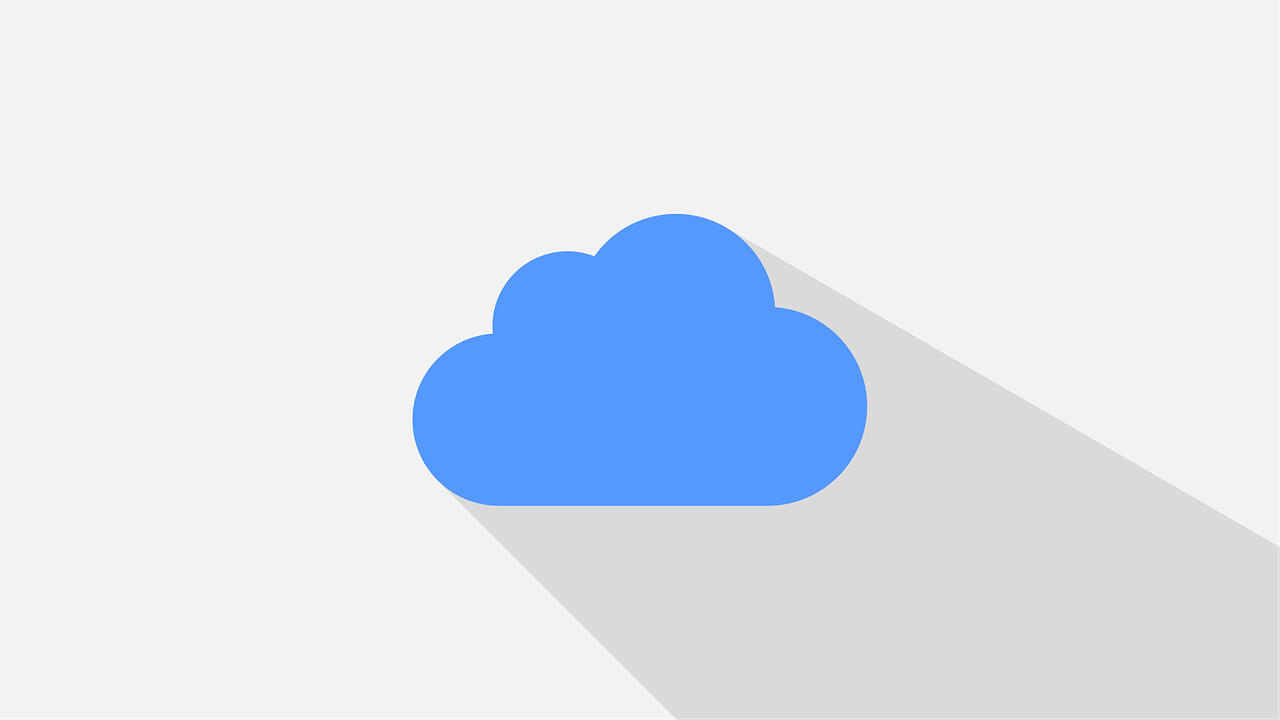
在Android应用开发中,经常需要与Excel文件进行数据的读取和写入操作,本文将详细介绍如何在Android平台上实现Excel文件的读取功能,并提供相应的源代码示例,以下是几种常用的方法:
使用JXL库读取Excel文件
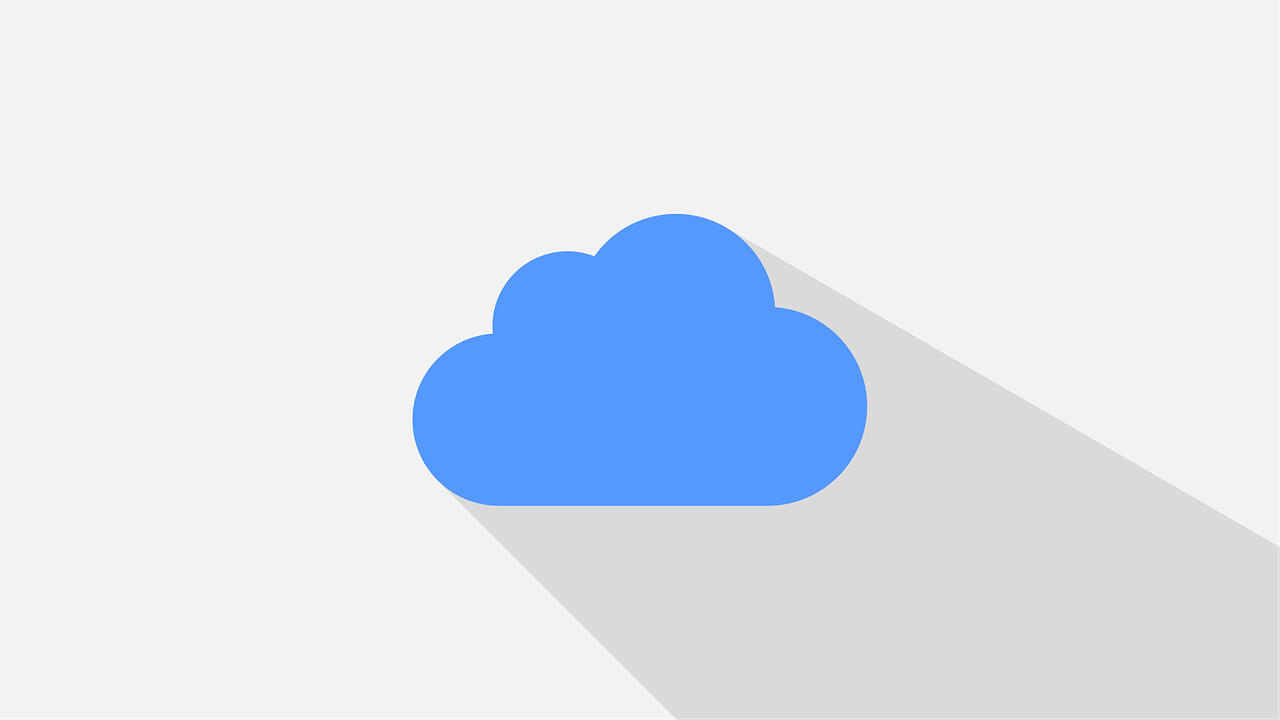
添加依赖
需要在项目的build.gradle
文件中添加JXL库的依赖项:
implementation 'net.sourceforge.jexcelapi:jxl:2.6.12'
读取Excel文件示例代码
以下是一个使用JXL库读取Excel文件的示例代码:
import android.os.Bundle; import android.util.Log; import jxl.*; public class ReadExcel extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); readExcel(); } public void readExcel() { try { String logFilePath = Environment.getExternalStorageDirectory() + File.separator + "Visitor" + File.separator + "test.xls"; File file = new File(logFilePath); Log.e("yy", "file=" + file.getAbsolutePath()); InputStream is = new FileInputStream(file); Workbook book = Workbook.getWorkbook(is); book.getNumberOfSheets(); Sheet sheet = book.getSheet(0); int rows = sheet.getRows(); for (int i = 1; i < rows; ++i) { String name = (sheet.getCell(0, i)).getContents(); String department = (sheet.getCell(1, i)).getContents(); String company = (sheet.getCell(2, i)).getContents(); String phone = (sheet.getCell(3, i)).getContents(); Log.e("yy", "第" + i + "行数据=" + name + "," + department + "," + company + "," + phone); } book.close(); } catch (Exception e) { Log.e("yy", "e" + e); } } }
