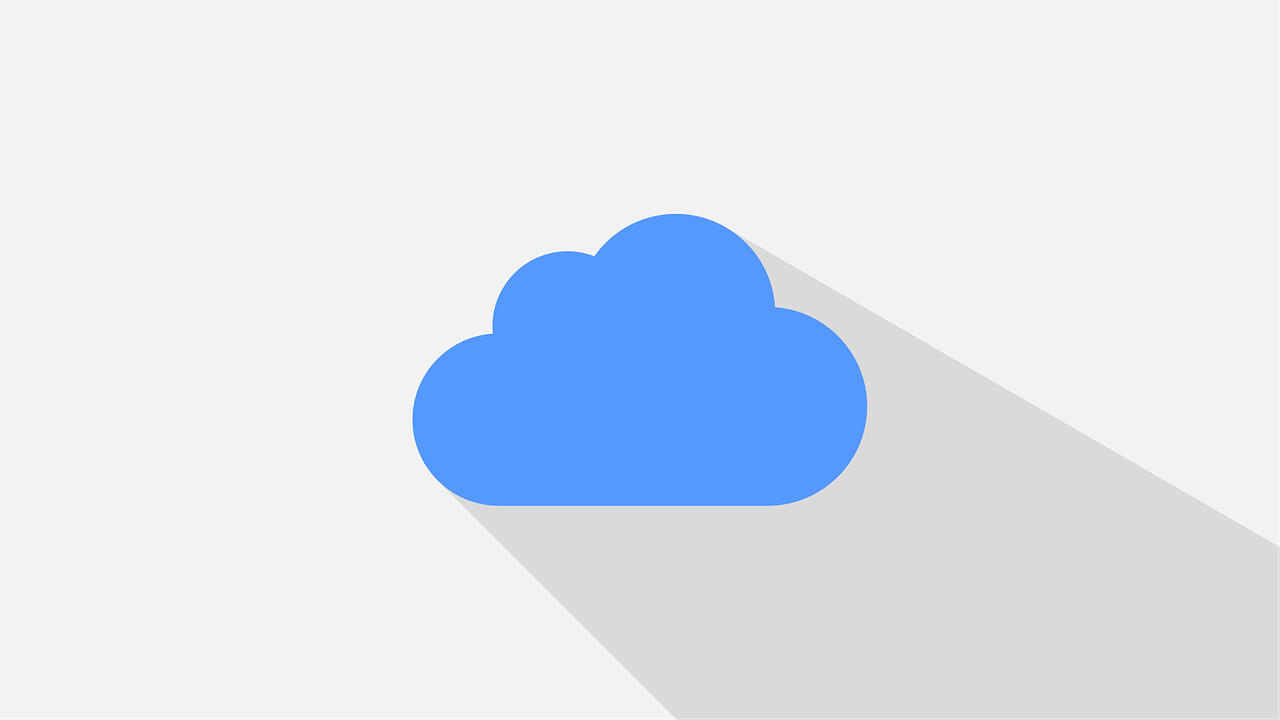
strcmp 是 C 标准库中用于比较两个字符串的函数,定义在<string.h>
头文件中,它用于按字典顺序比较两个以 null 结尾的字符串,并返回一个整数结果。
函数原型
int strcmp(const char *str1, const char *str2);
参数
str1
:指向第一个要比较的字符串的指针。
str2
:指向第二个要比较的字符串的指针。
返回值
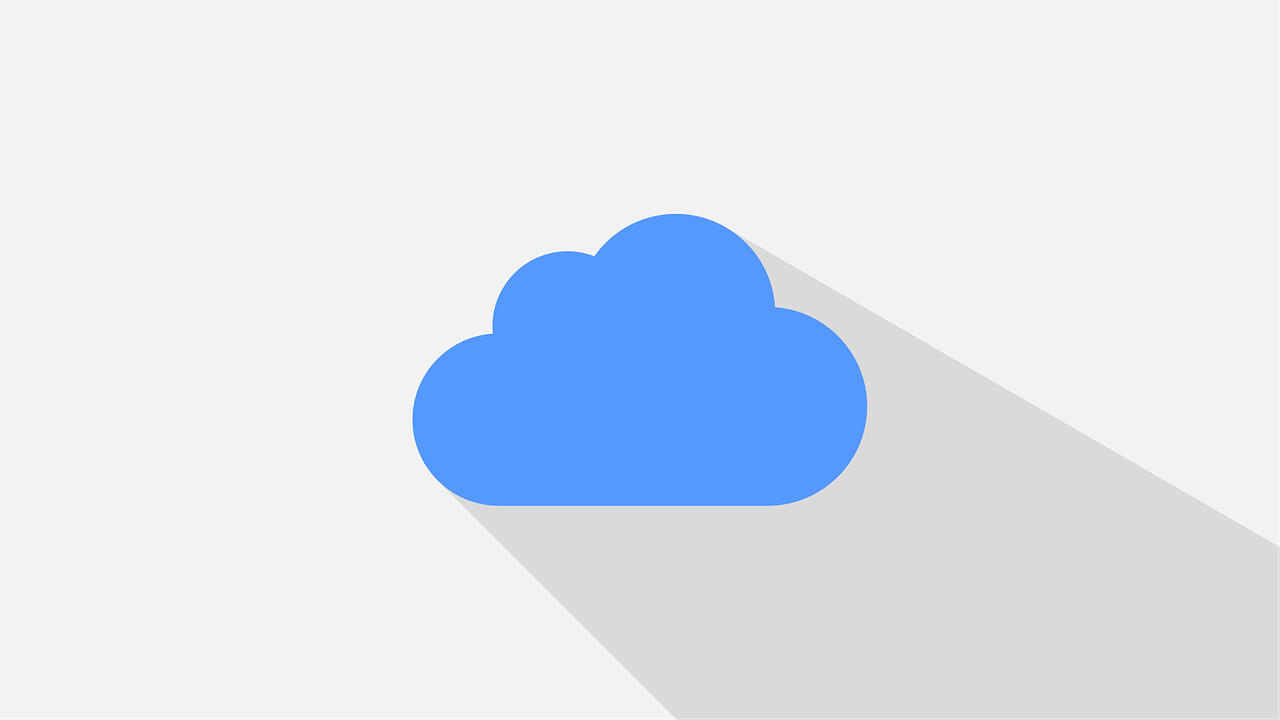
如果str1
小于str2
,返回负数。
如果str1
等于str2
,返回零。
如果str1
大于str2
,返回正数。
使用示例
以下是一个简单的例子,演示如何使用strcmp
函数来比较两个字符串:
#include <stdio.h> #include <string.h> int main() { char str1[] = "apple"; char str2[] = "banana"; int result = strcmp(str1, str2); if (result < 0) { printf("'%s' is less than '%s' ", str1, str2); } else if (result > 0) { printf("'%s' is greater than '%s' ", str1, str2); } else { printf("'%s' is equal to '%s' ", str1, str2); } return 0; }
在这个例子中,strcmp
函数将比较str1
和str2
,并根据比较结果输出相应的信息。
注意事项
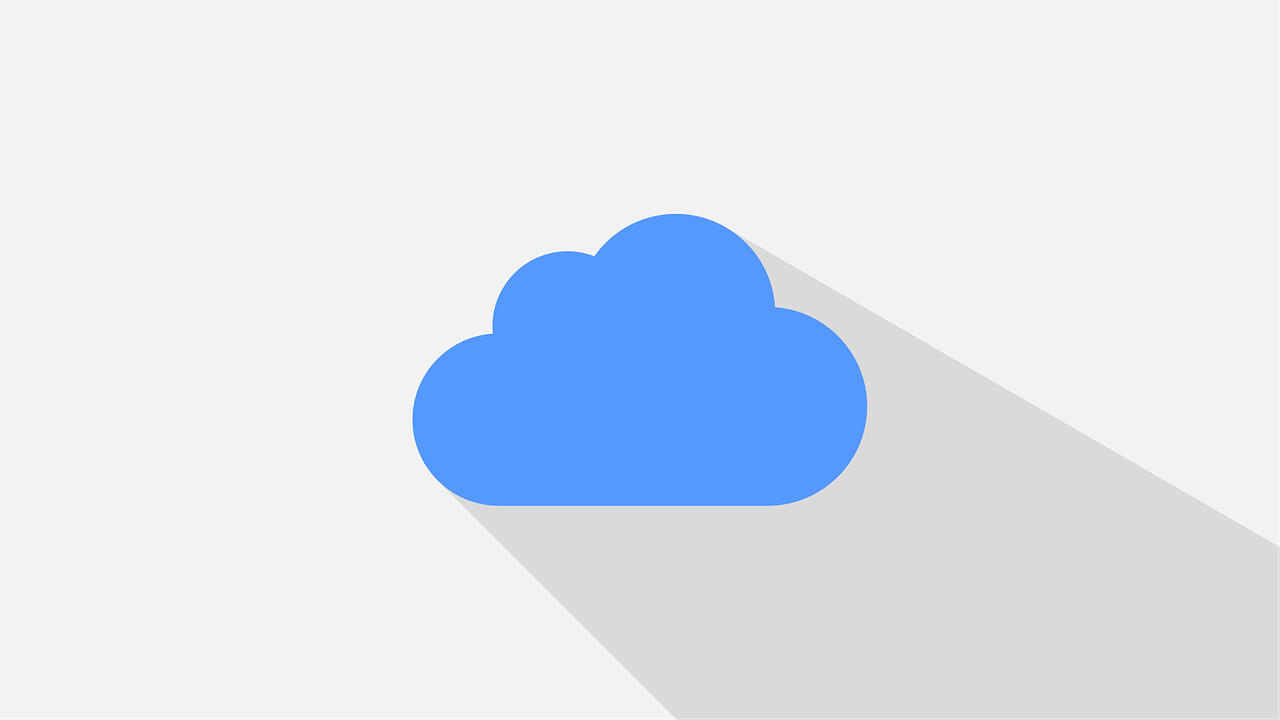
1、区分大小写:strcmp
函数是区分大小写的。"Apple" 和 "apple" 被认为是不同的字符串。
