浮动窗口(Floating Window)是一种在网页上显示的可拖动、可调整大小的弹出窗口,它通常用于提供额外的信息或功能,而不会干扰用户对主页面内容的查看,使用JavaScript可以方便地创建和管理浮动窗口。
基本概念
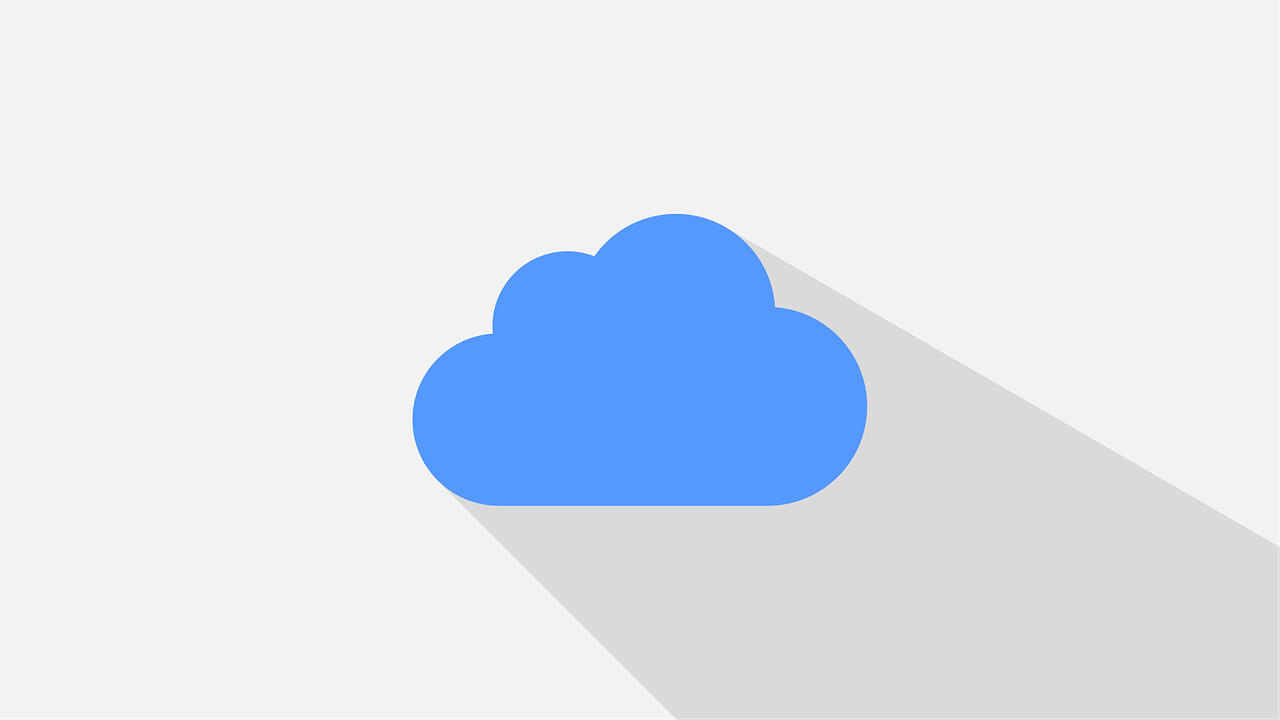
浮动窗口的基本概念包括:
位置:窗口在屏幕上的位置,可以通过坐标(x, y)来表示。
大小:窗口的宽度和高度。
:窗口中显示的内容,可以是HTML、文本或其他元素。
拖动:允许用户通过拖动窗口标题栏来移动窗口。
调整大小:允许用户通过拖动窗口边缘来改变窗口的大小。
HTML结构
我们需要一个基本的HTML结构来容纳浮动窗口。
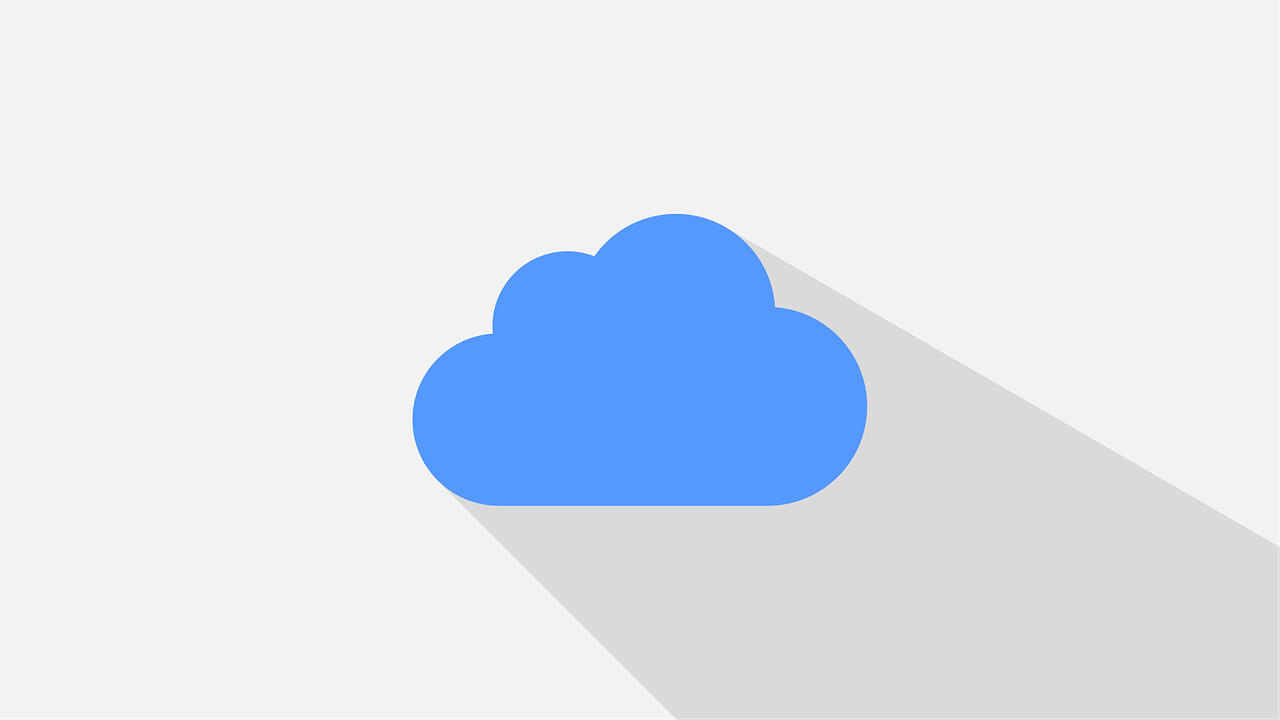
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>浮动窗口示例</title> <style> /* 样式定义 */ #floatingWindow { position: absolute; width: 300px; height: 200px; background-color: white; border: 1px solid #ccc; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); z-index: 1000; } #floatingWindowHeader { cursor: move; background-color: #f1f1f1; padding: 10px; border-bottom: 1px solid #ccc; } #floatingWindowContent { padding: 10px; } </style> </head> <body> <div id="floatingWindow"> <div id="floatingWindowHeader">浮动窗口</div> <div id="floatingWindowContent"> <p>这是一个浮动窗口。</p> </div> </div> <script src="floatingWindow.js"></script> </body> </html>
JavaScript实现
我们编写JavaScript代码来实现浮动窗口的功能。
// floatingWindow.js document.addEventListener('DOMContentLoaded', function() { const floatingWindow = document.getElementById('floatingWindow'); const header = document.getElementById('floatingWindowHeader'); let isDragging = false; let offsetX, offsetY; header.addEventListener('mousedown', function(e) { isDragging = true; offsetX = e.clientX floatingWindow.offsetLeft; offsetY = e.clientY floatingWindow.offsetTop; document.addEventListener('mousemove', onMouseMove); document.addEventListener('mouseup', onMouseUp); }); function onMouseMove(e) { if (isDragging) { floatingWindow.style.left =${e.clientX offsetX}px
