Android底部切换标签
一、背景介绍
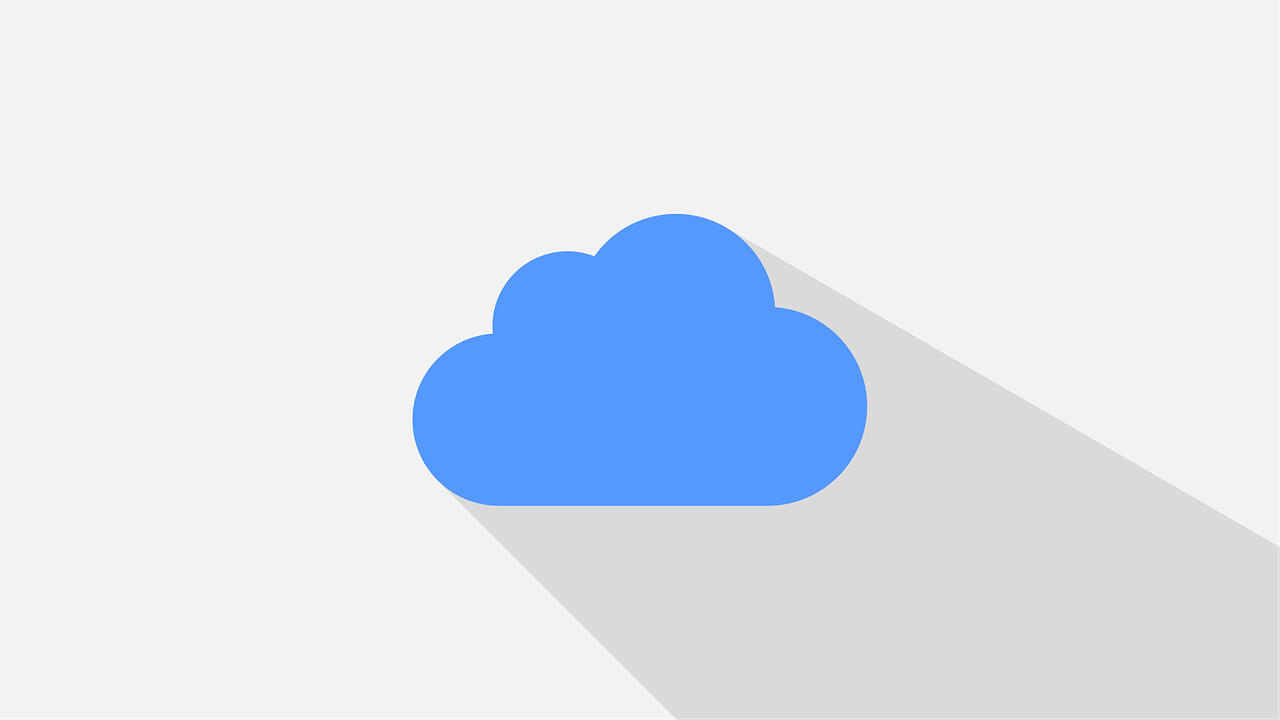
在现代Android应用开发中,底部切换标签是一种常见的界面设计模式,用于在不同功能模块或页面之间进行快速切换,这种设计模式不仅提高了用户操作的便捷性,还使得应用结构更加清晰,本文将详细介绍如何在Android应用中实现底部切换标签的功能,包括布局设计、Fragment管理以及事件处理等方面。
二、布局设计
1. 顶部导航栏布局
顶部导航栏通常包含应用的标题、Logo或其他标识性元素,它位于窗口的顶部,作为整个界面的视觉焦点之一。
<RelativeLayout android:id="@+id/top_tab" android:layout_width="match_parent" android:layout_height="50dip" android:background="@color/topbar_bg"> <ImageView android:id="@+id/iv_logo" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:focusable="false" android:src="@drawable/zhidao_logo" android:contentDescription="@null"/> </RelativeLayout>
2. 中部显示内容布局
中部显示内容布局用于展示当前选中标签对应的内容,这部分通常是一个FrameLayout
,用于承载不同的Fragment。
<FrameLayout android:id="@+id/content_layout" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_above="@+id/ll_bottom_tab" android:layout_below="@+id/top_tab" android:orientation="vertical"> <View android:id="@+id/line" android:layout_width="match_parent" android:layout_height="1dp" android:layout_above="@id/ll_bottom_tab" android:background="@color/line"/> </FrameLayout>
3. 底部标签布局
底部标签布局是实现标签切换的关键部分,它通常由一个水平线性布局(LinearLayout
)组成,其中包含多个标签项,每个标签项由一个图标和文本组成,用户可以点击不同的标签项来切换内容。
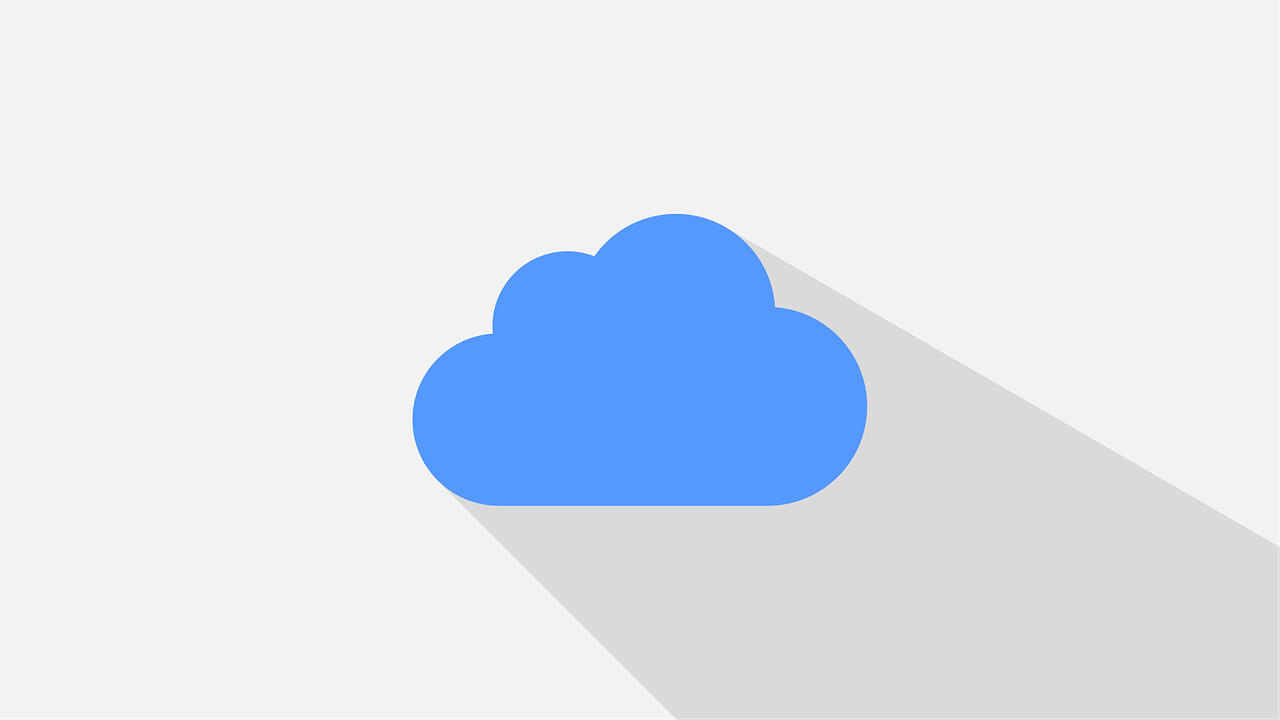
<LinearLayout android:id="@+id/ll_bottom_tab" android:layout_width="match_parent" android:layout_height="54dp" android:layout_alignParentBottom="true" android:gravity="center_vertical" android:orientation="horizontal"> <RelativeLayout