注意:此方法仅支持97-2003(xls)版本,不支持2007以上版本(xlsx)。
2. 使用Apache POI库读取Excel文件
添加依赖
在build.gradle
文件中添加Apache POI库的依赖项:
implementation 'org.apache.poi:poi:5.0.0' implementation 'org.apache.poi:poi-ooxml:5.0.0'
读取Excel文件示例代码
以下是一个使用Apache POI库读取Excel文件的示例代码:
import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; import org.apache.poi.ss.usermodel.*; import org.apache.poi.xssf.usermodel.XSSFWorkbook; import java.io.File; import java.io.FileInputStream; import java.io.IOException; public class ReadExcel extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); readExcel(); } private void readExcel() { try { String filePath = getExternalFilesDir(null).getAbsolutePath() + "/test.xlsx"; FileInputStream fis = new FileInputStream(filePath); Workbook workbook = new XSSFWorkbook(fis); Sheet sheet = workbook.getSheetAt(0); for (Row row : sheet) { for (Cell cell : row) { switch (cell.getCellType()) { case STRING: System.out.print(cell.getStringCellValue() + "t"); break; case NUMERIC: System.out.print(cell.getNumericCellValue() + "t"); break; default: System.out.print("未知类型t"); break; } } System.out.println(); } workbook.close(); fis.close(); } catch (IOException e) { e.printStackTrace(); } } }
注意:此方法支持97-2003(xls)和2007以上版本(xlsx)。
3. 使用EasyExcel库读取Excel文件
添加依赖
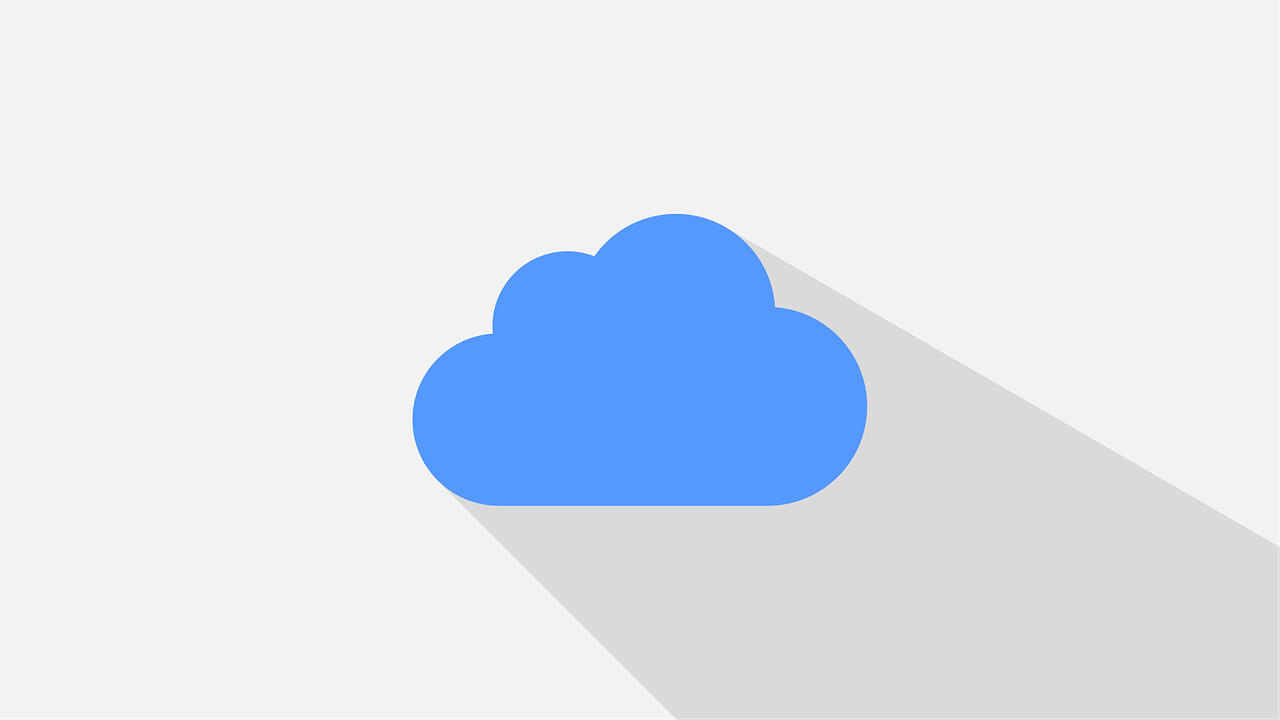
在build.gradle
