Android 布局实例
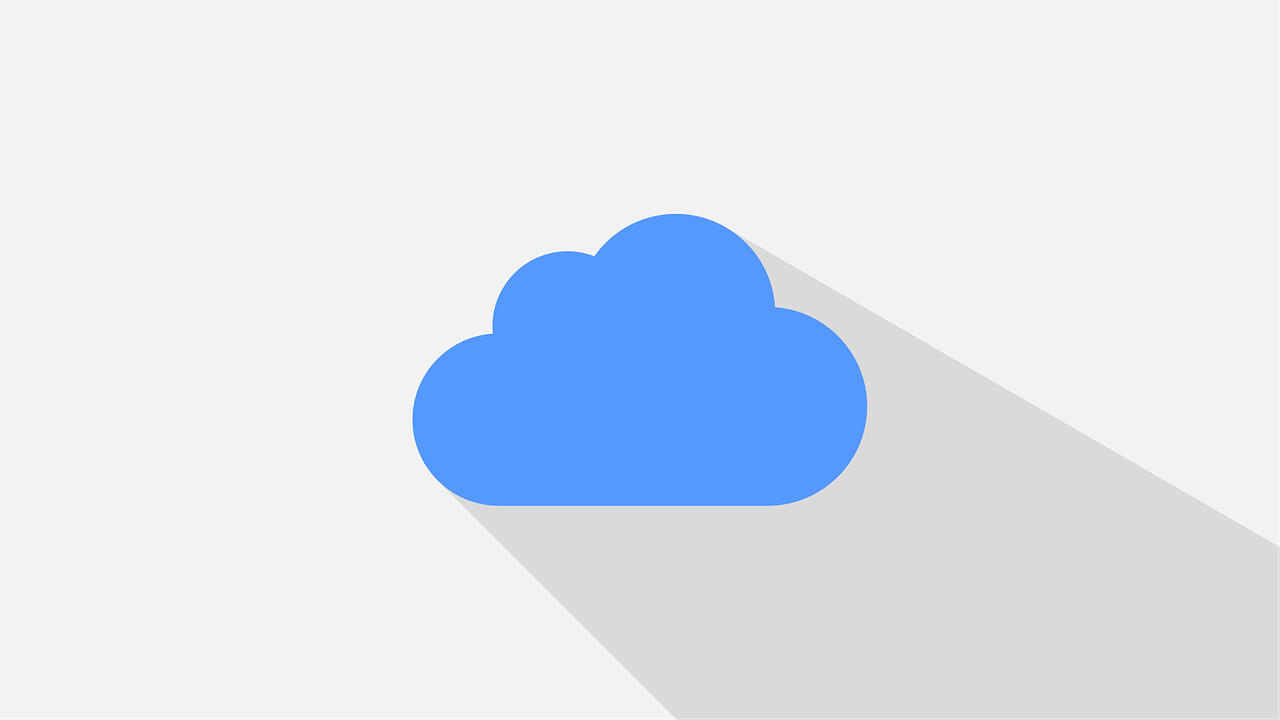
在Android开发中,布局是构建用户界面的基础,通过合理利用不同的布局管理器,可以实现各种复杂的UI设计,本文将详细介绍几种常见的布局方式,并通过实例代码展示其具体应用。
一、线性布局(LinearLayout)
水平排列
实现效果:
五个按钮水平排列。
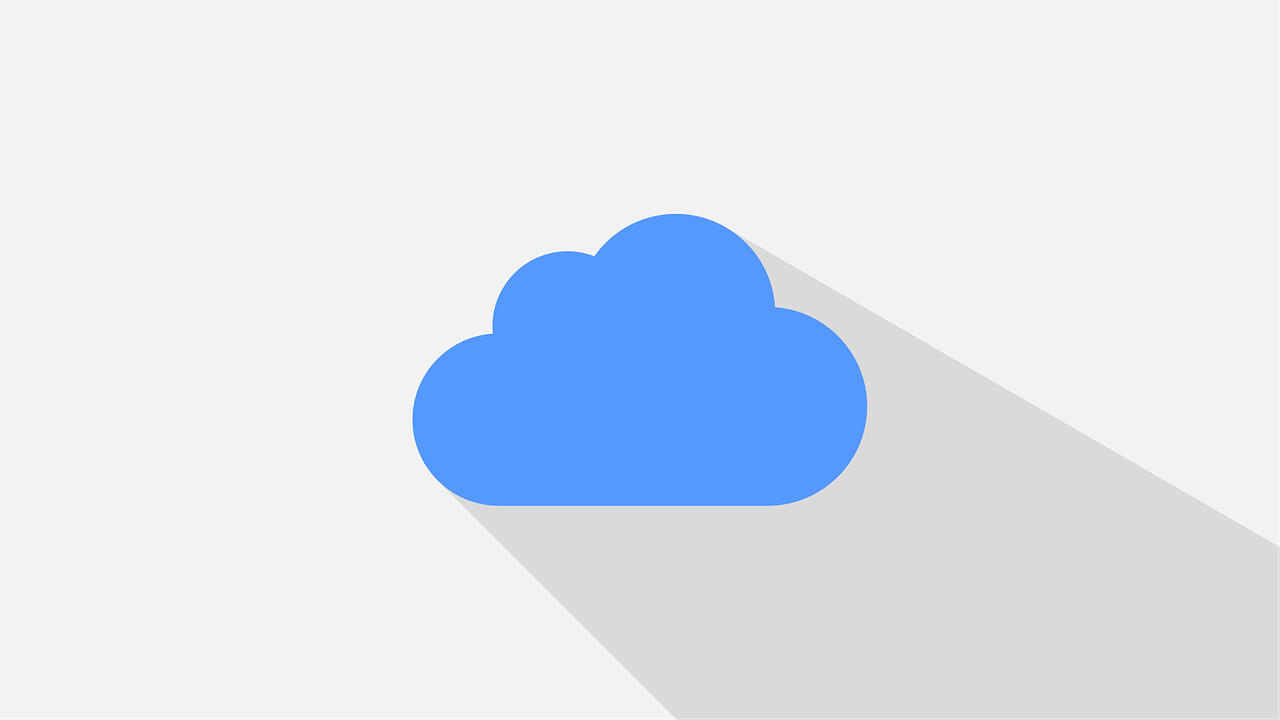
XML代码:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 2" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 3" /> <Button android:id="@+id/button4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 4" /> <Button android:id="@+id/button5" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 5" /> </LinearLayout>
垂直排列
实现效果:
五个按钮垂直排列。
XML代码:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 2" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 3" /> <Button android:id="@+id/button4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 4" /> <Button android:id="@+id/button5" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 5" /> </LinearLayout>
权重布局
实现效果:
两个按钮按比例分配宽度,比例为2:1。
XML代码:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <Button android:id="@+id/button1" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="2" android:text="Button 1" /> <Button android:id="@+id/button2" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:text="Button 2" /> </LinearLayout>
二、相对布局(RelativeLayout)
实现效果:
一个TextView居中显示,另一个TextView位于其下方。
XML代码:
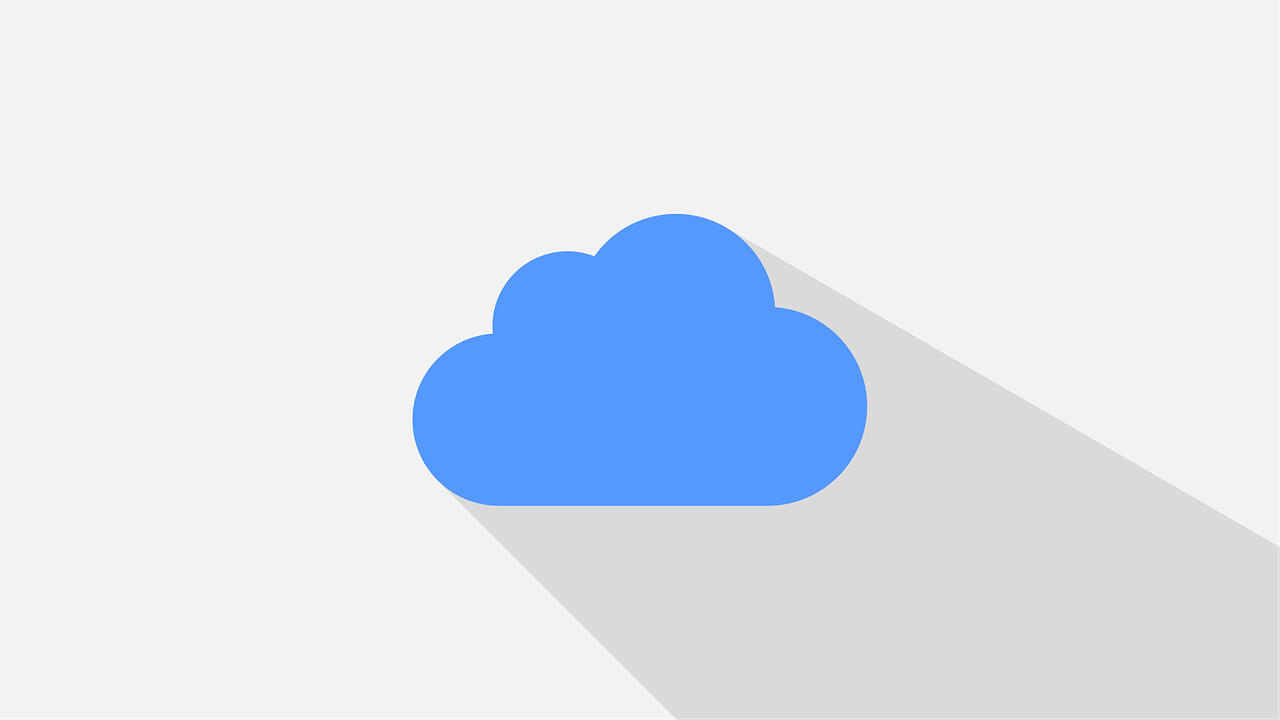
