Android实现蓝牙通信
一、应用
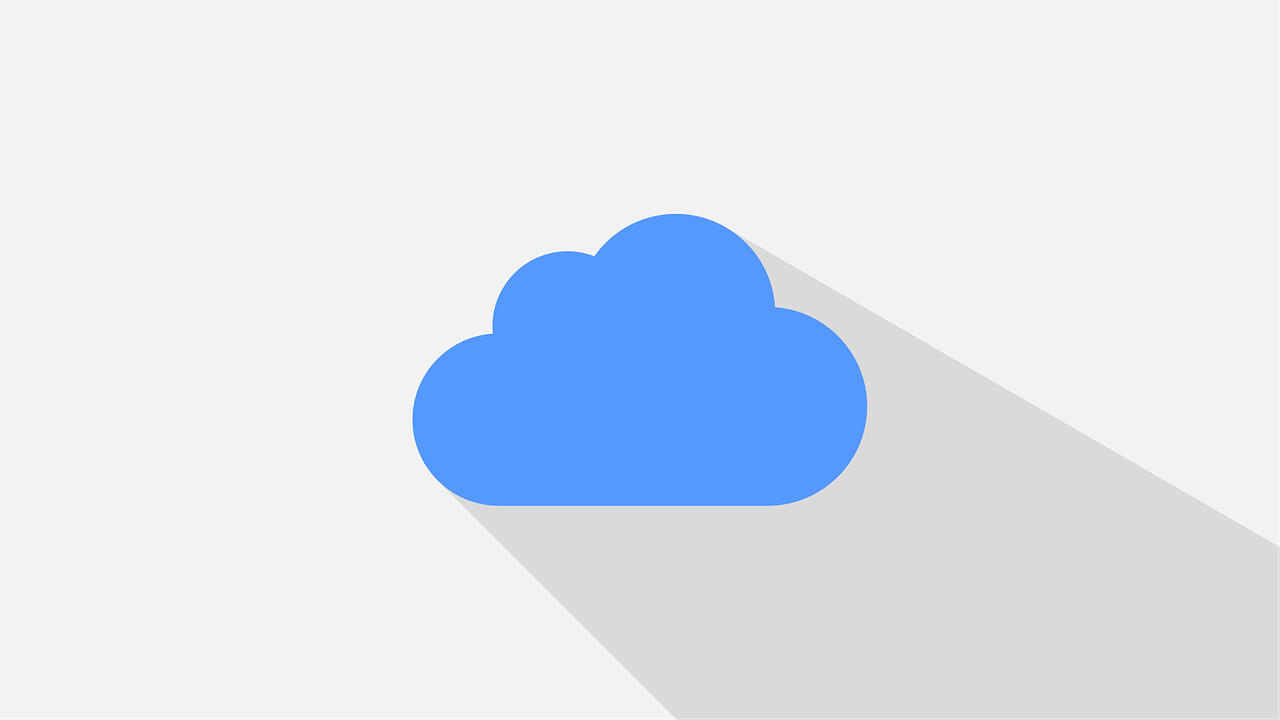
在Android设备上创建一个简单的DEMO应用,该应用使用蓝牙技术实现设备间的数据传输,这意味着我们需要在两个Android设备之间建立蓝牙连接,并通过这个连接发送和接收数据,为了简化开发过程,我们将使用Android SDK提供的蓝牙API。
二、开发环境设置
在开始编写代码之前,需要确保你的开发环境已经设置好,这包括安装Android Studio和配置合适的Android SDK,确保你的设备支持蓝牙,并且已经开启了蓝牙功能。
三、代码实现
1. 权限配置
在你的AndroidManifest.xml
文件中添加必要的权限:
<uses-permission android:name="android.permission.BLUETOOTH" /> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
2. 布局文件
创建一个新的Activity并在其布局文件中添加必要的UI元素,如文本框用于显示接收到的数据:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/title_left_text" style="?android:attr/windowTitleStyle" android:layout_width="0dp" android:layout_height="match_parent" android:layout_alignParentLeft="true" android:layout_weight="1" android:gravity="left" android:ellipsize="end" android:singleLine="true" /> <TextView android:id="@+id/title_right_text" android:layout_width="0dp" android:layout_height="match_parent" android:layout_alignParentRight="true" android:layout_weight="1" android:gravity="right" android:textColor="#fff" /> <ListView