android:id="@+id/rl_know" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1.0"> <ImageView android:id="@+id/iv_know" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:src="@drawable/btn_know_nor"/> <TextView android:id="@+id/tv_know" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/iv_know" android:layout_centerHorizontal="true" android:text="@string/bottom_tab_know" android:textColor="@color/bottomtab_normal" android:textSize="12sp"/> </RelativeLayout> <!-其他标签项类似 --> </LinearLayout>
三、Fragment管理
Fragment是Android中的一个独立UI组件,它可以嵌入到Activity中,实现模块化的UI设计,在底部切换标签的应用中,每个标签对应一个Fragment,通过切换Fragment来实现内容的切换。
1. 定义Fragment
需要定义与底部标签对应的Fragment,每个Fragment都有一个独立的布局文件,用于定义其UI结构。
public class KnowFragment extends Fragment { @Nullable @Override public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { return inflater.inflate(R.layout.main_tab1_fragment, container, false); } }
2. 添加Fragment到Activity
在Activity中,使用FragmentManager
来添加和管理Fragment,初始时,可以添加第一个Fragment到FrameLayout
容器中。
FragmentManager fragmentManager = getSupportFragmentManager(); fragmentManager.beginTransaction() .replace(R.id.content_layout, new KnowFragment()) .commit();
3. 切换Fragment
当用户点击底部标签时,需要根据所点击的标签切换到相应的Fragment,这可以通过为每个标签设置点击事件监听器来实现。
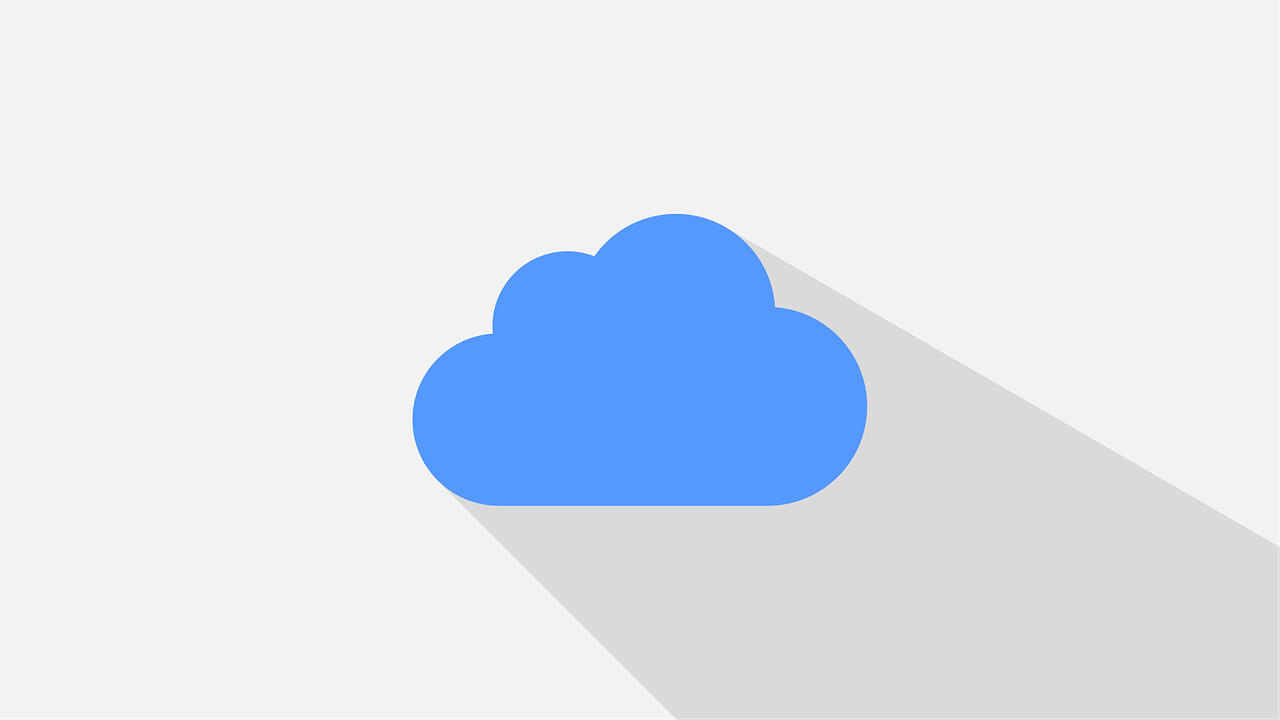
llBottomTab.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { switch (v.getId()) { case R.id.rl_know: fragmentManager.beginTransaction() .replace(R.id.content_layout, new KnowFragment()) .commit(); break; case R.id.rl_want_know: fragmentManager.beginTransaction() .replace(R.id.content_layout, new WantKnowFragment()) .commit(); break; // 其他标签类似 } } });
