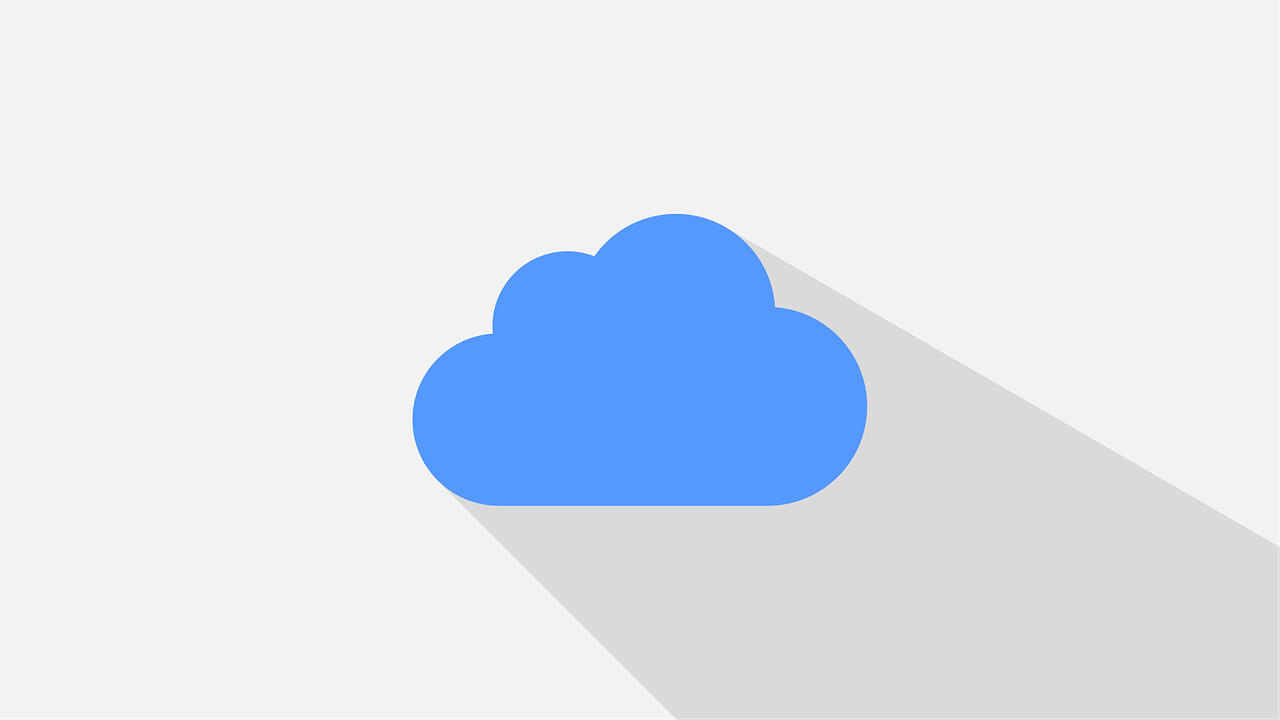
一、引言
Android开发中,布局(Layout)是构建用户界面的基础,布局定义了应用中各个视图组件(如按钮、文本框等)的排列方式和位置,本文将详细介绍Android中的布局API,涵盖其基本原理、常用布局类型及具体实现方法。
二、布局
布局管理器
为了让UI组件在不同设备上都能良好运行,Android提供了多种布局管理器,这些管理器负责调整组件的大小和位置,主要布局管理器包括:
LinearLayout: 线性布局,按行或列排列组件。
RelativeLayout: 相对布局,根据相对位置排列组件。
ConstraintLayout: 约束布局,通过设置约束条件更灵活地排列组件。
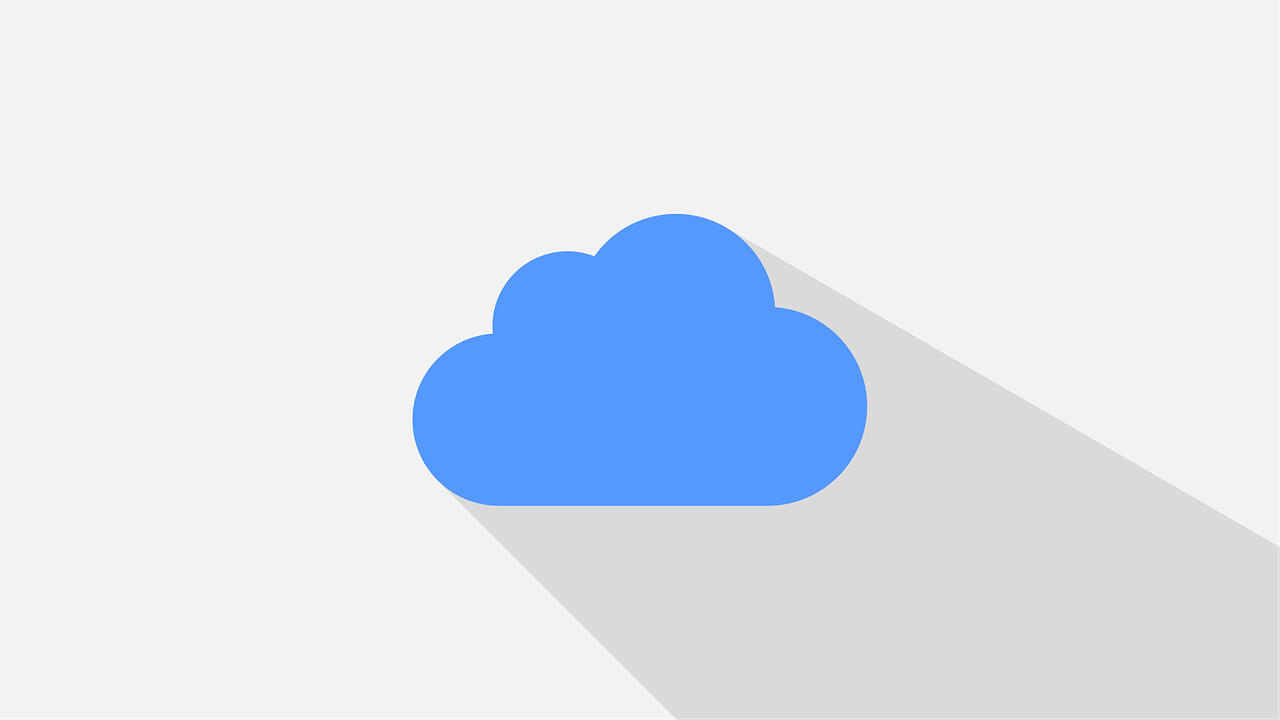
FrameLayout: 帧布局,所有组件叠加在一起。
TableLayout: 表格布局,以行和列的形式排列组件。
GridLayout: 网格布局,按网格排列组件。
布局文件解析
每当调用Activity.setContentView(@LayoutRes int layoutResID)
方法或通过LayoutInflater
对象加载布局时,XML文件中定义的布局会被解析并实例化为对应的View对象,每个大写的XML节点对应一个View对象,这些对象在整个Activity或Fragment的生命周期中都是UI层级的一部分。
三、常用布局类型及实现方法
LinearLayout
1.1
LinearLayout是一种线性布局,可以垂直或水平排列子视图,它通过设置android:orientation
属性来决定排列方向。
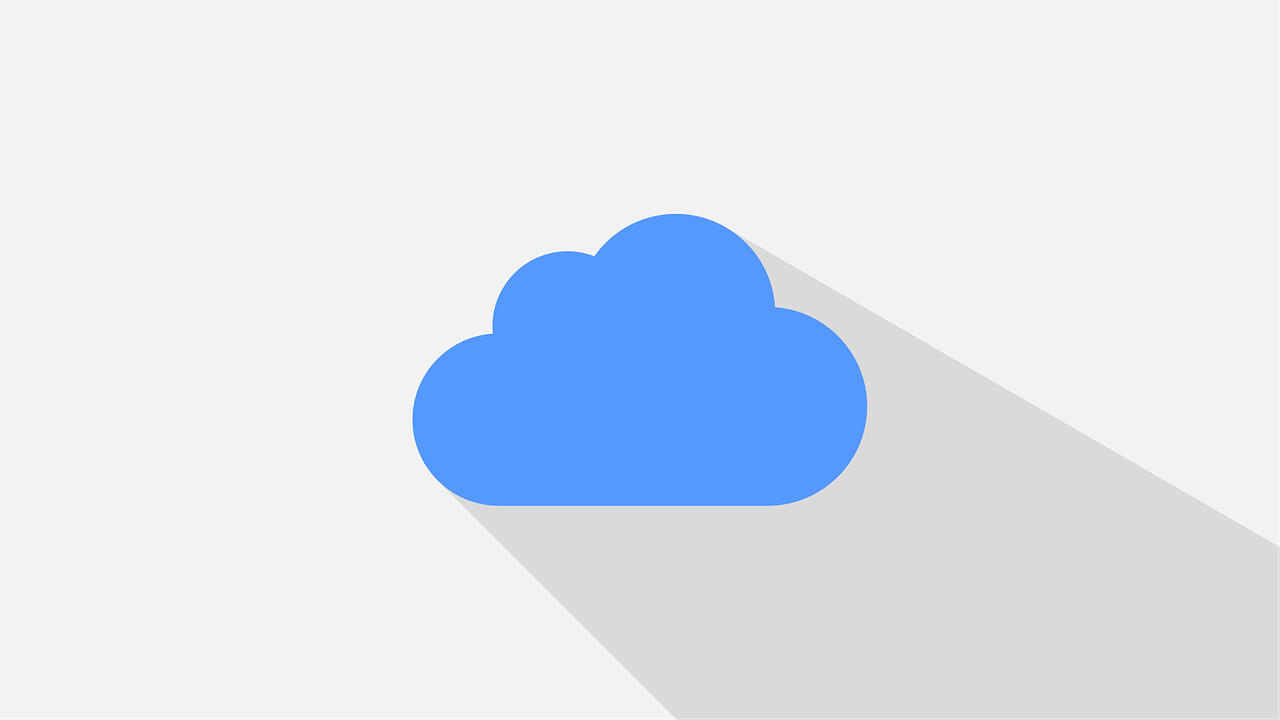
1.2 示例代码
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 2"/> </LinearLayout>
RelativeLayout
2.1
RelativeLayout允许子视图相对于其他视图或父布局进行定位,常用的属性包括android:layout_below
、android:layout_toRightOf
等。
2.2 示例代码
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/button1" android:text="Button 2"/> </RelativeLayout>
ConstraintLayout
3.1
ConstraintLayout是一种灵活的布局方式,通过设置约束条件来定位子视图,它可以替代RelativeLayout,并且在某些情况下更高效。
3.2 示例代码
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1" app:layout_constraintTop_toTopOf="parent" app:layout_constraintStart_toStartOf="parent"/> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 2" app:layout_constraintTop_toBottomOf="@id/button1" app:layout_constraintStart_toStartOf="parent"/> </androidx.constraintlayout.widget.ConstraintLayout>