android:id="@+id/in" android:layout_width="match_parent" android:layout_height="match_parent" android:stackFromBottom="true" android:transcriptMode="alwaysScroll" android:layout_weight="1" /> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="wrap_content" > <EditText android:id="@+id/edit_text_out" android:layout_width="wrap_content" android:layout_height="wrap_content"/> </LinearLayout> </LinearLayout>
3. 蓝牙连接逻辑
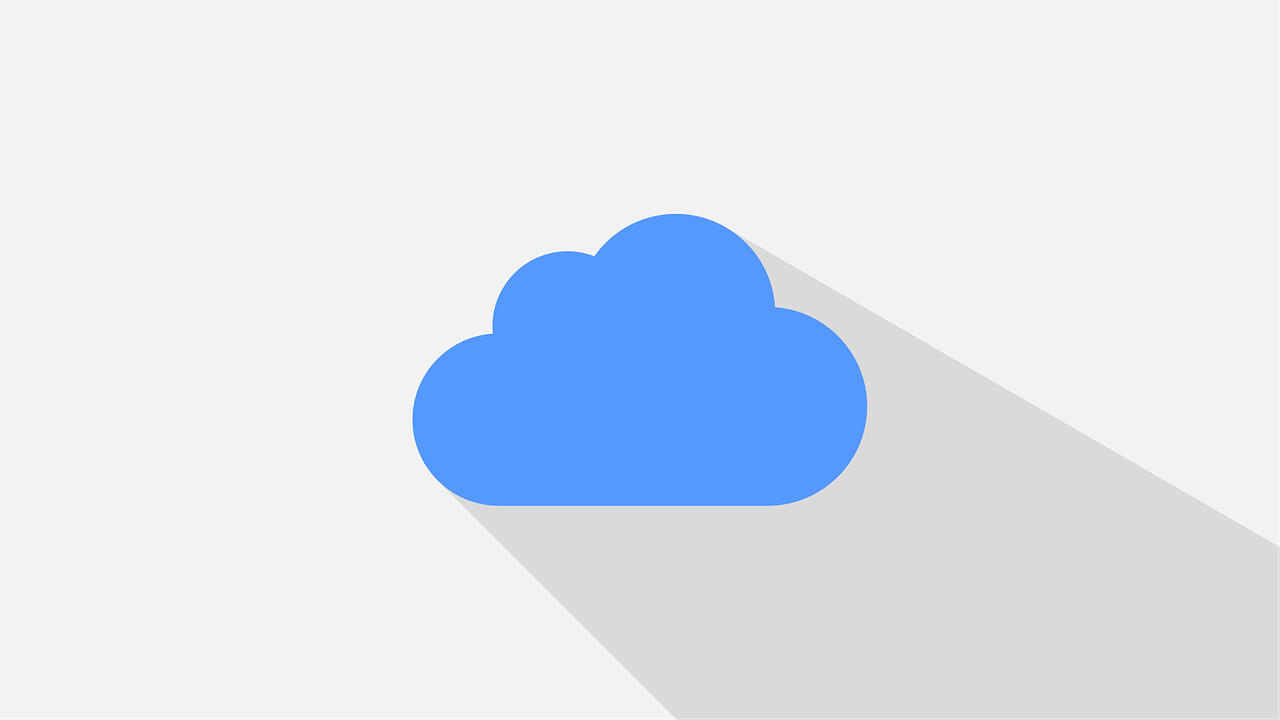
在Activity中实现蓝牙连接逻辑,这包括打开蓝牙、搜索设备、建立连接等步骤,你可以使用BluetoothAdapter
类提供的API来完成这些操作。
3.1 初始化蓝牙适配器
获取默认的蓝牙适配器并启用蓝牙功能:
BluetoothAdapter mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); if (!mBluetoothAdapter.isEnabled()) { Intent enabler = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); startActivityForResult(enabler, REQUEST_ENABLE); }
3.2 搜索设备
搜索周围的蓝牙设备并显示它们的相关信息:
mBluetoothAdapter.startDiscovery();
3.3 配对与连接
与指定的蓝牙设备配对并完成绑定:
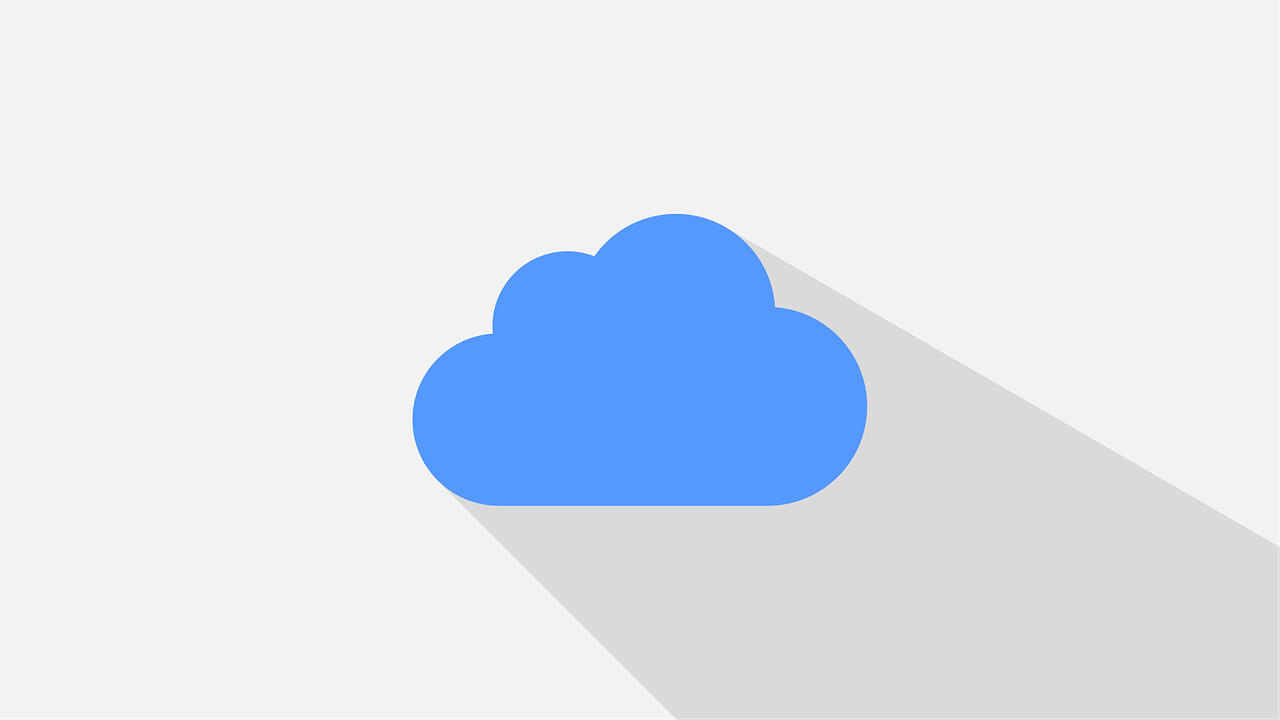
Set<BluetoothDevice> devices = mBluetoothAdapter.getBondedDevices(); // Example: Connect to a specific device named "Galaxy Nexus" for (BluetoothDevice device : devices) { if ("Galaxy Nexus".equals(device.getName())) { try { Method method = device.getClass().getMethod("createRfcommSocket", new Class[]{int.class}); socket = (BluetoothSocket) method.invoke(device, 1); socket.connect(); } catch (Exception e) { e.printStackTrace(); } } }
3.4 发送数据
通过套接字发送数据:
try { OutputStream outputStream = bluetoothSocket.getOutputStream(); outputStream.write("Hello, World!".getBytes()); } catch (IOException e) { e.printStackTrace(); }
3.5 接收数据
从输入流中读取数据:
try { InputStream inputStream = bluetoothSocket.getInputStream(); byte[] buffer = new byte[1024]; int bytesRead = inputStream.read(buffer); String receivedData = new String(buffer, 0, bytesRead); textView.setText(receivedData); } catch (IOException e) { e.printStackTrace(); }
四、测试与调试
完成代码编写后,你可以通过运行两个设备上的应用来进行测试,确保两个设备都开启了蓝牙,并处于可被发现的模式,它们应该能够互相找到对方并建立连接,一旦连接建立,你可以在其中一个设备上发送数据,并在另一个设备上接收数据,在测试过程中,请注意处理可能出现的异常情况,如连接失败、数据传输中断等,你可以使用Logcat来查看应用运行时的日志信息,以便进行调试。
以上就是关于“android实现蓝牙通信”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!
原创文章,作者:未希,如若转载,请注明出处:https://www.kdun.com/ask/1290351.html
本网站发布或转载的文章及图片均来自网络,其原创性以及文中表达的观点和判断不代表本网站。如有问题,请联系客服处理。
发表回复