Android工具类
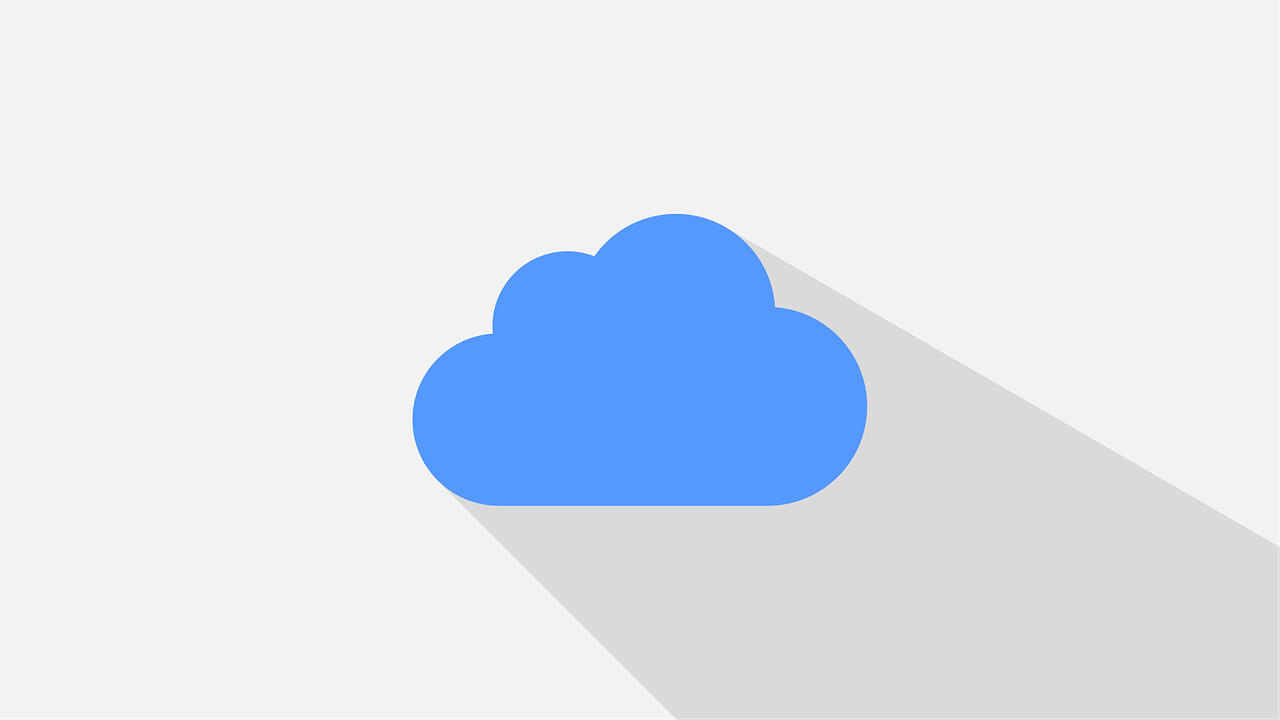
一、背景描述
Android开发中,工具类是提升开发效率和代码复用性的重要手段,本文将详细介绍几个常用的Android工具类,包括HttpUtils、DownloadManagerPro、ShellUtils、PackageUtils、PreferencesUtils、JSONUtils、FileUtils等,这些工具类涵盖了网络请求、下载管理、系统操作、文件处理等多个方面,通过具体的代码示例展示如何使用这些工具类来简化开发任务。
二、工具类介绍
HttpUtils
1.1 功能
HttpUtils是一个用于简化HTTP请求的工具类,支持GET和POST请求,并提供了设置超时、用户代理等功能。
1.2 方法
httpGet(HttpRequest request)
: 执行GET请求,返回HttpResponse。
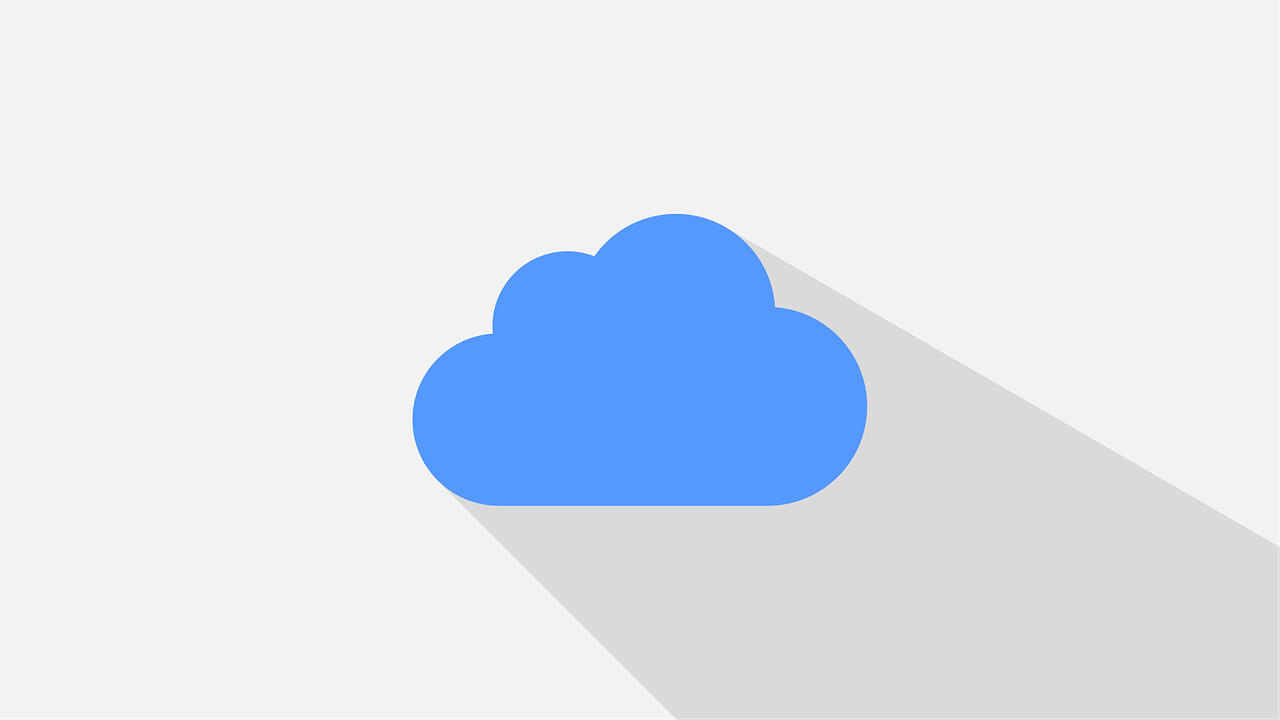
httpPost(HttpRequest request)
: 执行POST请求,返回HttpResponse。
httpGetString(String httpUrl)
: 简单的GET请求,返回字符串结果。
1.3 示例
// 创建HttpRequest对象 HttpRequest request = new HttpRequest(); request.setUrl("https://api.example.com/data"); request.setTimeout(5000); request.setUserAgent("MyApp"); // 执行GET请求 HttpResponse response = HttpUtils.httpGet(request); String result = response.getContent();
2. DownloadManagerPro
2.1 功能
DownloadManagerPro是对Android系统下载管理的增强工具类,用于获取下载状态、进度等信息。
2.2 方法
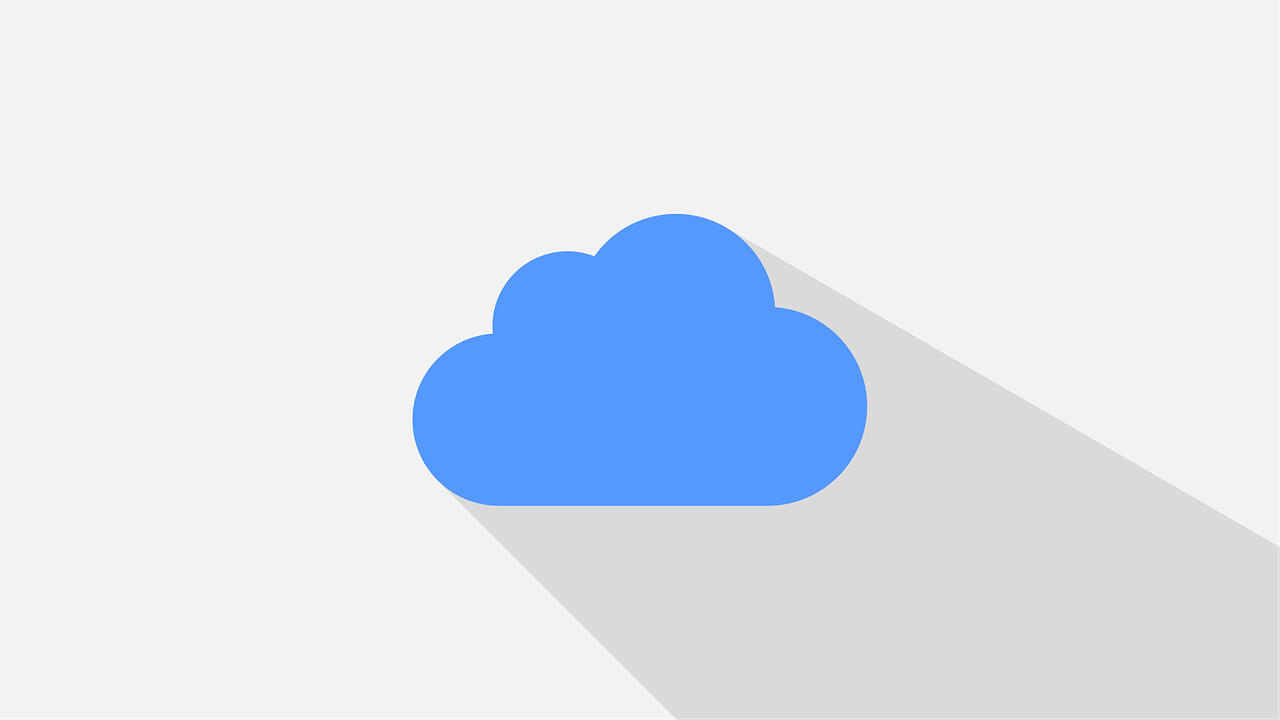
getStatusById(long id)
: 根据下载ID获取下载状态。
getDownloadBytes(long id)
: 获取下载进度信息。
getBytesAndStatus(long id)
: 获取下载进度和状态。
getFileName(long id)
: 获取下载文件路径。
getUri(long id)
: 获取下载URI。
getReason(long id)
: 获取下载失败或暂停原因。
getPausedReason(long id)
: 获取下载暂停原因。
getErrorCode(long id)
: 获取下载错误码。
2.3 示例
// 获取下载状态 int status = DownloadManagerPro.getStatusById(downloadId); // 获取下载进度信息 long[] bytesInfo = DownloadManagerPro.getDownloadBytes(downloadId);
ShellUtils
3.1 功能
ShellUtils是一个用于在Android设备上执行shell命令的工具类,可以检查root权限并在shell环境下执行命令。
3.2 方法
checkRootPermission()