FrameLayout
4.1
FrameLayout将所有子视图叠加在一起,通常用于放置单个视图或作为容器使用。
4.2 示例代码
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button 1"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:text="Button 2"/> </FrameLayout>
TableLayout
5.1
TableLayout以行和列的形式排列组件,适用于创建表格结构。
5.2 示例代码
<?xml version="1.0" encoding="utf-8"?> <TableLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TableRow> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Cell 1"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Cell 2"/> </TableRow> <TableRow> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Cell 3"/> <TextView> android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Cell 4"/> </TableRow> </TableLayout>
GridLayout
6.1
GridLayout按网格形式排列组件,适合创建复杂的网格结构。
6.2 示例代码
<?xml version="1.0" encoding="utf-8"?> <GridLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:columnCount="2"> <Button android:text="Button 1"/> <Button android:text="Button 2"/> <Button android:text="Button 3"/> <Button android:text="Button 4"/> </GridLayout>
四、高级布局技巧与性能优化
优化布局层次结构
减少不必要的嵌套,尽量使用扁平化的布局结构,以提高性能,可以使用ConstraintLayout替代多层嵌套的RelativeLayout。
2.通过<include>
重复使用布局
对于重复使用的布局部分,可以使用<include>
标签进行复用,减少冗余代码。
<include layout="@layout/common_view"/>
按需加载视图
在RecyclerView或ListView中,仅加载当前可见的视图项,避免一次性加载大量数据导致的性能问题。