; floatingWindow.style.top =${e.clientY offsetY}px
; } } function onMouseUp() { isDragging = false; document.removeEventListener('mousemove', onMouseMove); document.removeEventListener('mouseup', onMouseUp); } });
添加调整大小功能
为了增加调整大小的功能,我们可以在窗口的右下角添加一个可拖动的手柄。
<!-在浮动窗口内容部分添加一个调整大小的手柄 --> <div id="floatingWindowResizeHandle" style="position: absolute; bottom: 0; right: 0; width: 10px; height: 10px; background-color: #ccc; cursor: se-resize;"></div>
// floatingWindow.js 更新部分 document.addEventListener('DOMContentLoaded', function() { const floatingWindow = document.getElementById('floatingWindow'); const header = document.getElementById('floatingWindowHeader'); const resizeHandle = document.getElementById('floatingWindowResizeHandle'); let isDragging = false; let isResizing = false; let offsetX, offsetY, startWidth, startHeight; header.addEventListener('mousedown', function(e) { isDragging = true; offsetX = e.clientX floatingWindow.offsetLeft; offsetY = e.clientY floatingWindow.offsetTop; document.addEventListener('mousemove', onMouseMove); document.addEventListener('mouseup', onMouseUp); }); resizeHandle.addEventListener('mousedown', function(e) { isResizing = true; startWidth = floatingWindow.offsetWidth; startHeight = floatingWindow.offsetHeight; offsetX = e.clientX startWidth; offsetY = e.clientY startHeight; document.addEventListener('mousemove', onMouseMove); document.addEventListener('mouseup', onMouseUp); }); function onMouseMove(e) { if (isDragging) { floatingWindow.style.left =${e.clientX offsetX}px