: 检查设备是否具有root权限。
execCommand(String[] commands, boolean isRoot, boolean isNeedResultMsg)
: 在shell环境下执行命令。
execCommand(String command, boolean isRoot)
: 在shell环境下执行命令。
3.3 示例
// 检查root权限 boolean isRoot = ShellUtils.checkRootPermission(); if (isRoot) { // 执行命令 ShellUtils.execCommand(new String[]{"ls", "/"}, true, true); }
PackageUtils
4.1 功能
PackageUtils是一个用于安装、卸载应用以及判断应用是否为系统应用的工具类。
4.2 方法
install(Context context, String apkPath)
: 安装APK文件。
uninstall(Context context, String packageName)
: 卸载应用。
isSystemApplication(Context context, String packageName)
: 判断应用是否为系统应用。
4.3 示例
// 安装APK PackageUtils.install(context, "path/to/apk"); // 卸载应用 PackageUtils.uninstall(context, "com.example.app"); // 判断是否为系统应用 boolean isSystemApp = PackageUtils.isSystemApplication(context, "com.example.app");
PreferencesUtils
5.1 功能
PreferencesUtils是一个用于简化SharedPreferences操作的工具类,提供保存和读取各种数据类型的方法。
5.2 方法
putString(Context context, String key, String value)
: 保存字符串。
putInt(Context context, String key, int value)
: 保存整数。
getString(Context context, String key, String defaultValue)
: 读取字符串。
getInt(Context context, String key, int defaultValue)
: 读取整数。
deleteKey(Context context, String key)
: 删除键值对。
clear(Context context)
: 清除所有数据。
5.3 示例
// 保存数据 PreferencesUtils.putString(context, "username", "admin"); PreferencesUtils.putInt(context, "age", 25); // 读取数据 String username = PreferencesUtils.getString(context, "username", "default"); int age = PreferencesUtils.getInt(context, "age", 0);
JSONUtils
6.1 功能
