Android底部导航
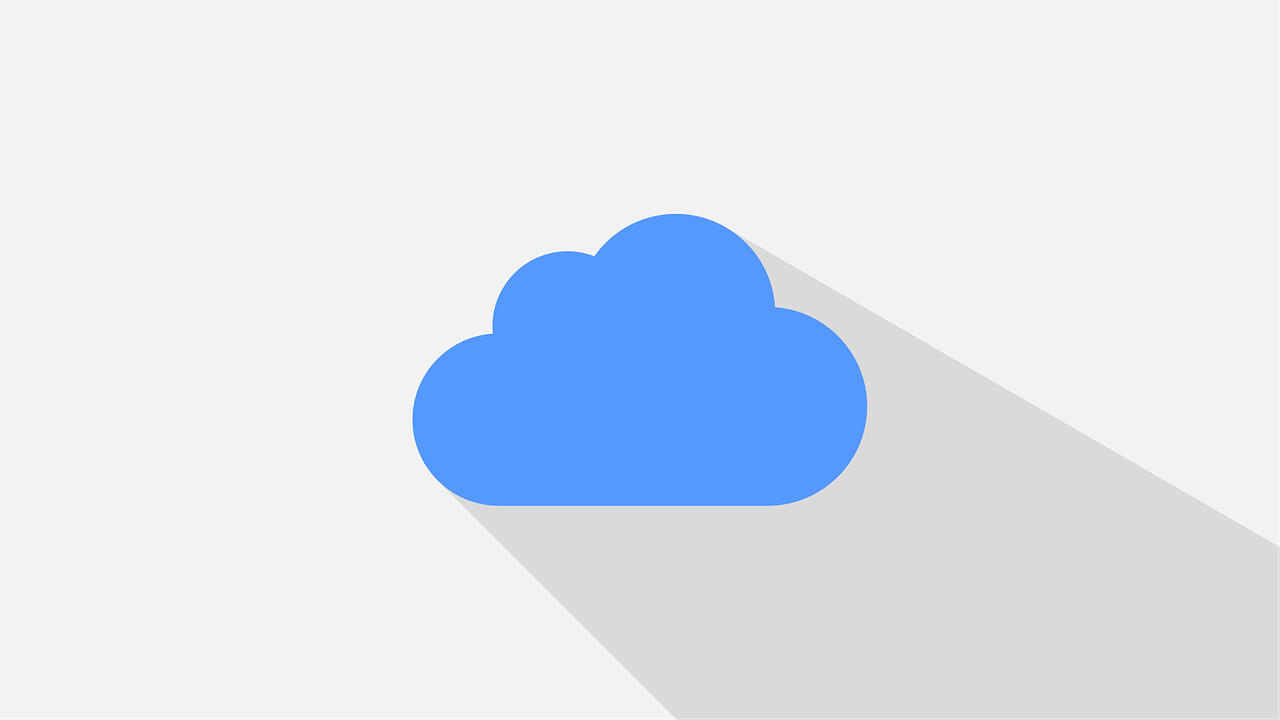
一、背景与概念
底部导航
底部导航(BottomNavigation)在Android应用中是一种常见的界面模式,通常用于提供快速访问应用的主要功能区域,它位于屏幕底部,便于用户在各个功能模块之间进行切换。
这种导航方式可以显著提升用户体验,因为它提供了一个清晰且易于访问的导航结构。
常见应用场景
社交媒体应用:如Facebook、Instagram等,通过底部导航实现动态、探索、消息和个人信息页之间的切换。
电子商务应用:如Amazon、淘宝等,通过底部导航实现商品浏览、购物车、订单和个人中心等功能的切换。
内容流媒体应用:如Netflix、Spotify等,通过底部导航实现视频、音频、搜索和账户设置等功能的切换。
二、底部导航实现方法
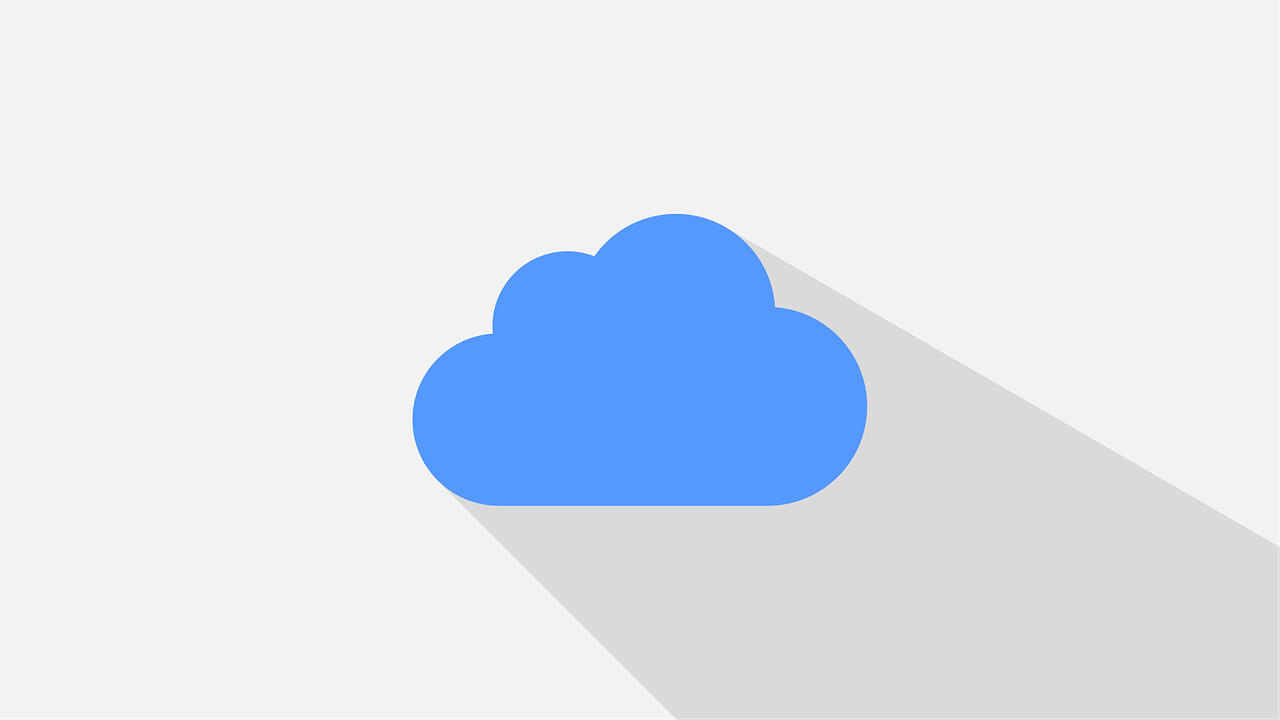
1. 使用BottomNavigationView
1.1 添加依赖
在项目的build.gradle文件中添加Material Design库的依赖:
implementation 'com.google.android.material:material:1.9.0'
1.2 布局文件配置
在res/layout/activity_main.xml中定义BottomNavigationView:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <FrameLayout android:id="@+id/fragment_container" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1"/> <com.google.android.material.bottomnavigation.BottomNavigationView android:id="@+id/bottom_navigation" android:layout_width="match_parent" android:layout_height="wrap_content" app:menu="@menu/bottom_nav_menu"/> </LinearLayout>
1.3 菜单资源文件
在res/menu/bottom_nav_menu.xml中定义菜单项:
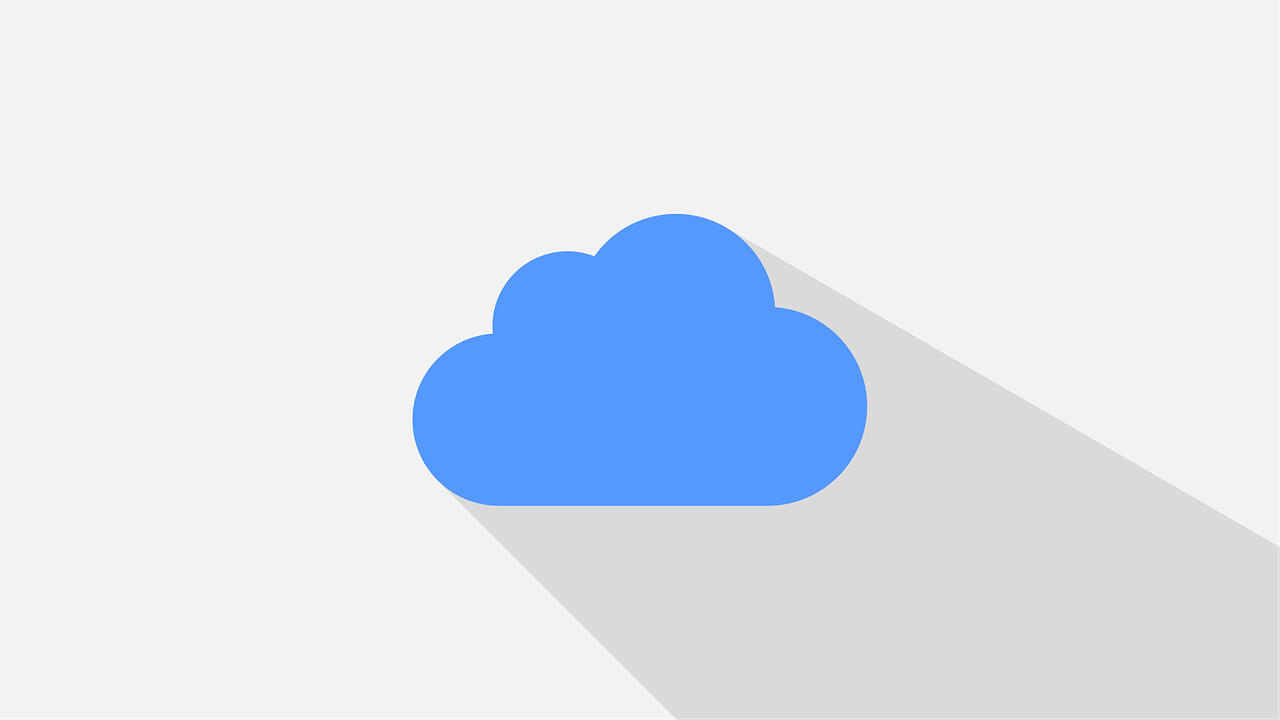
<?xml version="1.0" encoding="utf-8"?> <menu xmlns:android="http://schemas.android.com/apk/res/android"> <item android:id="@+id/navigation_home" android:icon="@drawable/ic_home" android:title="Home"/> <item android:id="@+id/navigation_dashboard" android:icon="@drawable/ic_dashboard" android:title="Dashboard"/> <item android:id="@+id/navigation_notifications" android:icon="@drawable/ic_notifications" android:title="Notifications"/> </menu>
