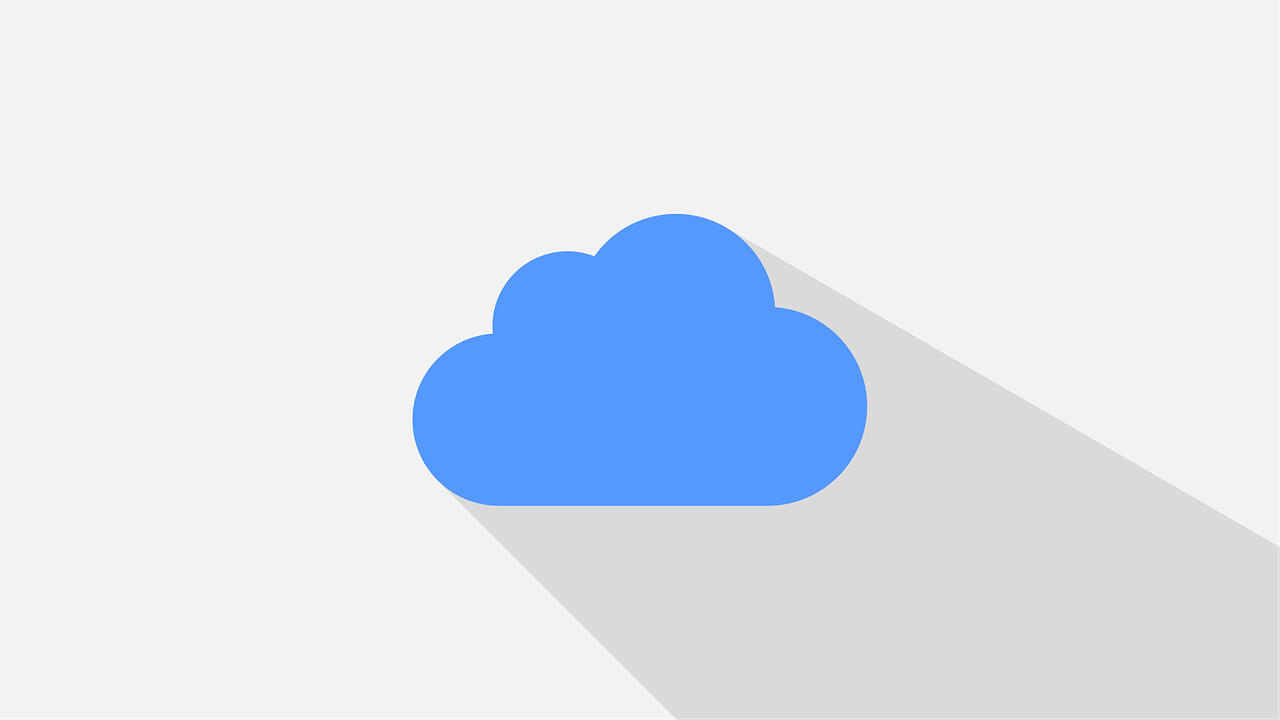
一、背景与概念
Android嵌套滚动
Android的嵌套滚动机制是在Android 5.0(API 21)引入的一种新特性,旨在解决复杂布局中子视图和父视图同时滚动的需求,嵌套滚动打破了传统的事件处理机制,提供了一种逆向的事件传递方式,在具有多个可滚动视图的界面中,当子视图滚动时,父视图也能够相应地滚动,从而实现联动效果,这一机制在实现下拉刷新、联动滚动等高级效果时特别有用。
嵌套滚动的应用场景
嵌套滚动常用于以下场景:
下拉刷新:当用户下拉刷新时,列表顶部的视图也需要移动。
联动滚动:如视差滚动效果,两个或多个视图需要同步滚动。
复杂布局滚动:在一个大的滚动视图中包含多个小的滚动视图,所有视图需要平滑滚动。
通过这些场景,开发者可以实现更加复杂且用户友好的界面交互效果。
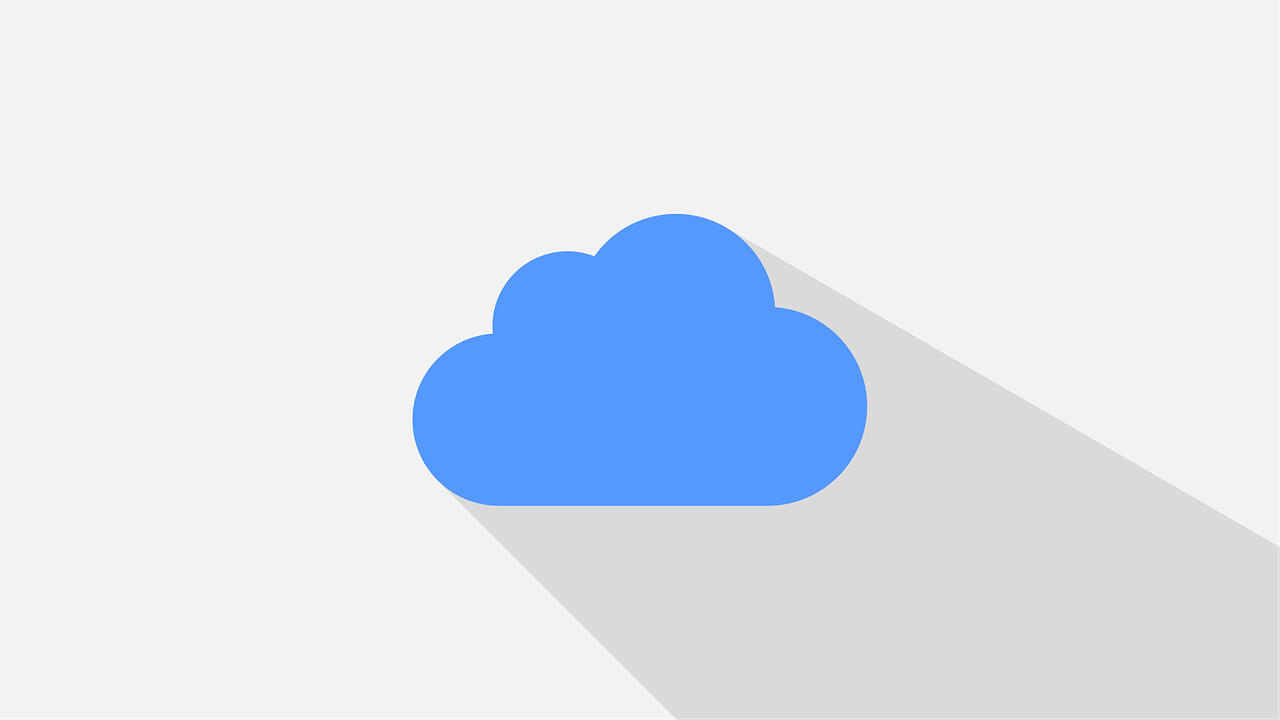
二、NestedScrolling基础
1. NestedScrolling接口介绍
1.1 NestedScrollingChild接口
NestedScrollingChild
接口定义了子视图需要实现的方法,以便支持嵌套滚动,主要方法包括:
setNestedScrollingEnabled(boolean enabled)
: 启用或禁用嵌套滚动。
startNestedScroll(int axes)
: 开始嵌套滚动。
stopNestedScroll()
: 停止嵌套滚动。
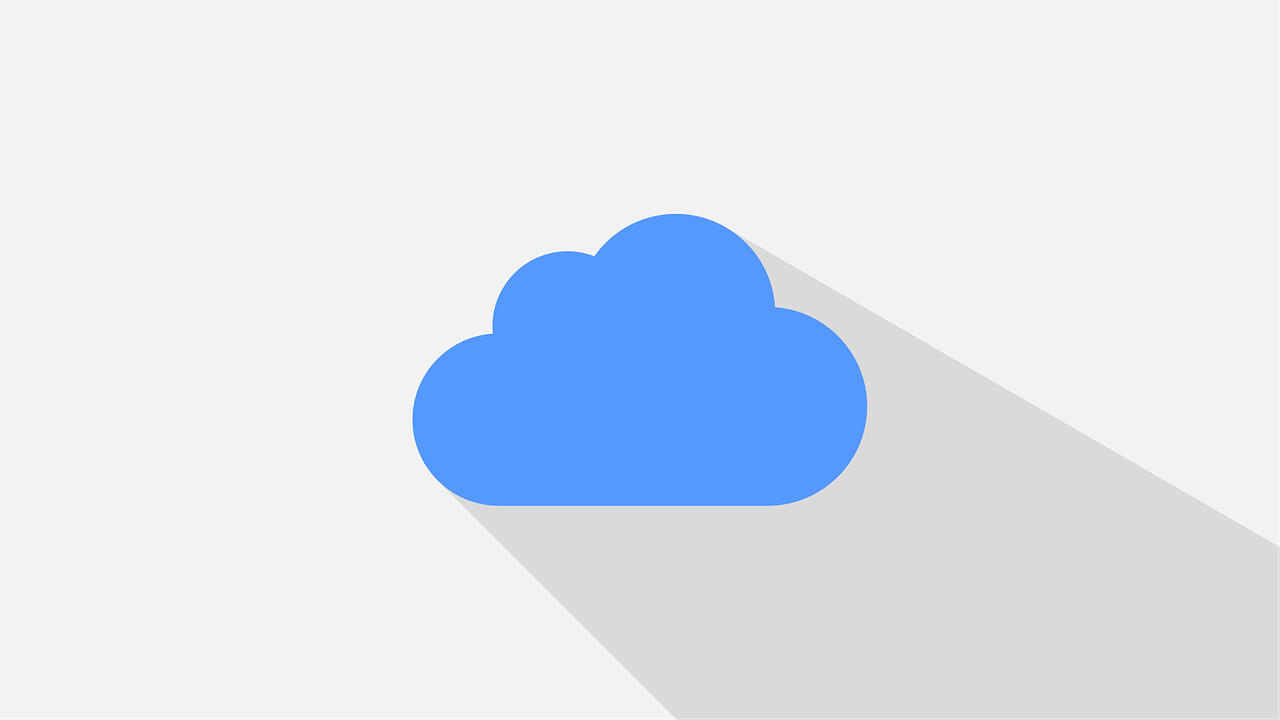
dispatchNestedScroll(int dxConsumed, int dyConsumed, int dxUnconsumed, int dyUnconsumed, int[] offsetInWindow)
: 分发滚动事件给父视图。
1.2 NestedScrollingParent接口
NestedScrollingParent
接口定义了父视图需要实现的方法,以响应子视图的嵌套滚动请求,主要方法包括:
onStartNestedScroll(View child, View target, int nestedScrollAxes)
: 开始嵌套滚动。
onStopNestedScroll(View target)
: 停止嵌套滚动。
onNestedScrollAccepted(View child, View target, int nestedScrollAxes)
: 接受嵌套滚动。
onNestedPreScroll(View target, int dx, int dy, int[] consumed)
: 预滚动回调。
2. NestedScrolling机制原理
嵌套滚动的工作原理可以概括为以下几个步骤:
1、启动嵌套滚动:子视图调用startNestedScroll
方法,表示希望开始一个嵌套滚动操作。
2、预滚动处理:父视图在onNestedPreScroll
方法中处理预滚动事件,返回未消费的距离。
3、滚动处理:子视图在dispatchNestedScroll