; floatingWindow.style.top =${e.clientY offsetY}px
; } else if (isResizing) { const newWidth = Math.max(100, e.clientX offsetX); // 最小宽度为100px const newHeight = Math.max(100, e.clientY offsetY); // 最小高度为100px floatingWindow.style.width =${newWidth}px
; floatingWindow.style.height =${newHeight}px
; } } function onMouseUp() { isDragging = false; isResizing = false; document.removeEventListener('mousemove', onMouseMove); document.removeEventListener('mouseup', onMouseUp); } });
进一步优化与扩展
为了使浮动窗口更加实用和美观,可以考虑以下优化和扩展:
动画效果:添加平滑的拖动和调整大小动画。
关闭按钮:添加一个关闭按钮,使用户可以手动关闭浮动窗口。
模态背景:当浮动窗口打开时,添加一个半透明的背景,防止用户与主页面的其他内容交互。
事件回调:提供回调函数,以便在窗口打开、关闭、拖动和调整大小时执行特定操作。
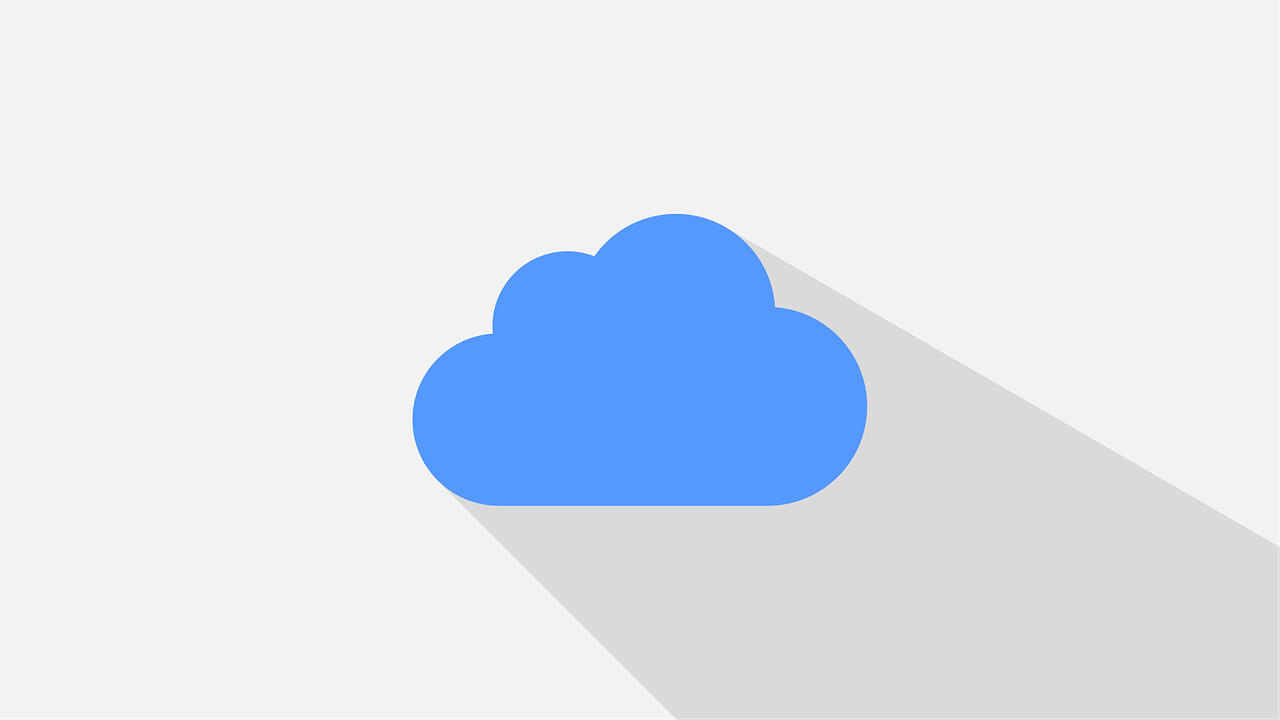
响应式设计:确保浮动窗口在不同设备和屏幕尺寸下都能良好显示。
完整示例代码
以下是一个完整的示例代码,包含上述所有功能:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>浮动窗口示例</title> <style> #floatingWindow { position: absolute; width: 300px; height: 200px; background-color: white; border: 1px solid #ccc; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); z-index: 1000; } #floatingWindowHeader { cursor: move; background-color: #f1f1f1; padding: 10px; border-bottom: 1px solid #ccc; } #floatingWindowContent { padding: 10px; } #floatingWindowResizeHandle { position: absolute; bottom: 0; right: 0; width: 10px; height: 10px; background-color: #ccc; cursor: se-resize; } </style> </head> <body> <div id="floatingWindow"> <div id="floatingWindowHeader">浮动窗口</div> <div id="floatingWindowContent"> <p>这是一个浮动窗口。