JSONUtils是一个用于简化JSON解析和生成的工具类,支持从JSONObject和JSONArray中读取数据,并将其转换为相应的Java对象。
6.2 方法
getString(JSONObject jsonObject, String key, String defaultValue)
: 从JSONObject中读取字符串。
getMap(JSONObject jsonObject, String key)
: 从JSONObject中读取Map。
getList(JSONArray jsonArray, Class<T> cls)
: 从JSONArray中读取列表。
parseObject(String jsonData, Class<T> cls)
: 将JSON字符串解析为Java对象。
parseArray(String jsonData, Class<T> cls)
: 将JSON字符串解析为列表。
toJson(Object object)
: 将Java对象转换为JSON字符串。
toJsonPretty(Object object)
: 将Java对象转换为格式化的JSON字符串。
6.3 示例
// 解析JSON字符串为Java对象 User user = JSONUtils.parseObject("{"name":"John", "age":30}", User.class); // 将Java对象转换为JSON字符串 String jsonStr = JSONUtils.toJson(user);
FileUtils
7.1 功能
FileUtils是一个用于简化文件操作的工具类,提供读写文件、复制文件、删除文件等功能。
7.2 方法
readFileToString(String filePath)
: 读取文件内容并返回字符串。
writeFileFromString(String data, String filePath)
: 将字符串写入文件。
copyFile(String srcFilePath, String destFilePath)
: 复制文件。
deleteFile(String filePath)
: 删除文件。
getFileSize(String filePath)
: 获取文件大小。
isFileExists(String filePath)
: 判断文件是否存在。
createDirectory(String dirPath)
: 创建目录。
listFiles(String dirPath)
: 列出目录下的所有文件。
getFileLastModifiedTime(String filePath)
: 获取文件最后修改时间。
getFileExtension(String filePath)
: 获取文件扩展名。
renameFile(String oldPath, String newPath)
: 重命名文件。
openFile(String filePath)
: 打开文件(需要调用系统的文件管理器)。
getFileMimeType(String filePath)
: 获取文件MIME类型。
getExternalStorageDirectory()
: 获取外部存储目录。
getExternalFilesDir()
: 获取外部文件目录。
getExternalCacheDir()
: 获取外部缓存目录。
getPublicPictureDir()
: 获取公共图片目录。
getPublicVideoDir()
: 获取公共视频目录。