四、事件处理
事件处理是底部切换标签功能的核心部分,通过监听用户的点击事件,可以动态地切换Fragment,并更新底部标签的状态(如改变图标颜色、文字颜色等)。
1. 设置点击事件监听器
为每个底部标签设置点击事件监听器,当用户点击某个标签时,触发相应的事件处理逻辑。
llBottomTab.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { switch (v.getId()) { case R.id.rl_know: // 切换到KnowFragment break; case R.id.rl_want_know: // 切换到WantKnowFragment break; // 其他标签类似 } } });
2. 更新标签状态
在切换Fragment的同时,需要更新底部标签的状态,以反映当前的选择,这可以通过改变图标和文字的颜色来实现。
private void updateTabState(int selectedTabId) { for (int i = 0; i < llBottomTab.getChildCount(); i++) { View tab = llBottomTab.getChildAt(i); if (tab.getId() == selectedTabId) { // 选中状态 ((ImageView) tab.findViewById(R.id.iv_know)).setImageResource(R.drawable.btn_know_sel); ((TextView) tab.findViewById(R.id.tv_know)).setTextColor(getResources().getColor(R.color.bottomtab_select)); } else { // 未选中状态 ((ImageView) tab.findViewById(R.id.iv_know)).setImageResource(R.drawable.btn_know_nor); ((TextView) tab.findViewById(R.id.tv_know)).setTextColor(getResources().getColor(R.color.bottomtab_normal)); } } }
底部切换标签是Android应用中一种常见的界面设计模式,它通过简单的布局设计和Fragment管理实现了复杂的功能,通过本文的介绍,读者可以了解到底部切换标签的基本实现方法和原理,并可以根据实际需求进行定制和扩展,随着Android技术的不断发展,底部切换标签的实现方式也将更加多样化和灵活化。
各位小伙伴们,我刚刚为大家分享了有关“Android底部切换标签”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1291785.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复