</p> </div> <div id="floatingWindowResizeHandle"></div> </div> <script> document.addEventListener('DOMContentLoaded', function() { const floatingWindow = document.getElementById('floatingWindow'); const header = document.getElementById('floatingWindowHeader'); const resizeHandle = document.getElementById('floatingWindowResizeHandle'); let isDragging = false; let isResizing = false; let offsetX, offsetY, startWidth, startHeight; header.addEventListener('mousedown', function(e) { isDragging = true; offsetX = e.clientX floatingWindow.offsetLeft; offsetY = e.clientY floatingWindow.offsetTop; document.addEventListener('mousemove', onMouseMove); document.addEventListener('mouseup', onMouseUp); }); resizeHandle.addEventListener('mousedown', function(e) { isResizing = true; startWidth = floatingWindow.offsetWidth; startHeight = floatingWindow.offsetHeight; offsetX = e.clientX startWidth; offsetY = e.clientY startHeight; document.addEventListener('mousemove', onMouseMove); document.addEventListener('mouseup', onMouseUp); }); function onMouseMove(e) { if (isDragging) { floatingWindow.style.left =${e.clientX offsetX}px
; floatingWindow.style.top =${e.clientY offsetY}px
; } else if (isResizing) { const newWidth = Math.max(100, e.clientX offsetX); // 最小宽度为100px const newHeight = Math.max(100, e.clientY offsetY); // 最小高度为100px floatingWindow.style.width =${newWidth}px
; floatingWindow.style.height =${newHeight}px
; } } function onMouseUp() { isDragging = false; isResizing = false; document.removeEventListener('mousemove', onMouseMove); document.removeEventListener('mouseup', onMouseUp); } }); </script> </body> </html>
小伙伴们,上文介绍了“浮动窗口的js”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1294206.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复