1.4 Fragment实现
创建多个Fragment类,例如HomeFragment、DashboardFragment和NotificationsFragment,并在各自的onCreateView方法中定义UI。
1.5 活动主类实现
在MainActivity中设置底部导航的点击事件监听器:
BottomNavigationView bottomNavigationView = findViewById(R.id.bottom_navigation); bottomNavigationView.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() { @Override public boolean onNavigationItemSelected(@NonNull MenuItem item) { Fragment selectedFragment = null; switch (item.getItemId()) { case R.id.navigation_home: selectedFragment = new HomeFragment(); break; case R.id.navigation_dashboard: selectedFragment = new DashboardFragment(); break; case R.id.navigation_notifications: selectedFragment = new NotificationsFragment(); break; } getSupportFragmentManager().beginTransaction().replace(R.id.fragment_container, selectedFragment).commit(); return true; } });
2. 使用ViewPager + TabLayout
2.1 布局文件配置
在res/layout/activity_main.xml中定义ViewPager和TabLayout:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <com.google.android.material.tabs.TabLayout android:id="@+id/tab_layout" android:layout_width="match_parent" android:layout_height="wrap_content"/> <androidx.viewpager.widget.ViewPager android:id="@+id/view_pager" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1"/> </LinearLayout>
2.2 创建适配器
