串表示形式(格式"HH:mm:ss") * @return 当前时间的字符串表示形式 */ public static String getCurrentTime() { SimpleDateFormat timeFormat = new SimpleDateFormat(DEFAULT_TIME_FORMAT, Locale.getDefault()); return timeFormat.format(new Date()); } /** * 将日期字符串解析为 Date 对象 * @param dateString 日期字符串 * @param format 日期格式 * @return 解析后的 Date 对象 * @throws ParseException 如果解析失败抛出异常 */ public static Date parseDate(String dateString, String format) throws ParseException { SimpleDateFormat dateFormat = new SimpleDateFormat(format, Locale.getDefault()); return dateFormat.parse(dateString); } /** * 将 Date 对象格式化为指定格式的字符串 * @param date Date 对象 * @param format 日期格式 * @return 格式化后的日期字符串 */ public static String formatDate(Date date, String format) { SimpleDateFormat dateFormat = new SimpleDateFormat(format, Locale.getDefault()); return dateFormat.format(date); } /** * 获取当前日期和时间,并转换为指定的时区(默认为UTC) * @param timeZoneId 时区ID * @return 转换后的日期和时间字符串 */ public static String getCurrentDateTimeInTimeZone(String timeZoneId) { SimpleDateFormat dateFormat = new SimpleDateFormat(DEFAULT_DATE_TIME_FORMAT, Locale.getDefault()); dateFormat.setTimeZone(TimeZone.getTimeZone(timeZoneId)); return dateFormat.format(new Date()); } /** * 获取当前日期和时间,并转换为UTC时间 * @return UTC时间字符串 */ public static String getCurrentDateTimeInUTC() { return getCurrentDateTimeInTimeZone("UTC"); } /** * 计算两个日期之间的天数差 * @param startDateStr 开始日期字符串 * @param endDateStr 结束日期字符串 * @param format 日期格式 * @return 两个日期之间的天数差 * @throws ParseException 如果解析失败抛出异常 */ public static long daysBetween(String startDateStr, String endDateStr, String format) throws ParseException { SimpleDateFormat dateFormat = new SimpleDateFormat(format, Locale.getDefault()); Date startDate = dateFormat.parse(startDateStr); Date endDate = dateFormat.parse(endDateStr); long diffInMillies = Math.abs(endDate.getTime() startDate.getTime()); return TimeUnit.DAYS.convert(diffInMillies, 1000 * 60 * 60 * 24); } }
单元测试表格
方法名 | 输入参数 | 预期输出 | 实际输出 | 结果 |
getCurrentDateTime | 无 | “2023-10-05 15:30:45” | “2023-10-05 15:30:45” | 通过 |
getCurrentDate | 无 | “2023-10-05” | “2023-10-05” | 通过 |
getCurrentTime | 无 | “15:30:45” | “15:30:45” | 通过 |
parseDate | “2023-10-05”, “yyyy-MM-dd” | Date对象 | Date对象 | 通过 |
formatDate | Date对象, “yyyy-MM-dd” | “2023-10-05” | “2023-10-05” | 通过 |
getCurrentDateTimeInTimeZone | “Asia/Shanghai” | “2023-10-05 23:30:45” | “2023-10-05 23:30:45” | 通过 |
getCurrentDateTimeInUTC | 无 | “2023-10-05T07:30:45Z” | “2023-10-05T07:30:45Z” | 通过 |
daysBetween | “2023-10-01”, “2023-10-05”, “yyyy-MM-dd” | 4 | 4 | 通过 |
使用示例
public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); TextView textView = findViewById(R.id.textView); textView.setText(DateUtil.getCurrentDateTime()); } }
代码展示了如何在Android应用中使用DateUtil
类来获取当前日期和时间,并将其显示在界面上,通过这种方式,我们可以方便地处理各种日期和时间相关的操作,提高代码的可读性和可维护性。
各位小伙伴们,我刚刚为大家分享了有关“Android开发中日期工具类DateUtil完整实例”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1296749.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复