方法中分发滚动事件,父视图在onNestedScroll
方法中处理实际滚动。
4、停止嵌套滚动:子视图调用stopNestedScroll
方法,表示嵌套滚动结束。
整个过程通过一系列回调函数实现父子视图间的协调和滚动处理。
三、实战:实现一个简单的嵌套滚动
要实现一个简单的嵌套滚动,我们可以通过自定义一个LinearLayout来实现,以下是详细步骤:
1. 创建自定义LinearLayout类
我们需要创建一个继承自LinearLayout的自定义类,并实现NestedScrollingParent
和NestedScrollingChild
接口。
public class CustomLinearLayout extends LinearLayout implements NestedScrollingParent, NestedScrollingChild { private int mTotalUnconsumed; public CustomLinearLayout(Context context) { super(context); init(context); } public CustomLinearLayout(Context context, AttributeSet attrs) { super(context, attrs); init(context); } public CustomLinearLayout(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(context); } private void init(Context context) { setOrientation(LinearLayout.VERTICAL); setLayoutDirection( LAYOUT_DIRECTION RTL); } @Override public boolean startNestedScroll(int axes) { return super.startNestedScroll(axes); } @Override public void stopNestedScroll() { super.stopNestedScroll(); } @Override public boolean hasNestedScrollingParent() { return super.hasNestedScrollingParent(); } @Override public boolean dispatchTouchEvent(MotionEvent ev) { // Your touch event handling logic here return super.dispatchTouchEvent(ev); } }
在这个例子中,我们重写了必要的方法,并在构造函数中初始化了一些属性,我们需要实现嵌套滚动的相关方法。
实现嵌套滚动逻辑
在自定义的LinearLayout类中,我们需要实现NestedScrollingParent
和NestedScrollingChild
接口的方法,以下是详细的实现:
@Override public boolean onStartNestedScroll(View child, View target, int nestedScrollAxes) { return true; // Start the nested scroll } @Override public void onStopNestedScroll(View target) { // Stop the nested scroll } @Override public void onNestedScrollAccepted(View child, View target, int nestedScrollAxes) { // Handle the nested scroll acceptance } @Override public void onNestedPreScroll(View target, int dx, int dy, int[] consumed) { // Handle the pre-scroll } @Override public void onNestedScroll(View target, int dxConsumed, int dyConsumed, int dxUnconsumed, int dyUnconsumed) { // Handle the nested scroll event }
通过以上步骤,我们可以实现一个简单的自定义LinearLayout,支持基本的嵌套滚动功能,这个示例展示了如何通过实现相关接口来处理嵌套滚动的逻辑,实际应用中可能需要根据具体需求进行更多的定制和优化。
四、高级应用:使用CoordinatorLayout实现复杂布局滚动
CoordinatorLayout
是Android提供的一个强大布局管理器,专门用于协调多种滚动事件的布局,它通常与AppBarLayout
,RecyclerView
,FloatingActionButton
等配合使用,以实现复杂的滚动和动画效果,下面介绍如何使用CoordinatorLayout
实现嵌套滚动。
1. CoordinatorLayout简介
CoordinatorLayout
是一个非可视的Layout,它可以持有一个或多个子视图,并协调它们的滚动事件,它的主要作用是帮助实现材料设计中的滚动效果和动画效果。
2. 使用CoordinatorLayout实现嵌套滚动
我们将通过一个简单的例子展示如何使用CoordinatorLayout
实现嵌套滚动效果,假设我们有一个RecyclerView
和一个AppBarLayout
,我们希望在用户滚动RecyclerView
时,AppBarLayout
能够协同滚动。
Step 1: 定义布局文件
在res/layout
目录下创建一个新的XML布局文件activity_main.xml
:
<?xml version="1.0" encoding="utf-8"?> <androidx.coordinatorlayout.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <com.google.android.material.appbar.AppBarLayout android:id="@+id/appbar" android:layout_width="match_parent" android:layout_height="wrap_content"> <!-Your AppBarLayout content --> </com.google.android.material.appbar.AppBarLayout> <androidx.recyclerview.widget.RecyclerView android:id="@+id/recycler_view" android:layout_width="match_parent" android:layout_height="match_parent" app:layoutManager="androidx.recyclerview.widget.LinearLayoutManager"/> </androidx.coordinatorlayout.widget.CoordinatorLayout>
Step 2: 设置依赖项和适配器
在MainActivity
中设置RecyclerView
的适配器:
public class MainActivity extends AppCompatActivity { private RecyclerView recyclerView; private MyAdapter adapter; private List<String> data = new ArrayList<>(); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); recyclerView = findViewById(R.id.recycler_view); recyclerView.setLayoutManager(new LinearLayoutManager(this)); adapter = new MyAdapter(data); recyclerView.setAdapter(adapter); loadData(); } private void loadData() { for (int i = 0; i < 50; i++) { data.add("Item " + i); } adapter.notifyDataSetChanged(); }}
Step 3: 配置行为(Behavior)
为了让AppBarLayout
在滚动时有相应的动画效果,我们需要定义一个Behavior
并绑定到AppBarLayout
上,在res/values
目录下创建一个新的XML文件dimen.xml
:
<resources> <dimen name="appbar_scrolling_view_behavior_enable">true</dimen> </resources>
然后在代码中设置Behavior
:
CoordinatorLayout coordinatorLayout = findViewById(R.id.coordinator_layout); AppBarLayout appBarLayout = findViewById(R.id.appbar); appBarLayout.setBackgroundColor(Color.parseColor("#3F51B5")); // Set background color for visibility during scrolling
通过以上步骤,我们实现了一个简单的嵌套滚动示例,其中RecyclerView
和AppBarLayout
可以协同滚动,实际应用中可以根据具体需求进一步调整和优化布局及行为。
五、常见问题与解决方案
在实现嵌套滚动过程中,开发者可能会遇到各种问题,以下是一些常见问题及其解决方案:
滑动冲突问题及解决方法
在复杂的嵌套滚动布局中,滑动冲突是一个常见问题,当子视图和父视图都有滚动功能时,如何确保它们能正确响应用户的滑动手势?解决方案包括:
明确滑动责任:确定哪个视图应该处理滑动事件,避免多个视图同时处理同一滑动事件。
使用嵌套滚动机制:通过实现NestedScrollingParent
和NestedScrollingChild
接口,让父视图和子视图协调滑动事件。
调整滑动优先级:使用requestDisallowInterceptTouchEvent
方法调整滑动优先级,确保特定视图优先处理滑动事件。
2. NestedScrolling机制中的回调函数说明及注意事项
在实现嵌套滚动时,理解各个回调函数的作用非常重要:
onStartNestedScroll:当子视图希望开始嵌套滚动时调用,父视图需要在这个方法中决定是否接受嵌套滚动请求。
onNestedScrollAccepted:父视图调用此方法表示接受嵌套滚动请求,在此方法中可以进行一些初始化操作。
onStopNestedScroll:当嵌套滚动结束时调用,父视图需要在这个方法中释放资源或进行清理工作。
onNestedPreScroll:父视图在处理滑动事件之前调用此方法,子视图可以在这个方法中预处理滑动事件。
onNestedScroll:父视图在处理滑动事件之后调用此方法,子视图可以在这个方法中进一步处理滑动事件。
注意事项:确保在适当的时机调用这些回调函数,以避免滑动冲突或异常情况发生,要注意保存和恢复视图的状态,以确保滚动的一致性和正确性。
六、归纳与展望
本文详细介绍了Android嵌套滚动的基础知识、实战案例以及常见问题的解决方案,通过实现一个简单的自定义LinearLayout和支持复杂布局的CoordinatorLayout示例,读者可以掌握嵌套滚动的基本概念和应用技巧,在实际开发中,嵌套滚动可以帮助实现更复杂、更流畅的用户界面交互效果,未来随着Android技术的不断发展,嵌套滚动机制也将进一步完善和优化,为开发者提供更多强大的工具和方法来构建优秀的应用程序。
到此,以上就是小编对于“android嵌套滚动入门实践”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1292248.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复