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="TextView 1" android:layout_centerInParent="true"/> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="TextView 2" android:layout_below="@id/textView1"/> </RelativeLayout>
三、帧布局(FrameLayout)
实现效果:
两个按钮重叠显示,其中一个按钮覆盖在另一个按钮之上。
XML代码:
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1"/> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 2"/> </FrameLayout>
四、表格布局(TableLayout)
实现效果:
两行两列的表格布局,其中包含四个按钮。
XML代码:
<?xml version="1.0" encoding="utf-8"?> <TableLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TableRow> <Button android:text="Button 1"/> <Button android:text="Button 2"/> </TableRow> <TableRow> <Button android:text="Button 3"/> <Button android:text="Button 4"/> </TableRow> </TableLayout>
五、绝对布局(AbsoluteLayout)
实现效果:
两个文本视图按照指定的x和y坐标位置显示。
XML代码:
<?xml version="1.0" encoding="utf-8"?> <AbsoluteLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <!--Creating a text view for heading on below line--> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_x="90dp" android:layout_y="350dp" android:text="Heading in AbsoluteLayout" android:textColor="#FF0000" android:textSize="20sp"/> <!-creating a text view for sub heading on below line--> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_x="150dp" android:layout_y="400dp" android:text="Sub Heading in AbsoluteLayout" android:textColor="#00FF00" android:textSize="18sp"/></AbsoluteLayout>
以上就是关于“android布局实例”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1295210.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复