创建一个ViewPager适配器,继承FragmentPagerAdapter或FragmentStatePagerAdapter:
public class ViewPagerAdapter extends FragmentPagerAdapter { private String[] tabTitles = new String[]{"Home", "Dashboard", "Notifications"}; private Fragment[] fragments = new Fragment[]{new HomeFragment(), new DashboardFragment(), new NotificationsFragment()}; public ViewPagerAdapter(FragmentManager fm) { super(fm); } @Override public Fragment getItem(int position) { return fragments[position]; } @Override public int getCount() { return fragments.length; } @Nullable @Override public CharSequence getPageTitle(int position) { return tabTitles[position]; } }
2.3 活动主类实现
在MainActivity中设置ViewPager和TabLayout:
ViewPager viewPager = findViewById(R.id.view_pager); TabLayout tabLayout = findViewById(R.id.tab_layout); ViewPagerAdapter adapter = new ViewPagerAdapter(getSupportFragmentManager()); viewPager.setAdapter(adapter); tabLayout.setupWithViewPager(viewPager);
2.4 ViewPager与Fragment交互
在每个Fragment中定义具体的UI和逻辑。
自定义底部导航栏
3.1 布局文件配置
在res/layout/activity_main.xml中定义自定义底部导航栏:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <FrameLayout android:id="@+id/fragment_container" android:layout_width="match_parent" android:layout_height="0dp" android:layout_above="@id/custom_bottom_navigation" android:layout_weight="1"/> <LinearLayout android:id="@+id/custom_bottom_navigation" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:orientation="horizontal"> <!-Custom buttons here --> </LinearLayout> </RelativeLayout>