getPublicMusicDir()
: 获取公共音乐目录。
getPublicDownloadDir()
: 获取公共下载目录。
getPublicDocumentsDir()
: 获取公共文档目录。
getPublicRingtonesDir()
: 获取公共铃声目录。
getPublicAlarmsDir()
: 获取公共闹钟目录。
getPublicNotificationsDir()
: 获取公共通知目录。
getPublicPodcastsDir()
: 获取公共播客目录。
getExpandableExternalStorageDirectory()
: 获取可扩展的外部存储目录。
getExpandableExternalFilesDir()
: 获取可扩展的外部文件目录。
getExpandableExternalCacheDir()
: 获取可扩展的外部缓存目录。
getExpandablePublicPictureDir()
: 获取可扩展的公共图片目录。
getExpandablePublicVideoDir()
: 获取可扩展的公共视频目录。
getExpandablePublicMusicDir()
: 获取可扩展的公共音乐目录。
getExpandablePublicDownloadDir()
: 获取可扩展的公共下载目录。
getExpandablePublicDocumentsDir()
: 获取可扩展的公共文档目录。
getExpandablePublicRingtonesDir()
: 获取可扩展的公共铃声目录。
getExpandablePublicAlarmsDir()
: 获取可扩展的公共闹钟目录。
getExpandablePublicNotificationsDir()
: 获取可扩展的公共通知目录。
getExpandablePublicPodcastsDir()
: 获取可扩展的公共播客目录。
getExpandablePublicPictureDir()
: 获取可扩展的公共图片目录。
getExpandablePublicVideoDir()
: 获取可扩展的公共视频目录。
getExpandablePublicMusicDir()
: 获取可扩展的公共音乐目录。
getExpandablePublicDownloadDir()
: 获取可扩展的公共下载目录。
getExpandablePublicDocumentsDir()
: 获取可扩展的公共文档目录。
getExpandablePublicRingtonesDir()
: 获取可扩展的公共铃声目录。
getExpandablePublicAlarmsDir()
: 获取可扩展的公共闹钟目录。
getExpandablePublicNotificationsDir()
: 获取可扩展的公共通知目录。
getExpandablePublicPodcastsDir()
: 获取可扩展的公共播客目录。
getExpandableExternalStorageDirectory()
: 获取可扩展的外部存储目录。
getExpandableExternalFilesDir()
: 获取可扩展的外部文件目录。
getExpandableExternalCacheDir()
: 获取可扩展的外部缓存目录。
getExpandablePublicPictureDir()
: 获取可扩展的公共图片目录。
getExpandablePublicVideoDir()
: 获取可扩展的公共视频目录。