2、空字符处理:如果一个字符串是另一个字符串的前缀,则较短的字符串将被认为较小(因为strcmp
会逐个字符进行比较,直到遇到不同字符或字符串结束)。
3、安全性:由于strcmp
不会检查输入字符串的长度,因此需要确保传入的字符串是有效的,并且以 null 否则可能会导致未定义的行为。
相关问答FAQs
问题1:如何忽略大小写比较字符串?
解答:C 标准库中没有直接提供忽略大小写的字符串比较函数,但是可以通过将字符串转换为统一的大小写形式后再进行比较来实现这一点,可以将两个字符串都转换为小写或大写,然后使用strcmp
进行比较,以下是一个示例代码:
#include <stdio.h> #include <string.h> #include <ctype.h> // 将字符串转换为小写 void to_lowercase(char *str) { for (; *str; ++str) { *str = tolower((unsigned char)*str); } } int main() { char str1[] = "Apple"; char str2[] = "apple"; to_lowercase(str1); to_lowercase(str2); int result = strcmp(str1, str2); if (result < 0) { printf("'%s' is less than '%s' ", str1, str2); } else if (result > 0) { printf("'%s' is greater than '%s' ", str1, str2); } else { printf("'%s' is equal to '%s' ", str1, str2); } return 0; }
在这个例子中,我们首先定义了一个to_lowercase
函数,将字符串中的每个字符转换为小写,然后我们将两个字符串都转换为小写后,再使用strcmp
进行比较。
问题2:如何在排序中使用strcmp
?
解答:在排序算法中,可以使用strcmp
作为比较函数来对字符串数组进行排序,以下是一个使用冒泡排序对字符串数组进行排序的示例:
#include <stdio.h> #include <string.h> // 交换两个字符串 void swap(chara, charb) { char *temp = *a; *a = *b; *b = temp; } // 冒泡排序 void bubble_sort(char *arr[], int n) { for (int i = 0; i < n 1; ++i) { for (int j = 0; j < n i 1; ++j) { if (strcmp(arr[j], arr[j + 1]) > 0) { swap(&arr[j], &arr[j + 1]); } } } } int main() { char *words[] = {"banana", "apple", "cherry", "date"}; int n = sizeof(words) / sizeof(words[0]); bubble_sort(words, n); printf("Sorted words: "); for (int i = 0; i < n; ++i) { printf("%s ", words[i]); } return 0; }
在这个例子中,我们定义了一个swap
函数来交换两个字符串指针的值,并使用冒泡排序算法对字符串数组进行排序,在排序过程中,使用strcmp
作为比较函数来确定两个字符串的顺序。
以上就是关于“strcmp”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1296478.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复