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.ViewHolder> { // ... other methods ... @Override public void onBindViewHolder(ViewHolder holder, int position) { // Inflate view and bind data here } }
自定义View组件
有时需要创建自定义的视图组件,可以通过继承现有的View类来实现。
public class MyCustomView extends View { private Paint paint; public MyCustomView(Context context) { super(context); init(); } private void init() { paint = new Paint(); } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); // Custom drawing code here } }
然后将其添加到布局文件中:
<com.example.MyCustomView android:layout_width="wrap_content" android:layout_height="wrap_content"/>
响应式布局与动态颜色
在Android 12中引入了新的API,使得Widget可以实现圆角、动态颜色等效果,使用系统参数设置圆角:
<shape android:shape="rectangle"> <corners android:radius="@android:dimen/system_app_widget_background_radius"/> </shape>
动态颜色:
<LinearLayout android:theme="@android:style/Theme.DeviceDefault.DayNight" android:background="?android:attr/colorBackground"> <ImageView android:tint="?android:attr/colorAccent"/> </LinearLayout>
响应式布局:根据Widget尺寸自动调整布局:
val viewMapping: Map<SizeF, RemoteViews> = mapOf(SizeF(180.0f, 110.0f) to remoteViewSmall, SizeF(300.0f, 220.0f) to remoteViewMedium, SizeF(450.0f, 330.0f) to remoteViewLarge)
五、上文归纳
本文详细介绍了Android中的布局API,包括常用布局类型及其实现方法,并探讨了高级布局技巧与性能优化策略,通过合理使用布局管理器和优化技术,开发者可以创建出高效且美观的用户界面,希望本文能帮助您更好地理解和应用Android布局API,提升应用开发水平。
各位小伙伴们,我刚刚为大家分享了有关“android布局api”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1294381.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复