文件中添加EasyExcel库的依赖项:
implementation 'com.alibaba:easyexcel:2.4.3'
读取Excel文件示例代码
以下是一个使用EasyExcel库读取Excel文件的示例代码:
import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; import com.alibaba.excel.EasyExcel; import com.alibaba.excel.read.listener.ReadListener; import com.alibaba.excel.read.metadata.ReadSheet; import java.util.List; import java.util.ArrayList; public class ReadExcel extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); readExcel(); } private void readExcel() { String fileName = getExternalFilesDir(null).getAbsolutePath() + "/test.xlsx"; EasyExcel.read(fileName, MyData.class, new ReadListener<MyData>() { @Override public void invoke(MyData data, AnalysisContext context) { System.out.println("读取到一条数据:" + data); } @Override public void doAfterAllAnalysed(AnalysisContext context) { System.out.println("所有数据解析完成"); } }).sheet().doRead(); } public static class MyData { @ExcelProperty("姓名") private String name; @ExcelProperty("年龄") private int age; // 构造函数、getter 和 setter 方法省略 } }
注意:此方法支持复杂的数据结构,包括嵌套的对象、Map、List等,并提供了数据转换和校验的功能。
在Android应用中读取Excel文件是一项常见的需求,通过使用JXL、Apache POI或EasyExcel等第三方库,可以方便地实现这一功能,根据具体的需求选择合适的库和方法,可以提高开发效率并简化代码逻辑。
到此,以上就是小编对于“Android应用读取Excel文件的方法”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1290652.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复