3.2 自定义按钮实现
在custom_bottom_navigation中添加自定义按钮:
<Button android:id="@+id/btn_home" android:layout_width="0dp" android:layout_height="match_parent" android:layout_weight="1" android:background="@drawable/button_selector" android:text="Home"/> <!-Add more buttons similarly -->
3.3 活动主类实现
在MainActivity中为每个按钮设置点击事件:
Button btnHome = findViewById(R.id.btn_home); btnHome.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { getSupportFragmentManager().beginTransaction().replace(R.id.fragment_container, new HomeFragment()).commit(); } }); // Set similar listeners for other buttons
3.4 自定义样式和动画效果
使用drawable资源创建按钮选择器和不同的状态样式,例如button_selector.xml:
<selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@color/colorPrimaryDark" android:state_pressed="true"/> <item android:drawable="@color/colorAccent"/> </selector>
三、底部导航最佳实践与优化策略
选择合适的导航模式
根据应用的需求选择合适的底部导航实现方式,BottomNavigationView适用于简单的页面切换,ViewPager + TabLayout适用于页面滑动切换,而自定义底部导航栏则适用于需要高度定制的场景。
确保清晰的视觉层次结构
确保底部导航在不同设备和屏幕尺寸上的可见性和可用性,合理使用颜色、图标和标签来区分不同的导航项。
优化性能与体验
避免在底部导航切换时执行耗时操作,使用Fragment的懒加载技术提高性能,确保导航切换的流畅性,减少不必要的重绘和布局更新。
4. 遵循Material Design指南
参考Google的Material Design指南,确保底部导航的设计符合最新的设计趋势和用户习惯,使用统一的图标风格和适当的间距。
测试与反馈循环
在多种设备和操作系统版本上进行广泛测试,收集用户反馈并进行迭代优化,关注用户的实际操作流程,发现并解决潜在的问题。
小伙伴们,上文介绍了“Android底部导航”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1292157.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复