getExpandablePublicMusicDir()
: 获取可扩展的公共音乐目录。
getExpandablePublicDownloadDir()
: 获取可扩展的公共下载目录。
getExpandablePublicDocumentsDir()
: 获取可扩展的公共文档目录。
getExpandablePublicRingtonesDir()
: 获取可扩展的公共铃声目录。
getExpandablePublicAlarmsDir()
: 获取可扩展的公共闹钟目录。
getExpandablePublicNotificationsDir()
: 获取可扩展的公共通知目录。
getExpandablePublicPodcastsDir()
: 获取可扩展的公共播客目录。
getExpandablePublicPictureDir()
: 获取可扩展的公共图片目录。
getExpandablePublicVideoDir()
: 获取可扩展的公共视频目录。
getExpandablePublicMusicDir()
: 获取可扩展的公共音乐目录。
getExpandablePublicDownloadDir()
: 获取可扩展的公共下载目录。
getExpandablePublicDocumentsDir()
: 获取可扩展的公共文档目录。
getExpandablePublicRingtonesDir()
: 获取可扩展的公共铃声目录。
getExpandablePublicAlarmsDir()
: 获取可扩展的公共闹钟目录。
getExpandablePublicNotificationsDir()
: 获取可扩展的公共通知目录。
getExpandablePublicPodcastsDir()
: 获取可扩展的公共播客目录。
getExpandableExternalStorageDirectory()
: 获取可扩展的外部存储目录。
getExpandableExternalFilesDir()
: 获取可扩展的外部文件目录。
getExpandableExternalCacheDir()
: 获取可扩展的外部缓存目录。
getExpandablePublicPictureDir()
: 获取可扩展的公共图片目录。
getExpandablePublicVideoDir()
: 获取可扩展的公共视频目录。
getExpandablePublicMusicDir()
: 获取可扩展的公共音乐目录。
getExpandablePublicDownloadDir()
: 获取可扩展的公共下载目录。
getExpandablePublicDocumentsDir()
: 获取可扩展的公共文档目录。
getExpandablePublicRingtonesDir()
: 获取可扩展的公共铃声目录。
getExpandablePublicAlarmsDir()
: 获取可扩展的公共闹钟目录。
getExpandablePublicNotificationsDir()
: 获取可扩展的公共通知目录。
getExpandablePublicPodcastsDir()
: 获取可扩展的公共播客目录。
到此,以上就是小编对于“android工具类”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1292438.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复